Background Processing in Flutter Applications: A Deep Dive

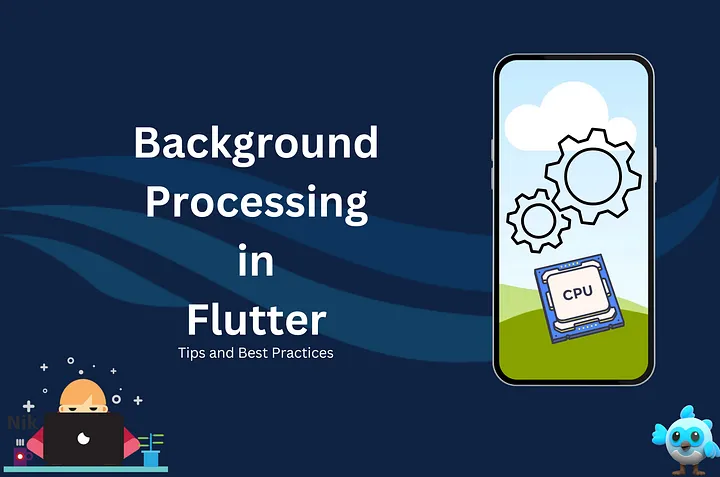
As Flutter applications grow in complexity, efficient background processing becomes crucial for maintaining smooth user experiences. In this comprehensive guide, weโll explore various approaches to implement background processing in Flutter applications.
Introduction
Background processing allows apps to perform resource-intensive tasks without blocking the main UI thread. Flutter provides several ways to handle background tasks, from simple compute functions to complex background services.
1. Understanding Isolates
Flutter runs on a single thread by default. Isolates allow you to run code on different threads, similar to workers in JavaScript.
import 'dart:isolate';
// Basic Isolate Example
Future<List<int>> processDataInBackground(List<int> data) async {
final receivePort = ReceivePort();
await Isolate.spawn((message) {
final input = message[0] as List<int>;
final sendPort = message[1] as SendPort;
// Perform intensive calculation
final result = input.map((e) => e * 2).toList();
sendPort.send(result);
}, [data, receivePort.sendPort]);
final result = await receivePort.first;
return result as List<int>;
}
Using compute() Function
For simpler cases, Flutter provides the compute
function:
import 'package:flutter/foundation.dart';
class DataProcessor {
Future<List<ProcessedData>> processLargeDataSet(List<RawData> data) async {
return await compute(_processData, data);
}
static List<ProcessedData> _processData(List<RawData> input) {
return input.map((data) => ProcessedData(
id: data.id,
value: _complexCalculation(data.value),
)).toList();
}
}
2. Background Services
For long-running tasks, implementing a background service is more appropriate.
class BackgroundService {
static Future<void> initialize() async {
final service = FlutterBackgroundService();
await service.configure(
androidConfiguration: AndroidConfiguration(
onStart: onStart,
autoStart: true,
isForegroundMode: true,
notificationChannelId: 'background_service',
initialNotificationTitle: 'App Service',
initialNotificationContent: 'Processing...',
),
iosConfiguration: IosConfiguration(
autoStart: true,
onForeground: onStart,
onBackground: onIosBackground,
),
);
}
static void onStart(ServiceInstance service) {
service.on('stopService').listen((event) {
service.stopSelf();
});
// Periodic task
Timer.periodic(const Duration(seconds: 10), (timer) async {
if (!(await service.isRunning())) {
timer.cancel();
return;
}
// Perform background task
await performPeriodicTask();
// Update notification
service.setNotificationInfo(
title: 'Background Service',
content: 'Last updated: ${DateTime.now()}',
);
});
}
}
3. WorkManager Integration
For scheduled tasks that need to survive app restarts, WorkManager is an excellent choice:
class WorkManagerService {
static void initialize() {
Workmanager().initialize(
callbackDispatcher,
isInDebugMode: true,
);
}
static void callbackDispatcher() {
Workmanager().executeTask((taskName, inputData) async {
switch (taskName) {
case 'syncData':
await syncData();
break;
case 'processImages':
await processImages();
break;
default:
print('Unknown task: $taskName');
}
return true;
});
}
static Future<void> schedulePeriodicTask() async {
await Workmanager().registerPeriodicTask(
'periodicSync',
'syncData',
frequency: const Duration(hours: 1),
constraints: Constraints(
networkType: NetworkType.connected,
requiresBatteryNotLow: true,
),
);
}
}
4. Platform-Specific Background Processing
Android Background Service
// android/app/src/main/kotlin/com/example/app/BackgroundService.kt
class BackgroundService : Service() {
override fun onStartCommand(intent: Intent?, flags: Int, startId: Int): Int {
val notification = NotificationCompat.Builder(this, CHANNEL_ID)
.setContentTitle("Background Service")
.setContentText("Running")
.build()
startForeground(1, notification)
return START_STICKY
}
}
iOS Background Tasks
// ios/Runner/AppDelegate.swift
@UIApplicationMain
class AppDelegate: FlutterAppDelegate {
override func application(
_ application: UIApplication,
didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?
) -> Bool {
if #available(iOS 13.0, *) {
BGTaskScheduler.shared.register(
forTaskWithIdentifier: "com.example.backgroundTask",
using: nil
) { task in
self.handleBackgroundTask(task: task as! BGProcessingTask)
}
}
return super.application(application, didFinishLaunchingWithOptions: launchOptions)
}
}
5. Best Practices and Performance Tips
1. Memory Management
class BackgroundTaskManager {
static final Map<String, Isolate> _activeIsolates = {};
static Future<void> startTask(String taskId, Function task) async {
if (_activeIsolates.containsKey(taskId)) {
await stopTask(taskId);
}
final receivePort = ReceivePort();
final isolate = await Isolate.spawn(task, receivePort.sendPort);
_activeIsolates[taskId] = isolate;
}
static Future<void> stopTask(String taskId) async {
final isolate = _activeIsolates[taskId];
if (isolate != null) {
isolate.kill();
_activeIsolates.remove(taskId);
}
}
}
2. Error Handling
class BackgroundTaskError extends Error {
final String message;
final String? stackTrace;
BackgroundTaskError(this.message, [this.stackTrace]);
@override
String toString() => 'BackgroundTaskError: $message\n$stackTrace';
}
Future<void> safeBackgroundExecution(Future<void> Function() task) async {
try {
await task();
} catch (e, stackTrace) {
// Log error
print('Background task failed: $e\n$stackTrace');
// Notify error monitoring service
await ErrorReporter.report(
BackgroundTaskError(e.toString(), stackTrace.toString()),
);
rethrow;
}
}
6. Monitoring and Debugging
class BackgroundTaskMonitor {
static final _taskTimings = <String, DateTime>{};
static final _taskMetrics = <String, Map<String, dynamic>>{};
static void startTracking(String taskId) {
_taskTimings[taskId] = DateTime.now();
}
static void endTracking(String taskId) {
final startTime = _taskTimings[taskId];
if (startTime != null) {
final duration = DateTime.now().difference(startTime);
_taskMetrics[taskId] = {
'duration': duration.inMilliseconds,
'completed': DateTime.now(),
};
_taskTimings.remove(taskId);
}
}
static Map<String, dynamic> getMetrics() {
return Map.from(_taskMetrics);
}
}
Conclusion
Effective background processing is essential for building robust Flutter applications. Key takeaways:
Use Isolates for CPU-intensive tasks
Implement background services for long-running operations
Use WorkManager for scheduled tasks
Handle platform-specific requirements
Monitor and optimize performance
Implement proper error handling
Follow for more Flutter development content!
#Flutter #MobileApp #Programming #BackgroundProcessing #Development #FlutterNik
Subscribe to my newsletter
Read articles from Ravi Patel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Ravi Patel
Ravi Patel
"๐ฑ Passionate Flutter Developer with 6+ years of experience, dedicated to crafting exceptional mobile applications. ๐ Adept at turning ideas into polished, user-friendly experiences using Flutter's magic. ๐จ Design enthusiast who believes in the power of aesthetics and functionality. ๐ ๏ธ Expertise in translating complex requirements into clean, efficient code. ๐ Committed to staying updated with the latest trends and continuously pushing boundaries. Let's create something extraordinary together!"