How to Implement Routing in React Using react-router-dom with createBrowserRouter and RouterProvider
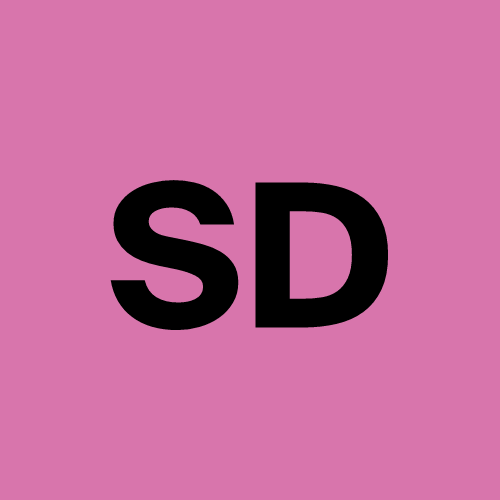
React Router is a popular library for handling routing in React applications. With React Router v6.4 and later, the new createBrowserRouter
and RouterProvider
APIs provide a more declarative and modern way to manage routing in React projects.
In this article, we'll guide you through setting up routing in a React app using createBrowserRouter
and RouterProvider
. Our example project will have a basic structure with three pages: Home, About, and Contact.
Set up a react project, if you have not already. You can follow Get Started With React to set up your project.
Steps to implement Routing in React
Install
react-router-dom
for handling the routing:npm install react-router-dom
Organize Your
src
folder.src/ ├── components/ // Folder ├── layout/ // Folder ├── pages/ // Folder └── App.jsx
Create Home.jsx (
src/pages/Home.js
)import React from 'react'; const Home = () => { return ( <div> <h1>Home Page</h1> <p>Welcome to the home page of our React app!</p> </div> ); }; export default Home;
Create About.jsx (
src/pages/About.js
)import React from 'react'; const About = () => { return ( <div> <h1>About Page</h1> <p>Learn more about our app and the team behind it.</p> </div> ); }; export default About;
Create Contact.jsx (
src/pages/Contact.js
)import React from 'react'; const Contact = () => { return ( <div> <h1>Contact Page</h1> <p>If you have any questions, feel free to contact us!</p> </div> ); }; export default Contact;
Create the Navbar Component (
src/components/Navbar.jsx
)import React from 'react'; import { Link } from 'react-router-dom'; const Navbar = () => { return ( <nav> <ul> <li> <Link to="/">Home</Link> </li> <li> <Link to="/about">About</Link> </li> <li> <Link to="/contact">Contact</Link> </li> </ul> </nav> ); }; export default Navbar;
Create the FooterComponent (
src/components/Footer.jsx
)import React from 'react'; const Footer = () => { return ( <footer> <p>© 2025 React Router Example</p> </footer> ); }; export default Footer;
Create the Layout: The Layout component (
MainLayout.jsx
) will act as a wrapper around the pages and will include theNavbar
andFooter
. This way, the navbar and footer will be present on every page.MainLayout.jsx (
src/layout/MainLayout.jsx
)import React from 'react'; import { Outlet } from "react-router-dom" import Navbar from '../components/Navbar'; import Footer from '../components/Footer'; const MainLayout = () => { return ( <div> <Navbar /> <Outlet /> <Footer /> </div> ); }; export default MainLayout;
Now Your Project Structure may look like:
src/ ├── components/ │ ├── Navbar.jsx │ └── Footer.jsx ├── layout/ │ └── MainLayout.jsx ├── pages/ │ ├── Home.jsx │ ├── About.jsx │ └── Contact.jsx └── App.jsx
Set Up Routing with
createBrowserRouter
andRouterProvider
Now, it's time to configure routing. We'll use
createBrowserRouter
to define the routes and their corresponding components. TheRouterProvider
will make the routing available to our application.App.jsx (
src/App.jsx
)import './App.css'; import React from 'react'; import { createBrowserRouter, RouterProvider } from 'react-router-dom'; import MainLayout from './layout/MainLayout'; import Home from './pages/Home'; import About from './pages/About'; import Contact from './pages/Contact'; // Create the router using createBrowserRouter const router = createBrowserRouter([ { path: "/", element: <MainLayout />, // Wrap the layout with the Navbar and Footer children: [ { path: '/', element: <Home /> }, { path: '/about', element: <About /> }, { path: '/contact', element: <Contact /> }, ], }, ]); // Main App component function App() { return <RouterProvider router={router} />; } export default App;
Add Some Styling (Optional)
You can add CSS to make the app look better. For example, create a simple
App.css
and import it into yourApp.js
:App.css (
src/App.css
)body { font-family: Arial, sans-serif; margin: 0; padding: 0; } nav ul { display: flex; justify-content: space-around; background-color: #282c34; padding: 1rem; margin: 0; } nav ul li { list-style: none; } nav ul li a { color: white; text-decoration: none; font-weight: bold; } footer { background-color: #282c34; color: white; text-align: center; padding: 1rem; } main { padding: 20px; }
Now that everything is set up, you can start the application using:
npm run dev
In this article, we've gone through how to set up routing in a React application using the modern
createBrowserRouter
andRouterProvider
API fromreact-router-dom
. We built a small project with three pages (Home, About, and Contact), along with a reusableNavbar
andFooter
. By usingcreateBrowserRouter
for route definitions andRouterProvider
to pass the router configuration to the app, we embraced the new declarative approach to routing in React.Happy Coding :)
Subscribe to my newsletter
Read articles from Surajit Das directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
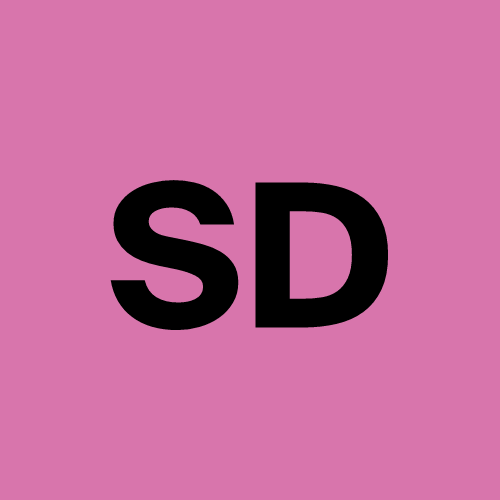