Understanding the Module Wrapper Function in JavaScript

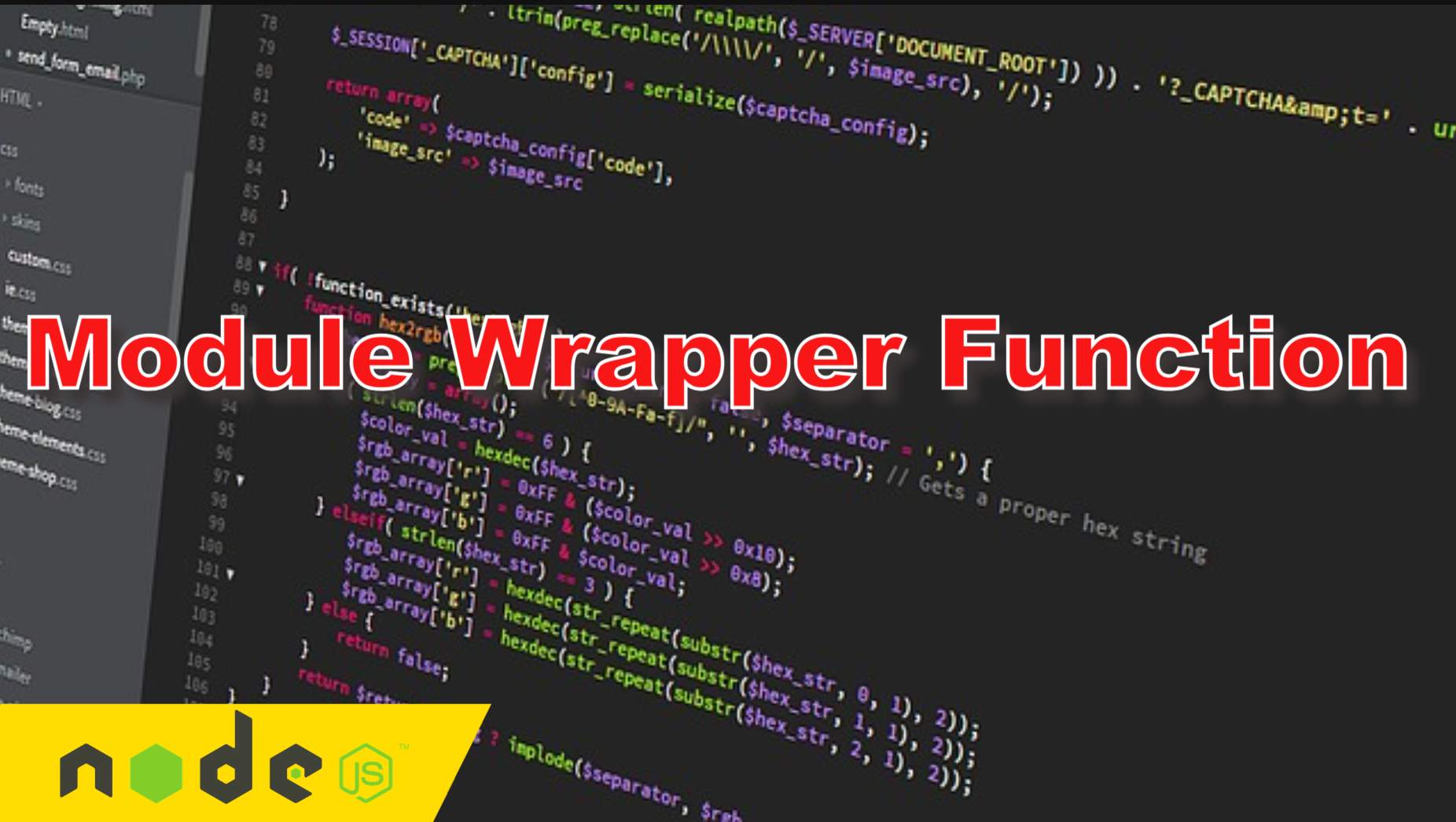
JavaScript uses a powerful yet often overlooked concept known as the Module Wrapper Function when working with modules, particularly in Node.js. This function plays a crucial role in how modules are structured, executed, and protected.
What is the Module Wrapper Function?
In Node.js, every module is wrapped inside an IIFE (Immediately Invoked Function Expression). This function provides a private scope for the module, preventing variable leakage into the global scope.
Before execution, Node.js wraps every module in the following function:
(function(exports, require, module, __filename, __dirname) {
// Module code goes here
});
This is known as the Module Wrapper Function. Node.js does this to provide each module with some useful variables and to ensure that the code inside the module does not interfere with other modules or the global scope.
Parameters of the Wrapper Function
The wrapper function provides five special arguments to each module:
exports
– A shortcut to export module contents.require
– A function to import modules.module
– Represents the current module (can be used instead ofexports
).__filename
– The absolute path of the current module file.__dirname
– The directory name of the current module.
Example of How the Wrapper Function Works
Consider a simple module greet.js
:
console.log(__filename); // Prints the full file path
console.log(__dirname); // Prints the directory name
function greet(name) {
console.log(`Hello, ${name}!`);
}
module.exports = greet;
Even though we do not explicitly define a function wrapping this code, Node.js automatically wraps it, so the actual execution looks like:
(function(exports, require, module, __filename, __dirname) {
console.log(__filename);
console.log(__dirname);
function greet(name) {
console.log(`Hello, ${name}!`);
}
module.exports = greet;
})(exports, require, module, __filename, __dirname);
Manually Implementing the Module Wrapper Function
To better understand how it works, let’s manually implement the wrapper function in a module:
Create a file wrapperExample.js
:
(function(exports, require, module, __filename, __dirname) {
let secret = "This is a secret message";
function revealSecret() {
return secret;
}
module.exports = { revealSecret };
})(exports, require, module, __filename, __dirname);
Now, create another file app.js
that imports wrapperExample.js
:
const wrapperExample = require('./wrapperExample');
console.log(wrapperExample.revealSecret()); // Output: This is a secret message
Here, we manually wrapped the module content inside an IIFE, simulating what Node.js does under the hood.
Why is the Module Wrapper Function Important?
Encapsulation & Avoiding Global Pollution:
Variables and functions declared inside a module do not affect the global scope.
This prevents naming conflicts between different modules.
Providing Useful Variables:
__filename
and__dirname
help in file path management.require
andmodule.exports
facilitate modular programming.
Code Security:
- Since each module has its own scope, it reduces the risk of unintentional data manipulation between modules.
Demonstrating the Module Wrapper Function in Action
Create a file math.js
with the following content:
let pi = 3.14159;
function add(a, b) {
return a + b;
}
module.exports = { pi, add };
Now, create another file app.js
that imports math.js
:
const math = require('./math');
console.log(math.pi); // Output: 3.14159
console.log(math.add(5, 10)); // Output: 15
Even though pi
and add()
are declared inside math.js
, they do not pollute the global scope because of the Module Wrapper Function.
Conclusion
The Module Wrapper Function is an essential part of Node.js modules. It ensures encapsulation, prevents global scope pollution, and provides useful built-in variables. Understanding this concept helps developers write modular, maintainable, and secure JavaScript code.
Next time you write a Node.js module, remember that it's always being wrapped inside this hidden function, keeping your code safe and organized!
Subscribe to my newsletter
Read articles from Mohit Uchit directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Mohit Uchit
Mohit Uchit
I'm a passionate Backend Engineer with 3 years of experience, specializing in building scalable and efficient systems. I work extensively with Node.js, Express.js, and REST APIs to create robust server-side applications. My database expertise includes MySQL, MongoDB, and Sequelize, while I leverage Redis for caching and optimization. I’m a strong advocate for microservices architecture, designing solutions that are modular and resilient. Through this blog, I share insights, tutorials, and tips from my journey in backend development. Let’s connect and build something amazing together!