Roadmap To Master Spring Boot

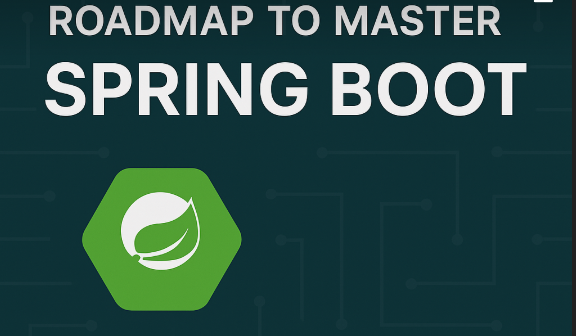
🛤️ Mastering Spring Boot: The Complete Roadmap for Modern Backend Development
Spring Boot has become the go-to framework for building robust, scalable, and production-ready Java applications. Whether you're just getting started or looking to level up your backend development game, this comprehensive roadmap is designed to guide you through every essential concept and advanced feature in the Spring Boot ecosystem.
In today’s software landscape, mastering Spring Boot is more than just knowing how to spin up REST APIs. It’s about understanding how to build secure, testable, production-ready, and cloud-native applications that can scale effortlessly. This blog breaks down the entire journey into carefully curated modules — starting from the fundamentals of Spring Boot MVC to advanced concepts like microservices architecture, Kafka integration, Docker, Kubernetes, and asynchronous task scheduling.
By following this roadmap:
✅ Beginners will gain a solid foundation in backend development using Spring Boot.
🚀 Intermediate developers will fill in the gaps and learn to write clean, maintainable, and secure code.
🏗️ Advanced developers will learn to architect scalable systems with cutting-edge tools like Kafka, Docker, and Kubernetes.
Whether you're aiming to land your first backend developer job, contribute to open-source Spring projects, or build enterprise-level applications, this guide will be your roadmap to becoming a Spring Boot Pro.
So let’s dive in — and take your Java backend skills to the next level!
🟢 1. Introduction to Spring Boot
What is Spring Boot?
Difference between Spring Framework and Spring Boot
Benefits of using Spring Boot
Spring Initializr and project structure
Dependency management with Maven/Gradle
Application properties and YAML
Spring Boot CLI basics
🌐 2. Spring Boot MVC
What is Spring MVC?
@Controller vs @RestController
DispatcherServlet and Request lifecycle
RequestMapping, GetMapping, PostMapping, etc.
Model, View, and Controller roles
Form handling and validation using
@Valid
View technologies (Thymeleaf, JSP basics)
🔗 3. RESTful APIs
Designing RESTful endpoints
CRUD operations with @RestController
Request and Response Entities
Path Variables vs Request Params
DTOs and Entity Conversion
Exception handling with
@ControllerAdvice
Versioning REST APIs
OpenAPI/Swagger integration
🗃️ 4. Hibernate and Spring Data JPA
What is ORM and Hibernate?
Entity, Repository, and Service layers
JpaRepository vs CrudRepository
Query methods and JPQL
Pagination and Sorting
Lazy vs Eager loading
Entity Relationships: OneToMany, ManyToOne, etc.
Native queries and projections
Transaction Management with @Transactional
🚀 5. Production-Ready Spring Boot Features
Spring Boot Actuator
Health checks, Metrics, and Monitoring
Custom Health Indicators
Externalized Configuration
Profiles and environment-specific configs
Logging strategies (Logback, Log4j2)
Admin server integration
🔐 6. Spring Security Fundamentals
Why Spring Security?
Authentication and Authorization basics
Security configuration class
In-memory authentication
Role-based access control
Password encoding
Securing REST APIs
Custom login/logout pages
🛡️ 7. Spring Security Advanced
JWT-based authentication
OAuth2 and OpenID Connect
Integrating with third-party identity providers (Google, GitHub)
CSRF protection and CORS
Method-level security with
@PreAuthorize
SecurityContext and filters
Stateless sessions
Refresh tokens
✅ 8. Spring Boot Testing
Unit testing with JUnit and Mockito
Testing REST APIs with
@WebMvcTest
Integration testing with
@SpringBootTest
TestContainers for DB testing
MockMVC and RestAssured
DataJpaTest for JPA layers
TestEntityManager
Profiles and config for tests
🔄 9. Aspect-Oriented Programming (AOP)
What is AOP?
Cross-cutting concerns
AOP Terminology (JoinPoint, Advice, Aspect)
Implementing AOP with
@Aspect
Logging, Monitoring, and Security with AOP
Pointcut expressions
⚡ 10. Caching and Concurrent Transaction Management
Spring Cache abstraction
@EnableCaching
and cache managersAnnotations:
@Cacheable
,@CachePut
,@CacheEvict
Integrating with Redis, EhCache, etc.
Understanding isolation levels
Optimistic vs Pessimistic locking
Handling concurrent updates
🧱 11. Introduction to Microservice Architecture
Monolith vs Microservices
Benefits and trade-offs of microservices
Core principles of microservices
Spring Cloud overview
Service discovery with Eureka
API Gateway with Spring Cloud Gateway
Configuration with Spring Cloud Config
🧠 12. Advanced Microservices Concepts
Circuit breakers with Resilience4j
Load balancing with Ribbon
Centralized logging (ELK stack)
Distributed tracing with Zipkin
Event-driven communication
Service orchestration vs choreography
Fault tolerance and retries
API versioning and Gateway routing
📬 13. Apache Kafka in Spring Boot
Introduction to Apache Kafka
Kafka architecture (brokers, topics, partitions)
Spring Kafka setup and configuration
Producer and Consumer APIs
KafkaTemplate usage
Consuming messages with
@KafkaListener
Message serialization/deserialization
Error handling and retries
🐳 14. Docker with Spring Boot
What is Docker and why use it?
Dockerfile for Spring Boot app
Multi-stage builds
Docker Compose for multi-container apps
Managing environment variables
Dockerizing a microservice
☸️ 15. Kubernetes Components
What is Kubernetes (K8s)?
Pods, Deployments, Services
ConfigMaps and Secrets
Health checks and probes
Horizontal Pod Autoscaling
Namespaces and resource limits
Volumes and persistent storage
🔧 16. Kubernetes Advanced
Helm charts and templating
CI/CD integration (GitHub Actions/Jenkins)
Kubernetes Dashboard
Observability: Prometheus + Grafana
Service Mesh with Istio
Rolling updates and rollbacks
Managing secrets and RBAC
🕰️ 17. Async and Blocking Task Scheduling in Spring Boot
@Async
and@EnableAsync
ThreadPool configuration
Handling future results and exceptions
@Scheduled
tasksCron expressions and fixed delays
Spring Batch for large data jobs
Non-blocking I/O with WebFlux (optional advanced)
🏁 Conclusion
As someone with over 3 years of hands-on experience in Spring Boot, I’ve had the opportunity to build scalable microservices, secure enterprise apps, and deploy real-world projects using the very tools and techniques discussed in this blog. This roadmap is not just theoretical—it's a culmination of everything I've learned and applied in production over the years.
This guide is meant to give you a structured learning path, but the real magic lies in consistent practice and deep dives into each topic. Don't hesitate to supplement this roadmap with:
🔍 Explore Alongside:
🎥 YouTube tutorials – For visual learners, channels like:
✍️ Other blogs & official documentation:
Dev.to Spring Boot Tag
📚 Recommended Study Resources:
Books:
Spring Boot in Action by Craig Walls
Cloud Native Java by Josh Long & Kenny Bastani
Spring Microservices in Action by John Carnell
Courses:
Spring & Hibernate for Beginners (Udemy - Chad Darby)
Spring Boot Microservices with Spring Cloud (Udemy - in28Minutes)
By following this roadmap and diving deeper using these resources, you'll be well on your way to becoming a Spring Boot expert—ready to tackle any challenge, build scalable systems, and contribute meaningfully to modern backend projects.
Keep building. Keep exploring. And remember—every pro was once where you are now.
Happy Coding! 💚🚀
Subscribe to my newsletter
Read articles from Abhilash Kurapati directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Abhilash Kurapati
Abhilash Kurapati
Master's Student At Saint Louis University majoring in Computer Science, Currently taking part in the development of some Backed projects and some really cool Generative AI Tools and agents. Hoping to put my learning into something highly productive in the near future.