The Importance of Naming Conventions in Software Development

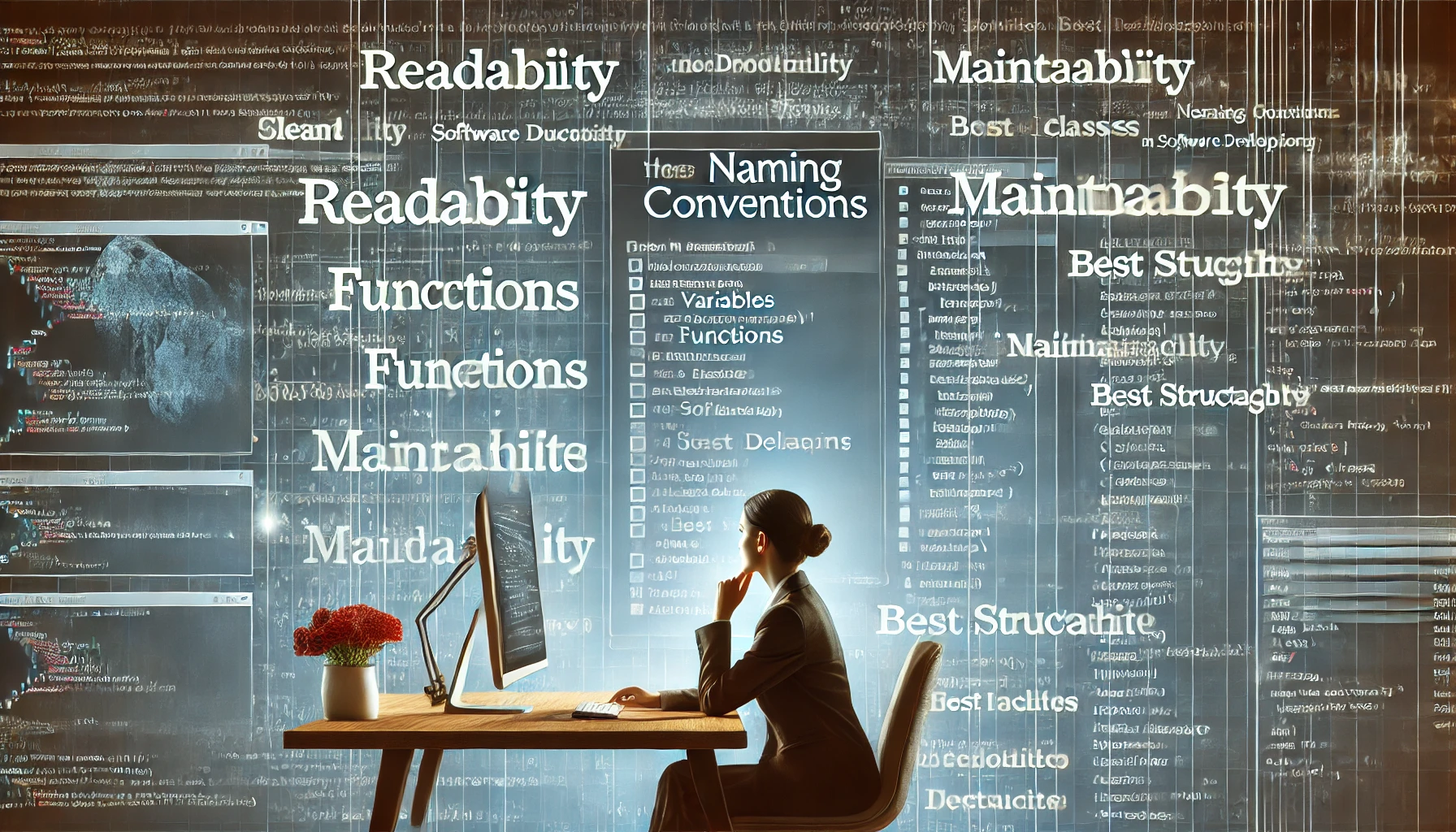
In the world of software development, writing clean, readable, and maintainable code is crucial. One of the most important, yet often overlooked, aspects of writing high-quality code is naming conventions. Naming conventions refer to the guidelines developers follow when naming variables, functions, classes, and other entities in their code. While naming conventions might seem like a small detail, they play a significant role in the quality of your codebase. In this blog, we’ll explore why naming conventions are so important, how they help improve collaboration and readability, and how they contribute to long-term maintainability in any software project.
Why Naming Conventions Matter
1. Improving Readability and Understanding
The main goal of using naming conventions is to improve the readability and understanding of the code. Whether you're working solo or as part of a team, clear, consistent names help developers immediately understand the purpose of variables, functions, or classes. This clarity reduces the cognitive load needed to comprehend the code and saves time in the long run.
Let’s take a look at some practical JavaScript examples:
Bad Naming Example:
let x = 10;
let y = "John";
let z = [1, 2, 3];
Good Naming Example:
let age = 10;
let name = "John";
let numbers = [1, 2, 3];
In the bad naming example, the variables x
, y
, and z
are vague and don’t give any hint about what they represent. A developer would need to dig deeper into the code to understand what each variable is used for. In the good naming example, variables like age
, name
, and numbers
provide immediate insight into their contents. This type of clarity is important for readability and maintenance, especially when revisiting code after a few months or working with a team.
2. Collaboration and Team Efficiency
In larger software projects, multiple developers often work on the same codebase. Without naming conventions, the lack of consistency in variable and function names can quickly lead to confusion. Adopting a shared naming convention ensures that everyone on the team understands the purpose of each piece of code, leading to better collaboration and fewer misunderstandings.
Bad Naming Example:
function a(x, y) {
return x + y;
}
Good Naming Example:
function addNumbers(x, y) {
return x + y;
}
In the bad naming example, the function name a
is not descriptive at all and doesn’t tell you anything about what the function does. However, in the good naming example, the function name addNumbers
is clear and self-explanatory, making it much easier for any developer reading the code to understand its behavior. This improves collaboration and efficiency, as there is no need for extra clarification or documentation.
3. Maintainability and Long-Term Code Health
As software projects evolve over time, code is often modified, updated, and expanded. Clear and consistent naming conventions make the code more maintainable and help reduce the chances of introducing errors during future changes. Without consistent naming, you might find yourself or your team struggling to decipher old code when you return to work on it months or years later.
Bad Naming Example:
let a = 5;
let b = 10;
let c = a + b;
Good Naming Example:
let basePrice = 5;
let taxAmount = 10;
let totalPrice = basePrice + taxAmount;
In the bad naming example, the variables a
, b
, and c
are generic and don’t convey any useful context. If you revisit this code later, it may take time to figure out what those variables represent. In contrast, the good naming example uses descriptive names like basePrice
, taxAmount
, and totalPrice
, making it much easier to understand what’s happening in the code and ensuring that future modifications are straightforward.
4. Consistency with Industry Conventions
In many programming languages, there are widely accepted naming conventions that help standardize code and ensure that it integrates smoothly with other projects. JavaScript, for example, has a few commonly followed naming conventions: camelCase for variables and functions, UPPERCASE_SNAKE_CASE for constants, and PascalCase for classes.
Bad Naming Example:
const Max_value = 100;
let user_id = 123;
Good Naming Example:
const MAX_VALUE = 100;
let userId = 123;
In the bad naming example, the constant Max_value
uses inconsistent casing, and the variable user_id
uses an underscore, which is not typical in JavaScript. In the good naming example, MAX_VALUE
is written in uppercase to follow the convention for constants, and userId
uses camelCase, which is the JavaScript standard for variables. Following these conventions ensures your code is consistent with the broader ecosystem and easier for other developers to follow.
5. Avoiding Ambiguity in Function Names
A key rule when naming functions is that the name should clearly describe what the function does. Ambiguous or generic names can lead to confusion and bugs down the road.
Bad Naming Example:
function handleData(a, b) {
return a + b;
}
Good Naming Example:
function sumNumbers(a, b) {
return a + b;
}
In the bad naming example, the function name handleData
is too vague. It doesn’t provide any indication of what kind of data is being handled or what the function is supposed to do. The good naming example, sumNumbers
, clearly tells you that the function is summing two numbers, making the code more intuitive and easier to maintain.
Conclusion
Naming conventions might seem like a minor detail in the grand scheme of software development, but they have a major impact on the quality, readability, and maintainability of your code. By adopting consistent naming conventions in your JavaScript projects (and in any programming language you use), you can improve collaboration, enhance code clarity, and ensure long-term maintainability.
Whether it’s choosing descriptive names for your variables and functions, following established naming patterns like camelCase, or ensuring your function names are clear and unambiguous, the time you invest in naming conventions will pay off in the long run. Your future self—and your team—will appreciate the effort, making it easier to maintain, scale, and improve the codebase as the project grows.
Subscribe to my newsletter
Read articles from Raj Goldar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
