Understanding LookupBoundary in Flutter

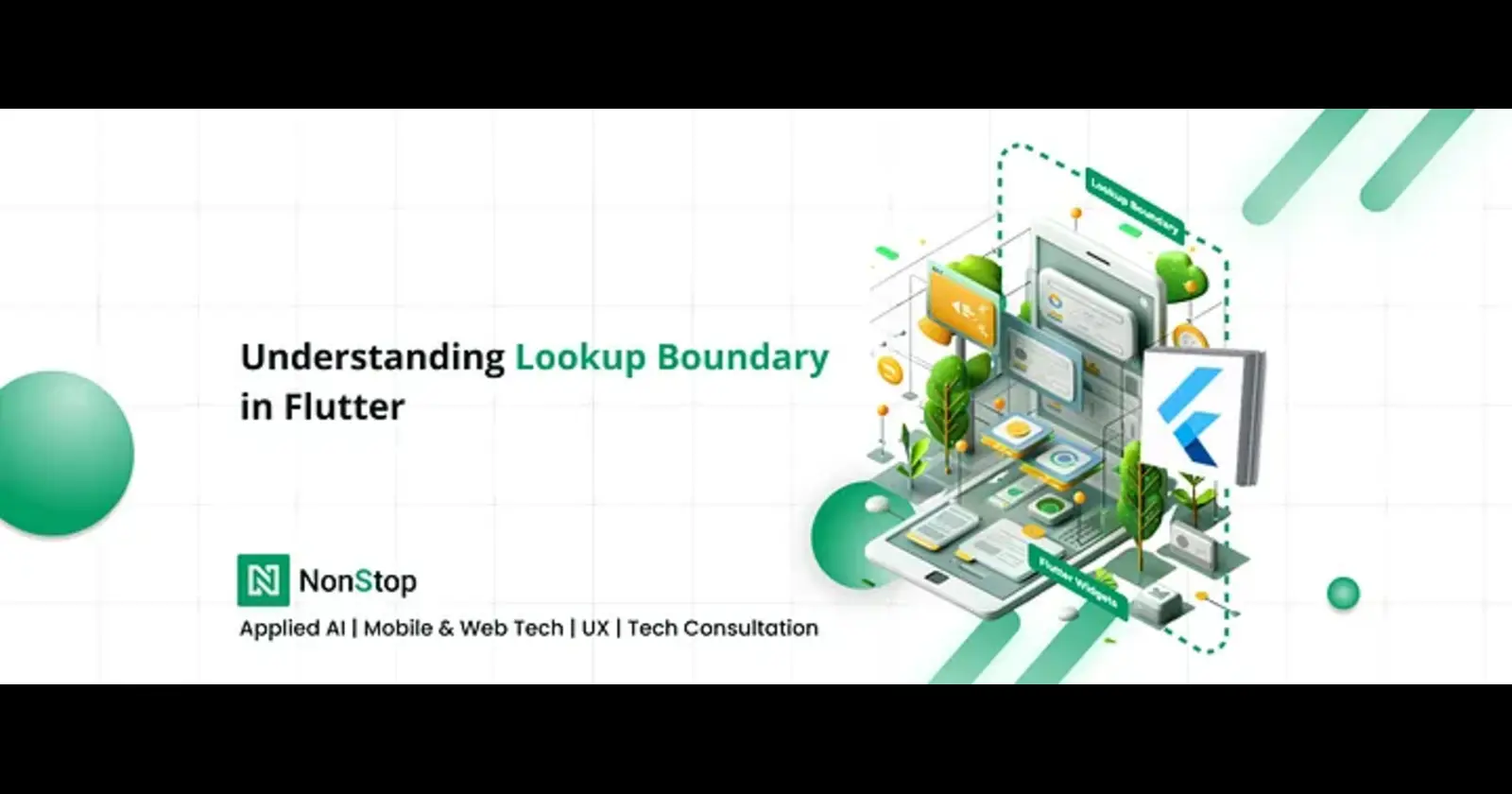
Flutter’s rich set of widgets and tools make it a popular framework for building beautiful, natively compiled applications for mobile, web, and desktop from a single codebase. One such powerful yet lesser-known widget is the LookupBoundary
. This blog will dive into what LookupBoundary
is, why it's useful, and how to use it with a practical example.
What is LookupBoundary?
A LookupBoundary
controls what entities are visible to descendants of the boundary via the static lookup methods provided by the boundary. The static lookup methods of the boundary mirror the lookup methods by the same name exposed on BuildContext
, and they can be used as direct replacements. Unlike the methods on BuildContext
, these methods do not find any ancestor entities of the closest LookupBoundary
surrounding the provided BuildContext
. The root of the tree is an implicit lookup boundary.
A Note on Direct Instantiation
Using
LookupBoundary
directly in an application is generally uncommon and not recommended unless you encounter specific scenarios where the render tree diverges from the element tree. This class is primarily used internally to address anomalies in the widget tree where render objects do not attach to the nearest ancestor. These anomalies can break certain widget assumptions about the structure of the render tree. Typically,LookupBoundary
is used to hide certain ancestors in such cases to maintain correct behavior in the widget tree. For more details, refer to the official documentation.
When to Use LookupBoundary?
Use LookupBoundary
when:
There is a need to control the scope of visibility for various entities in your widget tree.
You want to create clear boundaries for widget lookups in complex widget trees.
You need to optimize performance by limiting the lookup scope.
Example: Using LookupBoundary in a Flutter Application
Let’s walk through a simple example to understand how LookupBoundary
works. We'll create an application with a custom widget and use LookupBoundary
to demonstrate its effect.
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: HomePage(),
);
}
}
class MyWidget extends InheritedWidget {
final String data;
MyWidget({required this.data, required Widget child}) : super(child: child);
@override
bool updateShouldNotify(MyWidget oldWidget) {
return oldWidget.data != data;
}
static MyWidget? of(BuildContext context) {
return context.dependOnInheritedWidgetOfExactType<MyWidget>();
}
}
class HomePage extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('LookupBoundary Example'),
),
body: MyWidget(
data: 'Inherited Data',
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
// Inside LookupBoundary
LookupBoundary(
child: Builder(
builder: (BuildContext context) {
MyWidget? widget =
LookupBoundary.findAncestorWidgetOfExactType<MyWidget>(
context);
return Text(
'Inside LookupBoundary: ${widget?.data ?? "null"}');
},
),
),
// Outside LookupBoundary
Builder(
builder: (BuildContext context) {
MyWidget? widget = LookupBoundary.findAncestorWidgetOfExactType<MyWidget>(
context);
return Text(
'Outside LookupBoundary: ${widget?.data ?? "null"}');
},
),
],
),
),
);
}
}
Explanation
In this example, we have a simple Flutter application with the following components:
MyApp
: The root widget of the application.MyCustomWidget
: AnInheritedWidget
that holds some data.HomePage
: A stateless widget that contains two sections - one inside aLookupBoundary
and one outside.
The HomePage
widget builds a Scaffold
with an AppBar
and a Column
containing two Builder
widgets. One Builder
is wrapped in a LookupBoundary
, and the other is not. Each Builder
tries to access the MyCustomWidget
inherited widget.
Inside LookupBoundary: The lookup stops at the
LookupBoundary
, so the data is not found, and it displays "null".Outside LookupBoundary: The lookup proceeds up the tree and finds the
MyCustomWidget
, so it displays "Custom Data".
Feel free to explore more about
LookupBoundary
and other Flutter widgets to harness the full potential of Flutter's capabilities. Happy coding!
Subscribe to my newsletter
Read articles from NonStop io Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

NonStop io Technologies
NonStop io Technologies
Product Development as an Expertise Since 2015 Founded in August 2015, we are a USA-based Bespoke Engineering Studio providing Product Development as an Expertise. With 80+ satisfied clients worldwide, we serve startups and enterprises across San Francisco, Seattle, New York, London, Pune, Bangalore, Tokyo and other prominent technology hubs.