Terraform Associate: Perform Dynamic Operations with Functions

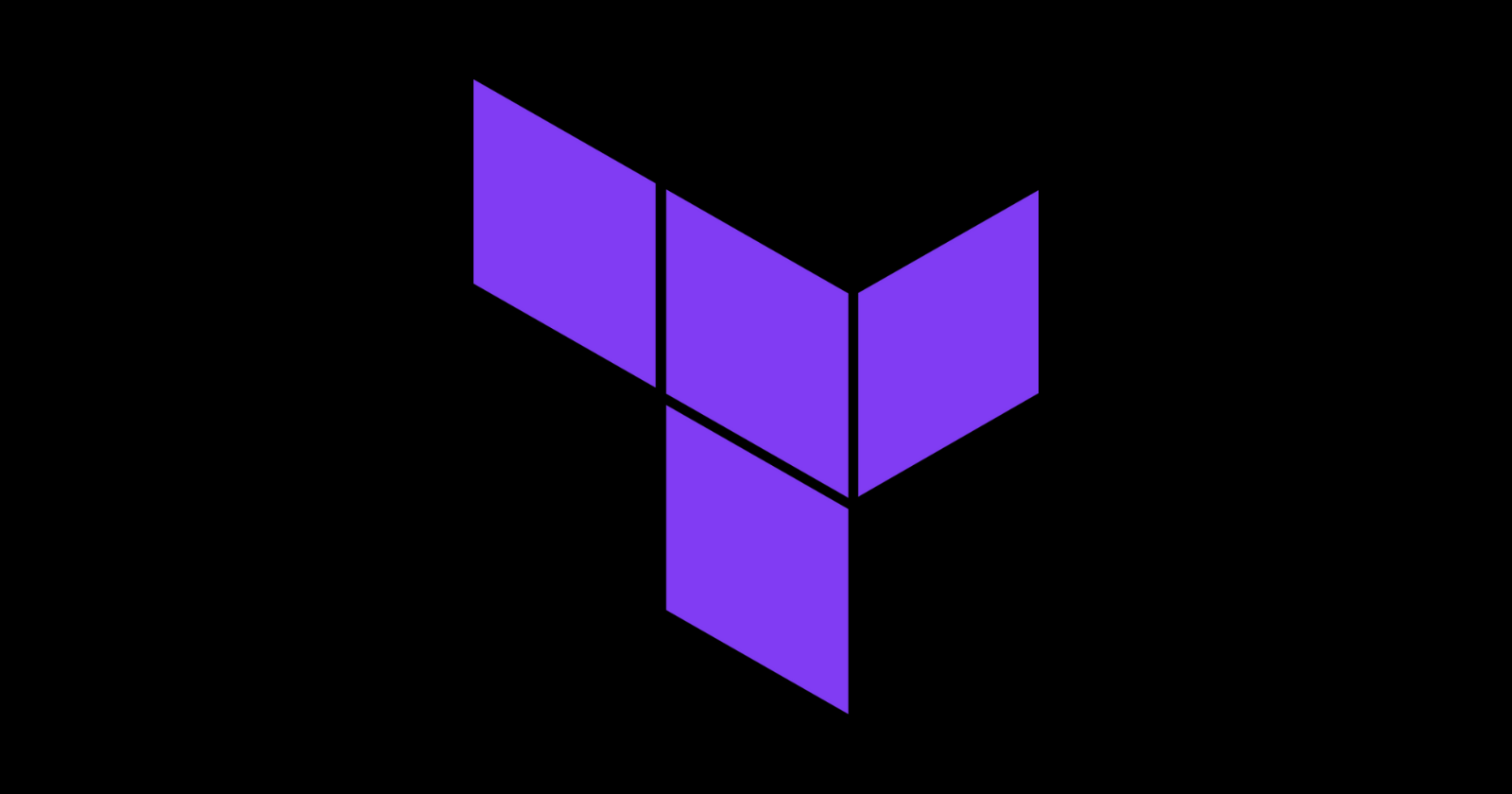
Terraform is a powerful infrastructure-as-code tool that not only provisions and manages resources but also offers a wide range of built-in functions to perform dynamic operations. These functions let you manipulate strings, numbers, collections, files, and even dates directly within your Terraform configurations. This dynamic capability enables more flexible, readable, and maintainable code—reducing the need for hardcoding values and streamlining your infrastructure management.
In this blog post, we’ll explore the different types of Terraform functions, provide practical examples for each category, and discuss best practices for using these functions effectively.
1. Types of Terraform Functions
Terraform functions fall into several broad categories, each serving a different purpose in your configuration:
Function Type | Example Functions |
String Functions | format() , join() , split() , replace() |
Numeric Functions | min() , max() , abs() , ceil() , floor() |
Collection Functions | length() , contains() , merge() , concat() |
Filesystem Functions | file() , templatefile() |
Encoding Functions | base64encode() , base64decode() |
Type Conversion | tostring() , tolist() , tomap() |
Date and Time | timestamp() , formatdate() |
Each of these function categories allows you to manipulate different data types, making your configurations more dynamic and adaptable.
2. String Manipulation
a) Dynamic String Formatting
Terraform’s format()
function helps create strings dynamically by inserting variables or computed values into a template.
output "formatted_string" {
value = format("Hello, %s! You are %d years old.", "John", 30)
}
Output:
Hello, John! You are 30 years old.
b) Concatenating Strings
You can join multiple strings using the join()
function, which is especially useful for constructing resource names or identifiers.
output "combined_string" {
value = join("-", ["aws", "us-east-1", "prod"])
}
Output:
aws-us-east-1-prod
3. Numeric Operations
Terraform’s numeric functions allow you to perform arithmetic operations and rounding tasks dynamically.
a) Finding Maximum or Minimum Values
Using max()
, you can easily retrieve the highest value from a set of numbers:
output "max_value" {
value = max(10, 20, 5)
}
Output:
20
b) Rounding Numbers
The ceil()
function rounds a number up to the nearest whole number:
output "rounded_value" {
value = ceil(4.3)
}
Output:
5
4. Working with Lists and Maps
Dynamic operations on collections are essential when managing multiple resources.
a) List Length
Determine how many elements a list contains using the length()
function:
variable "instance_types" {
default = ["t2.micro", "t3.medium", "t3.large"]
}
output "num_instance_types" {
value = length(var.instance_types)
}
Output:
3
b) Check for a Value in a List
The contains()
function verifies whether a particular value exists within a list:
output "contains_value" {
value = contains(["t2.micro", "t3.medium"], "t3.medium")
}
Output:
true
c) Merging Maps
Combine multiple maps into one using the merge()
function. This is particularly useful when you need to consolidate configuration settings from various sources.
output "merged_map" {
value = merge(
{ env = "prod", region = "us-east-1" },
{ project = "terraform", owner = "admin" }
)
}
Output:
{
"env" = "prod"
"region" = "us-east-1"
"project" = "terraform"
"owner" = "admin"
}
5. File Operations
Terraform can also interact with files directly.
a) Reading File Content
The file()
function reads the content of a file and returns it as a string:
output "file_content" {
value = file("config.json")
}
This reads and outputs the content of config.json
.
b) Using a Template File
For generating dynamic configuration files, templatefile()
lets you inject variable values into a file template.
Imagine you have a file config.tmpl
with the following content:
server_name = ${server}
port = ${port}
You can process it with:
output "template_output" {
value = templatefile("config.tmpl", {
server = "example.com"
port = "8080"
})
}
This generates a configuration with the specified server name and port.
6. Date and Time Functions
Working with date and time in Terraform is made simple with built-in functions.
a) Current Timestamp
The timestamp()
function returns the current date and time in ISO 8601 format:
output "current_timestamp" {
value = timestamp()
}
Output (example):
2024-01-30T12:34:56Z
7. Using Functions in Resource Configurations
Dynamic naming is a common requirement in resource configurations. For example, generating a bucket name based on environment variables:
resource "aws_s3_bucket" "example" {
bucket = format("my-bucket-%s", lower(var.env))
}
If var.env = "PROD"
, the bucket name will be:
my-bucket-prod
This dynamic operation ensures consistency and helps avoid naming collisions across environments.
8. Best Practices for Using Terraform Functions
Make Configurations Dynamic:
Use functions to generate values dynamically rather than hardcoding them. This increases flexibility and reusability.Test with
terraform console
:
Leverage the Terraform console to test functions interactively. This practice helps you understand function outputs before integrating them into your configurations.Utilize Collections:
Employ list and map functions for better resource management, especially when dealing with multiple resources or complex configurations.String Functions:
Functions likeformat()
,join()
, andreplace()
are indispensable for dynamic string generation. Use them to ensure your resource names and identifiers are consistent.Combine Functions:
Don’t hesitate to combine multiple functions to solve more complex problems. For example, you might merge maps and then convert them to strings for logging purposes.
Conclusion
Terraform's built-in functions are a robust toolkit for performing dynamic operations on your infrastructure data. They empower you to manipulate strings, perform arithmetic, handle collections, work with files, and manage dates and times—all directly within your configuration files. By leveraging these functions, you can create flexible, maintainable, and dynamic infrastructure setups that adapt to changing requirements without manual intervention.
Happy coding and automating with Terraform!
Reference
Terraform Built-in Functions Documentation
https://www.terraform.io/language/functions
Comprehensive documentation on all Terraform functions, including string, numeric, collection, file, encoding, and date operations.Terraform Language: Expressions
https://www.terraform.io/language/expressions
Detailed guidance on using expressions and functions to manipulate and dynamically generate values in Terraform configurations.Terraform CLI Console
https://www.terraform.io/cli/commands/console
Learn how to interactively test Terraform functions and expressions using the terraform console command.Terraform Best Practices
https://learn.hashicorp.com/tutorials/terraform/best-practices
A HashiCorp Learn tutorial offering strategies for writing dynamic, maintainable, and secure Terraform configurations.Dynamic Infrastructure with Terraform
https://www.hashicorp.com/resources/terraform
Explore additional resources and case studies on leveraging Terraform for dynamic infrastructure provisioning and management.
Subscribe to my newsletter
Read articles from Chintan Boghara directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Chintan Boghara
Chintan Boghara
Exploring DevOps ♾️, Cloud Computing ☁️, DevSecOps 🔒, Site Reliability Engineering ⚙️, Platform Engineering 🛠️, Machine Learning Operations 🤖, and AIOps 🧠