Building a Decentralized Lending Protocol on CrossFi (Beginner-Friendly Guide)

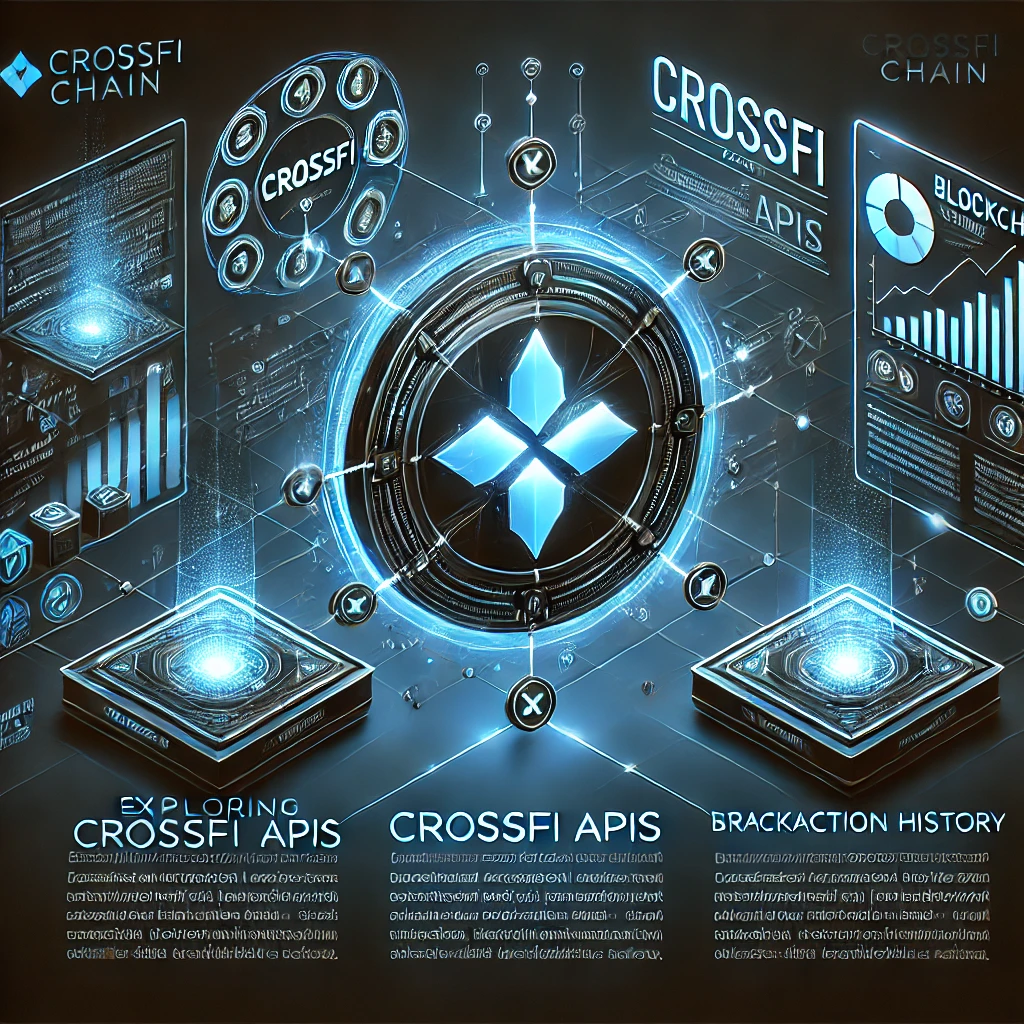
Introduction
DeFi (Decentralized Finance) allows anyone to lend and borrow assets without relying on banks. In this guide, we’ll build a simple lending protocol on CrossFi that lets users:
Deposit funds to earn interest
Borrow funds by locking collateral
Get liquidated if their collateral becomes too low
We’ll go through every step, from creating files and folders to writing smart contracts and deploying them.
1. Setting Up the Development Environment
Before writing any code, let’s set up everything on our computer.
A. Install Node.js and npm
Make sure you have Node.js installed. If not, download it from nodejs.org.
B. Install Hardhat
Hardhat is a development environment for writing and testing smart contracts.
Run this command in your terminal:
npm install -g hardhat
C. Create a New Project Folder
Open a terminal and run:
mkdir crossfi-lending
cd crossfi-lending
npm init -y
This creates a new folder called crossfi-lending
and initializes it as a Node.js project.
2. Setting Up Hardhat for Smart Contract Development
A. Initialize Hardhat
Inside your crossfi-lending
folder, run:
npx hardhat
It will ask you to choose a project type. Select:
Create a basic sample project
Type yes
when it asks to install dependencies
B. Project Folder Structure
After running Hardhat, your folder will look like this:
crossfi-lending/
│── contracts/ # Where smart contracts go
│ └── LendingPool.sol # Our lending contract
│── scripts/ # Deployment scripts
│ └── deploy.js # Script to deploy contracts
│── test/ # Automated tests (optional)
│── hardhat.config.js # Hardhat configuration file
│── package.json # Dependencies
3. Writing the Smart Contracts
A. Creating the LendingPool Contract
This contract allows users to deposit funds, borrow funds, and repay loans.
Create a new file: contracts/LendingPool.sol
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
contract LendingPool {
mapping(address => uint256) public deposits;
mapping(address => uint256) public borrowBalances;
uint256 public totalLiquidity;
function deposit() external payable {
deposits[msg.sender] += msg.value;
totalLiquidity += msg.value;
}
function borrow(uint256 amount) external {
require(amount <= totalLiquidity, "Not enough liquidity");
borrowBalances[msg.sender] += amount;
totalLiquidity -= amount;
payable(msg.sender).transfer(amount);
}
function repay() external payable {
borrowBalances[msg.sender] -= msg.value;
totalLiquidity += msg.value;
}
}
Users deposit into the pool
Borrowers borrow up to the available liquidity
They must repay loans with interest
B. Creating the Collateral Manager Contract
Borrowers must lock collateral before borrowing funds.
Create a new file: contracts/CollateralManager.sol
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
contract CollateralManager {
mapping(address => uint256) public collateral;
function depositCollateral() external payable {
collateral[msg.sender] += msg.value;
}
function withdrawCollateral(uint256 amount) external {
require(collateral[msg.sender] >= amount, "Not enough collateral");
collateral[msg.sender] -= amount;
payable(msg.sender).transfer(amount);
}
}
Users lock assets as collateral before borrowing
They can withdraw collateral if they don’t have an active loan
4. Deploying the Smart Contracts
A. Configure Hardhat to Use CrossFi
By default, Hardhat uses Ethereum for testing. To deploy on CrossFi, we need to configure it.
Open hardhat.config.js
and update it:
require("@nomicfoundation/hardhat-toolbox");
require("dotenv").config();
module.exports = {
solidity: "0.8.0",
networks: {
crossfi: {
url: "https://crossfi-testnet.g.alchemy.com/v2/wAz9j4RJUgEBiaMljD1yGbi45YBRKXTK",
accounts: [process.env.PRIVATE_KEY] // Your wallet private key
}
}
};
Store your private key in a .env
file (for security)
B. Install Dependencies
Run the following command:
npm install dotenv @nomicfoundation/hardhat-toolbox ethers
dotenv
: Loads environment variablesethers
: Helps interact with the blockchain
Create a .env
file in your project root and add:
PRIVATE_KEY=your_wallet_private_key
Replace your_wallet_private_key
with your actual private key (never share this).
C. Writing the Deployment Script
Create a new file: scripts/deploy.js
const hre = require("hardhat");
async function main() {
const LendingPool = await hre.ethers.getContractFactory("LendingPool");
const lendingPool = await LendingPool.deploy();
await lendingPool.deployed();
console.log(`LendingPool deployed at ${lendingPool.address}`);
const CollateralManager = await hre.ethers.getContractFactory("CollateralManager");
const collateralManager = await CollateralManager.deploy();
await collateralManager.deployed();
console.log(`CollateralManager deployed at ${collateralManager.address}`);
}
main();
This script deploys both contracts. It also will print the contract addresses after deployment
D. Deploying the Contracts on CrossFi
Run the following command to deploy:
npx hardhat run scripts/deploy.js --network crossfi
--network crossfi
tells Hardhat to deploy to CrossFi
5. Interacting with the Contracts
A. Using a Frontend (React + Web3.js)
We can use Ethers.js to interact with the contract from a website.
Example JavaScript Code to Deposit Funds
import { ethers } from "ethers";
const provider = new ethers.providers.Web3Provider(window.ethereum);
const signer = provider.getSigner();
const lendingPool = new ethers.Contract(
"0xYourLendingPoolAddress",
lendingPoolABI,
signer
);
async function depositFunds(amount) {
const tx = await lendingPool.deposit({ value: ethers.utils.parseEther(amount) });
await tx.wait();
console.log("Deposit successful!");
}
Connects the website to MetaMask
Allows users to deposit funds
6. Final Thoughts: How It All Works
Lenders deposit into the lending pool.
Borrowers lock collateral before borrowing funds.
If collateral is too low, the system liquidates the borrower.
Smart contracts manage everything securely.
Users interact via a frontend (React + Web3.js).
In this CrossFi lending system, everything runs seamlessly through smart contracts that ensure security and automation. Lenders deposit into a lending pool, allowing borrowers to take loans by locking up collateral. If the collateral value drops too low, the system automatically triggers liquidation to protect lenders.
Subscribe to my newsletter
Read articles from Iniubong directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
