Learning the Basics of Bash Scripting

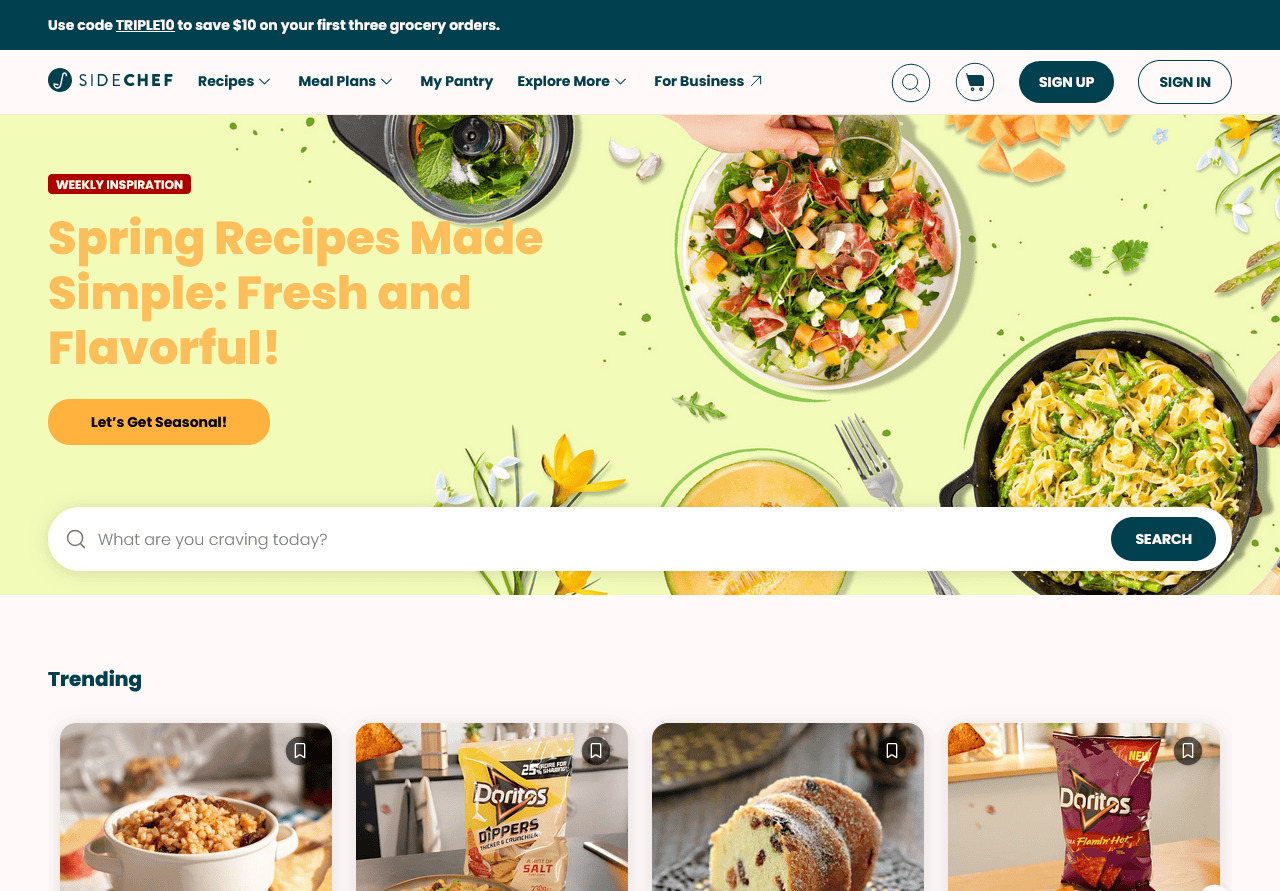
Learning the Basics of Bash Scripting: A Beginner's Guide
Bash scripting is a powerful skill for automating tasks, managing systems, and improving productivity. Whether you're a developer, sysadmin, or just a tech enthusiast, mastering Bash can save you time and effort. In this guide, we'll cover the fundamentals of Bash scripting, including variables, loops, conditionals, and functions.
If you're looking to monetize your programming skills, check out MillionFormula, a free platform where you can make money online without needing credit or debit cards.
What is Bash Scripting?
Bash (Bourne Again SHell) is a Unix shell and command language used to interact with the operating system. A Bash script is a file containing a series of commands that are executed sequentially.
Why Learn Bash Scripting?
Automation โ Run repetitive tasks automatically.
System Administration โ Manage servers and workflows efficiently.
Developer Productivity โ Speed up development tasks like file processing.
Getting Started with Bash Scripting
1. Writing Your First Bash Script
Create a file with a .sh
extension (e.g., hello.sh
) and add: bash Copy
#!/bin/bash
echo "Hello, World!"
#!/bin/bash
(shebang) tells the system to use Bash to execute the script.echo
prints text to the terminal.
Make the script executable and run it: bash Copy
chmod +x hello.sh
./hello.sh
2. Variables in Bash
Variables store data for later use. bash Copy
#!/bin/bash
name="John"
echo "Hello, $name!"
Variables are assigned without spaces (
name="John"
).Access them using
$variable_name
.
3. User Input
Use read
to get input from the user: bash Copy
#!/bin/bash
echo "What's your name?"
read name
echo "Hello, $name!"
Control Structures in Bash
1. Conditional Statements (if-else)
Check conditions using if
, elif
, and else
: bash Copy
#!/bin/bash
echo "Enter a number:"
read num
if [ $num -gt 10 ]; then
echo "$num is greater than 10."
elif [ $num -eq 10 ]; then
echo "$num is equal to 10."
else
echo "$num is less than 10."
fi
-gt
(greater than),-eq
(equal),-lt
(less than).Always leave spaces inside
[ ]
.
2. Loops (for, while)
For Loop: bash Copy
#!/bin/bash
for i in {1..5}; do
echo "Number: $i"
done
While Loop: bash Copy
#!/bin/bash
count=1
while [ $count -le 5 ]; do
echo "Count: $count"
((count++))
done
Functions in Bash
Functions help organize reusable code: bash Copy
#!/bin/bash
greet() {
echo "Hello, $1!"
}
greet "Alice"
greet "Bob"
$1
refers to the first argument passed.
Working with Files and Directories
Bash can manipulate files efficiently: bash Copy
#!/bin/bash
# Create a directory
mkdir test_folder
# Create a file
touch test_folder/file.txt
# Write to a file
echo "This is a test." > test_folder/file.txt
# Read a file
cat test_folder/file.txt
Advanced Bash Features
1. Command Substitution
Store command output in a variable: bash Copy
#!/bin/bash
current_date=$(date)
echo "Today is $current_date"
2. Exit Codes
Check if a command succeeded: bash Copy
#!/bin/bash
ls /nonexistent_folder
if [ $? -ne 0 ]; then
echo "Command failed."
fi
$?
holds the exit status of the last command (0
means success).
3. Debugging Bash Scripts
Use -x
to debug: bash Copy
bash -x script.sh
Where to Go from Here?
Now that you know the basics, explore:
If you want to earn money with your programming skills, MillionFormula is a great free platform to start.
Conclusion
Bash scripting is essential for automating tasks and improving efficiency. Start with simple scripts, experiment with loops and conditionals, and gradually move to advanced topics.
Happy scripting! ๐
Subscribe to my newsletter
Read articles from MillionFormula directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
