The Road Trip That Taught Us JavaScript

Table of contents
- Cheat Sheet Available at the End! 📖✨
- 1. push(" ") : return an updated array (Returns the new length of the array - Number)
- 2. pop() : removes and returns the last element in the array
- 3. shift() : removes and return the first element from the array
- 4. unshift(" ") : Adds new elements to the beginning of an array.(Returns the new length of the array - Number)
- 5. slice() : Extracts a section of an array without modifying the original array. (Returns a new array - Array)
- 6. splice( ) : Changes contents of an array by adding, removing, or replacing elements. (Returns an array of removed elements - Array)
- 7. join() : Converts an array into a single string with a specified separator. (Returns a string - String)
- 8. filter() : Creates a new array with only elements that pass a condition. (Returns a new filtered array - Array)
- The condition inside .filter() is applied individually to each element in the array.
- 9. map() : Creates a new array by transforming each element. (Returns a new transformed array - Array)
- The .map() method creates a new array by applying a function to each element of an existing array. It does not modify the original array.
- 10. reduce() : Reduces an array to a single value by applying a function. (Returns a single accumulated value - Any data type depending on the function)
- CHEATSHEET :
- Conclusion✨ :
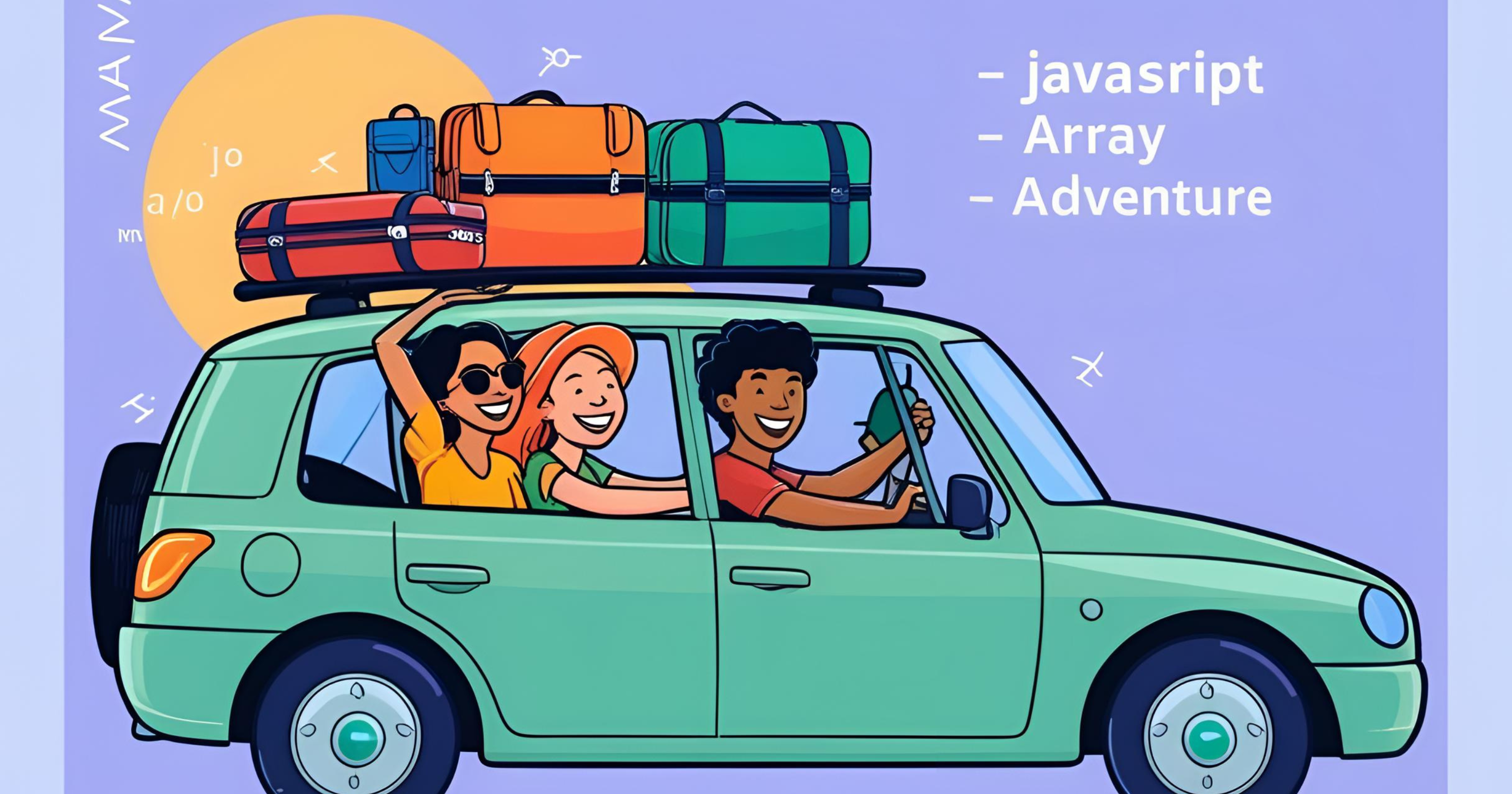
Cheat Sheet Available at the End! 📖✨
Aman said Road trip, guys? Let’s leave in an hour!
1. push(" ")
: return an updated array (Returns the new length of the array - Number)
As we packed all the items, Raghav the overpacker kept packing more stuff.
let packedItems = ["Bag", "Water Bottle", "Shoes"] ;
let N = packedItems.push("Slippers", "Snacks", "Umbrella") ;
console.log(packedItems) ; // [ 'Bag', 'Water Bottle', 'Shoes', 'Slippers', 'Snacks', 'Umbrella' ]
console.log(N) ; // 6
2. pop()
: removes and returns the last element in the array
Riya got a call. so she changes the plan and said “Guys, I will not be able to come for the trip”.
let tripMembers = ["Rohan", "Dhruv", "Riddhima", "Aman", "Riya"] ;
let popped_element = tripMembers.pop() ; // Valid only when Riya is at last otherwise different method
console.log(tripMembers) ; // [ 'Rohan', 'Dhruv', 'Riddhima', 'Aman' ]
console.log(popped_element) ; // Riya
3. shift()
: removes and return the first element from the array
Rohan just checked the clock. It’s 9pm, so i have to reach home before his fathersto comes.
let m = tripMembers.shift() ;
console.log(tripMembers) ; //[ 'Dhruv', 'Riddhima', 'Aman' ]
console.log(m);
4. unshift(" ")
: Adds new elements to the beginning of an array.(Returns the new length of the array - Number)
When all the members were enjoying, Dhruv’s brother called him up and said, I am also at the same place where you are so can I join the crew, Yay!!!
Aditya boards now.
let newLength =tripMembers.unshift("Aditya") ;
console.log(tripMembers) ; // [ 'Aditya', 'Dhruv', 'Riddhima', 'Aman' ]
console.log(newLength) ; // 4
5. slice()
: Extracts a section of an array without modifying the original array. (Returns a new array - Array)
When all were enjoying at the beach, not all pics were Instagram-worthy, so decided to slice out some.
let allPhotos = ["Blurry", "Perfect", "Weird Faces", "Cool Pic"] ;
let insta_worthy_pics = allPhotos.slice(1,3) ;
console.log(allPhotos) ; // [ 'Blurry', 'Perfect', 'Weird Faces', 'Cool Pic' ]
console.log(insta_worthy_pics) ; // [ 'Perfect', 'Weird Faces' ]
6. splice( )
: Changes contents of an array by adding, removing, or replacing elements. (Returns an array of removed elements - Array)
let tripPlan = ["Leave Home", "Go to Beach", "Drive Back"];
let removedPlan = tripPlan.splice(2, 1);
console.log(tripPlan); // [ 'Leave Home', 'Go to Beach' ]
console.log(removedPlan); // [ 'Drive Back' ]
let fun_activity = ["Scooba-Diving", "Boating", "Jogging"] ;
let neww = fun_activity.splice(1, 1, "Kite-Flying" ) ;
console.log(fun_activity); // [ 'Scooba-Diving', 'Kite-Flying', 'Jogging' ]
console.log(neww); // [ 'Boating' ]
let places = ["Goa", "Lakshadweep", "A&N Island"] ;
let new_places = places.splice(1, 0, "Maldives") ; // 0 means insert without deleting.
console.log(places); // [ 'Goa', 'Maldives', 'Lakshadweep', 'A&N Island' ]
console.log(new_places); // []
7. join()
: Converts an array into a single string with a specified separator. (Returns a string - String)
At the end of the trip, sitting at a restaurant, Dhruv summed up the entire adventure:
let tripSummary = ["Packing", "Riya left", "Rohan left", "Aditya joined", "took photos", "Slight change of plan" ] ;
let story = tripSummary.join(" --> ") ;
console.log(tripSummary); // ['Packing','Riya left','Rohan left','Aditya joined','took photos','Slight change of plan']
console.log(story); // Packing --> Riya left --> Rohan left --> Aditya joined --> took photos --> Slight change of plan
8. filter()
: Creates a new array with only elements that pass a condition. (Returns a new filtered array - Array)
The condition inside .filter()
is applied individually to each element in the array.
Each element is passed into the callback function, and the function must return true
or false
:
If
true
→ The element is kept in the new array.If
false
→ The element is filtered out.
Since Aman is veg, so he has to filter.
let menu = ["Paneer-Tikka", "Chicken wings", "Dahi bhalla", "Veg-Noodles", "Fish"] ;
let non_veg = menu.filter((item) => item !== "Chicken wings" && item !== "Fish" ) ;
console.log(menu); // ['Paneer-Tikka', 'Chicken wings', 'Dahi bhalla', 'Veg-Noodles', 'Fish']
console.log(non_veg); // [ 'Paneer-Tikka', 'Dahi bhalla', 'Veg-Noodles' ]
9. map()
: Creates a new array by transforming each element. (Returns a new transformed array - Array)
The .map()
method creates a new array by applying a function to each element of an existing array. It does not modify the original array.
After having the food, it’s time for bill distribution.
// let newArray = array.map(callback(element, index, array));
let bills = [240 , 440, 200 , 180 , 450] ;
let rounded_bill = bills.map(amount => Math.ceil(amount/100)*100) ;
// Math.ceil(number):number → The value you want to round up.
// Returns the smallest integer greater than or equal to the given number.
console.log(bills); // [ 240, 440, 200, 180, 450 ]
console.log(rounded_bill); // [ 300, 500, 200, 200, 500 ]
10. reduce()
: Reduces an array to a single value by applying a function. (Returns a single accumulated value - Any data type depending on the function)
array.reduce((accumulator, currentValue, index, array) => {
// return updated accumulator
}, initialValue);
Finally, Aman, the finance guy, pulled out his phone and did the math.
let total_expense = rounded_bill.reduce((acc, amt) => (acc + amt), 0) ;
console.log(total_expense); // 1700
CHEATSHEET :
// push() - Add to end
array.push("NewItem");
// pop() - Remove last element
array.pop();
// shift() - Remove first element
array.shift();
// unshift() - Add to beginning
array.unshift("NewItem");
// slice() - Extract without modifying
let newArray = array.slice(1, 3);
// splice() - Modify original array
array.splice(1, 1, "NewItem");
// join() - Convert to string
let str = array.join(", ");
// filter() - Select elements
let filtered = array.filter(item => item > 10);
// map() - Transform array
let mapped = array.map(item => item * 2);
// reduce() - Accumulate values
let total = array.reduce((acc, item) => acc + item, 0);
Conclusion✨ :
Life is like a road trip—some people join, some leave, and plans change along the way. But in the end, everything comes together to make a great story.
And just like that, our random trip turned into a lesson about coding, friendship, and unexpected adventures.🚀
Subscribe to my newsletter
Read articles from Dhruv Mittal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
