How to Set Up a New Next.js Project and Install Tailwind CSS (2025 Guide)

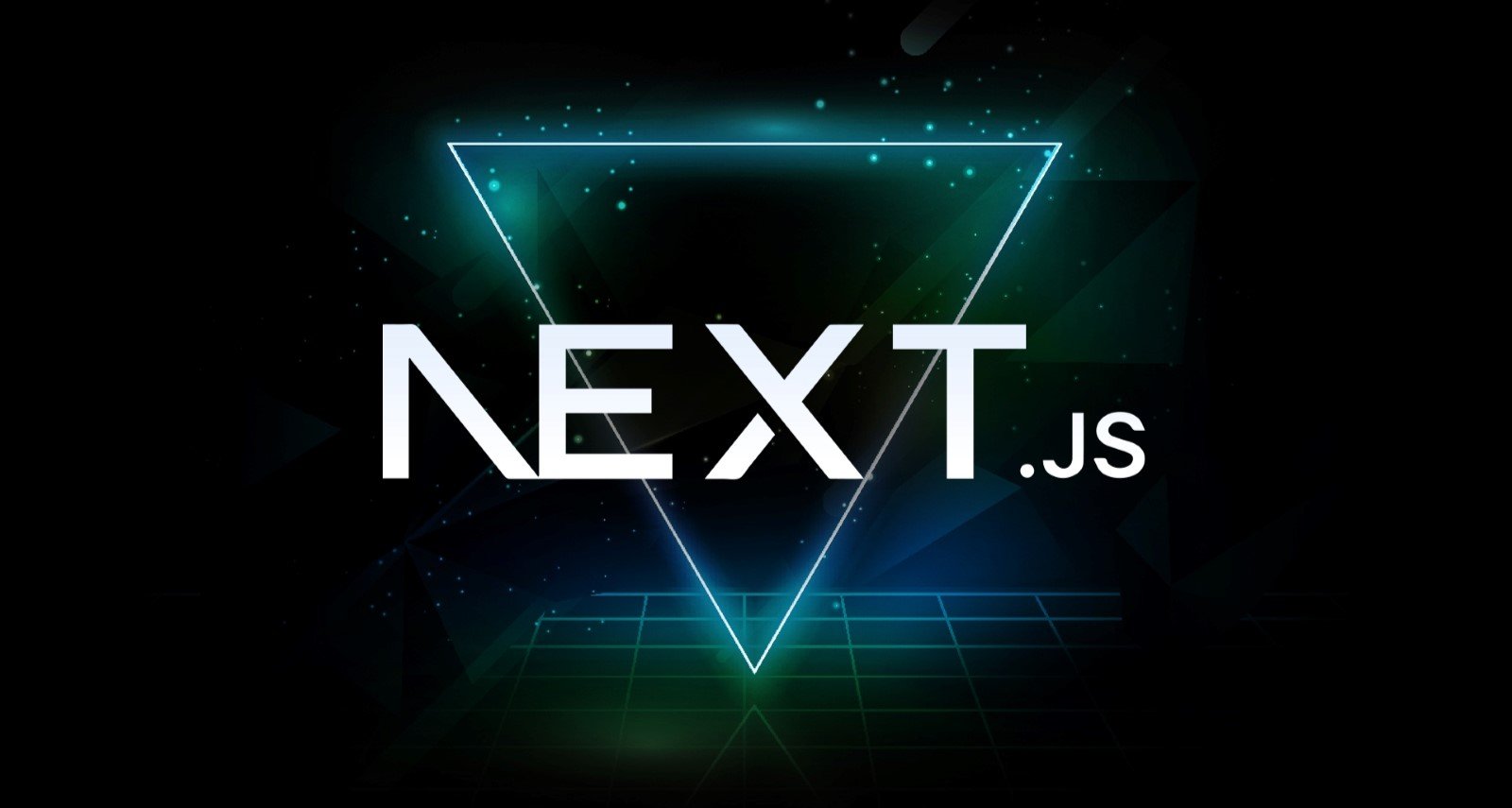
Next.js is a powerful React framework built by Vercel that makes it easy to create production-grade web applications. It extends React with powerful features like:
β Server-Side Rendering (SSR)
β‘ Static Site Generation (SSG)
π Hybrid Rendering (both SSR + SSG)
π§ Built-in API routes
π SEO optimization
π οΈ Image optimization, routing, and more!
When Next.js is paired with Tailwind CSS, it allows you to build sleek, fast, and scalable modern web applications.
In this guide, weβll walk you through the process of setting up a brand new Next.js project (using the latest v15+) and installing Tailwind CSS v4.1βstep by step using the latest create-next-app
prompts.
β Prerequisites
Make sure you have these installed:
Node.js (v18 or later recommended)
npm (comes with Node)
VS Code or your favorite code editor
βοΈ Step 1: Create a New Next.js App
Open your terminal and run:
npx create-next-app@latest
Youβll be prompted with several options to customize your setup:
β What is your project named? β¦ my-app
β Would you like to use TypeScript? β¦ Yes
β Would you like to use ESLint? β¦ Yes
β Would you like to use Tailwind CSS? β¦ Yes
β Would you like your code inside a `src/` directory? β¦ Yes
β Would you like to use App Router? (recommended) β¦ Yes
β Would you like to use Turbopack for `next dev`? β¦ Yes
β Would you like to customize the import alias (`@/*` by default)? β¦ No
π‘ Pro Tip: Select βYesβ for Tailwind CSS and App Router to unlock modern Next.js power.
Once complete, it sets up the entire project with Tailwind, ESLint, TypeScript, and even a Git repo. Hereβs what the terminal will show:
Success! Created my-app at /your/path/to/my-app
Alternatively, you can quickly scaffold a new Next.js project with Tailwind, TypeScript, ESLint, App Router, and more using this one-liner: npx create-next-app@latest my-app --typescript --eslint --tailwind --src-dir --app --import-alias "@/*"
π‘ This sets everything up automatically without the need to answer prompts manually!
Once the setup is complete, navigate into your project folder and open it in VS Code:
cd my-app
code .
π§΅ Step 2: Run the Dev Server
Spin up your dev server with:
npm run dev
If you chose Turbopack, you'll see a blazing-fast startup and your app will be live at:
http://localhost:3000
π You've successfully created a Next.js 15.2.4 project with Tailwind CSS, TypeScript, ESLint, App Router, and Turbopack β fully set up and running on http://localhost:3000
.
π¨ Step 3: Start Using Tailwind CSS
Since you selected Tailwind CSS in the setup, itβs already configured! You can now start styling using utility classes like this:
export default function Home() {
return (
<main className="flex min-h-screen items-center justify-center bg-gray-100">
<h1 className="text-4xl font-bold text-blue-600">Hello Tailwind + Next.js!</h1>
</main>
);
}
The global styles and Tailwind config are already placed inside the src
directory:
src/app/globals.css
tailwind.config.ts
postcss.config.mjs
π§ͺ Bonus: Folder Structure
Hereβs a quick peek at the auto-generated folder structure:
my-app/
ββ src/
β ββ app/
β β ββ page.tsx
β β ββ layout.tsx
β ββ styles/
β β ββ globals.css
ββ tailwind.config.ts
ββ postcss.config.mjs
ββ tsconfig.json
π Conclusion
Thatβs it! You now have a fully working Next.js + Tailwind CSS app using the latest features like App Router, Turbopack, and TypeScript β all preconfigured.
You can now:
Build full-stack apps using API routes
Add dynamic pages via the App Router
Easily style with Tailwind utility classes
Optimize performance with Turbopack
Happy building!
π Connect With Me
If you found this helpful, give it a like and share it with your dev friends. You can connect with me on:
Subscribe to my newsletter
Read articles from Deepak Modi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Deepak Modi
Deepak Modi
Hey! I'm Deepak, a Full-Stack Developer passionate about web development, DSA, and building innovative projects. I share my learnings through blogs and tutorials.