How Java Handles Memory: Management and Model Explained
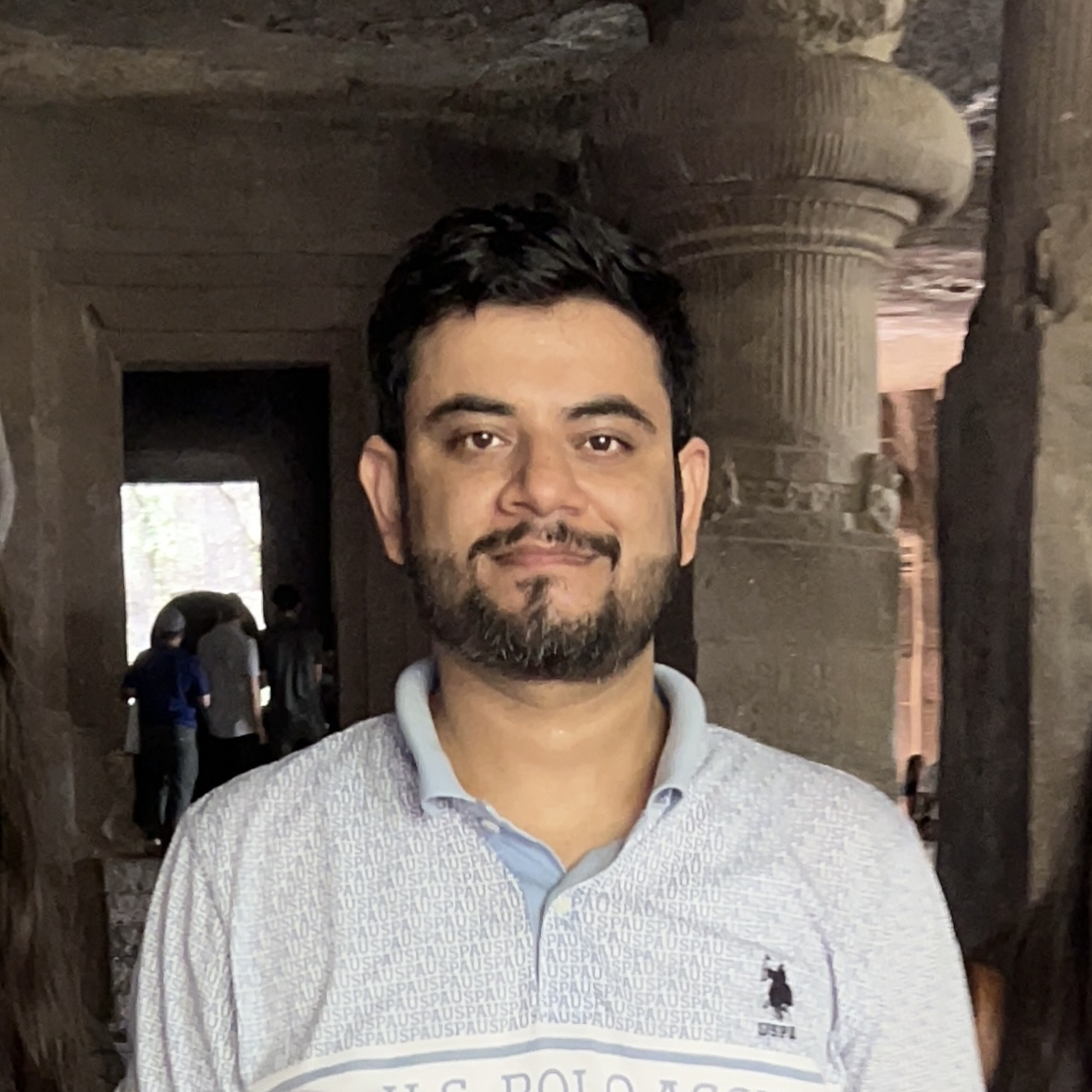
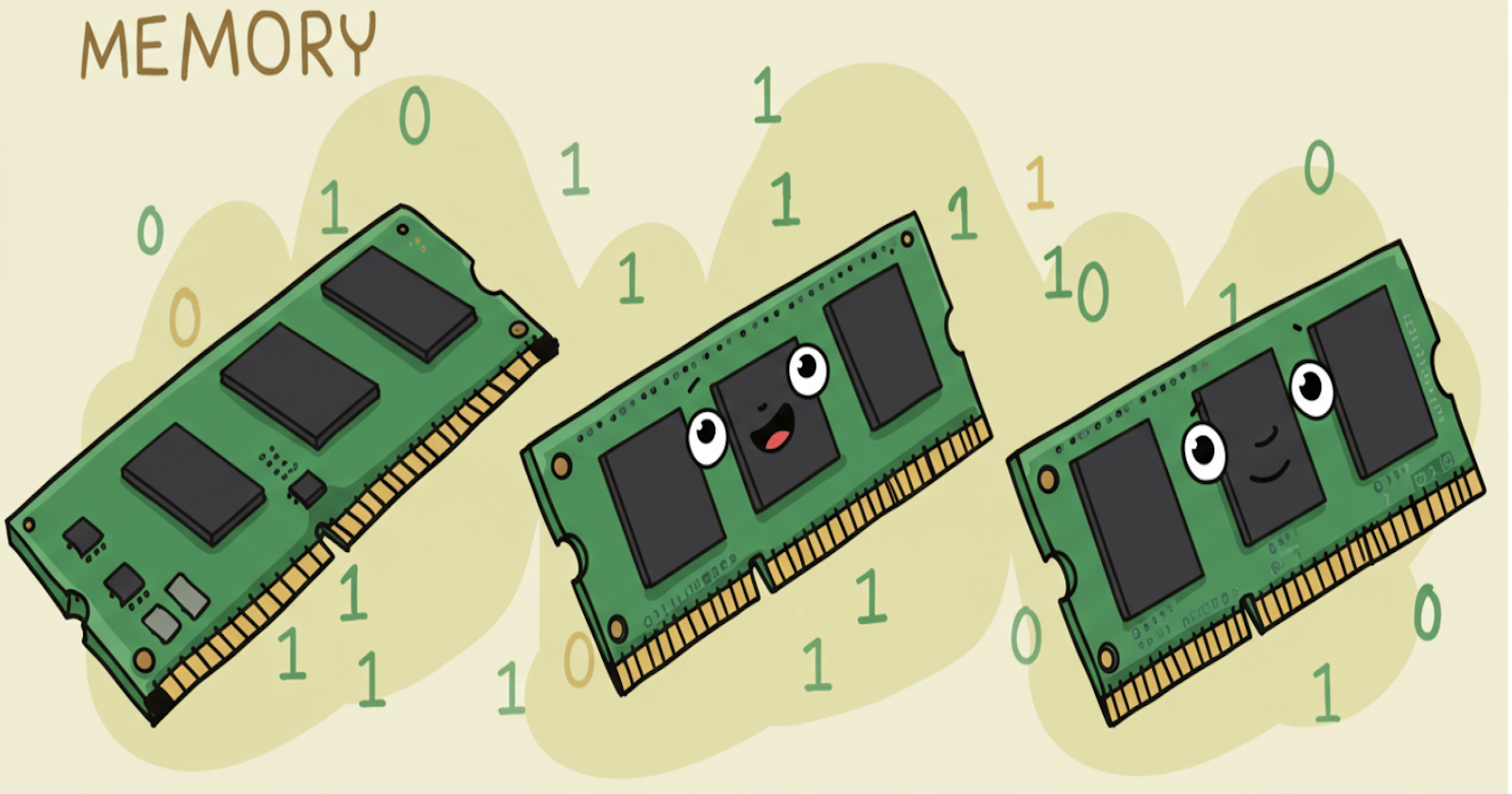
To run programs and store information, a computer's memory must be efficiently managed to allocate data to appropriate memory spaces. This article explores the fundamentals of memory management and the memory model in Java, including how memory is de-allocated and reclaimed when it is no longer needed.
Memory Management
Heap Space
Java objects are created as the instances of the Java classes. The JVM (Java Virtual Machine) internally represents these objects, and these representations are stored in the heap. As exhibited in the above diagrams, the Java Heap space is further divided into regions known as generations.
Young Generation
A memory region designated for short-lived objects, divided into Eden Space and Survivor Spaces.
Eden Space
- Memory is allocated here for objects created with the
new
keyword. When full, a Minor Garbage Collection occurs, transferring surviving objects to Survivor Spaces.
Survivor Space
A memory area for objects that have survived garbage collection from the Eden Space.
Minor Garbage Collection also examines the survivor objects and moves them to the other survivor space, ensuring one survivor space is always empty.
Old (Tenured) Generation
- A memory pool for objects objects that are long-lived and have survived multiple garbage collections, including those from the Survivor Space, and includes tenured and virtual (reserved) spaces.
Memory Model
Permanent Generation
The Permanent Generation, or "Perm Gen" stores application metadata necessary for the JVM to describe classes and methods.
It is important to note that Perm Gen is separate from the Java Heap memory.
The JVM populates Perm Gen at runtime, based on the classes utilized by the application.
MetaSpace
MetaSpace is a memory space introduced in Java 8 to replace the older Perm Gen space. Here are some key points about MetaSpace:
Dynamic Memory Allocation: MetaSpace can adjust its size dynamically, expanding and contracting based on application demands, unlike the fixed size of Perm Gen. This flexibility reduces the risk of OutOfMemoryError.
Native Memory Usage: It utilizes native memory outside the Java heap for storing class metadata, enhancing memory management efficiency.
Garbage Collection: The garbage collector can remove unused class metadata from Metaspace, improving memory management.
Method Area
The Method Area is part of the space in the Perm Gen and is used to store class structure, including runtime constants and static blocks/variables, as well as code for methods and constructors.
Method is part of MetaSpace from Java 8.
Memory Pool
Why Memory Pools are Created:
To efficiently manage and optimize memory usage by pooling immutable objects.
To reduce memory overhead and improve performance by reusing objects.
What Memory Pools Are:
Collections of immutable objects managed by JVM memory managers.
Examples include the String Pool, which stores string literals.
How Memory Pools Function:
Memory Pools can be part of either the Heap or Perm Gen, depending on the JVM implementation.
They allow for the reuse of objects, minimizing the need for frequent memory allocation and garbage collection.
Runtime Constant Pool
The Runtime Constant Pool is a per-class runtime representation of the constant pool found in a class.
It includes runtime constants and static methods specific to the class.
The Runtime Constant Pool is a component of the Method Area.
Stack Memory
Java Stack memory is utilized for thread execution. Each thread has it’s own runtime stack.
It stores method-specific values that are short-lived and references to heap objects used by the method.
Stack memory operates in a Last-In-First-Out (LIFO) order.
A new block is created in the stack memory each time a method is invoked to hold local primitive values and object references.
Once a method completes, its block is freed and available for reuse by subsequent methods.
Stack memory is significantly smaller in size compared to Heap memory.
Garbage Collection
Consider a program that reads large amounts of data from a network and writes it to a database on a hard drive. Typically, the data is read into a collection in memory, processed, and then written to the database. After writing, the collection must be cleared or recreated before processing the next batch. This operation may be repeated thousands of times. Hence, a process is required to identify and reclaim memory that is no longer in use by the program, specifically memory occupied by objects that are no longer referenced. This process is known as Garbage Collection. Garbage Collection is a form of automatic memory management used in Java. This process helps in preventing memory leaks and optimizing the available memory, allowing the program to run efficiently without manual intervention to free up memory. The garbage collector periodically scans the memory, identifies unused objects, and frees up the space they occupy, making it available for future allocations.
All garbage collection events in Java pause application threads until the process is finished, known as "Stop the World" events.
Minor GC:
Occurs in the Young Generation, which holds short-lived objects.
Typically fast and has minimal impact on application performance.
Major GC:
Involves the Old (Tenured) Generation, where long-lived objects reside.
Takes longer as it checks all live objects, potentially causing application unresponsiveness.
Frequent Major GC events can lead to timeout errors, so monitoring and tuning are essential for responsive applications.
Conclusion
In conclusion, Java's memory management plays a crucial role in ensuring the performance quality of applications. By effectively managing resources through the Java Memory Model, including Heap Space, MetaSpace, and Stack Memory, Java optimizes memory usage and enhances application responsiveness. Understanding these components allows developers to write applications that maintain high performance standards, minimizing latency and maximizing throughput. The automatic garbage collection process further contributes to performance quality by efficiently reclaiming unused memory, preventing memory leaks, and ensuring that applications run smoothly over time. This comprehensive memory management system is essential for delivering high-quality, performant Java applications that meet user expectations.
Subscribe to my newsletter
Read articles from Himanshu Soni directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
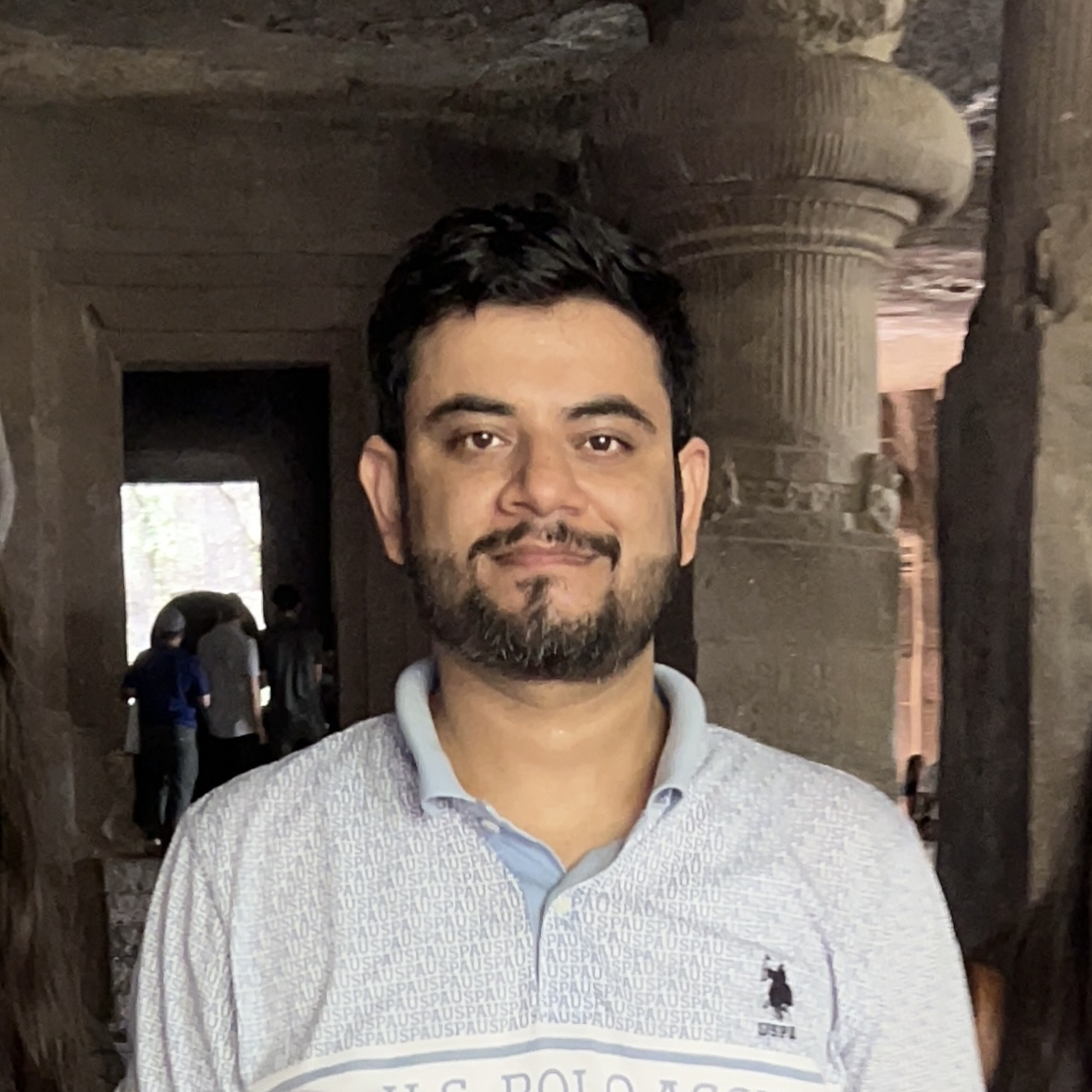
Himanshu Soni
Himanshu Soni
Seasoned QA Engineer with experience in Software Testing across Banking, Travel, and Telecom domains.