A Guide to Using Singleton Design Pattern in Android

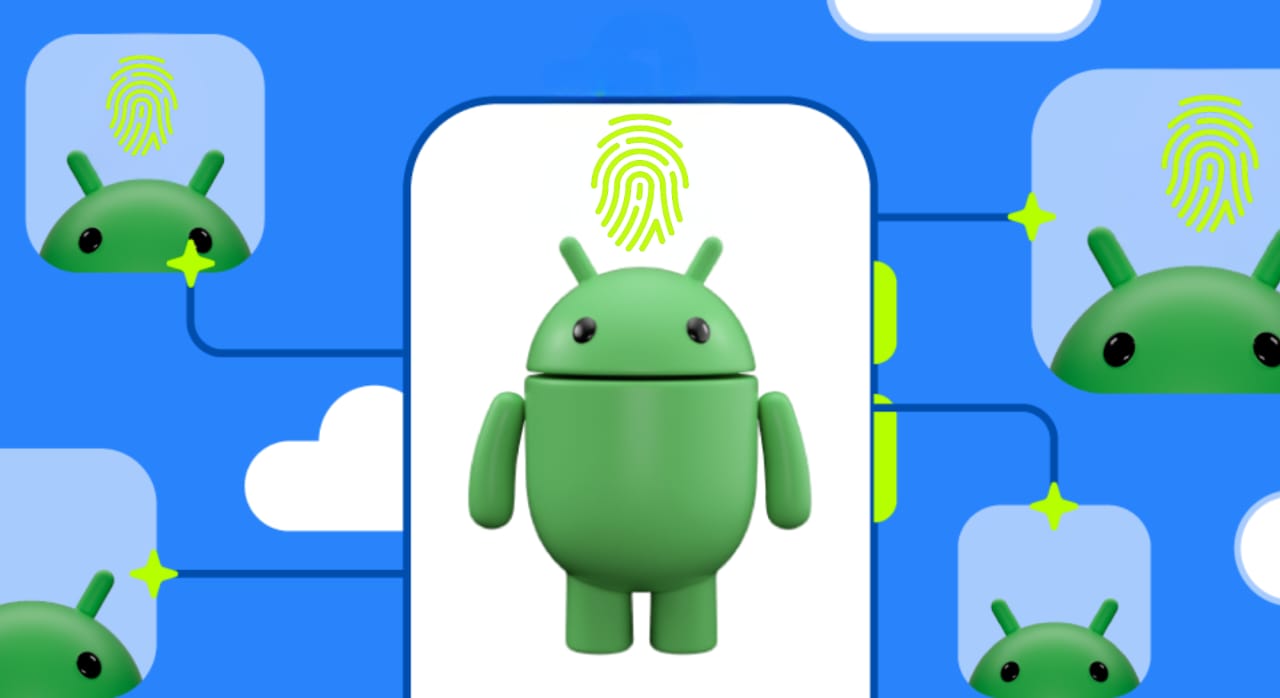
The Singleton pattern is one of the most commonly used design patterns in Android development. It ensures that a class has only one instance throughout the application's lifecycle and provides a global point of access to that instance.
Imagine a scenario where Class A is used in both Class B and Class C. Without the Singleton pattern, each class would need to create its own instance of Class A, leading to redundant object creation.
Now, if the number of dependent classes increases, multiple instances of Class A would be created unnecessarily, resulting in inefficient memory usage.
This is where the Singleton pattern comes into play. Instead of creating multiple instances, we create a single instance of Class A and share it across all dependent classes, ensuring efficient resource utilization.
Why Use Singleton Pattern?
Prevents multiple unnecessary instances.
Ensures a single shared instance is accessible across different parts of the application.
Useful for managing shared resources like database connections or network clients
Here is an example code of how you will create a singleton instance of any class in Java
public class A {
private static A instance;
private A() {}
public static synchronized A getInstance() {
if (instance == null) {
instance = new A();
}
return instance;
}
public void doSomething() {
System.out.println("Using Singleton Instance of A");
}
}
The synchronized
keyword ensures thread safety by allowing only one thread at a time to execute this method.
If multiple threads call getInstance()
simultaneously, synchronized
ensures that only one thread enters the method at a time.
This prevents race conditions, where multiple threads might create multiple instances, violating the Singleton pattern.
Singleton Implementation in Kotlin
In Kotlin, we can achieve the same Singleton behavior using the object
declaration, which ensures thread safety and avoids unnecessary boilerplate code.
object A {
fun doSomething() {
println("Using Singleton Instance of A")
}
}
Here, A is a Singleton because object
in Kotlin automatically creates a single instance.
Some examples of Singleton in Android
object NetworkClient {
private val retrofit: Retrofit = Retrofit.Builder()
.baseUrl("https://api.example.com/")
.addConverterFactory(GsonConverterFactory.create())
.build()
fun getRetrofitInstance(): Retrofit {
return retrofit
}
}
Here, NetworkClient
is a Singleton that provides a single instance of Retrofit
across the app.
Disadvantages of using Singleton Pattern :
Multiple classes depending on a shared instance can make testing and modifications harder.
Holding a reference to an Activity or Context can cause memory leaks if not handled properly.
Since Singletons act as global state, dependencies may not be explicitly visible, making debugging difficult.
Improper implementation can lead to race conditions or unnecessary synchronization overhead, affecting performance.
That’s all from me for today 😁. If I am lacking somewhere or this article needs some correction just comment down. Byeeee!!!👋
Follow me on socials :
X , LinkedIn , Github, Butterfly , Linktree
Subscribe to my newsletter
Read articles from Shalen Mathew directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Shalen Mathew
Shalen Mathew
I write about Android dev & Open Source