Creation of a Thread

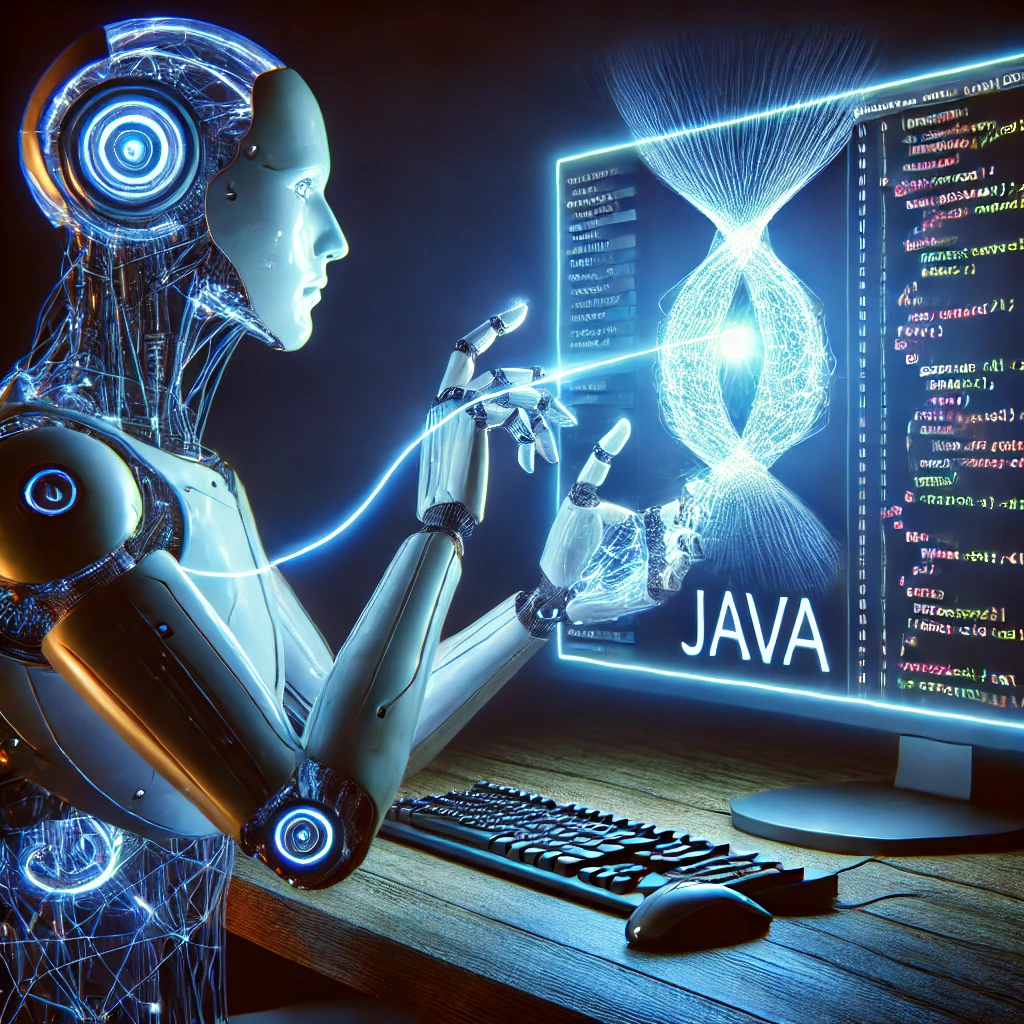
Introduction
By now, I hope you have a basic understanding of multithreading in Java. So, now we will learn how to create threads. Yes, we can create threads in Java! There are four different ways to create threads, and in this blog, we will explore all these four methods. So, let’s begin!
Before we start, I would like to clarify that when I refer to the "thread creation process," I actually mean the different ways to specify what code a Java thread should run.
1. Using the Java Thread Class
In Java, we already have a predefined class called Thread. Using this Thread class, we can specify the code that we want a thread to execute. How? Well, we can create a thread by simply extending the Thread class and overriding the run() method. Within the run() method, we define the code that should be executed by the thread. Here’s a code example:
class MyThread extends Thread {
@Override
public void run() {
System.out.println("Thread is running...");
}
}
public class ThreadExample {
public static void main(String[] args) {
MyThread t1 = new MyThread();
t1.start();
}
}
In the above class, we have created a class called MyThread, which extends the Thread class. Inside the class, we override the run() method. By doing this, we successfully define the code that needs to be executed by a thread.
Now, to run this code using a thread, we simply create an instance of our class in the main() function and call the start() method. This start() method is responsible for executing the code defined inside the run() method of our class.
Note: The start() method actually belongs to the Thread class.
2. Using the Runnable Interface
In Java, just like we have the predefined Thread class to create a thread, we also have a predefined interface called Runnable. By implementing this Runnable interface in our class, we can create threads. Here’s how:
Implement the Runnable interface in the class where you want to define the code for the thread.
Override the run() method, just like we did in the previous method.
Create an instance of the Thread class and pass the object of your class as a parameter.
Call the start() method to begin execution.
Here’s the code example:
class MyRunnable implements Runnable {
@Override
public void run() {
System.out.println("Thread is running...");
}
}
public class RunnableExample {
public static void main(String[] args) {
MyRunnable myRunnable = new MyRunnable();
Thread t1 = new Thread(myRunnable);
t1.start();
}
}
In the above code, we implemented the Runnable interface in our class MyRunnable and overrode the run() method. Then, in the main() function, we created an object of the Java Thread class and passed the instance of our class to its constructor. Finally, we called the start() method to begin the execution of the code defined in the run() method of our MyRunnable class.
3. Using an Anonymous Class
Since the Runnable interface is a functional interface (meaning it has only one abstract method), we can directly create an anonymous class and pass it to the Thread constructor instead of implementing the Runnable interface in a separate, well-defined class.
Here’s an example:
public class AnonymousClassExample {
public static void main(String[] args) {
Thread t1 = new Thread(new Runnable() {
@Override
public void run() {
System.out.println("Thread is running...");
}
});
t1.start();
}
}
In this example, instead of creating a separate class and implementing the Runnable interface, we directly created an anonymous class of type Runnable and implemented the run() method inside it. Then, we directly passed the object to the Thread constructor.
4. Using a Lambda Expression
The concept is the same as the previous method, but instead of using the new keyword to create an anonymous class, we can use a lambda expression to simplify the code.
Here’s an example:
public class LambdaExample {
public static void main(String[] args) {
Thread t1 = new Thread(() -> System.out.println("Thread is running..."));
t1.start();
}
}
Since Runnable is a functional interface, we can use a lambda expression to provide the implementation of the run() method concisely.
Conclusion
These are the four different ways to create a thread in Java. I hope you now have a clear understanding of the thread creation process! Happy coding!
Subscribe to my newsletter
Read articles from Jayita Saha directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
