Building Your First Android App: A Beginner's Guide

Table of contents
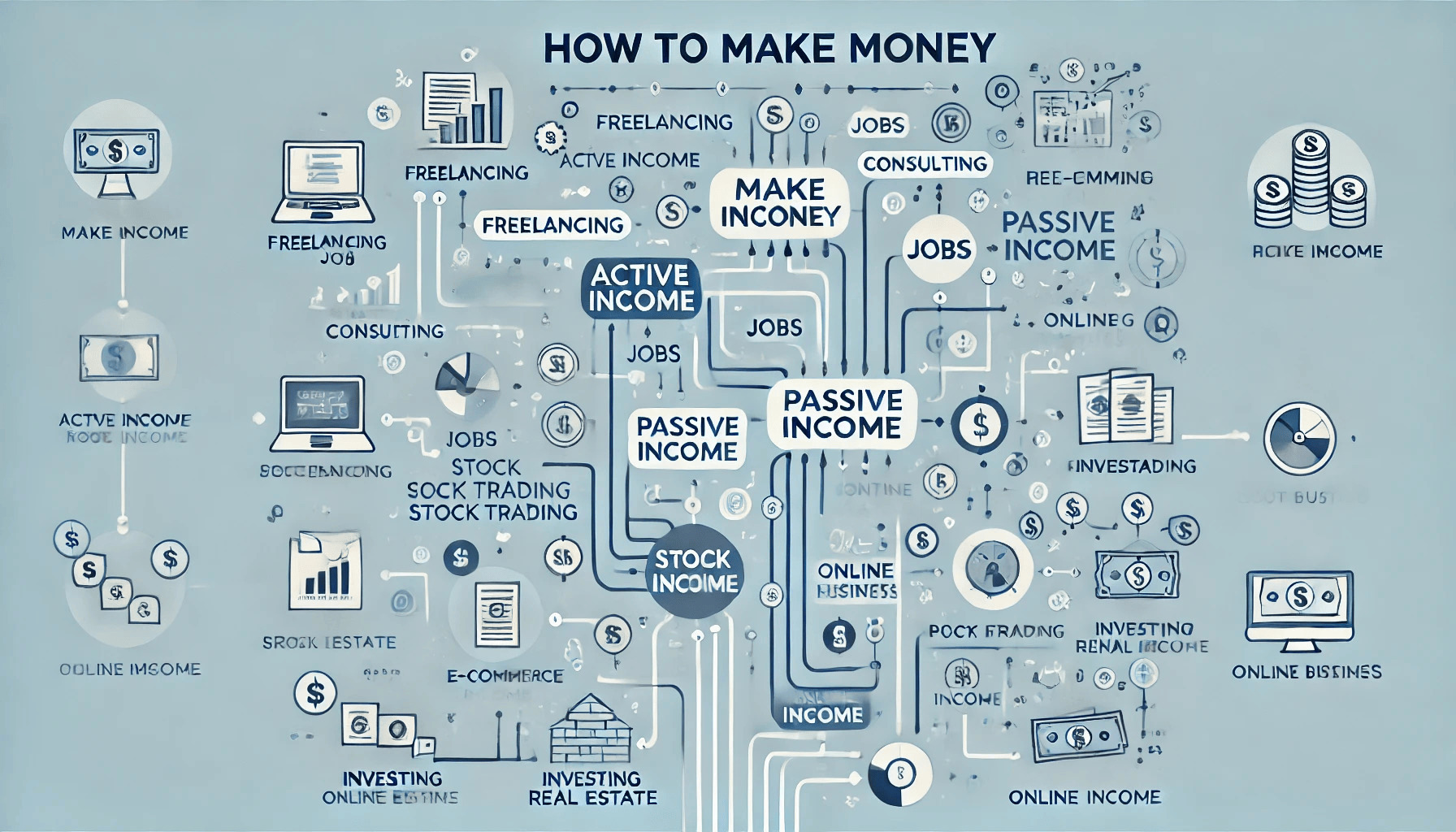
Building Your First Android App: A Beginner's Guide
Are you eager to dive into Android app development but don’t know where to start? You’re in the right place! This beginner-friendly guide will walk you through the essential steps to build your first Android app. By the end, you’ll have a functional app and the foundational knowledge to expand your skills.
Plus, if you're looking to monetize your programming expertise, check out MillionFormula, a fantastic platform to make money online—completely free, with no credit or debit cards required!
Prerequisites
Before we begin, ensure you have:
A computer running Windows, macOS, or Linux.
Android Studio installed (download from the official site).
Basic understanding of Java or Kotlin (we’ll use Kotlin in this guide).
Step 1: Setting Up Android Studio
Android Studio is the official IDE for Android development. After installing:
Open Android Studio and select "Start a new Android Studio project".
Choose "Empty Activity" and click Next.
Configure your project:
Name: MyFirstApp
Package name: com.example.myfirstapp
Save location: Choose a folder
Language: Kotlin
Minimum SDK: API 21 (Android 5.0)
Click Finish, and Android Studio will generate your project.
Step 2: Understanding the Project Structure
Your Android project consists of several key files:
MainActivity.kt
– Your app’s main logic.activity_main.xml
– The UI layout file.AndroidManifest.xml
– Defines app permissions and components.
Step 3: Designing the User Interface
Open activity_main.xml
and switch to the Design tab. Drag a Button and a TextView onto the screen.
Alternatively, edit the XML directly:
xml
Copy
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:gravity="center">
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hello World!" />
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Click Me" />
</LinearLayout>
Run HTML
Step 4: Adding Functionality
Now, let’s make the button update the text when clicked. Open MainActivity.kt
and modify it: kotlin Copy
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
val textView = findViewById<TextView>(R.id.textView)
val button = findViewById<Button>(R.id.button)
button.setOnClickListener {
textView.text = "Button Clicked!"
}
}
}
Step 5: Running Your App
Connect an Android device via USB (enable USB Debugging in Developer Options).
Or, use the Android Emulator (set up via AVD Manager in Android Studio).
Click the Run button (green play icon).
Your app should launch, and clicking the button will change the text!
Step 6: Publishing Your App (Optional)
Once your app is ready, you can publish it on the Google Play Store:
Create a Google Play Developer Account (sign up here).
Generate a signed APK or App Bundle (in Android Studio: Build > Generate Signed Bundle / APK).
Upload it to the Play Console and follow the submission steps.
Next Steps
Now that you’ve built your first app, consider learning:
RecyclerView for lists (official guide)
Networking with Retrofit (GitHub repo)
Firebase Integration for backend services (Firebase docs)
Monetize Your Skills
If you want to earn money with your programming skills, MillionFormula is a great platform to start—no hidden fees or credit card requirements!
Final Thoughts
Building your first Android app is an exciting milestone. Keep experimenting, learning, and refining your skills. The Android ecosystem is vast, and there’s always something new to explore.
Got questions? Drop them in the comments below! Happy coding! 🚀
Subscribe to my newsletter
Read articles from MillionFormula directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
