Mastering the Strategy Pattern in Java — A Practical Guide for Developers
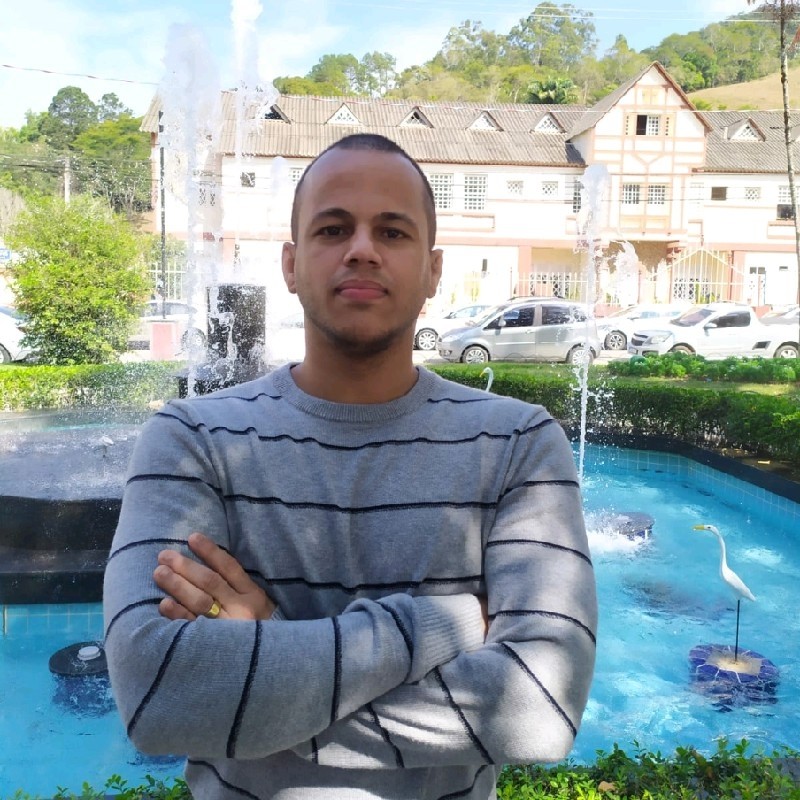
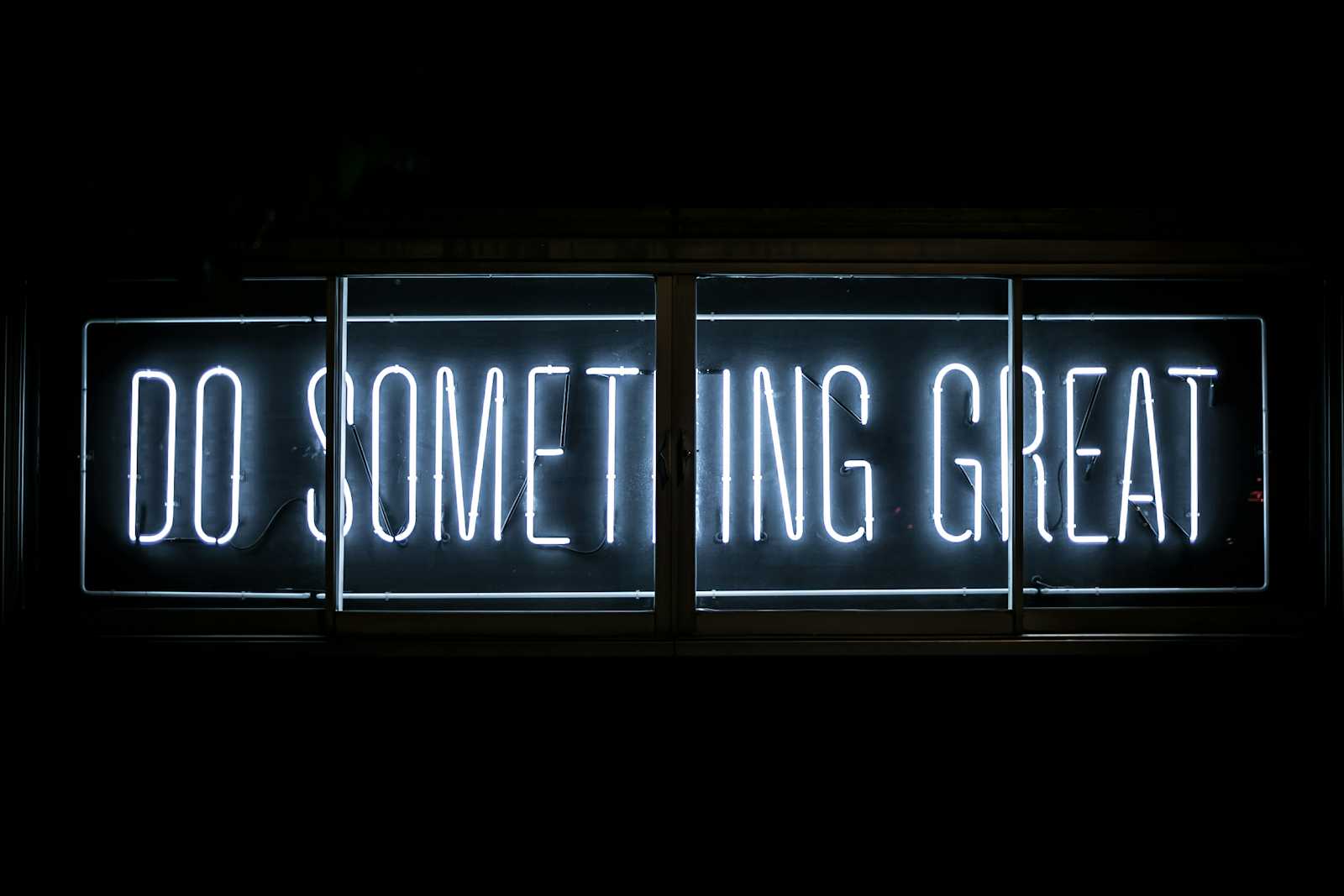
In our daily work as developers, we often find ourselves writing conditional logic that grows endlessly as new requirements appear. This is where the Strategy Pattern comes to the rescue.
In this post, I’ll guide you through:
✅ What the Strategy Pattern is
✅ When to use (and not use) it
✅ Common pitfalls without Strategy
✅ 3 real-world examples refactored using Strategy
🧠 What is the Strategy Pattern?
The Strategy Pattern is a behavioral design pattern that enables selecting an algorithm's behavior at runtime.
It does this by:
Defining a family of algorithms (strategies)
Encapsulating each one in its own class
Making them interchangeable through composition
Instead of hardcoding logic with if-else
or switch
, you delegate the responsibility to strategy implementations.
✅ Pros of the Strategy Pattern
➕ Scalability: Easily add new strategies without changing existing code.
➕ Open/Closed Principle: Your code is open for extension, closed for modification.
➕ Separation of concerns: Each strategy has a single responsibility.
❌ Cons of the Strategy Pattern
➖ More classes: You’ll create more files, which can feel verbose for small use cases.
➖ Requires good design upfront: Improper use may over-engineer simple problems.
➖ Dependency management: You may need a factory (wait for the next post!) or configuration to wire strategies.
🚦 When to use it
You have multiple algorithms or behaviors that could vary at runtime.
You’re using
if-else
orswitch
blocks that keep growing.You need to follow the Open/Closed Principle in business logic.
🛑 When not to use it
When there's only one behavior and no chance of variation.
When you don’t expect the logic to change or grow.
If the overhead of extra classes outweighs the benefit.
💥 Example 1 — Without Strategy
Let’s say we calculate different types of discounts:
public class DiscountService {
public double applyDiscount(String type, double price) {
if ("BLACKFRIDAY".equals(type)) {
return price * 0.5;
} else if ("CHRISTMAS".equals(type)) {
return price * 0.8;
} else if ("NEWYEAR".equals(type)) {
return price * 0.9;
}
return price;
}
}
🧨 Problem:
Adding new discounts requires editing the method.
Violates the Open/Closed Principle.
Becomes harder to maintain.
✅ Refactored with Strategy
Step 1 — Create the strategy interface:
public interface DiscountStrategy {
double apply(double price);
}
Step 2 — Implement strategies:
public class BlackFridayDiscount implements DiscountStrategy {
public double apply(double price) {
return price * 0.5;
}
}
public class ChristmasDiscount implements DiscountStrategy {
public double apply(double price) {
return price * 0.8;
}
}
public class NewYearDiscount implements DiscountStrategy {
public double apply(double price) {
return price * 0.9;
}
}
Step 3 — Context class:
public class DiscountService {
private final DiscountStrategy strategy;
public DiscountService(DiscountStrategy strategy) {
this.strategy = strategy;
}
public double applyDiscount(double price) {
return strategy.apply(price);
}
}
✨ Result:
Clean separation of discount types.
Easy to add new strategies.
No need to touch existing code.
💥 Example 2 — Without Strategy: File Exporter
public class FileExporter {
public void export(String type, List<String> data) {
if ("CSV".equals(type)) {
// export as CSV
} else if ("XML".equals(type)) {
// export as XML
} else if ("JSON".equals(type)) {
// export as JSON
}
}
}
👎 Problem:
Hard to test or extend.
Logic is coupled and grows with each new type.
✅ With Strategy: File Exporter
public interface ExportStrategy {
void export(List<String> data);
}
public class CsvExportStrategy implements ExportStrategy {
public void export(List<String> data) {
// Export to CSV
}
}
public class JsonExportStrategy implements ExportStrategy {
public void export(List<String> data) {
// Export to JSON
}
}
public class FileExporter {
private final ExportStrategy strategy;
public FileExporter(ExportStrategy strategy) {
this.strategy = strategy;
}
public void export(List<String> data) {
strategy.export(data);
}
}
✅ Now you can easily switch exporters without touching the core logic.
💥 Example 3 — Without Strategy: Payment Method
public class PaymentService {
public void process(String method, double amount) {
if ("CREDIT_CARD".equals(method)) {
// credit card logic
} else if ("PAYPAL".equals(method)) {
// PayPal logic
} else if ("PIX".equals(method)) {
// Pix logic
}
}
}
🔁 What if tomorrow they want Apple Pay or crypto?
✅ With Strategy: Payment Method
public interface PaymentStrategy {
void pay(double amount);
}
public class CreditCardPayment implements PaymentStrategy {
public void pay(double amount) {
// logic here
}
}
public class PaymentService {
private final PaymentStrategy strategy;
public PaymentService(PaymentStrategy strategy) {
this.strategy = strategy;
}
public void process(double amount) {
strategy.pay(amount);
}
}
🔥 Easy to plug and play new payment methods without changing core logic.
🎯 Conclusion
The Strategy Pattern empowers you to write cleaner, scalable, and maintainable code. Especially in real-world applications where business logic evolves, this pattern avoids the trap of long conditional chains and prepares your code for growth.
If you're a junior dev, I encourage you to practice converting messy conditionals into strategy-based designs. You'll be surprised how much cleaner and extensible your code becomes.
💬 Have you used Strategy in your projects? Drop your thoughts or questions below — let’s learn together!
Subscribe to my newsletter
Read articles from André Felipe Costa Bento directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
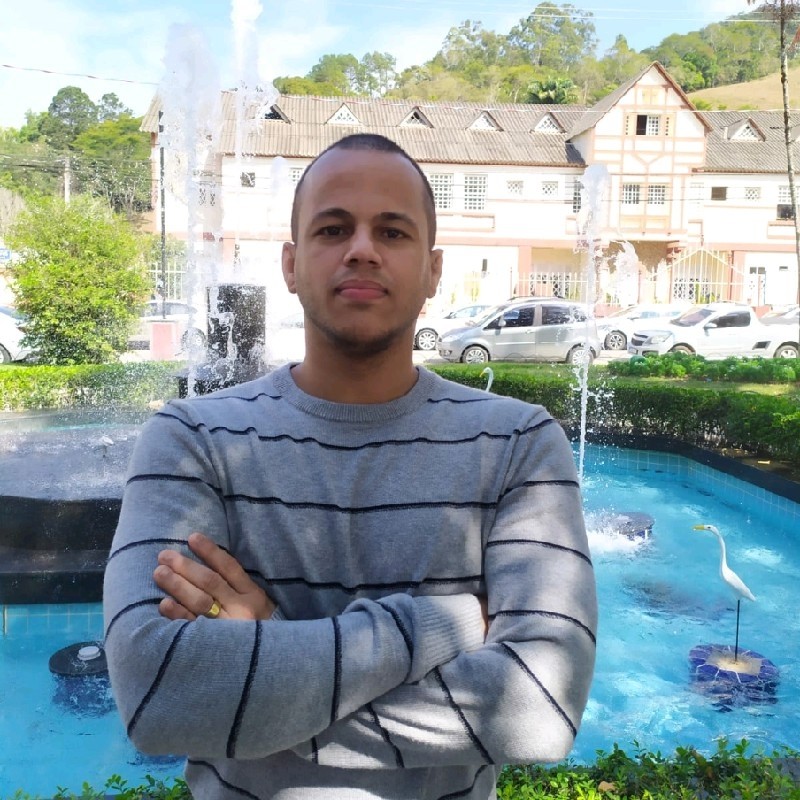
André Felipe Costa Bento
André Felipe Costa Bento
Fullstack Software Engineer.