React's Animation Paradox: Why Your CSS Transitions Break With Props
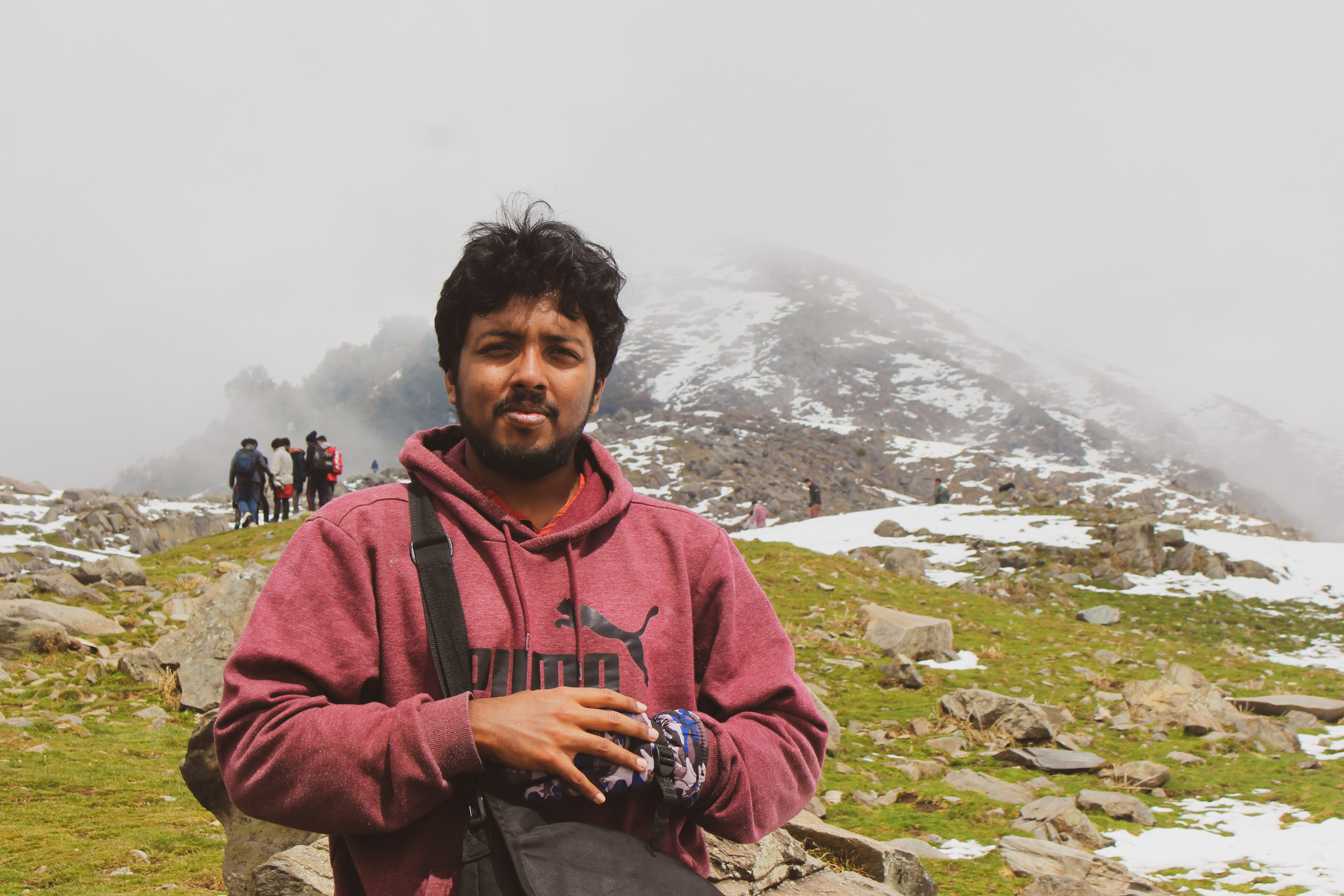
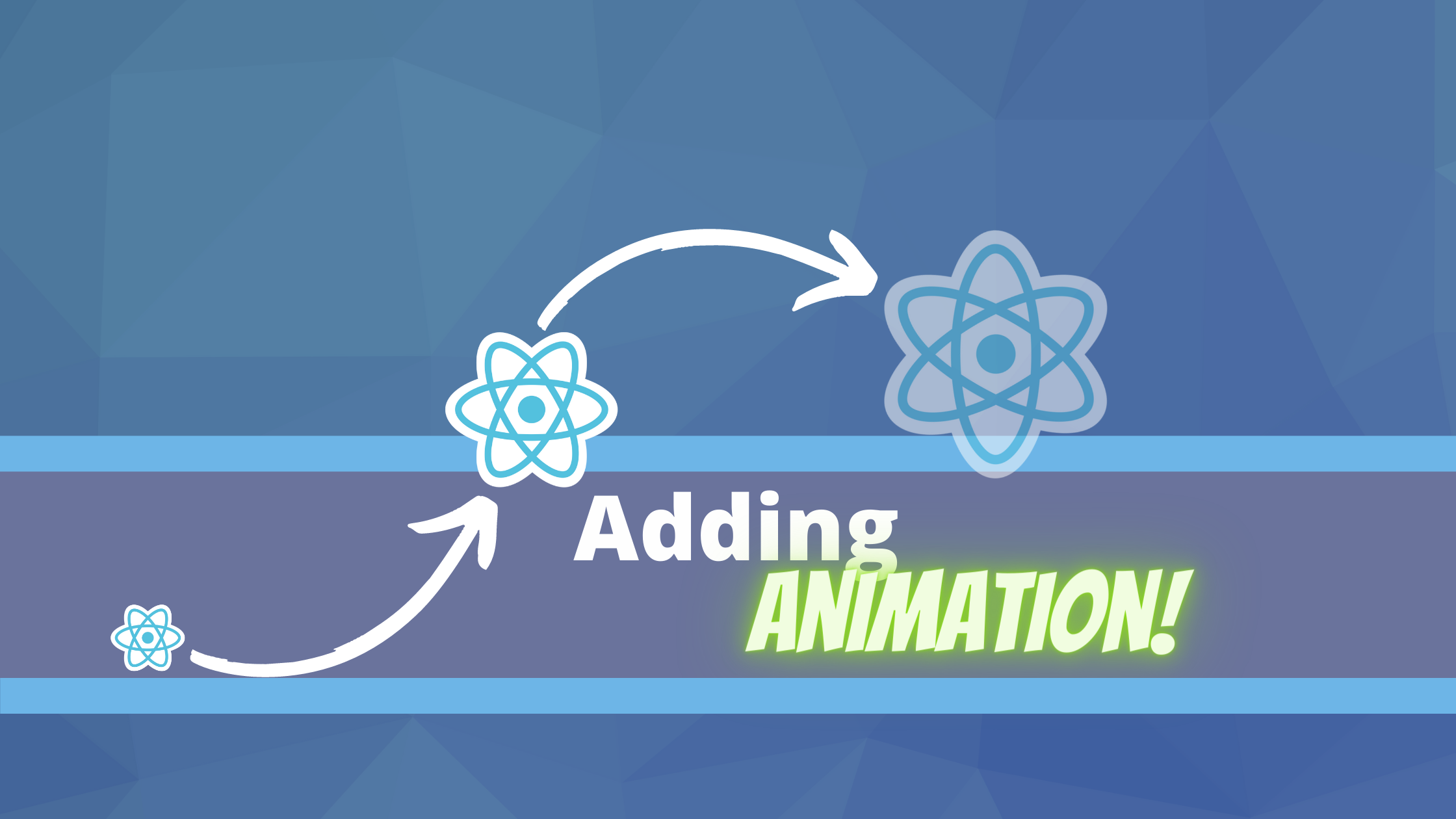
Have you ever built a beautiful React component with smooth animations, only to watch them mysteriously disappear when you refactor to pass down state from a parent component? If you've faced this frustrating scenario, you're not alone. This is a subtle but common issue that exposes some of the inner workings of React's rendering model and its interaction with browser animations.
Let's dive into a real-world example and uncover what's happening behind the scenes.
The Mysterious Case of Disappearing Transitions
Recently, I was working on a data visualization component that displayed activity information with smooth hover animations. The component worked flawlessly with internal state:
const ActivityInfo = ({ cpWidgetData }) => {
const [isHovered, setIsHovered] = useState(false);
const { domains, messages } = cpWidgetData;
return (
<div
onMouseEnter={() => setIsHovered(true)}
onMouseLeave={() => setIsHovered(false)}
>
{/* Icons with CSS transitions based on isHovered */}
<div style={{
opacity: isHovered ? 0 : 1,
transition: "opacity 0.3s"
}}>
<ActivityIcon />
</div>
<div style={{
opacity: isHovered ? 1 : 0,
transition: "opacity 0.3s, transform 0.3s"
}}>
{domains.map((domain, idx) => (
<div
key={idx}
style={{
transform: `translateY(${isHovered ? -(idx * 5) : 0}px)`,
transition: `transform 0.3s ease ${idx * 0.05}s`
}}
>
<Icon />
</div>
))}
</div>
</div>
);
};
Everything worked perfectly. The component smoothly animated elements when users hovered over it. But then came the refactor...
We needed to control the hover state from a parent component for a coordinated UI experience. So we changed the component to accept an isHovered
prop:
const ActivityInfo = ({
cpWidgetData,
isHovered,
onMouseEnter,
onMouseLeave
}) => {
// No local state anymore
const { domains, messages } = cpWidgetData;
return (
<div
onMouseEnter={onMouseEnter}
onMouseLeave={onMouseLeave}
>
{/* Same animations, now based on the prop */}
...
</div>
);
};
And suddenly, our beautiful animations disappeared! Elements would still show and hide, but they would snap instantly between states with no transition. What happened?
The Problem: React's Rendering Model vs. CSS Transitions
To understand this issue, we need to understand how CSS transitions and React's rendering cycle interact.
CSS transitions work by:
Tracking an element's initial state
Noticing when properties change
Animating smoothly between the old and new values
But for this to work, the browser needs to maintain a reference to the same DOM element before and after the change.
When a React component manages its own state:
State changes trigger a re-render
React updates the existing DOM nodes with new properties
The browser sees the property changes on the same elements and animates them
But when props change from a parent:
The parent component re-renders
The child component re-renders with new props
React might optimize by replacing DOM elements rather than updating them
The browser sees new elements appearing (not property changes on existing ones)
No transition occurs because there's no "before" state to animate from
This subtle difference in how React handles internal state changes versus prop changes is at the heart of our animation problem.
The Solution: A Hybrid State Approach
After much experimentation, I discovered a reliable pattern that fixes this issue. It combines three key React features:
Local state management for smooth animations
useEffect to sync with external props
useRef to stabilize DOM elements
Here's the working solution:
const ActivityInfo = ({
cpWidgetData,
isHovered: externalIsHovered,
onMouseEnter,
onMouseLeave
}) => {
// Internal state for animations
const [isHovered, setIsHovered] = useState(externalIsHovered);
// DOM stability reference
const elementRef = useRef(null);
const { domains, messages } = cpWidgetData;
// Sync with external hover state
useEffect(() => {
setIsHovered(externalIsHovered);
}, [externalIsHovered]);
// Local handlers that update internal state AND call parent handlers
const handleMouseEnter = () => {
setIsHovered(true);
onMouseEnter();
};
const handleMouseLeave = () => {
setIsHovered(false);
onMouseLeave();
};
return (
<div
ref={elementRef}
onMouseEnter={handleMouseEnter}
onMouseLeave={handleMouseLeave}
>
{/* Same animations, now based on internal state */}
<div style={{
opacity: isHovered ? 0 : 1,
transition: "opacity 0.3s"
}}>
<ActivityIcon />
</div>
<div style={{
opacity: isHovered ? 1 : 0,
transition: "opacity 0.3s, transform 0.3s"
}}>
{domains.map((domain, idx) => (
<div
key={idx}
style={{
transform: `translateY(${isHovered ? -(idx * 5) : 0}px)`,
transition: `transform 0.3s ease ${idx * 0.05}s`
}}
>
<Icon />
</div>
))}
</div>
</div>
);
};
With this approach, our animations work smoothly again, even when the hover state is controlled by a parent component!
Why This Works: The Technical Deep Dive
Let's break down why each part of this solution is necessary:
1. Internal State
By maintaining our own internal state, we ensure that the component's re-renders are triggered by state changes, which React handles differently than prop changes. This gives the browser a chance to see both the before and after states for animation.
2. useEffect for Synchronization
The useEffect
hook synchronizes our internal state with the external prop. This creates a deliberate delay between receiving new props and updating the DOM, giving the browser time to process transitions.
3. useRef for DOM Stability
This is the least obvious but most critical part. The useRef
ensures that React maintains the same DOM nodes across renders. Without it, React might optimize by replacing DOM elements entirely when props change significantly, which would break animations.
By attaching a ref to our container element, we're telling React: "Keep this DOM node stable, even if you think it should be replaced." This stability is essential for CSS transitions.
The React Rendering Mental Model
To understand this issue fully, we need to update our mental model of how React works:
React doesn't just update DOM properties; it decides whether to update or replace elements
State changes within a component typically lead to updates of existing DOM nodes
Prop changes can sometimes cause React to replace DOM nodes entirely
CSS transitions need stable DOM nodes to work correctly
This explains why animations often work with internal state but break with props. It's not a bug in React—it's a consequence of its optimization strategy colliding with how CSS transitions work.
When To Use This Pattern
You should consider this hybrid state approach when:
Your component has CSS transitions or animations
The animation trigger (like hover state) needs to be controlled by a parent component
You notice animations working with internal state but breaking with props
The pattern adds some complexity, but it's a reliable way to ensure smooth animations in components that need to respond to external state changes.
Conclusion
React's component model and the browser's animation system sometimes have subtle interactions that can lead to unexpected behavior. By understanding these interactions and using patterns like the hybrid state approach, we can build components that maintain smooth animations while fitting into React's parent-child state flow.
The next time your CSS transitions mysteriously disappear after a refactor, remember: it might not be a bug, but a gap between React's rendering model and the browser's animation engine. And now you have the tools to bridge that gap.
Happy animating!
Subscribe to my newsletter
Read articles from Vysyakh Ajith directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
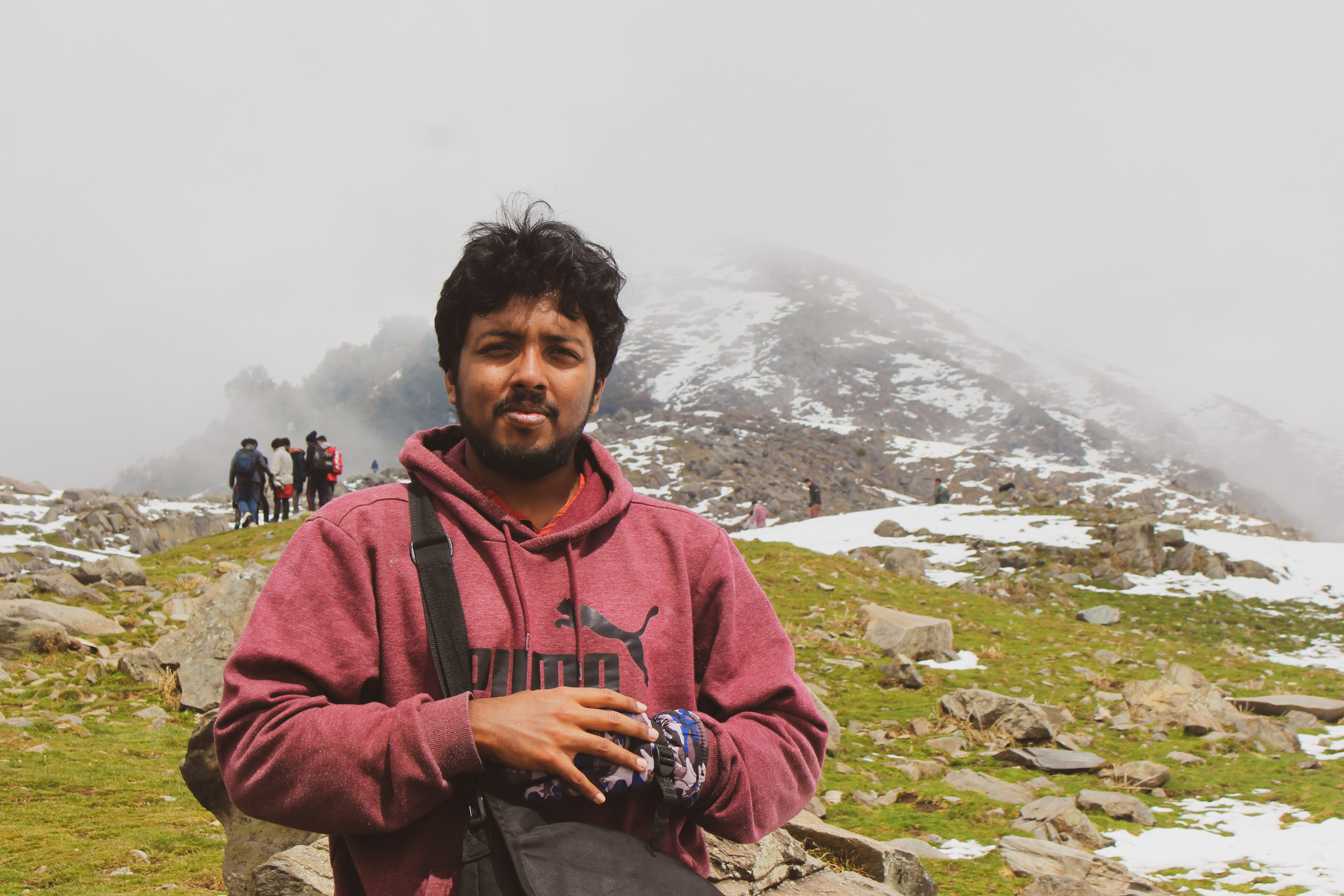
Vysyakh Ajith
Vysyakh Ajith
Aspiring full-stack developer looking forward to create end-to-end reactive web solutions with experience innovation as the motto