Keep Your Data Safe: Implementing SQLite Encryption with SQLCipher

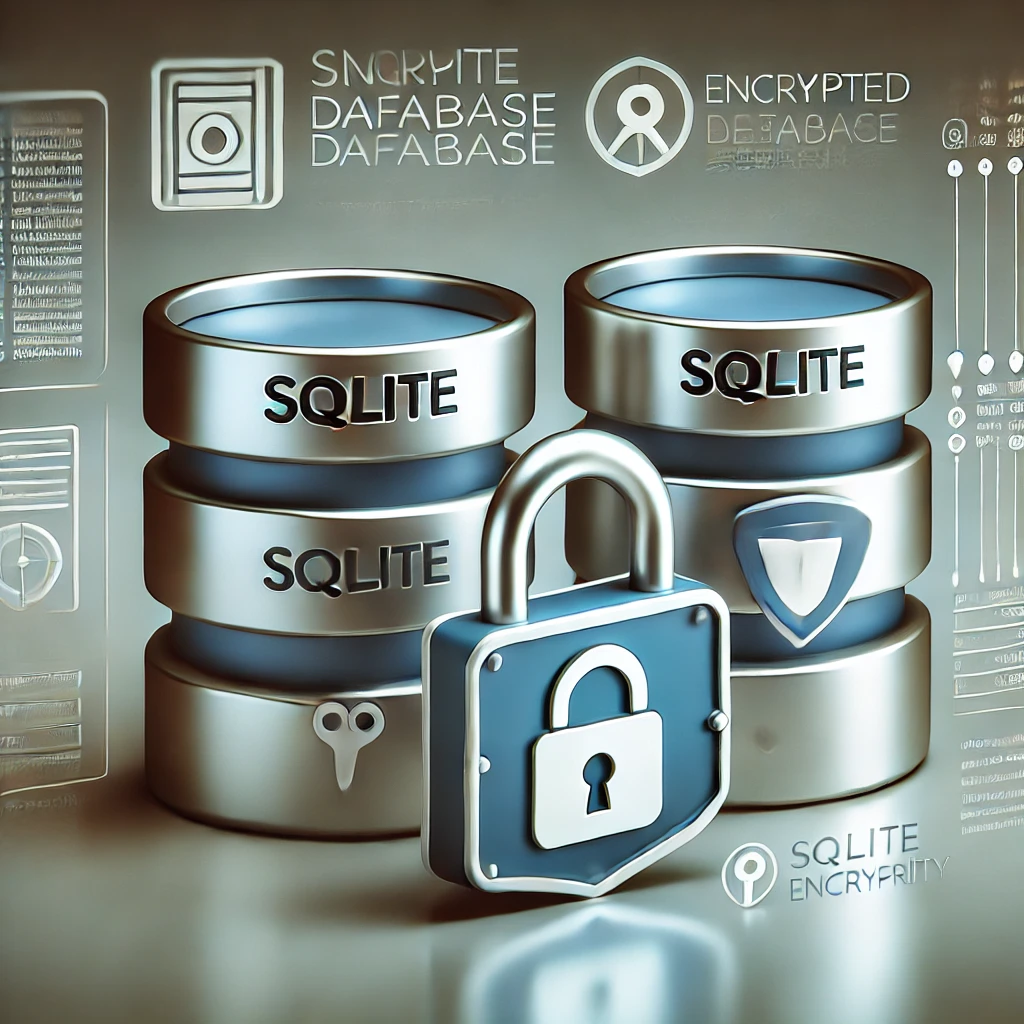
Due to its simplicity and portability, developers frequently use SQLite as a lightweight, file-based database. Being file-based, SQLite allows for easy transfer and viewing of the entire database, which is convenient but also poses significant security risks. Without proper security measures, sensitive data stored within these databases, such as user credentials or personal information, can be easily accessed if the database file is compromised. To mitigate this risk, it is crucial to add a password and implement encryption for the database. Encryption ensures that even if the database file is obtained, the data remains secure and inaccessible without the correct credentials.
Additionally, encrypting the SQLite database is important for regulatory compliance, such as adhering to GDPR or HIPAA, which mandate data protection standards. While encryption might slightly affect performance, the trade-off is necessary for applications that handle sensitive data. Tools like SQLCipher offer a straightforward way to integrate encryption into SQLite, providing a seamless solution for developers to prioritize data security without drastically altering their workflow. Thus, adding encryption and a password to an SQLite database is an essential step to protect data from unauthorized access and ensure the safety of critical information.
To integrate SQLCipher into your SQLite database and ensure encryption, follow these steps. SQLCipher is an open-source extension that provides transparent 256-bit AES encryption for SQLite databases. Here’s how you can get started with integrating SQLCipher into your project:
1. Install SQLCipher
For Linux or macOS:
You can install SQLCipher using the package manager Homebrew
(macOS) or manually compile it (Linux).
# Install SQLCipher via Homebrew (macOS)
brew install sqlcipher
For Linux, the steps are similar but might require you to compile from source:
sudo apt-get install libsqlcipher-dev
For Windows:
You can download precompiled binaries or use package managers like vcpkg:
vcpkg install sqlcipher
2. Set Up SQLCipher in Python (or any language)
You can integrate SQLCipher with SQLite in Python by using the pysqlcipher3
library. This library adds SQLCipher support to SQLite.
Install the Python Library:
pip install pysqlcipher3
Example Python Script Using SQLCipher:
from pysqlcipher3 import dbapi2 as sqlite
# Connect to the database
conn = sqlite.connect('encrypted_database.db')
# Enable encryption
conn.execute("PRAGMA key = 'your_secret_password'")
# Create a table
conn.execute('CREATE TABLE IF NOT EXISTS users (id INTEGER PRIMARY KEY, username TEXT, password TEXT)')
# Insert some data
conn.execute("INSERT INTO users (username, password) VALUES (?, ?)", ('user1', 'password1'))
conn.commit()
# Close connection
conn.close()
This example script sets up a database, encrypts it using SQLCipher, and performs basic operations like table creation and data insertion.
3. Encrypt an Existing SQLite Database
If you have an existing SQLite database and you want to encrypt it using SQLCipher, follow these steps:
Open the existing unencrypted database.
Attach a new encrypted database.
Export the data from the unencrypted database to the encrypted one.
$ sqlcipher my_existing_db.db
sqlite> ATTACH DATABASE 'encrypted_db.db' AS encrypted KEY 'your_secret_password';
sqlite> SELECT sqlcipher_export('encrypted');
sqlite> DETACH DATABASE encrypted;
This process converts the database from unencrypted to encrypted using the provided password.
4. Use SQLCipher Commands for Database Security
You can run the following SQLCipher commands in your SQLite session to ensure security:
PRAGMA key = 'your_password';
This is the key that encrypts or decrypts the database.PRAGMA cipher_version;
Check the SQLCipher version you're using.PRAGMA cipher_default_kdf_iter = 64000;
This can be used to specify the number of key derivation function iterations for security hardening.
5. Testing Encrypted Database
To test if the database is truly encrypted, try opening it without the password. SQLCipher-encrypted databases cannot be opened with regular SQLite tools without providing the decryption key.
To test whether your SQLite database is properly encrypted using SQLCipher, you can use the hexdump
command to view the raw contents of the database file. If the file is encrypted, you should not be able to discern any readable plain-text data like table or column names.
Here’s how to test it:
1. Create the Encrypted Database
First, ensure that your database is encrypted using SQLCipher. For example, using the Python script provided earlier or using sqlcipher
directly:
$ sqlcipher encrypted_db.db
sqlite> PRAGMA key = 'your_secret_password';
sqlite> CREATE TABLE test (id INTEGER PRIMARY KEY, data TEXT);
sqlite> INSERT INTO test (data) VALUES ('Sensitive Information');
sqlite> .exit
2. Use hexdump
to Inspect the Encrypted Database
hexdump
allows you to view the raw hexadecimal data of the file, which can reveal whether the data is encrypted or not. To inspect the database:
hexdump -C encrypted_db.db | head -n 20
3. Interpreting the Output
For an Encrypted Database: The output should look like random data. You should not see human-readable information, such as tables, column names, or data.
Example of an encrypted database output:
00000000 fa 41 8b 39 92 6e 54 73 f2 a1 72 9d 9f b5 13 8e |.A.9.nTs..r.....| 00000010 9a 13 97 e7 9b d6 95 b3 e8 5b 48 b7 9a 6c 6f 8d |.........[H..lo.| 00000020 7a 9d 8b e9 64 49 a6 d5 92 14 a6 73 6d 6d a2 87 |z...dI.....smm..| 00000030 a1 6f b7 a1 72 71 b5 a8 39 f2 82 76 93 a7 8d 94 |.o..rq..9..v....|
For an Unencrypted Database: If the database is not encrypted, you will see readable text such as SQL statements, table names, and possibly even the data you’ve inserted.
Example of an unencrypted database output:
00000000 53 51 4c 69 74 65 20 66 6f 72 6d 61 74 20 33 00 |SQLite format 3.| 00000010 04 00 01 01 00 40 20 20 00 00 04 00 00 00 00 00 |.....@ ........| 00000020 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 |................| 00000030 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 |................| 00000040 00 00 00 00 00 00 00 00 00 2e 64 61 74 61 00 01 |.........data...|
4. Testing an Unencrypted Database
To compare, if you create a database without encryption and run the hexdump
command, you’ll be able to see the clear-text content, which shows how easily readable a non-encrypted SQLite database can be:
sqlite3 unencrypted_db.db
sqlite> CREATE TABLE test (id INTEGER PRIMARY KEY, data TEXT);
sqlite> INSERT INTO test (data) VALUES ('Sensitive Information');
sqlite> .exit
Then run:
hexdump -C unencrypted_db.db | head -n 20
You will likely see readable text such as:
00000000 53 51 4c 69 74 65 20 66 6f 72 6d 61 74 20 33 00 |SQLite format 3.|
00000010 04 00 01 01 00 40 20 20 00 00 04 00 00 00 00 00 |.....@ ........|
00000020 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 |................|
00000030 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 |................|
00000040 00 00 00 00 00 00 00 00 00 2e 53 65 6e 73 69 74 |.........Sensit|
6. Deploy SQLCipher in Production
Once you have encrypted your database, you can deploy it with your application. SQLCipher works seamlessly with existing SQLite applications, with the additional benefit of encryption.
Conclusion:
By integrating SQLCipher, you enhance the security of your SQLite databases with encryption. Whether you're handling sensitive user information or confidential data, SQLCipher ensures your SQLite databases remain protected from unauthorized access. Use this powerful tool to bolster data security, ensuring compliance with regulations like GDPR or HIPAA.
Happy coding! 😊
Subscribe to my newsletter
Read articles from Jeevan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
