Stop returning a list of sys_id's
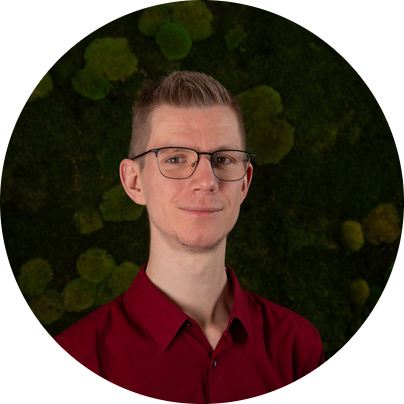

In ServiceNow, records are uniquely identified by their sys_id. When there's a need to retrieve a list of records, the sys_id is often used to fetch these records. However, is this the most efficient method for returning a list of records?
Let’s break it down into a simple example. You got a business rule running on the Incident table. The business rule should contain a script to close all related incident tasks. You also got a script include “IncidentService” containing all business logic for incidents. The objective is to retrieve all the related incident task records and close them.
How NOT to do it
The following example is executing a GlideRecord, followed by adding all the sys_id to an array. At the end of the query, the array is returned.
getRelatedIncidentTasks: function(incident){
var taskIds = [];
var grIncidentTask GlideRecord('incident_task');
grIncidentTask.addQuery('parent', incident.getUniqueValue());
grIncidentTask.query();
while(grIncidentTask.next()){
taskIds.push(grIncidentTask.getUniqueValue());
}
return taskIds;
}
Pros & cons
✅ Very performance friendly
✅ Can be returned to the client side
❌ No possibility to retrieve additional attributes or related parent references
How to do it right
Instead of fetching just the sys_id, and returning it, return a queried variant of the GlideRecord Object. This is a very simple and reliable way to return the results whilst still being able to decide how the result should be utilized.
getRelatedIncidentTasks: function(incident){
var taskIds = [];
var grIncidentTask GlideRecord('incident_task');
grIncidentTask.addQuery('parent', incident.getUniqueValue());
grIncidentTask.query();
return grIncidentTask;
}
This is what the business rule could look like.
We are fetching the queried GlideRecord object, then we query over the results.
var incidentService = new IncidentService();
var grIncidentTask = incidentService.getRelatedIncidentTasks(current);
while(grIncidentTask.next()){
grIncidentTask.setValue('state','7'); //close
grIncidentTask.update();
}
Pros & cons
✅ Still performance friendly
✅ Reusable for fetching values (or parent records) without the need for additional GlideRecord
❌ GlideRecord instances cannot be retrieved on the Client side.
Conclusion
In conclusion, while using sys_id to retrieve records in ServiceNow is common, it may not be the most efficient method. Returning a queried variant of the GlideRecord Object enhances performance and reusability, allowing for more flexible data handling. Although it has limitations, such as not retrieving GlideRecord instances on the client side, this approach aligns with the service pattern by consolidating business logic within the service layer, leading to more robust and maintainable code.
In the next chapter, we will cover about delegating responsibilities to the service layer.
Subscribe to my newsletter
Read articles from Quinten Van den Berghe directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
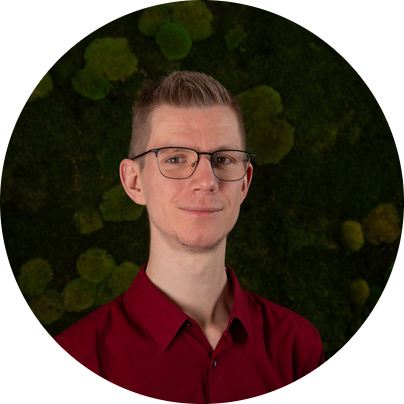
Quinten Van den Berghe
Quinten Van den Berghe
I am a technical architect and ServiceNow enthousiast. Since then I have been obsessed with development and later on application design & architecture. Today I'm using my skills to design solutions for IT companies within the ServiceNow industry whilst in my free time, spent the majority of my time creating applications for all kind of things unrelated to ServiceNow.