F#: The Functional Powerhouse for Modern Developers

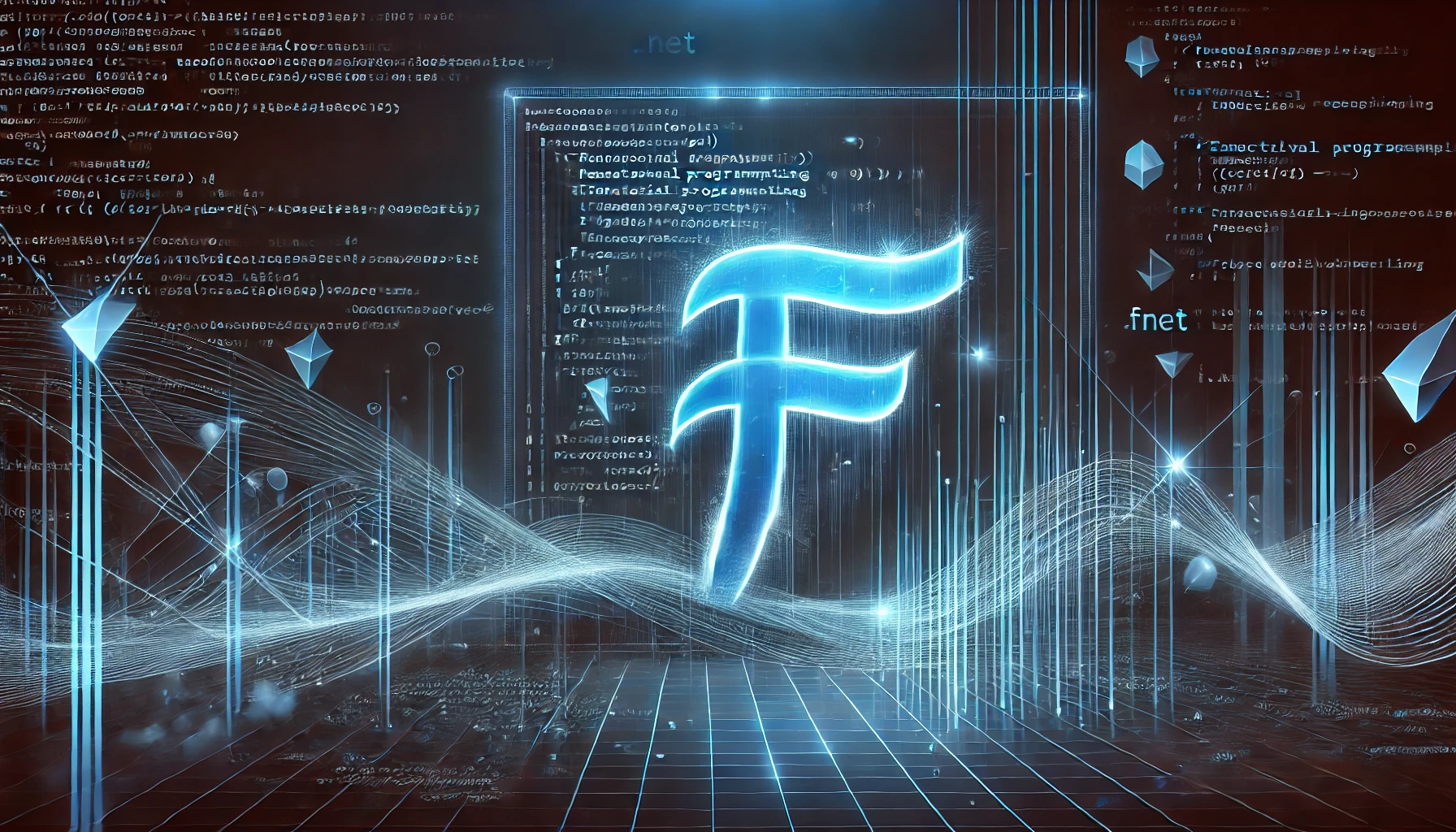
Introduction
F# is an open-source, cross-platform programming language that seamlessly blends functional and general-purpose programming. Initially developed by Microsoft, it has gained popularity among developers for its simplicity, type safety, and strong interoperability with .NET languages like C# and VB.NET. With a concise syntax and a strong focus on immutability, F# offers a fresh approach to problem-solving, making it an excellent choice for modern software development.
Why Choose F#?
1. Functional First Approach
Unlike traditional imperative languages, F# prioritizes functional programming, allowing developers to write concise and expressive code. Features like pattern matching, higher-order functions, and immutability enhance code reliability and maintainability.
2. Interoperability with .NET Ecosystem
F# runs on the .NET runtime, meaning developers can leverage powerful .NET libraries, frameworks, and tools. Whether integrating with C#, accessing Azure services, or utilizing .NET Core, F# ensures a seamless experience.
3. Concise and Readable Syntax
F# eliminates boilerplate code and emphasizes expressiveness. Compared to C# and Java, it reduces verbosity, making development faster and debugging easier. This simplicity is often compared to Python, making F# an attractive option for developers transitioning from scripting languages.
4. Strong Type Inference and Safety
F# features an advanced type inference system that reduces the need for explicit type declarations while maintaining strict type safety. This minimizes runtime errors and enhances the stability of applications.
5. Asynchronous and Parallel Programming
With built-in support for asynchronous programming and parallel execution, F# simplifies handling concurrent operations. Its lightweight asynchronous workflows make it an excellent choice for scalable web applications and cloud computing.
Real-World Applications of F
Data Science and Machine Learning: With libraries like FSharp.Data and ML.NET, F# is used for data analytics, statistical modeling, and AI-driven applications.
Finance and Quantitative Analysis: Many financial institutions use F# for risk modeling, algorithmic trading, and predictive analytics.
Web Development: Frameworks like Giraffe enable developers to build robust web applications using F#.
Game Development: With Unity and .NET compatibility, F# is increasingly being adopted in game development for scripting and AI logic.
Implementing F# in My Recent Project
The Challenge
As a developer, I was working on a web-based financial analytics platform that required high-speed computations and seamless data processing. Initially, the backend was built using C# and SQL, but as the project scaled, maintaining the code became cumbersome. The system needed a more efficient way to handle computations while keeping the codebase clean and easy to manage.
Why I Chose F
After researching various functional programming languages, I decided to integrate F# due to its interoperability with .NET, concise syntax, and strong type inference. Since F# runs on the .NET framework, I knew it would blend well with the existing C# components of the project.
Implementation Process
Refactoring Core Logic
The first step was to rewrite the core business logic using F# functions. Instead of relying on loops and mutable states, I leveraged F#'s higher-order functions and recursion.
Example: Replacing traditional loops with map and filter functions to process financial data.
Seamless Integration with .NET
One major advantage was that I could still use existing C# libraries while migrating key components to F#.
Used F# for handling API requests while keeping authentication and database interactions in C#.
Optimizing Data Processing with F# Pipelines
Financial data processing involves multiple transformations, and F#'s pipeline operator (
|>
) helped create cleaner and more readable transformations.Example:
let processData data = data |> filterValidEntries |> transformData |> analyzeTrends
Utilizing F#'s Async Workflows for Performance Boost
The platform needed to handle thousands of concurrent requests, so I implemented F#'s asynchronous workflows to improve performance.
Compared to traditional thread-based approaches, F#'s async features allowed non-blocking computations without excessive resource usage.
Results & Benefits Observed
Code Readability and Maintainability Improved
The functional approach eliminated redundant code and made debugging easier.
Code review sessions became faster since function purity reduced unexpected side effects.
Performance Boost
- The new F# modules handled data transformations 30% faster than the original C# implementation.
Better Developer Experience
Writing concise and expressive code reduced development time.
Debugging became easier with F#'s strong type inference.
Lessons Learned
Adopting a Functional Mindset Takes Time
- Initially, thinking in terms of immutability and pure functions was challenging, but the benefits became apparent as I wrote more code.
Interoperability with C# is a Strength
- F# didnโt require a complete rewrite; instead, it complemented the existing C# codebase.
Functional Programming is a Game Changer for Data Processing
- Using F# for computations resulted in cleaner and more efficient data handling.
Getting Started with F
Installation
To start coding in F#, install the .NET SDK and run:
dotnet new console -lang F# -o MyFSharpApp
Sample Code
Hereโs a simple F# program demonstrating functional programming:
let square x = x * x
let result = square 5
printfn "The square of 5 is %d" result
Conclusion
F# is a powerful language that combines the best of functional and object-oriented paradigms. With its concise syntax, strong type safety, and robust .NET interoperability, F# is a great choice for modern developers looking to build scalable, high-performance applications. As the software industry continues to embrace functional programming, learning F# can be a strategic career move for developers aiming to stay ahead of the curve.
By implementing F# in my recent project, I was able to enhance performance, simplify data processing, and reduce complexity in my codebase. If you're looking for a programming language that offers simplicity without sacrificing power, F# is definitely worth exploring.
Subscribe to my newsletter
Read articles from Binshad directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Binshad
Binshad
๐ป Exploring the intersection of technology and finance. ๐ Sharing insights on tech dev, Ai,market trends, and innovation. ๐ก Simplifying the complex world of investing