🔥 JavaScript Exposed: 7 Mind-Blowing Concepts That Will Transform Your Coding Forever!

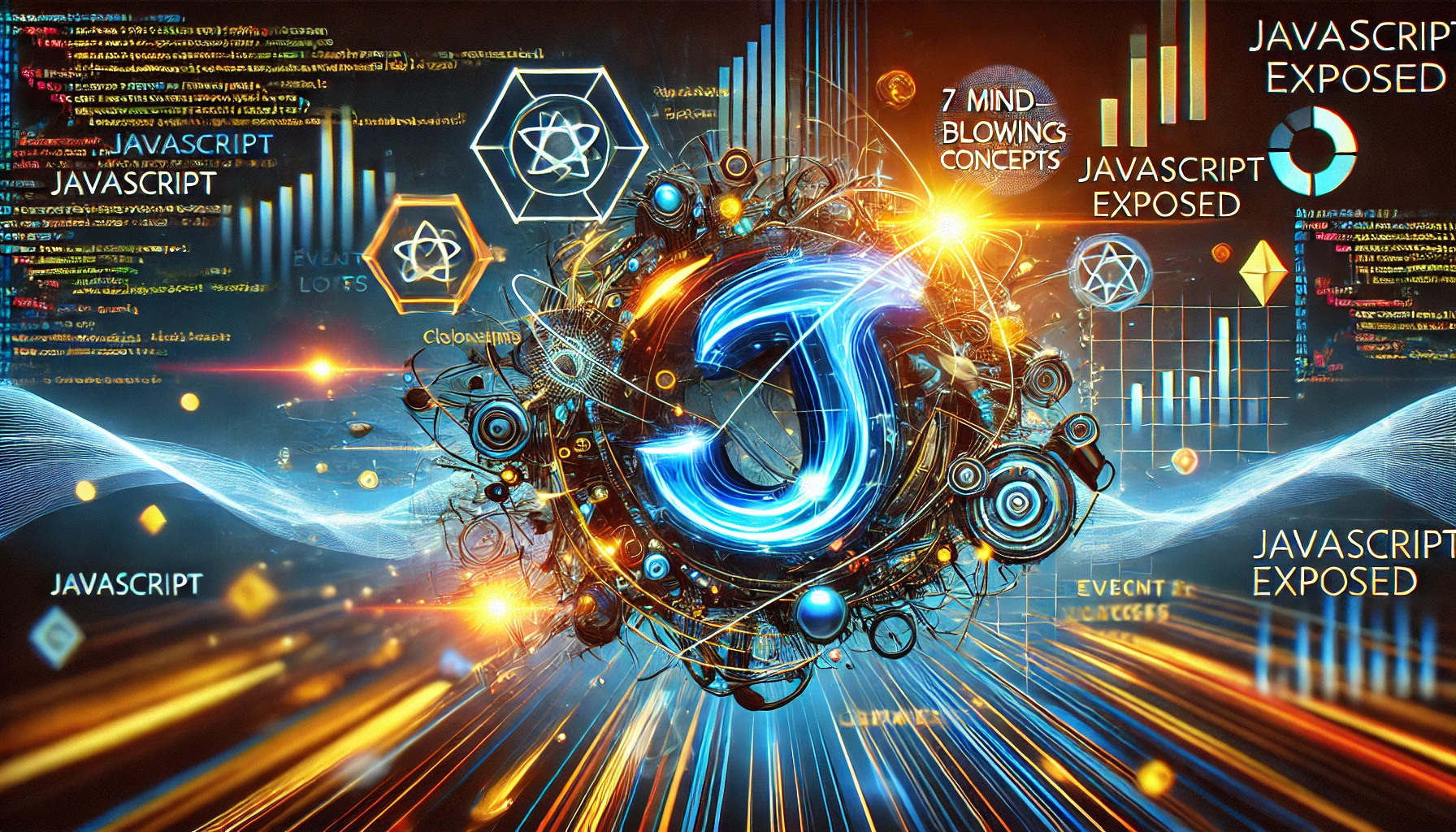
Welcome, fellow developer, to a deep dive into some of JavaScript's most advanced topics! Whether you're a seasoned pro or still finding your footing, this guide is packed with insights, code snippets, and visual diagrams to help you unlock the language's hidden superpowers. Ready to explore the magic behind call
, bind
, apply
, closures, debouncing, and more? Let's get started!
1. Call, Bind, Apply – Deep Dive
One of the cornerstones of JavaScript is how functions handle context. Ever wondered how you can change the this
value on the fly? Enter call
, bind
, and apply
.
What Are They?
call
, apply
, and bind
are powerful methods in JavaScript that help you control function execution context:
call
: Invokes a function with a giventhis
value and arguments provided individually.apply
: Similar tocall
, but takes arguments as an array.bind
: Returns a new function with a boundthis
value.
Code Example
const person = {
name: "Alice",
};
function greet(greeting, punctuation) {
console.log(`${greeting}, ${this.name}${punctuation}`);
}
// Using call
greet.call(person, "Hello", "!"); // Hello, Alice!
// Using apply
greet.apply(person, ["Hi", "!!"]); // Hi, Alice!!
// Using bind
const greetAlice = greet.bind(person, "Hey");
greetAlice("?"); // Hey, Alice?
Context Binding Flowchart
flowchart TD
A[Function Definition]
B[Call: Immediate Invocation]
C[Apply: Immediate Invocation with Array of Args]
D[Bind: Returns New Bound Function]
A --> B
A --> C
A --> D
Using these methods effectively can make your code more flexible and reusable. But how often do you really consider context when designing your functions? It's a game-changer once you start!
2. Debouncing and Throttling in JavaScript
Have you ever experienced lag or performance issues on a web page due to repeated function calls? Enter debouncing and throttling—techniques designed to improve performance by limiting how often a function executes.
Debouncing
Debouncing ensures that a function is only executed after a specified amount of time has passed since the last invocation. Perfect for events like window resizing or text input where rapid-fire events are common.
Code Example: Debounce Implementation
function debounce(func, delay) {
let timeoutId;
return function (...args) {
// Clear previous timeout
clearTimeout(timeoutId);
// Set a new timeout
timeoutId = setTimeout(() => {
// Apply the function with the current context and arguments
func.apply(this, args);
}, delay);
};
}
const handleResize = debounce(() => {
console.log("Resize event handled!");
}, 300);
window.addEventListener("resize", handleResize);
Throttling
Throttling limits a function to execute only once every specified interval. Ideal for scroll events and other continuous actions.
Code Example: Throttle Implementation
function throttle(func, limit) {
let inThrottle;
return function (...args) {
if (!inThrottle) {
// Execute the function immediately
func.apply(this, args);
inThrottle = true;
// Reset the throttle after the specified time
setTimeout(() => {
inThrottle = false;
}, limit);
}
};
}
const handleScroll = throttle(() => {
console.log("Scroll event handled!");
}, 200);
window.addEventListener("scroll", handleScroll);
Performance Comparison Flowchart
flowchart TD
A[Event Trigger]
B[Debounce: Wait for Pause]
C[Throttle: Limit Frequency]
D[Function Execution]
A --> B
A --> C
B --> D
C --> D
These techniques can drastically improve performance by reducing unnecessary function calls, creating smoother and more efficient web applications.
3. Closures in JavaScript
Closures are one of the most powerful yet often misunderstood features in JavaScript. They allow functions to access variables from an enclosing scope even after that outer function has finished execution.
Simple Closure Example
function createCounter() {
let count = 0;
return function () {
count++;
console.log(`Current count: ${count}`);
};
}
const counter = createCounter();
counter(); // Current count: 1
counter(); // Current count: 2
In this example, the inner function retains access to the count
variable even after createCounter
has completed execution. This persistent memory is what makes closures so powerful for creating private state and data encapsulation.
Call Stack Visualization of Closures
flowchart TD
A["Global Execution Context"]
B["createCounter() Function Call"]
C["Inner Function - Closure Created"]
D["Inner Function Call - counter()"]
E["Accesses count Variable"]
A --> B
B --> C
C --> D
D --> E
This visualization demonstrates how a closure maintains a reference to its lexical environment, preserving variable state across multiple function invocations.
4. Understanding the new
and this
Keyword
The new
keyword creates instances of objects defined by a constructor function, while this
refers to the context in which a function is executed.
Context Examples
function Person(name) {
this.name = name;
}
Person.prototype.greet = function () {
console.log(`Hello, my name is ${this.name}`);
};
const bob = new Person("Bob");
bob.greet(); // Hello, my name is Bob
Context Change Flowchart
flowchart LR
A[Function Invocation Context]
B[Global Context]
C[Method Context]
D[Constructor Context]
E[Explicit Binding]
A --> B[window/global object]
A --> C[Object method owner]
A --> D[New object instance]
A --> E[Specified by call/bind/apply]
5. JavaScript Modules
ES6 introduced a native module system with import
and export
statements. Modules are loaded asynchronously and maintain their own scope.
Module Example
math.js
export function add(a, b) {
return a + b;
}
export const PI = 3.14159;
app.js
import { add, PI } from "./math.js";
console.log(`Sum: ${add(2, 3)}`); // Sum: 5
console.log(`Value of PI: ${PI}`);
6. Error Handling in JavaScript
Robust error handling ensures your application remains resilient and provides meaningful feedback.
Error Handling Example
class CustomError extends Error {
constructor(message) {
super(message);
this.name = "CustomError";
}
}
function riskyOperation() {
throw new CustomError("Operation failed!");
}
try {
let result = riskyOperation();
console.log(result);
} catch (error) {
console.error("An error occurred:", error);
}
Final Thoughts
Throughout this exploration, we've uncovered advanced JavaScript topics that can transform your coding approach. From understanding function contexts to implementing performance optimization techniques, you're now equipped with powerful tools to write more efficient and maintainable code.
Remember, mastering these concepts is a journey. Keep practicing, experimenting, and staying curious about the nuances of JavaScript.
Happy coding, and may your JavaScript adventures be both enlightening and bug-free!
Stay tuned for more deep dives and insider tips on modern web development. If you enjoyed this guide, share it with your fellow developers!
Subscribe to my newsletter
Read articles from Jatin Verma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
