Mastering the Linux Shell: Essential Commands

Table of contents
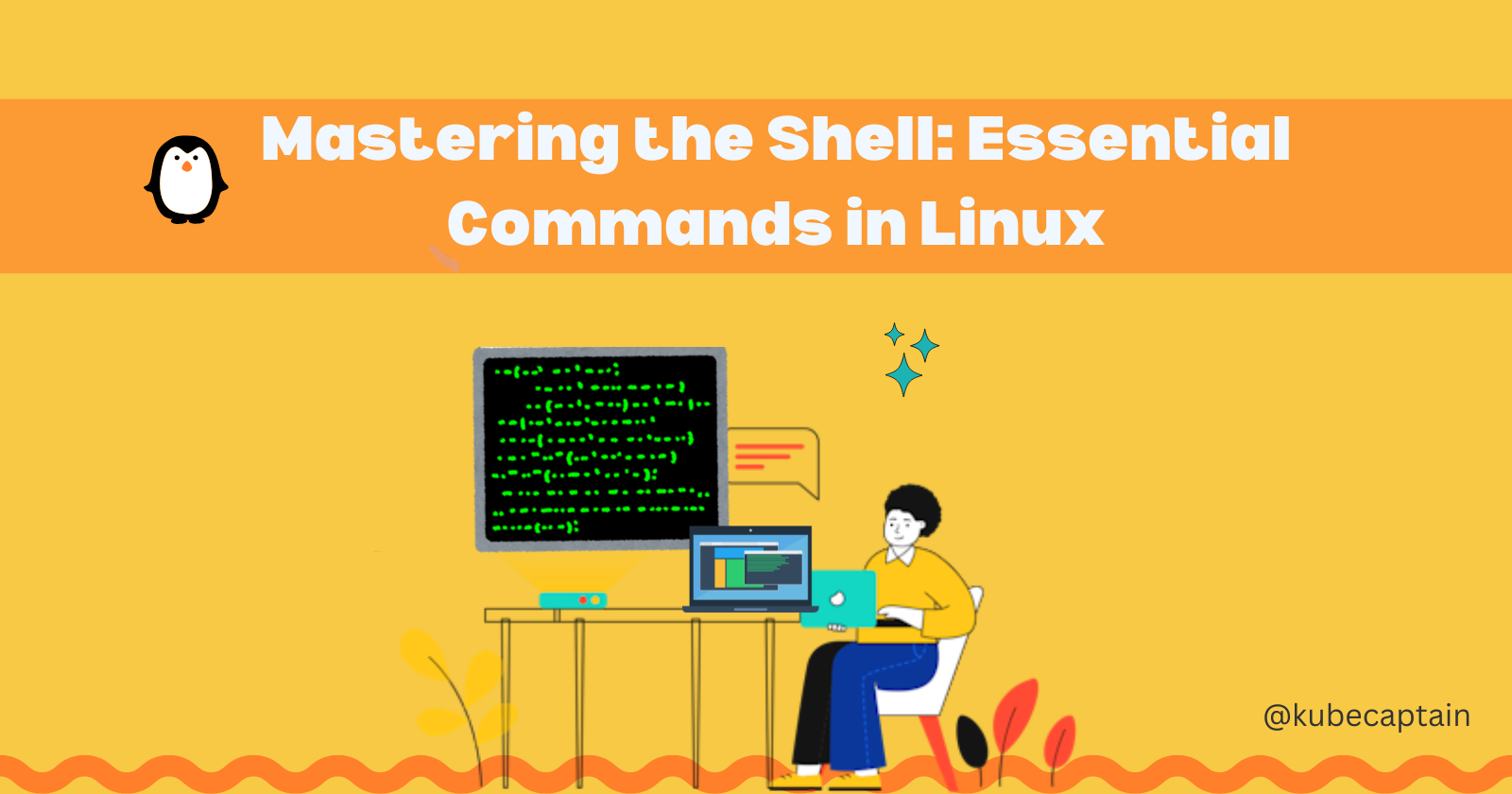
Learning how to navigate the command line is an essential concept in the tech. It helps you work efficiently as a developer and speed up tasks. It also helps you automate tasks by writing the commands in a script file and scheduling it for later.
Linux command line is an important pre-requisite for DevOps and Cloud Computing. Learning how to use the command line helps you to troubleshoot problems, trace the network, manage servers and security configurations.
Basic Shell Commands
Understanding the Linux File System
All files in Linux are stored in a file-system. The root
or /
is at the topmost part and the file system follows a tree like format with directories and sub-directories.
The /
is the root
directory and the starting point of the file system. Here is an example of a complete path starting from the root
:
/home/alex/babel
To check your file system, you can use the tree -d -L 1
command while in root folder. Use the command cd /
to go to root. You’ll learn more about cd
the change directory command in the coming sections.
In the below command, the -L
flag represents the depth of the tree.
tree -d -L 1
.
├── bin -> usr/bin
├── boot
├── cdrom
├── dev
├── etc
├── home
├── lib -> usr/lib
├── lib64 -> usr/lib64
├── lib.usr-is-merged
├── lost+found
├── media
├── mnt
├── opt
├── proc
├── root
├── run
├── sbin -> usr/sbin
├── snap
├── srv
├── sys
├── tmp
├── usr
└── var
24 directories
Note that, depending on your Linux distribution, the output would differ.
Navigating the File System
Absolute path vs relative path
The absolute path refers to the path from the root directory to the destination file or directory. It always starts with a /
. For example, /home/bob/pictures
.
The relative path refers to the path from the current directory to the destination file or directory. It starts from the current directory to the destination file or directory. For example, documents/js/index.js
Locating your current working directory
While in the command line, you might need to find out where you are in the file system. This could be useful for inputs to other commands or just to know where you are. You can locate your current directory using the pwd
command.
pwd
# output
/home/itmasterclass/Downloads
Changing directories using the cd
command
The command to change directories in the command line is cd
. You can use the cd
command to navigate to a different directory in the file system. Here is an example to change directories using the absolute path:
cd /home/bob/pictures
Here is an example to change directories using the relative path:
cd documents/js
You can use the following shortcuts with the cd
command:
cd ..
-> Move up one directorycd ~
-> Move to the home directorycd -
-> Move to the previous directory
Manipulating Files and Folders in the Command Line
Listing files and directories using the ls
command
The ls
command is used to list the files and directories in the current directory. By default, it displays the names of files and directories in the current directory.
ls
The ls
command can also be used with a combination of options to display additional information about the files and directories. For example, the -l
option displays the files and directories in a long listing format, which includes additional information such as file permissions, owner, group, size, and modification date.
# long listing format
ls -l
# output
total 3
-rw-r--r-- 1 user user 0 Jan 1 00:00 file1
-rw-r--r-- 1 user user 0 Jan 1 00:00 file2
drwxr-xr-x 2 user user 4096 Jan 1 00:00 directory1
In the output above, you can view the following information:
The first column represents the file type and permissions. The first character is the file type, where the most common types are:
-
(dash) for regular file.d
for directory.
The permissions are represented by 9 characters. r
stands for read, w
stands for write, and x
stands for execute. The first three characters represent the owner's permissions, the next three characters represent the group's permissions, and the last three characters represent others' permissions. Presence of a permission is represented by the corresponding character, and the absence of a permission is represented by -
.
The second column represents the number of links to the file or directory.
The third and fourth columns represent the owner and group of the file or directory.
The fifth column represents the size of the file or directory in bytes.
The sixth and seventh columns represent the modification date and time of the file or directory.
The last column represents the name of the file or directory.
Here are some more examples of using the ls
command with different options:
# long listing format with human-readable file sizes
ls -lh
# long listing format with hidden files
ls -la
# long listing format with sorting by modification time and reverse order
# reverseLearning how to navigate the command line is an essential concept in the tech. It helps you work efficiently as a developer and speed up tasks. It also helps you automate tasks by writing the commands in a script file and scheduling it for later.Learning how to navigate the command line is an essential concept in the tech. It helps you work efficiently as a developer and speed up tasks. It also helps you automate tasks by writing the commands in a script file and scheduling it for later.r order means the oldest files are at the top and the newest at the bottom
ls -ltr
Creating directories using the mkdir
command
The mkdir
command is used to create directories in the current directory. You can specify the name of the directory that you want to create as an argument to the mkdir
command.
# create a directory named 'directory1'
mkdir directory1
You can recursively create directories using the -p
option. This option creates parent directories as needed.
# create a directory named 'directory2' with parent directories 'parent1' and 'parent2'
mkdir -p parent1/parent2/directory2
Creating files with the touch
and echo
commands
The touch
command is used to create empty files in the current directory. You can specify the name of the file that you want to create as an argument to the touch
command.
# create a file named 'file1'
touch file1
The echo
command is used to write text to a file. You can use the echo
command with the >
operator to write text to a file.
# write 'Hello, World!' to a file named 'file2'
echo 'Hello, World!' > file2
If you use >>
instead of >
, the text will be appended to the existing file content.
Note that just using >
overwrites the existing content.
# append 'Hello, Universe!' to the file named 'file2'
echo 'Hello, Universe!' >> file2
You can also create files using text editors like vim
. You’ll learn more on it later on.
Viewing file contents using cat
and more
You can view existing file contents using the cat
and more
commands.
Here are some examples of using these commands:
- The
cat
command is used to display the contents of a file.
# display the contents of a file named 'file1'
cat file1
- The
more
command displays the contents of a file one screen at a time. You can navigate through the file using the spacebar. To move back one line, press theb
key.
# view the contents of a file named 'file2' using more
more file2
Copying removing and removing files using cp
, mv
and rm
Copying creates a duplicate of a file or directory. The cp
command is used to copy files and directories.
# copy a file named 'file1' to a new file named 'file1_copy'
cp file1 file1_copy
You can also copy the file to another directory by specifying the destination directory as the second argument. The destination directory can either be an absolute path or a relative path.
# copy a file named 'file1' to a directory named 'directory1'
cp file1 directory1
You can also copy folders and their contents using the -r
option where r
stands for recursive.
# copy a directory named 'directory1' to a new directory named 'directory1_copy'
cp -r directory1 directory1_copy
The command mv
is used to move files and directories. Moving means that the file and directory are transferred from one location to another.
# move a file named 'file1' to a new location named 'file1_new_destination'
mv file1 file1_new_destination
The mv
command is also used to rename files and directories.
# rename a file named 'file1' to 'file1_new_name'
mv file1 file1_new_name
There is another difference in copying and moving files. copying changes the file's creation date, while moving retains the original creation date.
Exploring the Vim Text Editor in Linux
Linux offers a variety of text editors. In this section, we'll focus only on Vim- a powerful text editor that comes pre-installed in most Linux distributions.
To open a file in Vim, you can use the following command:
vim filename
If the file doesn’t exist, it would be created.
To navigate through the file, you can use the following keys/ commands:
Arrow keys -> move the cursor up, down, left, or right.
0
-> move the cursor to the beginning of the line.$
-> move the cursor to the end of the line.gg
-> move the cursor to the beginning of the file.G
-> move the cursor to the end of the file.
Vim is a mode-based text editor, which means it has different modes for manipulating the file contents. The three main modes are:
Command mode: This is the default mode when you open a file in Vim. Through this mode, you can access other modes and perform various operations like searching, etc.
Insert or Edit mode: In this mode, you can insert text into the file.
To switch to Insert mode, press i
. Once you have finished editing, switch back to command mode, press Esc
.
Visual mode: This mode allows you to select text visually.
To switch to Visual mode, press v
when you are in Command mode.
You can use the below combination of keys to perform various operations in the Visual mode:
Shift + v
-> select text line by line.Ctrl + v
-> select text block by block.V
-> select text character by character.y
-> copy the selected text.p
-> paste the copied text.
Note: to switch between modes, you first need to be in Command mode.
In short:
To switch from Command mode to Insert mode, press
i
.To switch from Insert mode to Command mode, press
Esc
.To switch from Command mode to Visual mode, press
v
.
Saving and Exiting Vim:
You can use the following shortcuts to exit and save the file or exit without saving.
:wq
-> To save and exit Vim.:q!
-> To exit Vim without saving.
Advanced Shell Commands
In this section you'll learn about some powerful shell commands that can help you manage your system more effectively.
Searching and Filtering
Using grep
for Pattern Searching
grep
stands for "Global Regular Expression Print". With grep
, you can search for patterns within files using regular expressions. You can also pipe the output of other commands to grep
to filter the output.
Using grep
to Search in All Files in a Directory
grep pattern file
Here is an example that finds the word "hello" in the file file.txt
:
grep hello file.txt
If the pattern is found, grep
will print the line containing the pattern.
There are many options available with grep
to customize the search. Here are a few common ones:
-i
-> Ignore case.-n
-> Print the line number where matches occur.-v
-> Invert the match (print lines that do not match the pattern).-r
-> Recursively search directories.
Using grep
to Search for a Specific Pattern in all Files in a Directory
Let's say you have a folder with 500 log files and you want to filter only the files that contain the IP address 192.168.10.100
. You’ll filter the results like this:
grep -l "192.168.10.100" /path/to/directory/*
In the above command, the l
flag is used to list the matching files. As a result, you will get a list of files that only contain the mentioned IP address.
Piping Output to grep
Some Linux commands produce a lot of output. You can use grep
to filter the output to only show lines that match a specific pattern.
For example, you have a directory with multiple items and you want to find the files or folders that contain the word "hello" in their name:
ls -l | grep hello
Using the find
Command
The find
command is used to search for files and folders that match a set of criteria. You can filter files based on file names, file types, file sizes, and more.
Filtering Files by Name
To find all files in the current directory that contain the word "hello" in their name:
find . -name "*hello*"
In the above command:
.
-> Represents the current directory.-name
-> Specifies the pattern to match.
Filtering Files by Type
File types can either be files or folders. Note that folders are also considered files in Linux.
To find all .txt
files in the current directory:
find . -type f -name "*.txt"
In the above command:
-type f
-> Specifies that only files should be matched.
To find all folders that contain the word temp
in their name:
find . -type d -name "*temp*"
In the above command:
-type d
-> Specifies that only directories should be matched.
Process management
Processes refer to the running instances of a program.
In Linux, you can manage processes using various commands like ps
, top
, and kill
.
View Running Processes with ps
To view all the processes running on your system, you can use the ps
command:
ps
Here is a sample output of the ps
command:
PID TTY TIME CMD
1234 pts/0 00:00:00 bash
5678 pts/0 00:00:10 ps
In the output above,
PID
-> Process ID.TTY
-> Terminal associated with the process.TIME
-> Total CPU time used by the process.CMD
-> Command that started the process.
Real-Time Process Information with top
The ps
command only shows a current snapshot of the processes running on your system.
To view the real-time status of processes, you can use the top
command:
top
The top
command updates every few seconds and displays other information as well like CPU usage, memory usage, and more.
Terminating Processes with kill
Sometimes you might need to terminate a process that is not responding or consuming too many resources. You can manually stop a process using the kill
command.
To terminate the process, you should know the process ID (PID) of the process. You can find the PID using the ps
command.
Let's say you want to terminate the VSCode process. First, find the PID of the process:
ps aux | grep VSCode
In the above command, ps aux
lists all the processes running on the system, and grep
filters the output show the n.n. Mmm process.
mmmmmbvbbvv BB
kill PID
-> Sends a SIGTERM signal to the process.kill -9 PID
-> Sends a SIGKILL signal to the process.
SIGTERM is a graceful way to terminate a process. It allows the process to clean up resources before exiting. However, SIGKILL is a forceful way to terminate a process and should be used as a last resort.
You can verify the process has been terminated by running the ps
command again. No process with that ID would be listed.
Networking Commands
Troubleshooting network issues is a common task for system administrators. In this section, you will learn some basic networking commands that can help you diagnose network problems.
Testing Network Connectivity with ping
The ping
command is used to test network connectivity between two nodes. It sends a request to a remote host and waits for a response. If the round trip completes successfully, it means the network connection is working.
To ping a remote host:
ping remote_host
Here, remote_host
can be an IP address or a domain name.
Note that by default, the ping
sends packets indefinitely. You can stop it by pressing Ctrl + C
. However, you can specify the number of packets to send using the -c
flag:
# ping remote_host 5 times
ping -c 5 remote_host
Let's try to ping google.com
:
ping -c 5 google.com
You should notice the stats at the end of the output showing the packet loss and round trip time.
Here is a sample of the stats:
--- google.com ping statistics ---
5 packets transmitted, 5 received, 0% packet loss, time 4000ms
rtt min/avg/max/mdev = 10.000/10.000/10.000/0.000 ms
min
-> Minimum round trip time.avg
-> Average round trip time.max
-> Maximum round trip time.mdev
-> Standard deviation.
Using netstat
for Network Statistics
Another important network command is netstat
. This command displays numerous stats like network connections, routing tables, interface statistics, and more.
You can use the netsat
command in the following ways:
netstat -a
- Displays both listening and non-listening ports.netstat -u
- Displays all UDP connections.netstat -l
- Displays only the listening connections.netstat -r
- Displays the routing table.netstat -s
- Displays network statistics.
Conclusion
In this article you learned how to work with the Linux command line. You also learned some advanced commands to work efficiently. We hope you found this article useful.
Consider sharing it with your friends! Don’t forget to subscribe to our newsletter below.
Subscribe to my newsletter
Read articles from KubeCaptain directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
