MutationObserver vs Event Listeners: The Best Way to Watch DOM Changes
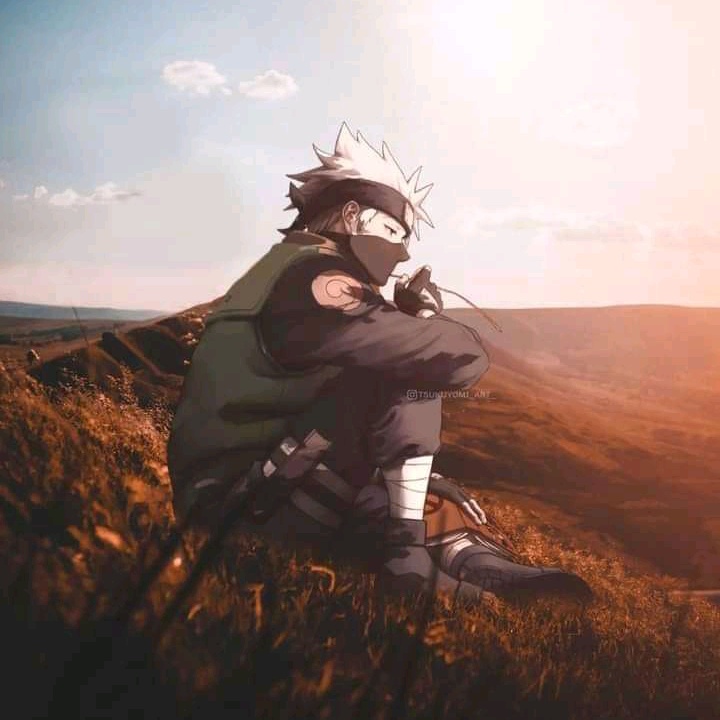
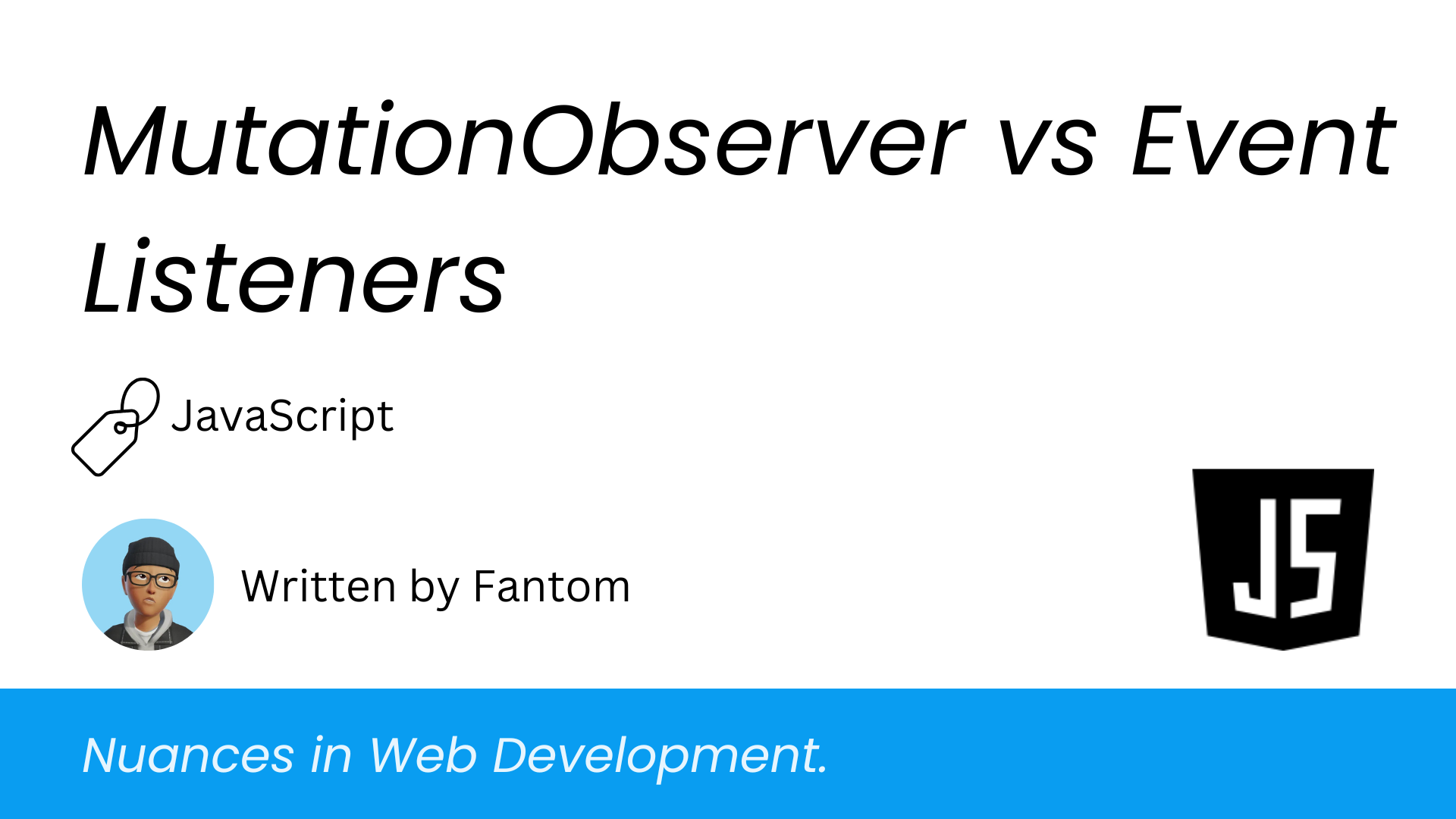
In modern web development, detecting changes in the DOM (Document Object Model) is a common requirement. Whether you're updating UI elements dynamically, handling user interactions, or syncing content, choosing the right method to observe DOM changes is crucial.
Two primary approaches are MutationObserver and Event Listeners. While both can detect changes, they work differently and serve distinct purposes. In this article, we'll explore their differences, use cases, and when you should use one over the other.
1. Event Listeners: Handling User Interactions and Manual Changes
Event Listeners are the traditional way to detect user interactions or specific events in the DOM, such as clicks, input changes, or attribute modifications.
element.addEventListener(event, callback);
event
: The event type (e.g.,"click"
,"input"
,"change"
).callback
: The function to execute when the event occurs.
Examples
Detecting Input Changes
const input = document.querySelector("#myInput");
input.addEventListener("input", (event) => {
console.log("Input changed to:", event.target.value);
});
Detecting Click Events on a Button
const button = document.querySelector("#myButton");
button.addEventListener("click", () => {
console.log("Button clicked!");
});
Key Takeaways
Works well for user-driven actions like clicks, key presses, or input changes.
Cannot detect structural changes in the DOM (e.g., new elements added dynamically).
Requires manually attaching listeners to elements.
2. MutationObserver: Tracking Structural Changes in the DOM
The MutationObserver API provides a more advanced way to detect changes in the DOM, such as added or removed elements, attribute modifications, or text updates. Unlike event listeners, MutationObserver works asynchronously and detects changes without requiring direct event triggers.
const observer = new MutationObserver(callback);
observer.observe(targetNode, options);
callback
: A function executed when mutations are detected.targetNode
: The element to observe.options
: Specifies the types of changes to watch for.
Examples
Observing Changes in a Parent Element
const targetNode = document.querySelector("#container");
const observer = new MutationObserver((mutations) => {
mutations.forEach((mutation) => {
console.log("DOM changed:", mutation);
});
});
observer.observe(targetNode, { childList: true, subtree: true });
#container
.Tracking Attribute Changes on an Element
const element = document.querySelector("#myElement");
const observer = new MutationObserver((mutations) => {
mutations.forEach((mutation) => {
console.log(`Attribute ${mutation.attributeName} changed`);
});
});
observer.observe(element, { attributes: true });
#myElement
.Key Takeaways
Can detect changes in structure, attributes, and text content.
Works asynchronously, reducing performance overhead.
Suitable for dynamic applications where elements are added or modified frequently.
3. Key Differences at a Glance
Feature | Event Listeners | MutationObserver |
Detects user actions? | Yes | No |
Detects added/removed elements? | No | Yes |
Detects attribute changes? | No | Yes |
Detects input changes? | Yes ("input" event) | No |
Works asynchronously? | No | Yes |
Requires manual attachment? | Yes | No (works on parent elements) |
4. Usecases for Each
Scenario | Best Choice |
Detecting clicks, keypresses, form input changes | Event Listener |
Monitoring dynamically added elements | MutationObserver |
Watching for attribute changes (e.g., style , class ) | MutationObserver |
Handling user interactions like hovering, scrolling | Event Listener |
Keeping track of structural changes in a dynamic UI | MutationObserver |
Conclusion
While both MutationObserver and Event Listeners allow you to track changes in the DOM, they serve different purposes. Event Listeners are best for handling user interactions, whereas MutationObserver is the go-to choice for monitoring structural and attribute changes in the DOM.
Choosing the right approach depends on whether your focus is on user-driven events or automatic DOM updates.
This is the 2nd article in the Nuances in Web Development series, where we uncover subtle but important differences in web technologies. Subscribe to the newsletter to ensure you don't miss the next article in the series. Don't forget to share and leave a like ❤️.
Subscribe to my newsletter
Read articles from Peter Bamigboye directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
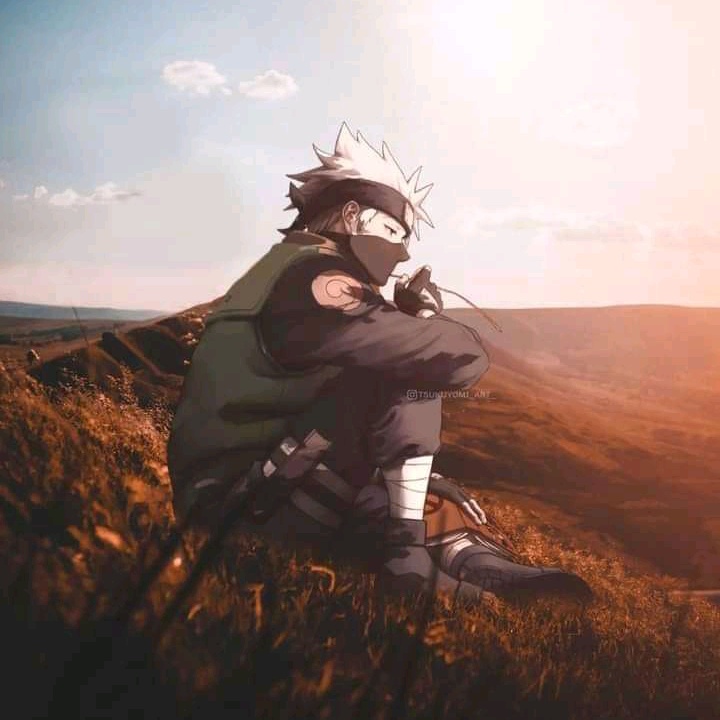
Peter Bamigboye
Peter Bamigboye
I am Peter, a front-end web developer who writes on current technologies that I'm learning or technologies that I feel the need to simplify.