π Securing Your GitHub Repositories: Handling API Key Exposure and Push Protection Errors
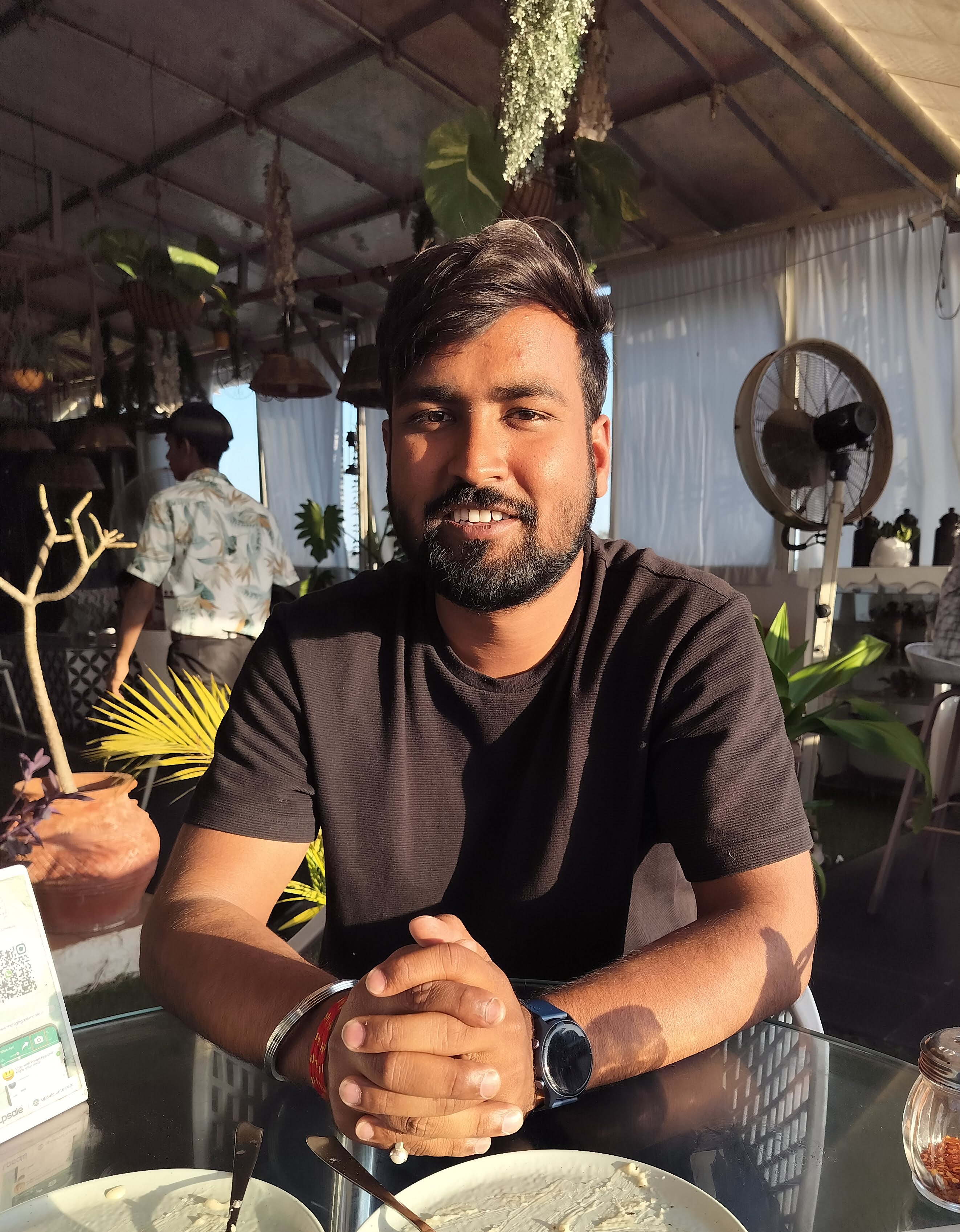

While working on a backend API for an AI-powered application, I encountered a GitHub Push Protection error that blocked my push due to an exposed API token in the code. This incident gave me a deeper understanding of GitHubβs security mechanisms and highlighted the importance of securing secrets in repositories.
In this blog, Iβll cover:
π― What GitHub Push Protection is and how it works
β οΈ How I detected and removed exposed secrets
π οΈ Techniques to clean Git history and prevent future leaks
π₯ Best practices to safeguard API tokens and sensitive information
π― The Problem: Push Blocked Due to Exposed API Key
During a routine push to GitHub, I encountered the following error:
vbnetCopyEditremote: error: GH013: Repository rule violations found for refs/heads/master.
remote: - GITHUB PUSH PROTECTION
remote: Resolve the following violations before pushing again.
remote:
remote: - Push cannot contain secrets
remote: - Hugging Face User Access Token found in backend/index.js:43
β Root Cause:
The culprit? An API token was inadvertently hard-coded into my index.js
file:
javascriptCopyEditconst hfToken = "hf_xxxYourAccessTokenxxx"; // Exposed Token
GitHub flagged and blocked the push because it detected a secret format matching a Hugging Face User Access Token. This security mechanism, known as Push Protection, prevented me from inadvertently pushing sensitive information to a public or private repository.
π Understanding GitHub Push Protection
π§ What is Push Protection?
Push Protection is a security feature provided by GitHubβs Secret Scanning functionality. It automatically blocks commits and pushes that contain sensitive information such as API tokens, access keys, and credentials.
π How It Works:
Pre-Push Detection: Before pushing, GitHub scans the commit history for any identifiable secrets.
Blocking the Push: If a known secret format is detected, the push is rejected to prevent leaking sensitive information.
Notification and Action: GitHub notifies the user with the exact location of the secret and provides a link to unblock the push (if absolutely necessary).
π₯ Common Secrets Detected:
AWS Access Keys
GitHub Personal Access Tokens
Hugging Face API Tokens
Stripe API Keys
Slack Webhooks
Twilio API Keys
π οΈ Step-by-Step Solution: How I Fixed It
After realizing the mistake, I took the following steps to remove the exposed token and clean my commit history.
π Step 1: Remove the Token and Use Environment Variables
The first step was to replace the hardcoded token with an environment variable. I updated the code to dynamically load the token:
javascriptCopyEdit// Load token securely from environment variables
const hfToken = process.env.HF_TOKEN;
Then, I created a .env
file to store the token securely:
iniCopyEditHF_TOKEN=hf_xxxYourAccessTokenxxx
I also updated the .gitignore
file to ensure that .env
and other sensitive files were not tracked by Git:
bashCopyEdit# Ignore .env files
.env
π Step 2: Cleaning Git History to Remove Exposed Token
Simply removing the token from the file is not enough because the sensitive information remains in the commit history. I needed to rewrite the Git history to clean the token.
β‘ Option 1: Using git filter-repo
To clean up the commit history, I used git filter-repo
, a more modern and faster alternative to git filter-branch
.
β Installation:
bashCopyEditpip install git-filter-repo
β Remove the File or Specific Token:
bashCopyEditgit filter-repo --path backend/index.js --replace-text <(echo "hf_xxxYourAccessTokenxxx==>REMOVED")
β Force Push the Cleaned History:
bashCopyEditgit push origin master --force
β‘ Option 2: Using BFG Repo-Cleaner (Alternative)
If you prefer another option, BFG Repo-Cleaner is a widely used tool that performs a similar task efficiently.
π Installation:
Download the JAR file from BFG Repo-Cleaner.
π Run BFG to Clean Secrets:
bashCopyEditbfg --delete-files backend/index.js
π Force Push Cleaned History:
bashCopyEditgit push origin master --force
π Step 3: Revoke and Regenerate the API Token
Since the token had been exposed, I immediately revoked it from my Hugging Face account and generated a new one. Itβs critical to revoke any compromised token to prevent unauthorized access.
π Generate a New Token
π¨ Preventing Future Secret Leaks: Best Practices
To avoid facing similar issues in the future, I implemented the following best practices.
β 1. Use Environment Variables for Secrets
Always load sensitive information such as API tokens, database credentials, and access keys from environment variables or secret management tools.
β
2. Add Sensitive Files to .gitignore
Ensure that sensitive files, such as .env
and configuration files, are listed in .gitignore
to prevent them from being tracked by Git.
bashCopyEdit# Ignore environment variables
.env
β 3. Enable Push Protection for Private Repositories
Push Protection is enabled by default for public repositories, but it can also be manually enabled for private repositories.
π To Enable Push Protection:
Navigate to Settings > Security & Analysis.
Enable Push Protection for all branches.
β 4. Use Pre-Commit Hooks for Secret Detection
Set up Git hooks to scan for secrets before committing code. Tools like git-secrets
can help detect sensitive data locally before pushing.
π₯ Install git-secrets
:
bashCopyEditbrew install git-secrets
git secrets --install
git secrets --add 'API_KEY'
π₯ Add Hooks:
bashCopyEditgit secrets --add '.*API_KEY.*'
git secrets --scan
β 5. Monitor Repositories with Secret Scanning
Enable Secret Scanning on GitHub to automatically detect and alert on exposed secrets. This adds an extra layer of protection by scanning both past commits and incoming pushes.
π Conclusion: Lessons Learned
Encountering this GitHub Push Protection error was a wake-up call to follow secure coding practices diligently. By using environment variables, cleaning Git history properly, and enabling security tools, Iβve reinforced the protection of sensitive information in my projects.
If youβve encountered a similar situation or have any questions about secret management and GitHub Push Protection, feel free to share your experiences in the comments! π
Subscribe to my newsletter
Read articles from Aman Shrivastava directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
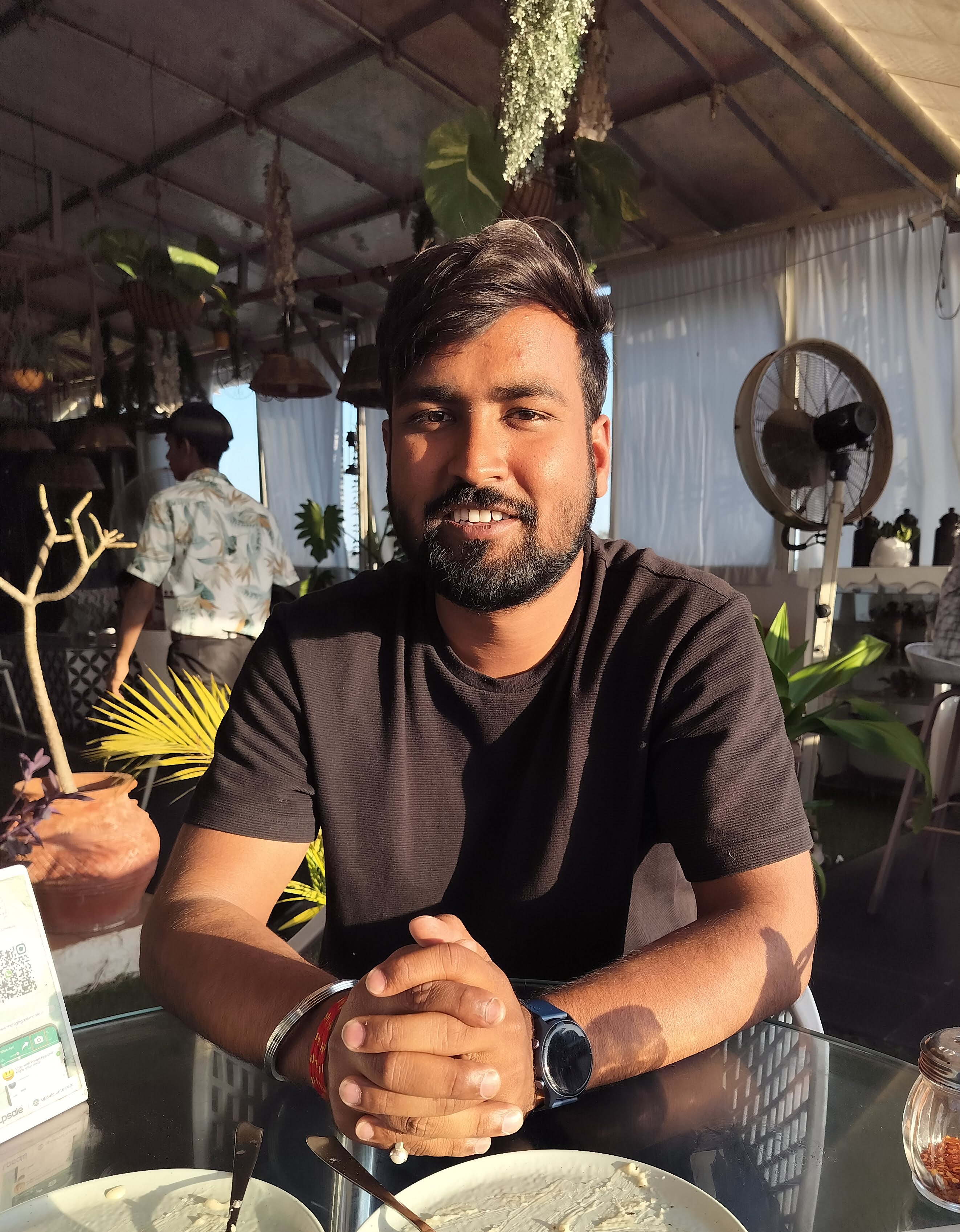
Aman Shrivastava
Aman Shrivastava
I'm a lifelong learner who is eager to experiment with new technologies and areas. I enjoy learning about new technology and applying them to real-world situations. Furthermore, I believe in grinding my knowledge.