Why Clean Code Matters: Tips for Developers

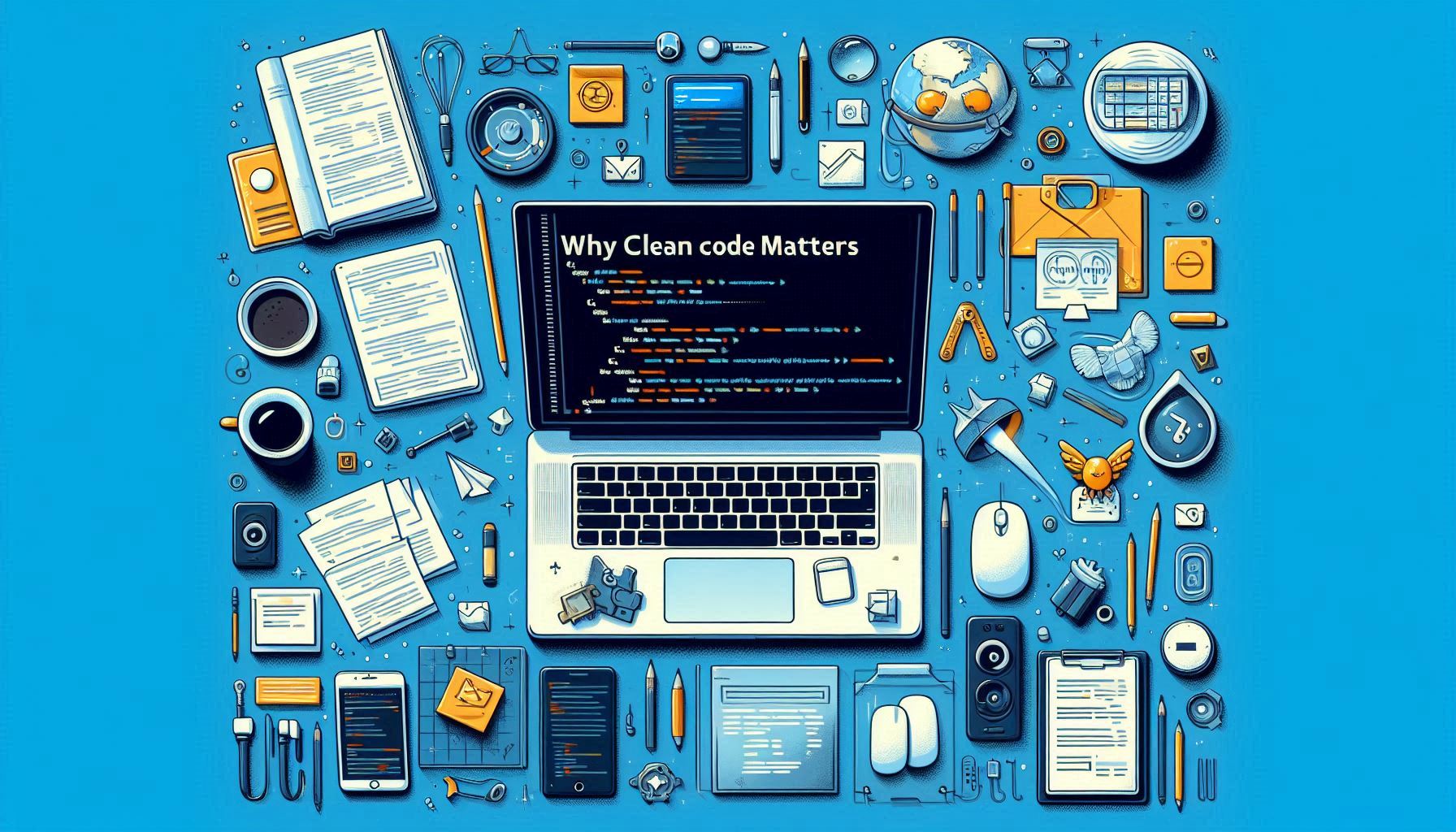
Introduction
Clean code is not just a nice-to-have feature in modern software development; it's a necessity. In an era where the pace of technological change is accelerating, and teams are becoming more distributed, writing clean and maintainable code is vital. Whether you're working alone or as part of a team, maintaining clean code will not only improve your efficiency but also enhance collaboration and reduce errors. Clean code practices are essential for long-term project success, helping teams scale, adapt, and continuously deliver quality software.
While the concept of clean code is straightforward, implementing it consistently requires diligence, discipline, and understanding. This blog delves into the fundamental principles behind clean code and offers actionable tips for developers who want to write code that is easier to read, maintain, and scale.
What is Clean Code?
Clean code refers to writing code that is easy to understand, read, and maintain. It's the kind of code that makes sense at first glance and reduces complexity for future developers. It focuses on readability, simplicity, and sustainability. The primary goal of clean code is to minimize the need for additional documentation by writing code that is self-explanatory.
Why Clean Code Matters: The Real-World Benefits
1. Improved Collaboration
When teams work on the same codebase, clear and clean code is key to effective collaboration. Clean code allows developers to communicate more efficiently because the code itself "speaks" the language. When everyone follows common standards and best practices, team members can jump into each other's work without a steep learning curve. This leads to faster onboarding and fewer misunderstandings in code reviews.
For instance, consider a team working on a large-scale project like a web application. If one developer writes poorly structured code with cryptic variable names and lack of documentation, other developers will waste time trying to understand what’s going on. In contrast, clean code is like a map that guides everyone, allowing the team to move faster and make fewer mistakes.
2. Faster Debugging and Problem Solving
Bugs are inevitable in software development, but clean code can make debugging easier. By adhering to naming conventions, avoiding unnecessary complexity, and writing modular functions, developers can pinpoint issues quickly without needing to decipher convoluted logic. Clean code leads to fewer bugs, and when bugs do arise, they’re easier to fix.
For example, a function that only does one thing and has clear parameters is much easier to test and debug compared to a large, monolithic block of code. By sticking to clean coding practices, you make life easier for your debugging team and reduce the time spent on bug fixes.
1. Follow Naming Conventions: Clarity is Key
Descriptive Variable and Function Names
The names you choose for your variables, functions, and classes play a significant role in making your code clean and understandable. Avoid cryptic abbreviations and choose meaningful names that convey the purpose of the variable or function.
Consistency in Naming
Consistency is critical. Stick to a naming convention across your codebase. Whether you use camelCase, snake_case, or PascalCase, make sure to apply it consistently to avoid confusion.
2. Keep Functions and Methods Small and Focused
Single Responsibility Principle
Each function or method should have one responsibility. It should do one thing and do it well. This is known as the Single Responsibility Principle (SRP), one of the SOLID principles of object-oriented programming.
Bad Practice: A function that handles both data processing and UI rendering.
Good Practice: A function that handles data processing, and another one that focuses on UI rendering.
Why It Matters
Smaller functions make it easier to test, debug, and maintain code. They also promote reusability and ensure that each function performs a specific task without unnecessary side effects.
3. Use Comments Wisely
Commenting Best Practices
While clean code should be self-explanatory, comments still have their place. Use comments to explain why certain decisions were made, especially if the code is complex or contains a workaround.
However, avoid over-commenting. If the code is simple and intuitive, it doesn’t need excessive comments.
Bad Practice: Adding comments that state the obvious.
// Add 1 to x
Good Practice: Comments that explain why the code is doing something unusual.
// Add 1 to x to account for the off-by-one error in the indexing logic
4. Eliminate Redundancy: Don't Repeat Yourself (DRY)
DRY Principle
The Don't Repeat Yourself (DRY) principle states that code duplication is a bad practice. Redundant code increases the chance of errors and makes it harder to maintain.
Instead of duplicating logic, you can create reusable functions or methods that handle common tasks.
Bad Practice: Copy-pasting the same logic in multiple places.
Good Practice: Refactor the logic into a reusable function and call it whenever needed.
5. Refactor Regularly
Keep Your Code Clean Over Time
Refactoring is the process of restructuring existing code without changing its external behavior. Over time, codebases accumulate technical debt, which can make them harder to maintain. Regular refactoring ensures that the codebase stays clean, efficient, and easy to extend.
Bad Practice: Ignoring technical debt and letting the codebase grow more complex.
Good Practice: Regularly reviewing and refactoring your code to remove inefficiencies, simplify logic, and improve readability.
Benefits of Refactoring
Improved performance
Easier testing and debugging
More maintainable code
6. Implement Proper Error Handling
Handle Errors Gracefully
Error handling is an important aspect of clean code. Unhandled exceptions and poorly managed errors can lead to crashes or unexpected behavior in applications.
Bad Practice: Ignoring potential errors or using generic error messages.
Good Practice: Implementing specific error-handling mechanisms, such as try-catch blocks, and logging relevant information to diagnose issues.
7. Prioritize Code Readability Over Cleverness
Simplicity is Key
It can be tempting to use clever tricks or short, complex code to achieve a result, but this often sacrifices readability. Developers should prioritize simple, understandable solutions over clever ones.
Bad Practice: Writing one-liners or complex logic that only a few developers can understand.
Good Practice: Writing simple and easy-to-follow code that is accessible to all team members.
8. Use Version Control for Collaboration
Benefits of Git and Other Version Control Systems
A good version control system (like Git) is essential for maintaining a clean codebase. It allows you to track changes, collaborate with others, and roll back changes when necessary. Proper use of version control makes it easier to keep the code organized and maintainable.
Bad Practice: Working without version control or committing incomplete code.
Good Practice: Committing small, logical changes with clear, descriptive commit messages.
Key Version Control Best Practices
Commit often, with meaningful messages.
Use branches for new features or bug fixes.
Rebase instead of merging to avoid cluttering the commit history.
9. Apply SOLID Principles
SOLID Principles for Maintainability
The SOLID principles are a set of five object-oriented design principles that help developers write clean, maintainable, and flexible code.
S – Single Responsibility Principle
O – Open/Closed Principle
L – Liskov Substitution Principle
I – Interface Segregation Principle
D – Dependency Inversion Principle
By applying these principles, you’ll be able to create code that’s easier to maintain, test, and extend.
10. Test Your Code
Write Automated Tests for Your Code
Testing is an integral part of writing clean code. By writing unit tests, integration tests, and end-to-end tests, you can ensure that your code is reliable and that changes won’t introduce new bugs.
Bad Practice: Writing code without testing it.
Good Practice: Implementing a robust testing strategy and using tools like Jest, Mocha, or JUnit to automate tests.
Conclusion
In conclusion, writing clean code is not just a best practice—it’s a way to ensure that your software is sustainable, scalable, and maintainable over time. By following the principles of clean code, such as meaningful naming, small functions, and eliminating duplication, you can improve the quality of your code and enhance collaboration within your team. Keep in mind that clean code is a mindset that requires continual effort and improvement, but the rewards are well worth it.
Investing time and effort in writing clean code leads to more maintainable, bug-free, and flexible applications. Start applying these principles today, and watch your development practices—and your software—improve.
Subscribe to my newsletter
Read articles from ana buadze directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
