Building an On-Chain Identity & Credit Scoring System Using CrossFi APIs

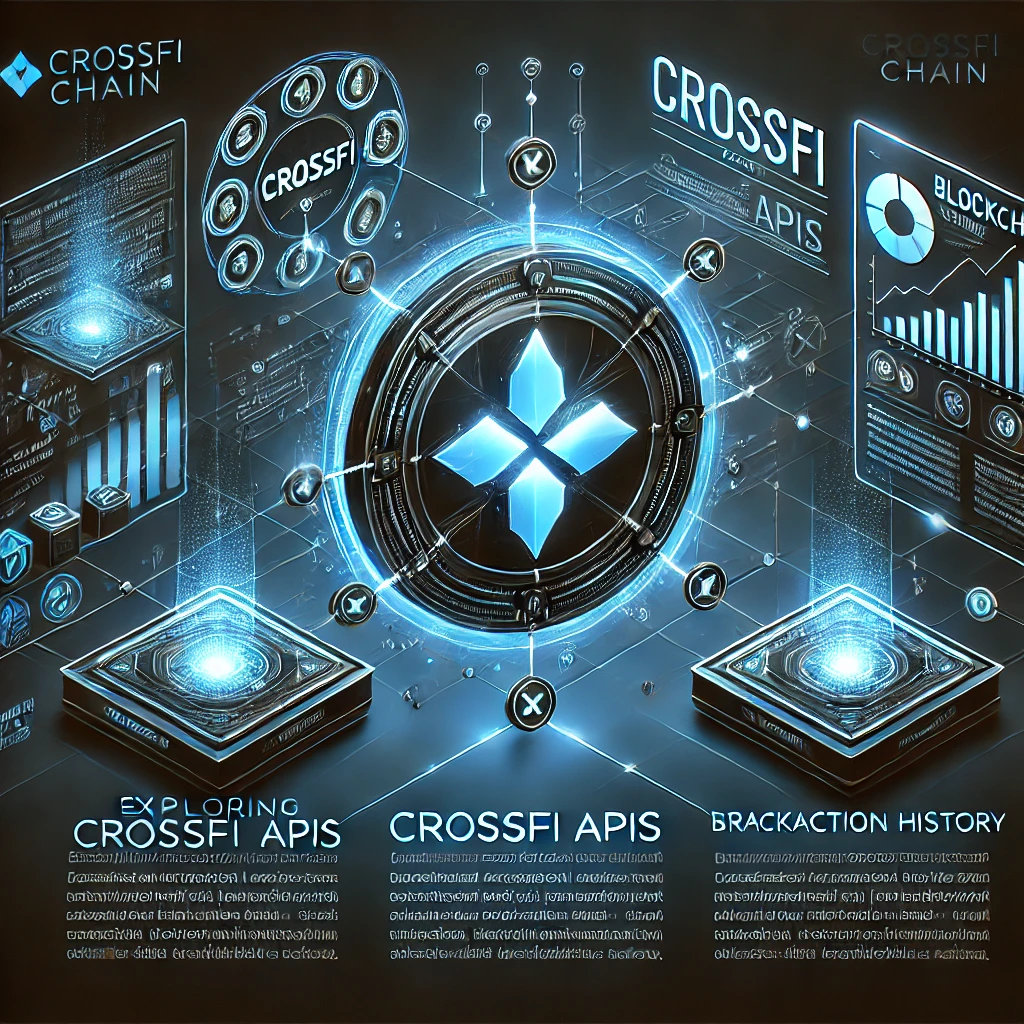
Introduction
In traditional finance, credit scores help banks assess borrower risk based on factors like loan history, income, and transaction behavior. However, DeFi (Decentralized Finance) lacks a universal credit scoring system, making undercollateralized lending nearly impossible.
In this guide, we'll build a decentralized credit scoring system using CrossFi APIs, which will:
Analyze on-chain transaction history to determine financial behavior.
Assign a credit score based on staking, lending, and repayment patterns.
Help lenders assess risk and provide loans based on a user’s blockchain activity.
1. How Will It Work?
This system will:
Fetch transaction history from the CrossFi blockchain using the API.
Analyze transactions (e.g., staking, borrowing, repaying, and wallet activity).
Calculate a credit score based on predefined risk models.
Expose an API for lenders to retrieve credit scores.
2. Fetching On-Chain Transactions
API Endpoint
We’ll use CrossFi's API to fetch transaction history:
GET https://test.xfiscan.com/api/1.0/txs?address={wallet_address}&page={page}&limit={limit}
Example Response
{
"txhash": "fe828e6bb3...",
"addresses": ["0x8465de...", "mx1s3jau..."],
"auth_info": {
"fee": {
"amount": [
{ "denom": "mpx", "amount": "1561680000000000000" }
]
}
},
"body": {
"messages": [
{
"inputs": [
{ "address": "mx1s3jau...", "coins": [ { "denom": "mpx", "amount": "1000000000000000000" } ] }
],
"outputs": [
{ "address": "mx1qeg...", "coins": [ { "denom": "mpx", "amount": "1000000000000000000" } ] }
]
}
]
},
"gas_used": "129095",
"height": 7665269,
"timestamp": "2025-02-23T10:50:29.194Z"
}
3. Building the Credit Scoring System
Step 1: Setup a Flask API
Create a Flask API that fetches on-chain data and calculates a credit score.
Install dependencies
pip install flask requests
Folder Structure
onchain-credit-score/
│── app.py # Main Flask API
│── config.py # API settings
│── models.py # Credit scoring logic
│── requirements.txt # Dependencies
Step 2: Fetch Transaction History
In app.py
:
from flask import Flask, request, jsonify
import requests
from config import CROSSFI_API_BASE
app = Flask(__name__)
def fetch_transactions(address):
url = f"{CROSSFI_API_BASE}/txs"
params = {"address": address, "page": 1, "limit": 20}
response = requests.get(url, params=params)
return response.json() if response.status_code == 200 else {"error": "Failed to fetch transactions"}
@app.route('/credit-score', methods=['GET'])
def get_credit_score():
address = request.args.get("address")
if not address:
return jsonify({"error": "Address required"}), 400
transactions = fetch_transactions(address)
credit_score = calculate_credit_score(transactions)
return jsonify({"address": address, "credit_score": credit_score})
if __name__ == "__main__":
app.run(debug=True)
Step 3: Define the Credit Scoring Model
Create models.py
:
def calculate_credit_score(transactions):
score = 500 # Default base score
for tx in transactions.get("txs", []):
gas_used = int(tx.get("gas_used", 0))
amount_sent = sum(int(coin["amount"]) for msg in tx["body"]["messages"] for coin in msg.get("inputs", [{}])[0].get("coins", []))
# Reward users for sending large transactions
if amount_sent > 10**18:
score += 20
# Penalize for high gas usage
if gas_used > 150000:
score -= 10
return min(max(score, 300), 850) # Normalize score between 300 and 850
4. Testing the API
Run the Flask API:
python app.py
Test it using CURL or Postman:
GET http://127.0.0.1:5000/credit-score?address=0x8465de71a0c24c...
Example Response
{
"address": "0x8465de71a0c24c...",
"credit_score": 720
}
Subscribe to my newsletter
Read articles from Big Fibo directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
