How to Build Your First React App from Scratch
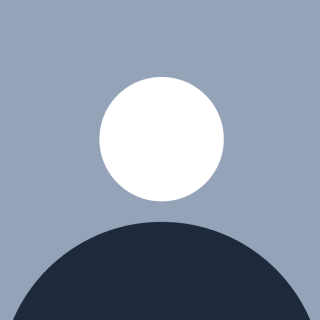
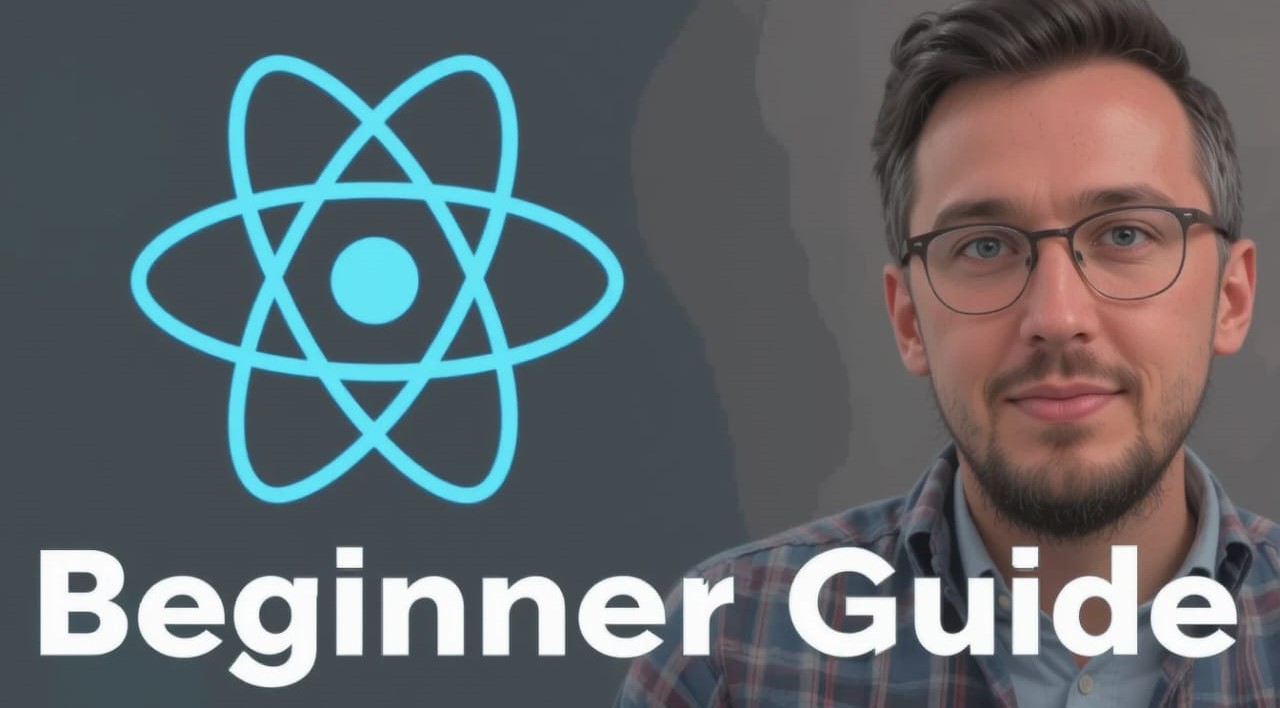
Introduction
React.js is one of the most popular JavaScript libraries for building interactive user interfaces. If you're new to React, building your first app might seem overwhelming, but don’t worry! In this guide, we’ll go step by step to set up a React project, understand its structure, and build a simple app.
Prerequisites
Before we start, make sure you have the following installed on your system :
Node.js (Download from nodejs.org)
npm (Node Package Manager) (comes with Node.js) or yarn
You can check if Node.js and npm are installed by running:
node -v
npm -v
If both return version numbers, you’re good to go!
step 1 : create react app
npx create-react-app my-first-app cd my-first-app
npm start
Your default React app should now be running on http://localhost:3000/
step 2 : create a new component
Inside src/, create a new file Welcome.js :-
import React from 'react';
function Welcome() { return
Welcome to My First React App!
; }
export default Welcome;
step 3 : use the component in app.js
Modify src/App.js :
import React from 'react'; import Welcome from './Welcome';
function App() { return (
Hello, React!
); }
export default App;
step 4 : add basic styling
Modify src/App.css :-
h1 { color: #007bff; text-align: center; } h2 { color: #28a745; text-align: center; }
Import it in App.js:
import './App.css';
step 5 : add interactivity with useState
Modify Welcome.js to add a button that changes text on click :-
import React, { useState } from 'react';
function Welcome() { const [message, setMessage] = useState("Welcome to My First React App!");
return (
{message}
<button onClick={() => setMessage("You clicked the button!")}>Click Me
); }
export default Welcome;
Congratulations! 🎉 You’ve built your first React app from scratch. You learned how to :-
✅ Set up a React project
✅ Create components
✅ Use CSS for styling
✅ Manage state with useState
Now, explore more features like API calls, React Router, and advanced hooks. Keep building and experimenting! 🚀
Need a custom webpage ? Hire me on FIVERR :- Gigs
Subscribe to my newsletter
Read articles from Harshad Solkar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Harshad Solkar
Harshad Solkar
I am an IT student with a strong foundation in FRONTEND DEVELOPMENT and design. With a hands-on experience, I have honed my skills in creating responsive and visually appealing websites. combining technical knowledge and creativity to create user-friendly web solutions.