Implementing password reset functionality in Spring
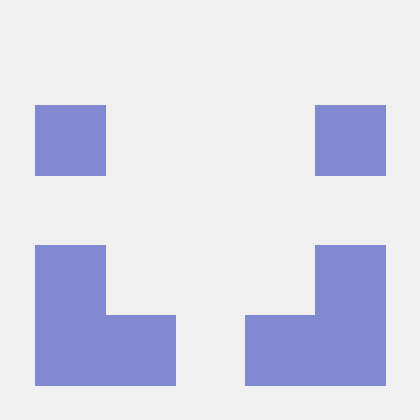
Hi. This is the twenty-sixth part of the diary about developing the “Programmers’ diary” blog. Previous part: https://hashnode.programmersdiary.com/implementing-change-password-functionality-in-programmersdiary-project. The open source code of this project is on: https://github.com/TheProgrammersDiary. The first diary entry explains why this project was done: https://medium.com/@vievaldas/developing-a-website-with-microservices-part-1-the-idea-fe6e0a7a96b5.
Next entry:
—————
2024-01-28
We have just implemented the password change functionality. Now let’s implement forgotten password reset.
Two good sources on how to implement it:
https://security.stackexchange.com/questions/117854/how-to-implement-forgot-password-functionality - we will need to generate a token and send it via email. However if we put the token in the link we could get a referrer leakage. So instead we will ask a user to copy and paste the token on the page once the link loads the reset password change.
https://stackoverflow.com/questions/1102781/best-way-for-a-forgot-password-implementation - short list on what + how to implement password reset.
We can add a new table, which can store a reset token and map it to user. Reset token must be encrypted, so if someone has breached our database the hacker still would not be able to reset users’ password. Also, the reset token should expire after a short time to protect the theft of the unencrypted token which is sent to user.
In blog microservice let’s do TDD:
@Test
void resetsPassword() throws IOException {
mother.loginNewUser("testUser", "email@gmail.com", "forgottenPassword");
passwordResetRepository.save(
new PasswordResetRepository.PasswordResetEntry(
new BCryptPasswordEncoder().encode("token"), "email@gmail.com"
)
);
controller.resetPassword(new PasswordReset("email@gmail.com", "token", "newPassword"));
controller.login(
new User("testUser", "email@gmail.com", "newPassword"),
new FakeHttpServletResponse()
);
Authentication authentication = SecurityContextHolder.getContext().getAuthentication();
assertNotNull(authentication);
assertTrue(authentication.isAuthenticated());
}
@ParameterizedTest
@NullSource
@ValueSource(strings = {"", "wrongToken"})
void failsToResetPasswordIfTokenIsInvalid(String wrongToken) throws IOException {
mother.loginNewUser("tester", "testeremail@gmail.com", "forgottenPassword");
passwordResetRepository.save(
new PasswordResetRepository.PasswordResetEntry(
new BCryptPasswordEncoder().encode("token"), "testeremail@gmail.com"
)
);
assertThrows(
RuntimeException.class,
() -> controller.resetPassword(
new PasswordReset("testeremail@gmail.com", wrongToken, "newPassword")
)
);
SecurityContextHolder.getContext().setAuthentication(null);
SecurityContextHolder.clearContext();
assertThrows(
BadCredentialsException.class,
() -> controller.login(
new User("tester", "testeremail@gmail.com", "newPassword"),
new FakeHttpServletResponse()
)
);
assertNull(SecurityContextHolder.getContext().getAuthentication());
}
@Test
void requestsPasswordResetOnLocallyRegisteredUser() {
mother.loginNewUser("localUser", "localuser@gmail.com", "notImportant");
controller.requestPasswordReset("localuser@gmail.com");
assertTrue(passwordResetRepository.existsByEmail("localuser@gmail.com"));
}
@Test
void failsToRequestPasswordResetOnOauthUser() throws IOException {
loginViaOauth2("oauthuser", "oauthuser@gmail.com");
controller.requestPasswordReset("oauthuser@gmail.com");
assertFalse(passwordResetRepository.existsByEmail("oauthuser@gmail.com"));
}
Here I check if user gets the password reset if he provided the correct token or password reset failed if the token was incorrect. Password reset is only allowed on locally registered user, since OAuth users do not have password in our database, therefore the password cannot be reset.
Let’s add the repository:
@Repository
public interface PasswordResetRepository extends CrudRepository<PasswordResetRepository.PasswordResetEntry, String> {
Optional<PasswordResetEntry> findFirstByEmailOrderByDateCreatedDesc(String email);
boolean existsByEmail(String email);
@Entity(name = "password_reset")
class PasswordResetEntry {
@Id
@Column(unique = true)
public String resetToken;
@Column(nullable = false)
public String email;
@CreationTimestamp
@Temporal(TemporalType.TIMESTAMP)
@Column(nullable = false)
public Date dateCreated;
public PasswordResetEntry(String resetToken, String email) {
this.resetToken = resetToken;
this.email = email;
this.dateCreated = new Date();
}
public PasswordResetEntry() {
}
}
}
There can be many non-unique email entries since the same user can ask password to be reset multiple times. However, we need to only recognize the last request as valid. Thats why we order the find query by date.
We need to add a new liquibase file:
CREATE TABLE password_reset (
reset_token VARCHAR(255) NOT NULL PRIMARY KEY,
email VARCHAR(255) NOT NULL,
date_created TIMESTAMP NOT NULL
);
The liquibase.changelog_master.yaml now looks like this:
databaseChangeLog:
- include:
file: classpath:/liquibase/postgresql/changelog/001_user.sql
- include:
file: classpath:/liquibase/postgresql/changelog/002_user.sql
- include:
file: classpath:/liquibase/postgresql/changelog/001_password_reset.sql
[Note from the future: liquibase is very nice then you are running a production database and can’t temporarily shut it down. With liquibase you execute ALTER TABLE or similar queries and the db structure changes. However, if you can shut down a database you can write a single database SQL query to create a table you need.]
With real repo done, let’s implement a fake repo:
public class FakePasswordResetRepository implements PasswordResetRepository {
private final Map<String, PasswordResetEntry> entries = new HashMap<>();
@Override
public Optional<PasswordResetEntry> findFirstByEmailOrderByDateCreatedDesc(String email) {
return entries.values()
.stream()
.filter(user -> user.email.equals(email))
.max(Comparator.comparing(user -> user.dateCreated));
}
@Override
public boolean existsByEmail(String email) {
return entries.values().stream().anyMatch(user -> user.email.equals(email));
}
@Override
public <S extends PasswordResetEntry> S save(S entry) {
entries.put(entry.email, entry);
return entry;
}
@Override
public <S extends PasswordResetEntry> Iterable<S> saveAll(Iterable<S> entities) {
throw new UnsupportedOperationException("Not implemented.");
}
@Override
public Optional<PasswordResetEntry> findById(String id) {
return Optional.ofNullable(entries.get(id));
}
@Override
public boolean existsById(String id) {
return entries.get(id) != null;
}
@Override
public Iterable<PasswordResetEntry> findAll() {
return entries.values();
}
@Override
public Iterable<PasswordResetEntry> findAllById(Iterable<String> strings) {
throw new UnsupportedOperationException("Not implemented.");
}
@Override
public long count() {
return entries.size();
}
@Override
public void deleteById(String entry) {
entries.values().remove(entry);
}
@Override
public void delete(PasswordResetEntry entry) {
throw new UnsupportedOperationException("Not implemented.");
}
@Override
public void deleteAllById(Iterable<? extends String> ids) {
throw new UnsupportedOperationException("Not implemented.");
}
@Override
public void deleteAll(Iterable<? extends PasswordResetEntry> entries) {
throw new UnsupportedOperationException("Not implemented.");
}
@Override
public void deleteAll() {
entries.clear();
}
}
This fake is similar to FakeUserRepository (https://github.com/TheProgrammersDiary/Blog/blob/main/blog/src/test/java/com/evalvis/blog/user/FakeUserRepository.java).
Now let’s implement a class to handle user password reset requests:
public class PasswordResetRequest {
private final String email;
public PasswordResetRequest(String email) {
this.email = email;
}
public void request(
PasswordResetRepository passwordResetRepository, UserRepository userRepository, Email emailSender,
PasswordEncoder encoder
) {
userRepository.findByEmail(email).ifPresent(user -> {
if(user.getPassword() == null) {
return;
}
String secureGuid = secureGuid().toString();
passwordResetRepository.save(new PasswordResetRepository.PasswordResetEntry(encoder.encode(secureGuid), email));
emailSender.sendEmail(
email,
"Password reset request",
"Hi. You or somebody else has requested a password reset on your account. If it was not you"
+ " no action is required. If it was you, please copy this token: " + secureGuid +
" and paste it in the same page you requested your password."
);
});
}
private static UUID secureGuid() {
try {
SecureRandom secureRandom = SecureRandom.getInstanceStrong();
return new UUID(secureRandom.nextLong(), secureRandom.nextLong());
} catch(NoSuchAlgorithmException e) {
throw new RuntimeException("Failed to generate secure GUID.");
}
}
}
We use secureGuid so it would be hard for the hacker to guess the token. Also, by returning if password is null we make OAuth user cannot reset the password we don’t own.
We need to send an email with the generated token so let’s implement we interface:
public interface Email {
void sendEmail(String toEmail, String subject, String body);
}
Let’s add a fake one which will do nothing (we don’t want to send emails when we test):
public class FakeSmtpEmail implements Email {
@Override
public void sendEmail(String toEmail, String subject, String body) {
}
}
For the real implementation, we need a dependency:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-mail</artifactId>
<version>3.2.2</version>
</dependency>
SMTP implementation:
@Service
public class SmtpEmail implements Email {
@Value("${spring.mail.username}")
private String sourceEmail;
@Autowired
private JavaMailSender emailSender;
@Override
public void sendEmail(String toEmail, String subject, String body) {
SimpleMailMessage message = new SimpleMailMessage();
message.setFrom(sourceEmail);
message.setTo(toEmail);
message.setSubject(subject);
message.setText(body);
emailSender.send(message);
}
}
Let’s add a password reset request to user controller:
@PostMapping("/request-password-reset")
void requestPasswordReset(@RequestBody String email) {
new PasswordResetRequest(email).request(passwordResetRepository, userRepository, emailSender, encoder);
}
With these changes from backend perspective user can request password reset. However, we lack actual resetting functionality. Let’s implement it:
public class PasswordReset {
private final String email;
private final String resetToken;
private final String newPassword;
public PasswordReset(String email, String resetToken, String newPassword) {
this.email = email;
this.resetToken = resetToken;
this.newPassword = newPassword;
}
public void reset(
PasswordResetRepository passwordResetRepository, PasswordEncoder encoder, UserRepository userRepository,
Email emailSender
) {
passwordResetRepository
.findFirstByEmailOrderByDateCreatedDesc(email)
.ifPresent(passwordChangeEntry -> {
if(
!encoder.matches(resetToken, passwordChangeEntry.resetToken)
|| new Date()
.after(new Date(passwordChangeEntry.dateCreated.getTime() + 60 * 60 * 1000)
)
) {
throw new UnauthorizedException("Reset token is incorrect or has already expired.");
}
emailSender.sendEmail(
email,
"Your password was reset",
"Hi. Your password was reset. If you did this, no action is required." +
"If someone else did this your account is breached, please contact us immediately."
);
userRepository.save(
UserRepository.UserEntry.withChangedPassword(
encoder.encode(newPassword), userRepository.findByEmail(email).get()
)
);
});
}
}
We only change user password if a valid token which has not yet expired (was created less than 1 hour ago) is provided.
Note that we also send an email notifying a user that the password has been reset if a hacker somehow got the right token.
For the Unauthorized exception we simply create a class:
public class UnauthorizedException extends RuntimeException {
public UnauthorizedException(String message) {
super(message);
}
}
and in ControllerException class we map it as client error:
@ExceptionHandler
public ResponseEntity<String> clientException(UnauthorizedException e) {
return clientError(e, HttpStatus.UNAUTHORIZED);
}
Now to allow password reset let’s add a PATCH request:
@PatchMapping("/reset-password")
ResponseEntity<String> resetPassword(@RequestBody PasswordReset passwordReset) {
passwordReset.reset(passwordResetRepository, encoder, userRepository, emailSender);
return ResponseEntity.ok().build();
}
Let’s also modify the permitted methods, adding our two new endpoints:
.requestMatchers(
"/users/signup", "/users/login",
"/actuator/prometheus",
"/comments/list-comments/{postId}", "/comments/create",
**"/users/request-password-reset", "/users/reset-password"**
)
.permitAll()
Let’s also update ITUserTestConfig:
@Bean
public PasswordResetRepository fakePasswordResetRepository() {
return new FakePasswordResetRepository();
}
@Bean
public Email fakeSmtpEmail() {
return new FakeSmtpEmail();
}
Since there are application integration tests we need to mock the repository and the email provider or Spring will fail (since we are using ITUserTestConfig class context).
We also need to update configuration properties file:
spring:
mail:
test-connection: true
host: smtp.gmail.com
port: 587
username: ${smtp_email}
password: ${smtp_password}
properties:
mail:
smtp:
auth: true
starttls:
enable: true
required: true
ssl:
trust: smtp.gmail.com
debug: false
And inject SMTP properties to docker-compose.yaml:
smtp_email: ${smtp_email}
smtp_password: ${smtp_password}
BE is finished let’s look at FE. In Login component let’s add a reset password link:
<Link
href="/request-password-reset"
className="font-medium text-blue-600 hover:underline"
>
Forgot password?
</Link>
on click let’s redirect to /request-password-reset/page.tsx:
"use client"
import "../../globals.css";
import { useForm } from "react-hook-form";
import { blogUrl } from "../../next.config.js";
import { useRouter } from "next/navigation";
export default function RequestPasswordReset() {
const { register, handleSubmit, reset } = useForm();
const router = useRouter();
return (
<div className="relative flex flex-col items-center justify-center min-h-screen overflow-hidden">
<div className="w-full p-6 bg-white rounded-md shadow-md lg:max-w-xl">
<h1 className="text-3xl font-bold text-center text-gray-700">Request password reset</h1>
<form className="mt-6" onSubmit={handleSubmit(onSubmit)}>
<div className="mb-4">
<label
htmlFor="email"
className="block text-sm font-semibold text-gray-800"
>
Email
</label>
<input
{...register("email", { required: true })}
name="email"
type="email"
className="block w-full px-4 py-2 mt-2 text-gray-700 bg-white border rounded-md focus:border-gray-400 focus:ring-gray-300 focus:outline-none focus:ring focus:ring-opacity-40"
/>
</div>
<div className="mt-2">
<button className="w-full px-4 py-2 tracking-wide text-white transition-colors duration-200 transform bg-gray-700 rounded-md hover:bg-gray-600 focus:outline-none focus:bg-gray-600">
Submit
</button>
</div>
</form>
</div>
</div>
);
async function onSubmit(data, event) {
event.preventDefault();
try {
const response = await fetch(
blogUrl + "/users/request-password-reset",
{
method: "POST",
body: data.email,
credentials: "omit"
}
);
reset();
router.push("/reset-password");
} catch (error) {
}
}
}
We simply ask for the email and send the BE a request to start password reset procedure. We don’t respond if email is not found to prevent user enumeration attacks.
On request sent the page redirects us to /reset-password/page.tsx:
"use client"
import "../../globals.css";
import { useForm } from "react-hook-form";
import { blogUrl } from "../../next.config.js";
import { useState } from "react";
export default function ResetPassword() {
const { register, setError, handleSubmit, reset, formState } = useForm();
const [responseMessage, setResponseMessage] = useState(<p></p>);
return (
<div className="relative flex flex-col items-center justify-center min-h-screen overflow-hidden">
<div className="w-full p-6 bg-white rounded-md shadow-md lg:max-w-xl">
<h1 className="text-3xl font-bold text-center text-gray-700">Reset password</h1>
<form className="mt-6" onSubmit={handleSubmit(onSubmit)}>
<div className="mb-4">
<label
htmlFor="email"
className="block text-sm font-semibold text-gray-800"
>
Email
</label>
<input
{...register("email", { required: true })}
name="email"
type="email"
className="block w-full px-4 py-2 mt-2 text-gray-700 bg-white border rounded-md focus:border-gray-400 focus:ring-gray-300 focus:outline-none focus:ring focus:ring-opacity-40"
/>
</div>
<div className="mb-4">
<label
htmlFor="resetToken"
className="block text-sm font-semibold text-gray-800"
>
Reset token (to confirm your identity)
</label>
<input
{...register("resetToken", { required: true })}
name="resetToken"
type="password"
className="block w-full px-4 py-2 mt-2 text-gray-700 bg-white border rounded-md focus:border-gray-400 focus:ring-gray-300 focus:outline-none focus:ring focus:ring-opacity-40"
/>
</div>
<div className="mb-4">
<label
htmlFor="newPassword"
className="block text-sm font-semibold text-gray-800"
>
New password
</label>
<input
{...register("newPassword", { required: true })}
name="newPassword"
type="password"
className="block w-full px-4 py-2 mt-2 text-gray-700 bg-white border rounded-md focus:border-gray-400 focus:ring-gray-300 focus:outline-none focus:ring focus:ring-opacity-40"
/>
</div>
<div className="mb-4">
<label
htmlFor="repeatedNewPassword"
className="block text-sm font-semibold text-gray-800"
>
Repeat new password
</label>
<input
{...register("repeatedNewPassword", { required: true })}
name="repeatedNewPassword"
type="password"
className="block w-full px-4 py-2 mt-2 text-gray-700 bg-white border rounded-md focus:border-gray-400 focus:ring-gray-300 focus:outline-none focus:ring focus:ring-opacity-40"
/>
</div>
<div className="mt-2">
<button className="w-full px-4 py-2 tracking-wide text-white transition-colors duration-200 transform bg-gray-700 rounded-md hover:bg-gray-600 focus:outline-none focus:bg-gray-600">
Submit
</button>
</div>
</form>
{formState.errors.repeatedNewPassword && (
<p className="text-red-500">{formState.errors.repeatedNewPassword.message}</p>
)
}
{responseMessage}
</div>
</div>
);
async function onSubmit(data, event) {
setResponseMessage(<p></p>);
event.preventDefault();
if (data.newPassword !== data.repeatedNewPassword) {
setError("repeatedNewPassword", {
type: "manual",
message: "New password and repeated new password do not match.",
});
return;
}
const body = { "email": data.email, "resetToken": data.resetToken, "newPassword": data.newPassword };
try {
const response = await fetch(
blogUrl + "/users/reset-password",
{
method: "PATCH",
body: JSON.stringify(body),
headers: { "Content-Type": "application/json" },
credentials: "omit"
}
);
if (response.status === 401) {
const text = await response.text();
throw new Error(text);
}
reset();
setResponseMessage(<p className="text-green-500">Password was reset successfully.</p>);
} catch (error) {
setResponseMessage(<p className="text-red-500">{error.message}</p>);
}
}
}
This asks us to enter an email, the reset token, a new password and repeat a new password. If passwords do not match or the reset token is incorrect an error will be shown. If everything is successful the green success line will be shown and user can now login with a new password.
As last thing for this day the authentication was changed to accept the email instead of username. I think email is more stable than username. Also, when logging in with Google users are given username equal to their name. If username would be already taken a clash would occur. Then we would need to ask for a custom username first time on sign up with Google.
This required big refactoring, so only pain points are shown:
UserDetails has getUsername method. I would like for it to have getEmail or a word which would fit both email and username. It does not have this method, so getUsername now returns email.
UserDetailsService has method loadUserByUsername(String username). This sucks since we want to load by email. To migitate this naming nonsense let’s at least name the parameter email and let’s search by email in our repository like this:
@Override
@Transactional
public UserDetails loadUserByUsername(String email) throws UsernameNotFoundException {
UserRepository.UserEntry user = userRepository
.findByEmail(email)
.orElseThrow(() -> new UsernameNotFoundException("User Not Found with email: " + email));
return new com.evalvis.security.User(user.getEmail(), user.getPassword());
}
By doing this and lots of more boring stuff, code was refactored and login by email was achieved.
Password reset functionality and a big refactoring is enough for today.
—————
Thanks for reading.
The project logged in this diary is open source, so if you would like to code or suggest changes, please visit https://github.com/TheProgrammersDiary.
Next part: https://hashnode.programmersdiary.com/programmersdiary-blog-a-short-day.
Subscribe to my newsletter
Read articles from Evaldas Visockas directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
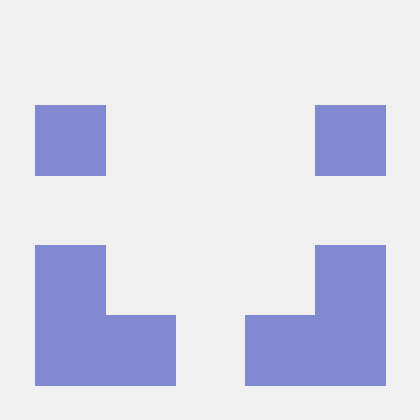