[LeetCode] Top Interview 150 Problems Solving # 151 Reverse Words in a String

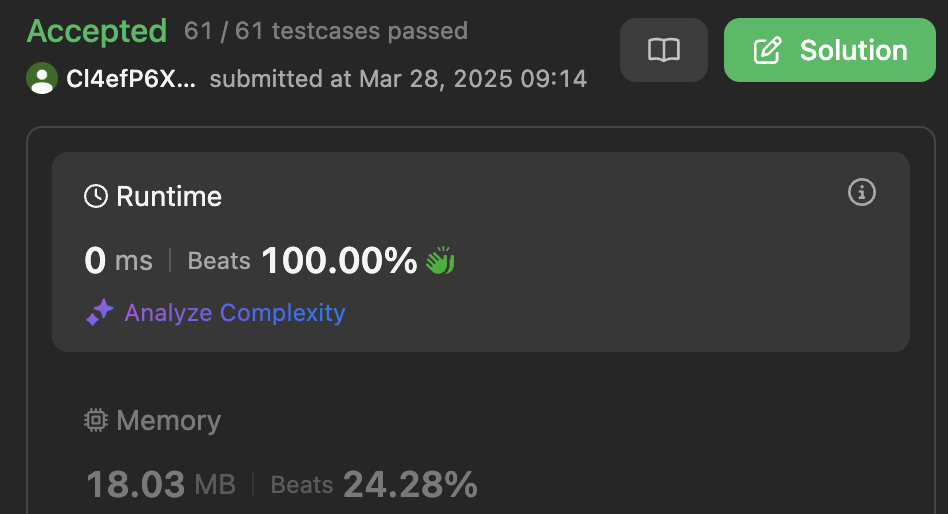
Understanding the Problem
A string is given with spaces. The string could be a single character or a complete sentence. It is to reverse the string by words. The spaces at the edges should be cut off.
# example 1
Input: s = "the sky is blue"
Output: "blue is sky the"
# example 2
Input: s = " the sky is blue "
Output: "blue is sky the"
Approach
I would first make it a list, the reverse it. Quite a simple problem it seems.
Solution
I made the string a list by split()
so it will automatically remove all the spaces and make a word list. s_list
below will have the value s_list = [“the”, ”sky”, ”is”, ”blue”]
. Afterwards, I reversed the list with s_list[::-1]
. This index sorting means it will be reversed with the value -1
. In slicing index has got the sequence as [start:stop:step]. When the step has a negative value, this means in a reverse order, so in our case it was to select every elements by emptying start and stop, then reverse it with -1
to enumerate every element.
When the list is reversed, then I simply joined the list.
class Solution:
def reverseWords(self, s: str) -> str:
s_list = s.split()
return " ".join(s_list[::-1])
Reflection
If I had no knowledge about the internal methods like split()
, join()
and slicing technique, this problem would’ve been a conundrum. Luckily my answer had the time complexity of 0ms.
Subscribe to my newsletter
Read articles from Ramhee Yeon directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Ramhee Yeon
Ramhee Yeon
South Korean, master's degree of AI. Programmer for LLM, app and web development. I am seeking opportunities to go to the USA and Canada, and Europe. I used to live in Frankfurt, Moscow, Pretoria, Baguio. Where to next?