How to check Unused AWS Elastic IP’s with the help of CLI
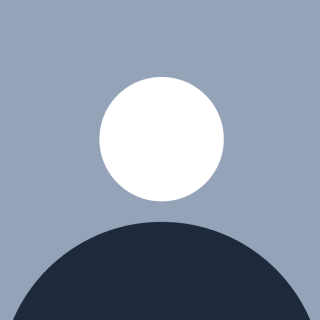
Overview:
This Bash script is designed to interact with AWS EC2 services using the AWS CLI. It retrieves a list of all Elastic IPs (EIPs) in a specified AWS region, then identifies which EIPs are in use and which are unused. The results are displayed in a clear and user-friendly format.
Purpose:
The purpose of this script is to help AWS users and administrators quickly assess which Elastic IP addresses are in use and which are available for reassignment or release. This can help reduce unused resources and avoid unnecessary costs.
Use Cases:
Cost Optimization: Unused Elastic IPs in AWS incur a cost. This script helps you identify these IPs so that they can be released and avoid unnecessary charges.
Resource Management: By identifying unused Elastic IPs, administrators can better manage their resources, ensuring that only necessary resources are allocated.
Automation: This script can be scheduled to run periodically to keep track of Elastic IP usage.
Prerequisites:
Before using this script, ensure that you meet the following requirements:
AWS CLI installed: Ensure that the AWS CLI is installed on the machine running this script.
You can install it following the AWS CLI installation guide.
AWS credentials configured: The AWS CLI must be configured with the appropriate AWS credentials and permissions to access EC2 services. Run
aws configure
to set up your credentials.Bash environment: The script is written in Bash, so it must be executed in a Bash-compatible shell.
#!/bin/bash
# Set AWS region
REGION="us-east-1" # Replace with your desired region
# Get all Elastic IP allocations
ALL_EIPS=$(aws ec2 describe-addresses --region $REGION --query "Addresses[*].PublicIp" --output text)
# Get used Elastic IPs (those with an association)
USED_EIPS=$(aws ec2 describe-addresses --region $REGION --query "Addresses[?AssociationId!=null].PublicIp" --output text)
# Initialize array for unused Elastic IPs
UNUSED_EIPS=()
# Find unused Elastic IPs
for EIP in $ALL_EIPS; do
if ! grep -q "$EIP" <<< "$USED_EIPS"; then
UNUSED_EIPS+=("$EIP")
fi
done
# Display results
echo "Checking Elastic IP usage in region $REGION..."
echo -e "\n--- Used Elastic IPs ---"
if [[ -n "$USED_EIPS" ]]; then
echo "$USED_EIPS"
else
echo "No used Elastic IPs found."
fi
echo -e "\n--- Unused Elastic IPs ---"
if [[ ${#UNUSED_EIPS[@]} -gt 0 ]]; then
for UNUSED in "${UNUSED_EIPS[@]}"; do
echo "$UNUSED"
done
else
echo "No unused Elastic IPs found."
fi
Sample Output:
In this example:
The used Elastic IP (
3.238.123.45
) is associated with an EC2 instance or other resource.Two Elastic IPs (
54.239.12.34
,34.201.65.98
) are unused and could potentially be released or reassigned
Checking Elastic IP usage in region us-east-1...
--- Used Elastic IPs ---
3.238.123.45
--- Unused Elastic IPs ---
54.239.12.34
34.201.65.98
Customisation:
Region Change: Modify the
REGION
variable to target a different AWS region.AWS CLI Profile: If you’re using a specific AWS CLI profile, you can add the
--profile
option to theaws ec2 describe-addresses
commands.Example:
ALL_EIPS=$(aws ec2 describe-addresses --region $REGION --profile my-profile --query "Addresses[*].PublicIp" --output text)
Conclusion:
This script is a simple yet effective way to monitor and manage the usage of Elastic IP addresses in AWS. By differentiating between used and unused EIPs, it enables you to optimize your AWS resources, potentially saving on costs associated with idle resources.
Subscribe to my newsletter
Read articles from Jignesh Singh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Jignesh Singh
Jignesh Singh
Dedicated and Result driven Cloud Engineer with extensive experience in cloud platforms, including AWS and GCP, and a strong proficiency in Linux systems. Skilled in cloud automation, System Patching, and Monitoring to ensure seamless Infrastructure Management and Optimal Performance. Demonstrates a Solid understanding of Containerization Technologies, with hands-on experience in Docker and Kubernetes.