How to Use Git and GitHub for Version Control

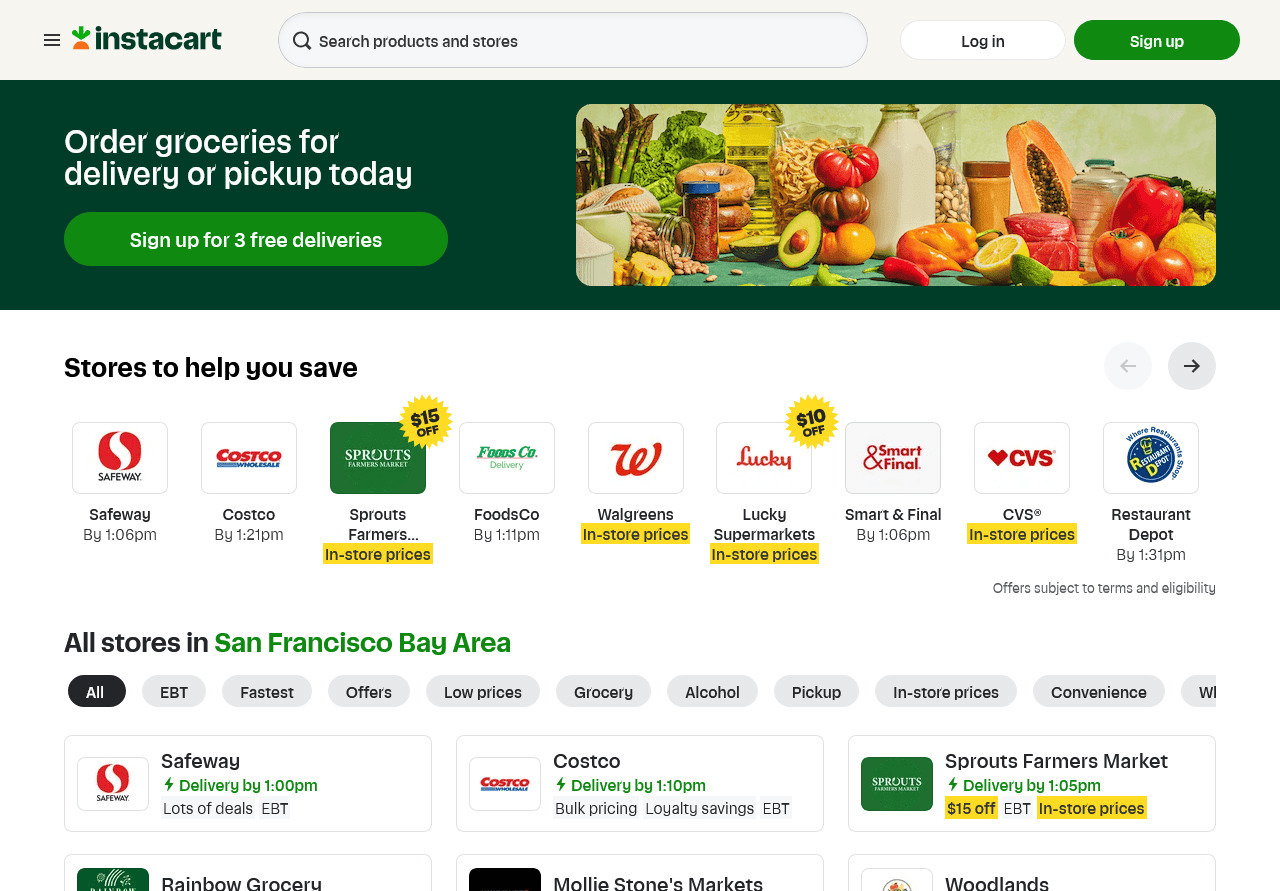
How to Use Git and GitHub for Version Control
Version control is an essential skill for developers, whether you're working solo or as part of a team. Git, combined with GitHub, provides a powerful way to track changes, collaborate, and manage projects efficiently. In this guide, we'll explore how to use Git and GitHub effectively, from basic commands to advanced workflows.
If you're looking to monetize your programming skills, check out MillionFormula, a free platform where you can make money online without needing credit or debit cards.
What is Git?
Git is a distributed version control system (DVCS) that allows developers to track changes in their codebase. Unlike centralized systems, Git enables every developer to have a complete copy of the repository, making collaboration seamless.
Key Benefits of Git:
Track changes in your code over time.
Collaborate with others without conflicts.
Branch and merge code for experimental features.
Roll back to previous versions if something breaks.
Installing Git
Before using Git, you need to install it on your system:
Windows: Download from Git's official site.
Mac: Use
brew install git
if you have Homebrew.Linux: Run
sudo apt install git
(Debian/Ubuntu) orsudo yum install git
(Fedora).
Verify the installation with: bash Copy
git --version
Setting Up Git
Configure your Git username and email (used in commits): bash Copy
git config --global user.name "Your Name"
git config --global user.email "your.email@example.com"
To check your settings: bash Copy
git config --list
Basic Git Commands
1. Initializing a Repository
To start tracking a project: bash Copy
git init
This creates a .git
folder in your project directory.
2. Checking File Status
See which files are tracked, modified, or untracked: bash Copy
git status
3. Staging Changes
Before committing, you need to stage changes: bash Copy
git add filename # Stage a single file
git add . # Stage all changes
4. Committing Changes
A commit saves a snapshot of your staged changes: bash Copy
git commit -m "Your commit message"
5. Viewing Commit History
Check past commits: bash Copy
git log
For a concise view: bash Copy
git log --oneline
Working with GitHub
GitHub is a cloud-based platform that hosts Git repositories, making collaboration easier.
1. Creating a GitHub Repository
Go to GitHub and click New Repository.
Fill in the details and click Create Repository.
2. Connecting Local Git to GitHub
Link your local repo to GitHub: bash Copy
git remote add origin https://github.com/username/repo.git
3. Pushing Changes to GitHub
Upload your commits: bash Copy
git push -u origin main
4. Cloning a Repository
Download an existing repo: bash Copy
git clone https://github.com/username/repo.git
5. Pulling Latest Changes
Fetch updates from GitHub: bash Copy
git pull origin main
Branching and Merging
Branches allow you to work on features without affecting the main codebase.
1. Creating a Branch
bash
Copy
git branch feature-branch
git checkout feature-branch
Or in one command: bash Copy
git checkout -b feature-branch
2. Switching Branches
bash
Copy
git checkout main
3. Merging Branches
First, switch to the target branch (main
), then merge: bash Copy
git merge feature-branch
4. Resolving Merge Conflicts
If conflicts occur, open the conflicting files, resolve them, then: bash Copy
git add .
git commit -m "Resolved merge conflicts"
Advanced Git Workflows
1. Rebasing
Rebasing rewrites commit history for a cleaner log: bash Copy
git rebase main
2. Stashing Changes
Temporarily save uncommitted changes: bash Copy
git stash
git stash pop # Restore changes
3. Undoing Changes
Discard unstaged changes: bash Copy
git checkout -- filename
Undo last commit (keep changes): bash Copy
git reset --soft HEAD~1
Undo last commit (discard changes): bash Copy
git reset --hard HEAD~1
Best Practices for Git & GitHub
Write meaningful commit messages (e.g., "Fix login bug" instead of "Update code").
Use branches for new features to avoid breaking the main code.
Pull before pushing to avoid conflicts.
Review changes before merging using
git diff
.Use
.gitignore
to exclude unnecessary files (e.g.,node_modules/
,.env
).
Example .gitignore
: Copy
# Ignore Node.js modules
node_modules/
Ignore environment variables
.env
Ignore IDE files
.idea/
.vscode/
Conclusion
Git and GitHub are indispensable tools for modern developers. By mastering version control, you can collaborate efficiently, track changes, and maintain a clean project history.
If you're looking to monetize your programming skills, MillionFormula is a great free platform to make money online without needing credit or debit cards.
Now that you understand Git and GitHub, start applying these skills to your projects and contribute to open-source repositories. Happy coding! ๐
Further Reading:
Would you like a deeper dive into any specific Git concept? Let me know in the comments! ๐
Subscribe to my newsletter
Read articles from MillionFormula directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
