Copying Files Using System Calls in C

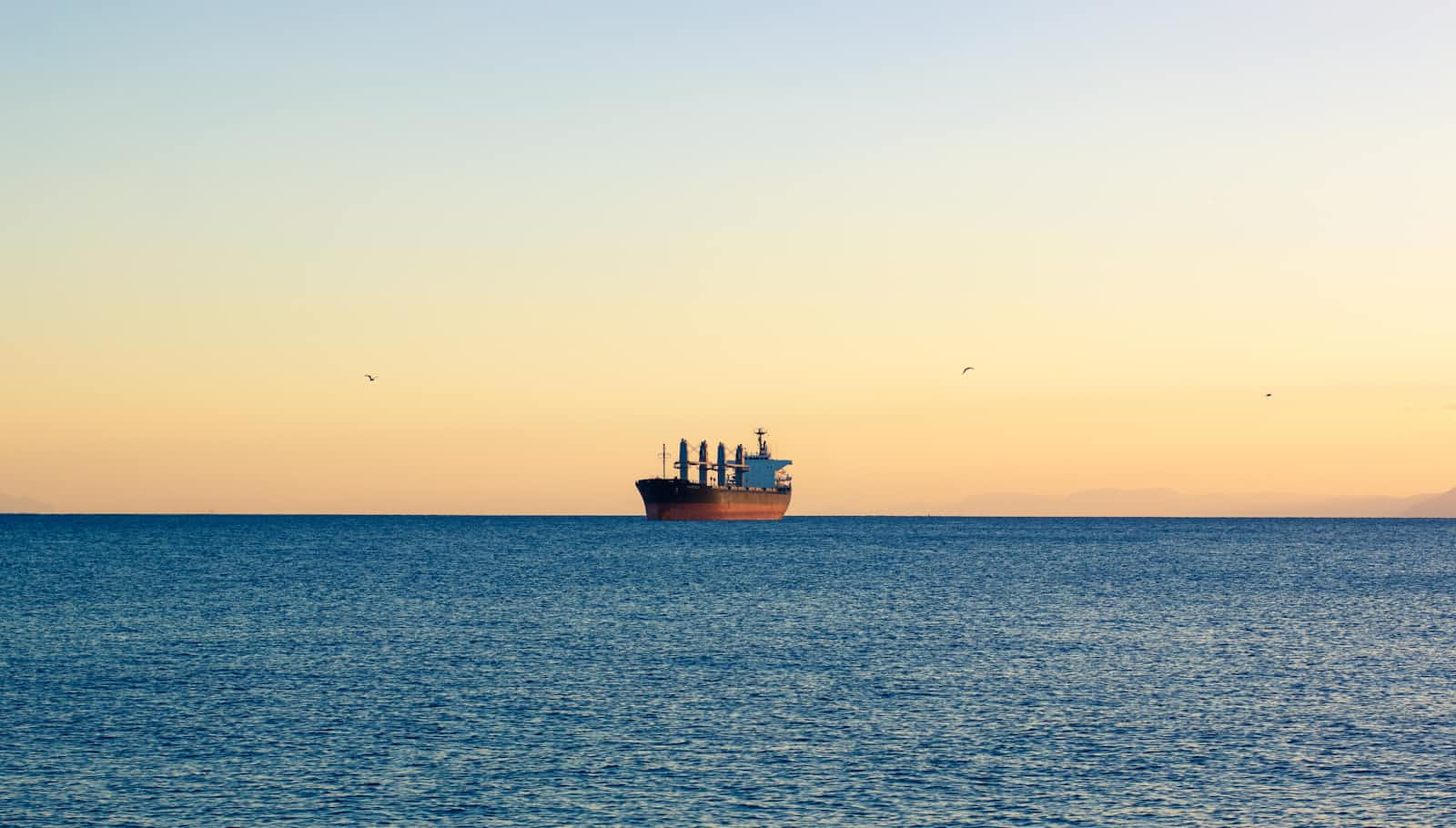
This C program copies a file from one place to another through a system call. The user supplies the source and destination file names as arguments, and the program executes a Linux shell command to do the copy.
How It Works
The program has two arguments:
The source file (the file to be copied).
The destination file (where the copy will go).
It then builds a Linux cp command with snprintf() and runs it with system(). It displays an error message if the command is unsuccessful.
Parent and Child Process in System Calls When system() is called, the parent process (the main program) creates a child process to run the command. The child executes the cp command and then exits, returning control to the parent process. This is an important idea in process management in operating systems.
Why This Is Useful This tool is designed to automate file copying with system calls, and thus it can be applied to scripting or file management in C-based automation. It adopts the same parent-child process model applied in most system operations.
#include <stdio.h>
#include <stdlib.h>
#include <sys/types.h>
#include <sys/stat.h>
#include <dirent.h>
#include <unistd.h>
#include <pwd.h>
#include <grp.h>
#include <time.h>
int main(int argc, char *argv[]) {
/*if(argc!=3){
printf("noo");
exit(EXIT_FAILURE);
}*/
// Construct the Linux command to find and remove empty files
char command[1024];
snprintf(command, sizeof(command), "cp '%s' '%s'",argv[1],argv[2]);
// Execute the command
int ret = system(command);
// Check if the command executed successfully
if (ret == -1) {
perror("Error executing command");
return EXIT_FAILURE;
}
return 0;
}
I developed a password generator program and created an app for it, and I'll share more about it later after my vacation. Hope you learnt something today.
Happy Coding…
Subscribe to my newsletter
Read articles from Gagan G directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Gagan G
Gagan G
I'm passionate about coding and enjoy working with embedded systems, app development, and compiler design.