reCAPTCHA in Rails: How to Stop Bots and Enhance Security

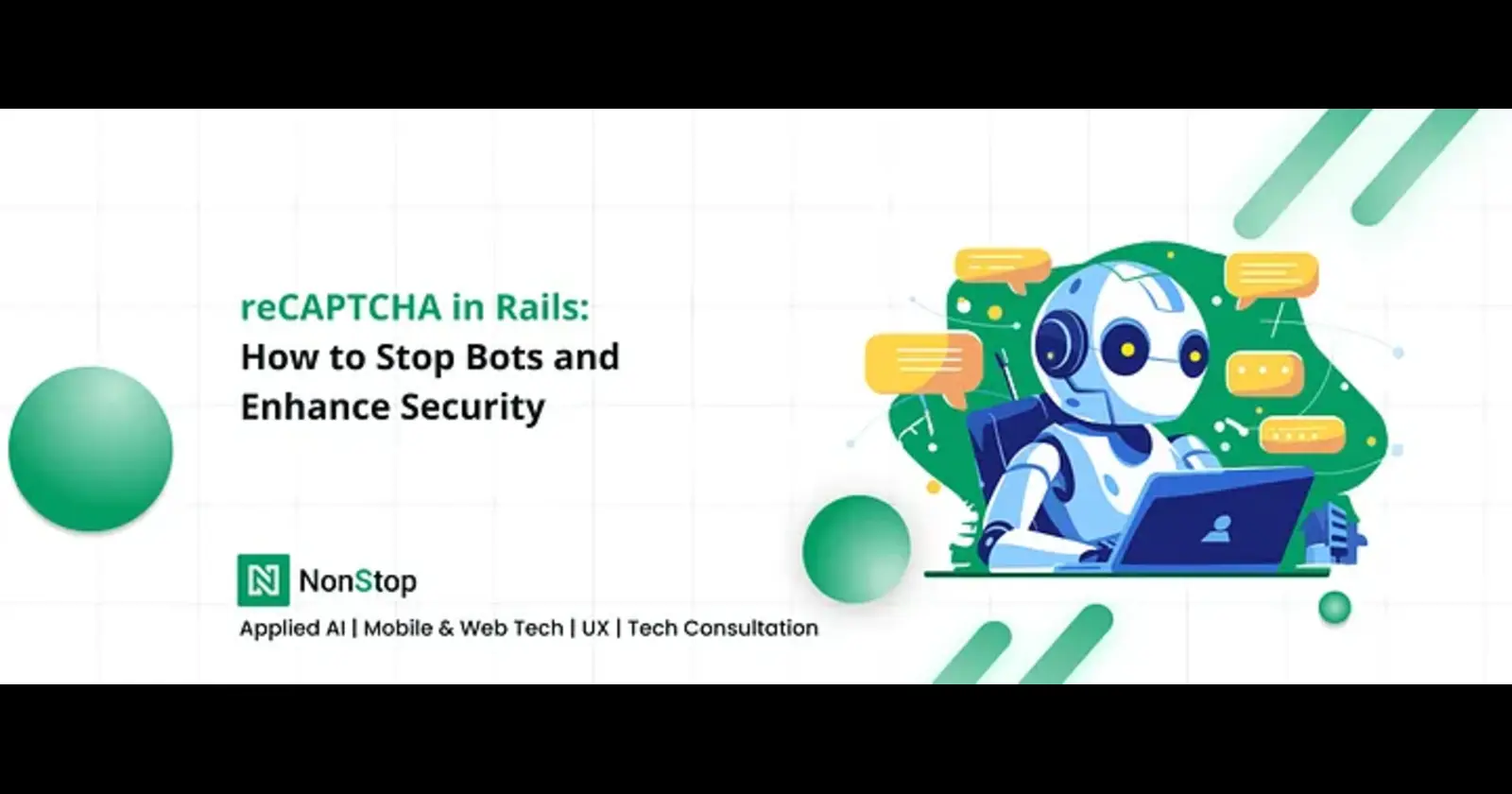
In this blog, I will cover how to implement reCAPTCHA functionality in your Rails application, explore the best options to choose from, and discuss how to manage the analytics and security of your application effectively.
So, what exactly is reCAPTCHA? Let’s explore its functionality, advantages, and how to implement it.
What is reCAPTCHA?
reCAPTCHA is a CAPTCHA system owned by Google. It enables web hosts to distinguish between human and bots trying to access the websites.It does this by prompting a login test that only human users can pass and not bots.
How does reCAPTCHA work?
reCAPTCHA provides a challenge-response test that is easy for humans to pass but difficult for bots to solve.
The user is presented with a test, such as:
- A simple checkbox
- Selecting the appropriate images from an image grid.
- An audio challenge
- A distorted image challenge.
When a user attempts the test, validation, and behavior analysis take place in the background. Based on the analysis, a report is generated. If the user’s test score matches the report, they are allowed to proceed; otherwise, a validation error is displayed, and the user is temporarily blocked.
Types of reCAPTCHA
Choosing the type of reCAPTCHA There are four types of reCAPTCHA to choose from when creating a new site.
reCAPTCHA v3
reCAPTCHA v3 allows you to verify if an interaction is legitimate without any user interaction. It is a pure JavaScript API returning a score, giving you the ability to take action in the context of your site: for instance requiring additional factors of authentication, sending a post to moderation, or throttling bots that may be scraping content.
reCAPTCHA v2 (“I’m not a robot” Checkbox)
The “I’m not a robot” checkbox requires the user to check a box to confirm they are not a robot. This may either immediately pass the user (with No CAPTCHA) or prompt them with a challenge to verify their humanity. This is the simplest option to integrate and only requires two lines of HTML to render the checkbox.
Integrating reCAPTCHA into a Rails Application
1. Install gem
To Implement reCAPTCHA in your application, you can use a gem that works directly with Google reCAPTCHA!
Add gem 'recaptcha'
to Gemfile
, and don’t forget to runbundle install
to install the gem.
reptcha gem
gem'recaptcha'
2. Obtain reCAPTCHA API Keys from the Admin console
Go to the Google reCAPTCHA Admin Console, Register your site, and select reCAPTCHA (‘I’m not a robot’ checkbox). Add your site name (e.g., app.localhost
in my case). Copy the site key and secret key, as you will need them for configuring your Rails application.
3. Configure reCAPTCHA Keys in Rails
Now that you have the site_key
and secret_key
generated from the Google console, it's time to add them to your application. You can create a .env
file at the root of your project and include the reCAPTCHA keys as follows:
# .env
RECAPTCHA_SITE_KEY=ABDXYZ_yyyyyyyyyyyyyyyyy
RECAPTCHA_SECRET_KEY=ABDXYZ_xxxxxxxxxxxxxxxxx
Alternative API key setup
# config/initializers/recaptcha.rb
Recaptcha.configure do |config|
config.site_key = '6Lc6BAAAAAAAAChqRbQZcn_yyyyyyyyyyyyyyyyy'
config.secret_key = '6Lc6BAAAAAAAAKN3DRm6VA_xxxxxxxxxxxxxxxxx'
# Uncomment the following line if you are using a proxy server:
# config.proxy = 'http://myproxy.com.au:8080'
# Uncomment the following lines if you are using the Enterprise API:
# config.enterprise = true
# config.enterprise_api_key = 'AIzvFyE3TU-g4K_Kozr9F1smEzZSGBVOfLKyupA'
# config.enterprise_project_id = 'my-project'
end
4. reCAPTCHA UI 🤖
To display the reCAPTCHA checkbox, you only need to add a single line of code to your .erb
file. Once added, you'll see the reCAPTCHA appear on your UI, complete with the classic 'I'm not a robot' checkbox, bringing an extra layer of security to your application!
<%= recaptcha_tags %>
5. How to validate the reCAPTCHA?
In the controller, you can use the verify_recaptcha
method provided by the gem to further validate the user.
# app/controllers/users_controller.rb
def create
@user = User.new(params[:user].permit(:name))
recaptcha_valid = verify_recaptcha(model: @user, action: 'registration')
if recaptcha_valid
if @user.save
redirect_to @user
else
render 'new'
end
else
# Score is below threshold, so user may be a bot. Show a challenge, require multi-factor
# authentication, or do something else.
render 'new'
end
end
6. I18 Support
reCAPTCHA supports the I18n gem (it comes with English translations) To override or add new languages, add to config/locales/*.yml
# config/locales/en.yml
en:
recaptcha:
errors:
verification_failed: 'reCAPTCHA was incorrect, please try again.'
recaptcha_unreachable: 'reCAPTCHA verification server error, please try again.'
7. Successfully verifying the reCAPTCHA
In the Admin Console, you can view analytics, including graphs showing the number of reCAPTCHA passes and failures.
8. Some Pitfalls
While implementing reCAPTCHA, you may encounter an issue where the reCAPTCHA is not visible. This happened to me as well and was due to the following error. However, there’s no need to panic, this error is expected and can be resolved!
Refused to load the script 'https://www.recaptcha.net/recaptcha/api.js'
because it violates the following Content Security Policy directive:
"script-src 'unsafe-eval' 'unsafe-inline' admin.localhost media.localhost
https://maps.googleapis.com https://checkout.stripe.com https://js.stripe.com
https://stripecdn.com https://snb.ifg.iata.org https://stats.pusher.com".
Note that 'script-src-elem' was not explicitly set, so 'script-src' is used as
a fallback.
This error occurs due to a Content Security Policy (CSP) restriction, which is a security feature that helps prevent a variety of attacks, such as Cross-Site Scripting (XSS) and data injection attacks, by specifying which resources (like scripts, stylesheets, and images) are allowed to be loaded and executed on your web page.
How to Fix This:
To resolve this issue, you need to update your Content Security Policy to allow the reCAPTCHA script to be loaded. Specifically, you should add https://www.recaptcha.net
to your script-src
directive.
Solution:
You can modify your CSP header to include https://www.recaptcha.net
. Here’s an example of how you can update the policy:
plaintextContent-Security-Policy:
script-src 'unsafe-eval' 'unsafe-inline' admin.localhost media.localhost
https://maps.googleapis.com https://checkout.stripe.com https://js.stripe.com
https://stripecdn.com https://snb.ifg.iata.org https://stats.pusher.com
https://www.recaptcha.net;
By adding https://www.recaptcha.net
to the script-src
directive, the browser will now allow the reCAPTCHA script to be loaded and executed.
9. Accessibility
reCAPTCHA works with major screen readers such as ChromeVox (Chrome OS), JAWS (IE/Edge/Chrome on Windows), NVDA (IE/Edge/Chrome on Windows), and VoiceOver (Safari/Chrome on Mac OS). reCAPTCHA will alert screen readers of status changes, such as when the reCAPTCHA verification challenge is complete. The status can also be found by looking for the heading titled “recaptcha status” in the “recaptcha widget” section of the page. See reCAPTCHA ARIA Status Messages for more information.
People with visual disabilities can opt for an audio test instead of the image verification in reCAPTCHA.
Subscribe to my newsletter
Read articles from NonStop io Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

NonStop io Technologies
NonStop io Technologies
Product Development as an Expertise Since 2015 Founded in August 2015, we are a USA-based Bespoke Engineering Studio providing Product Development as an Expertise. With 80+ satisfied clients worldwide, we serve startups and enterprises across San Francisco, Seattle, New York, London, Pune, Bangalore, Tokyo and other prominent technology hubs.