Solidity Series: Part 1 - Introduction to Solidity

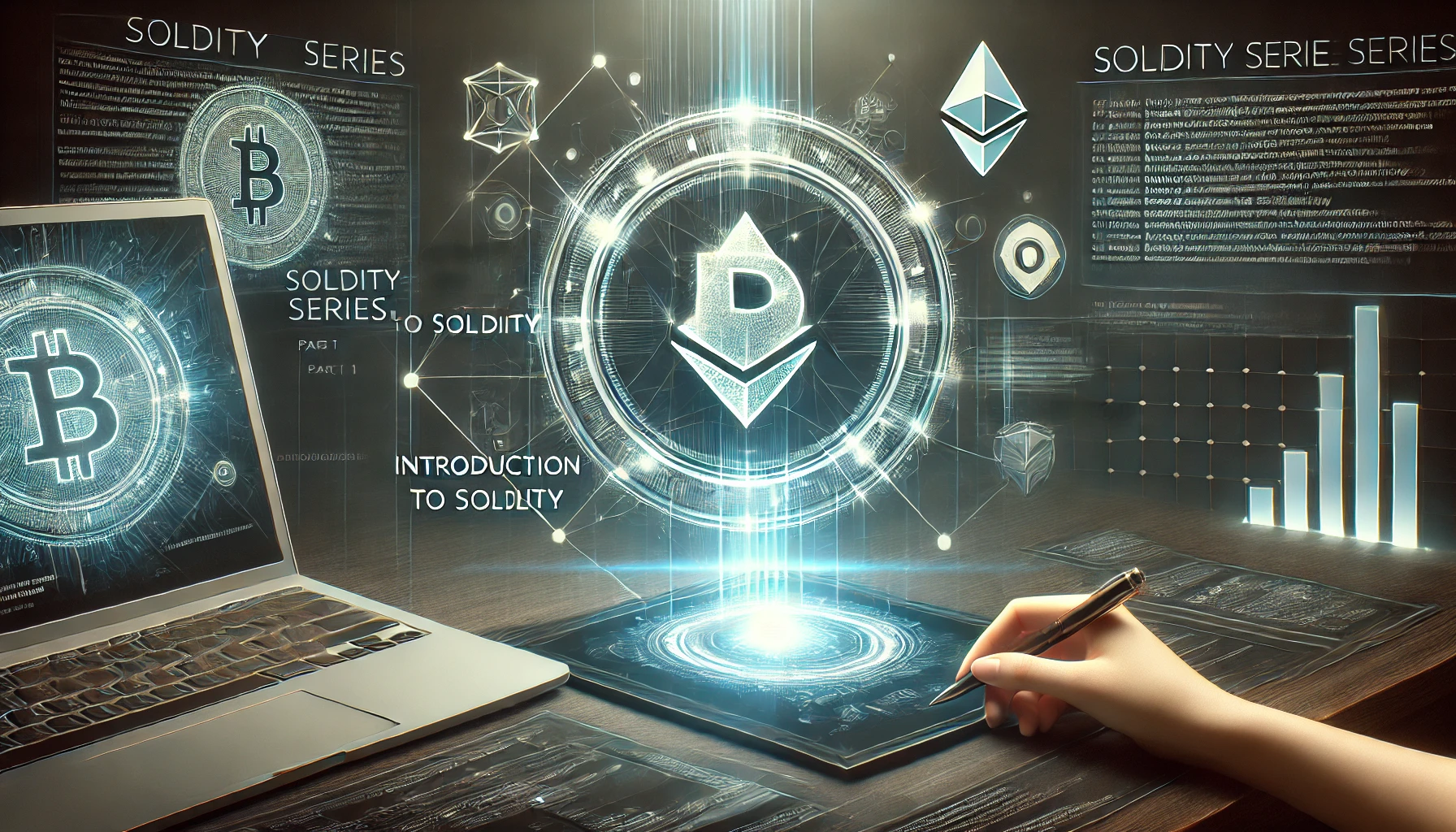
Solidity is a high-level, object-oriented programming language designed for developing smart contracts that run on blockchain networks, primarily Ethereum. Solidity enables developers to write self-executing contracts with predefined rules and automation.
High-Level Language: Solidity is designed to be developer-friendly, with syntax similar to JavaScript, Python, and C++. This makes it accessible to programmers familiar with traditional coding languages.
Object-Oriented: Solidity follows object-oriented programming (OOP) principles, allowing for structured and modular contract development. Concepts like inheritance, polymorphism, and encapsulation improve code organization and reusability.
Smart Contract Development: Solidity is purpose-built for writing smart contracts, enabling decentralized transactions, token creation, voting mechanisms, and more.
Blockchain Integration: Solidity executes on the Ethereum Virtual Machine (EVM), ensuring secure and deterministic execution of contract logic across the blockchain.
If you are unfamiliar with blockchain or smart contracts, consider reading this article before proceeding.
Setting Up Your Development Environment
Before writing and deploying smart contracts, you need to set up a Solidity development environment. Here’s how:
Requirements:
Node.js & npm: Install from nodejs.org.
Hardhat (Recommended) or Truffle: These frameworks help with smart contract development and testing.
Setting up Hardhat:
Install Node.js and npm.
Create a new project:
mkdir solidity && cd solidity
npm init -y
Install Hardhat:
npm install --save-dev hardhat
npx hardhat
You’ll see a prompt asking, “What do you want to do?”. Select “Create a JavaScript project”.
Understanding the Hardhat Project Folder Structure
Your project folder will have the following structure:
solidity-project/
|── contracts/ # Where you write your Solidity smart contracts
│── scripts/ # Deployment scripts for deploying contracts
│── test/ # Test scripts to verify contract functionality │── hardhat.config.js # Configuration file for Hardhat
contracts/
→ This folder contains your smart contracts. Hardhat generates a sample contract (Lock.sol
) to help you get started.scripts/
→ This folder includes JavaScript files for deploying contracts to the blockchain. The sampledeploy.js
script provides a deployment example.test/
→ This folder holds test scripts to verify contract logic before deploying to a real network.hardhat.config.js
→ The main configuration file where you define Solidity versions, networks, and plugins.Simple Solidity Contract Example
// SPDX-License-Identifier: MIT pragma
solidity ^0.8.0;
contract SimpleStorage {
uint256 private storedData; // Variable to store a value
// Constructor to initialize the contract
constructor() {
storedData = 0; // Initialize storedData to 0
}
// Function to store a new value
function set(uint256 data) public {
storedData = data; // Store the value passed to the function
}
// Function to retrieve the stored value
function get() public view returns (uint256) {
return storedData; // Return the stored value
}
}
This contract allows users to store and retrieve a single integer value. It starts by declaring a state variable
storedData
of typeuint256
, which holds the value. The contract includes a constructor that sets thestoredData
variable to 0 when the contract is first deployed.The
set
function lets anyone store a new value by passing a number to it, which replaces the previous value instoredData
. This function ispublic
, meaning any user can call it externally. Theget
function, alsopublic
, is a view function that allows anyone to retrieve the current value stored instoredData
without changing the contract state. It simply returns the stored value.In summary, this contract shows how to store a value on the blockchain and retrieve it later, serving as a basic example of Solidity's ability to handle state and interact with smart contracts.
Conclusion
In this article, we introduced Solidity and its core features, including its use in building decentralized applications on Ethereum. We also covered setting up a Solidity development environment with Hardhat and created a basic contract to store and retrieve data.
In the next article, we will dive deeper into Solidity, exploring data types, functions, inheritance, and other advanced concepts to help you write more powerful and efficient smart contracts. Keep experimenting and learning!
Subscribe to my newsletter
Read articles from Zahab kakar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
