Getting Started with Go and Gin: A Beginner’s Guide
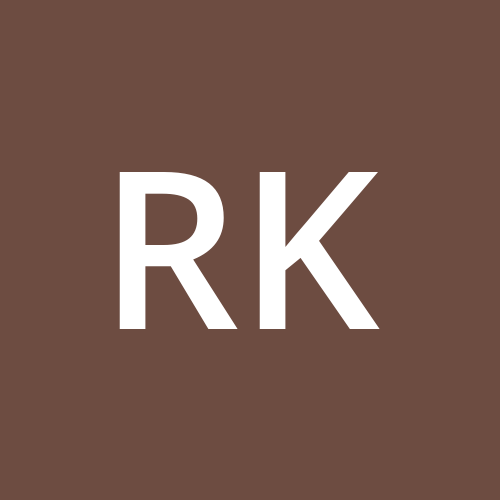
Overview
Go (or Golang) is a simple and powerful programming language designed by Google. It’s known for its speed, simplicity, and built-in support for concurrency (running multiple tasks at the same time). If you're coming from languages like Python or JavaScript, Go might feel a bit different at first, but once you get the hang of it, it’s super fun and efficient to use.
In this post, I’ll introduce Go basics and then show you how to build a simple API using Gin, a lightweight and fast web framework for Go.
1. Writing Your First Go Program
First, install Go from golang.org. Then, create a new file called main.go and write this:
package main
import "fmt"
func main() {
fmt.Println("Hello, Go!")
}
What’s Happening Here?
package main
– Every Go program starts with a package. If your program is meant to run as an executable, use main.import "fmt"
– We’re importing Go’s built-in fmt package, which helps us print text.func main()
– The main function is the entry point of every Go program.fmt.Println("Hello, Go!")
– Prints text to the console.
Run the program:
go run main.go
You should see:
Hello, Go!
2. Variables in Go
Go has strong typing, meaning every variable must have a type. Here are some ways to declare variables:
package main
import "fmt"
func main() {
var name string = "John" // Explicitly declaring type
age := 25 // Go automatically detects it's an integer
fmt.Println("Name:", name)
fmt.Println("Age:", age)
}
var name string = "John"
– Declares a string variable with an explicit type.age := 25
– The:=
operator lets Go infer the type.
3. Getting Started with Gin
Gin is a fast and minimalist web framework for Go. It helps you build APIs quickly. Let’s install and set up a simple API.
Install Gin
Run:
go get -u github.com/gin-gonic/gin
Writing a Basic Gin API
Create a file, main.go and add:
package main
import (
"github.com/gin-gonic/gin"
"net/http"
)
func main() {
r := gin.Default() // Creates a new Gin router
r.GET("/", func(c *gin.Context) {
c.JSON(http.StatusOK, gin.H{"message": "Welcome to my first Gin API!"})
})
r.Run(":8080") // Starts the server on port 8080
}
Run the API:
go run main.go
Now open your browser and go to http://localhost:8080/ – you should see:
{"message": "Welcome to my first Gin API!"}
4. What’s Next?
This is just the beginning! In the next articles, I’ll cover:
Using a database with Go
Go is simple, fast, and fun to learn. If you’re new to backend development, Gin makes it easy to build APIs quickly. I’ll keep documenting everything I learn in this series, so stay tuned!
Have you tried Go before? What was your first impression? Let’s chat in the comments!
Subscribe to my newsletter
Read articles from Racheal Kuranchie directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
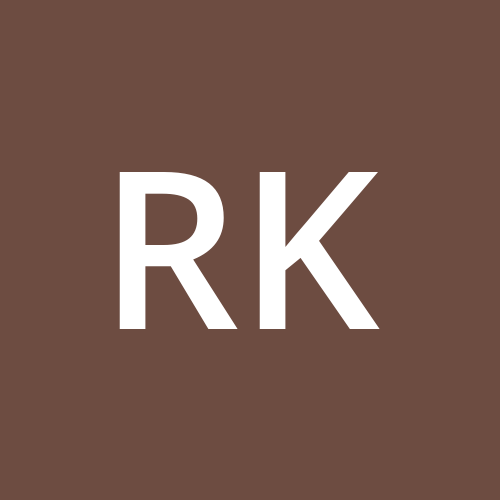