Password Strength Analyzer with Real-Time Visual Feedback

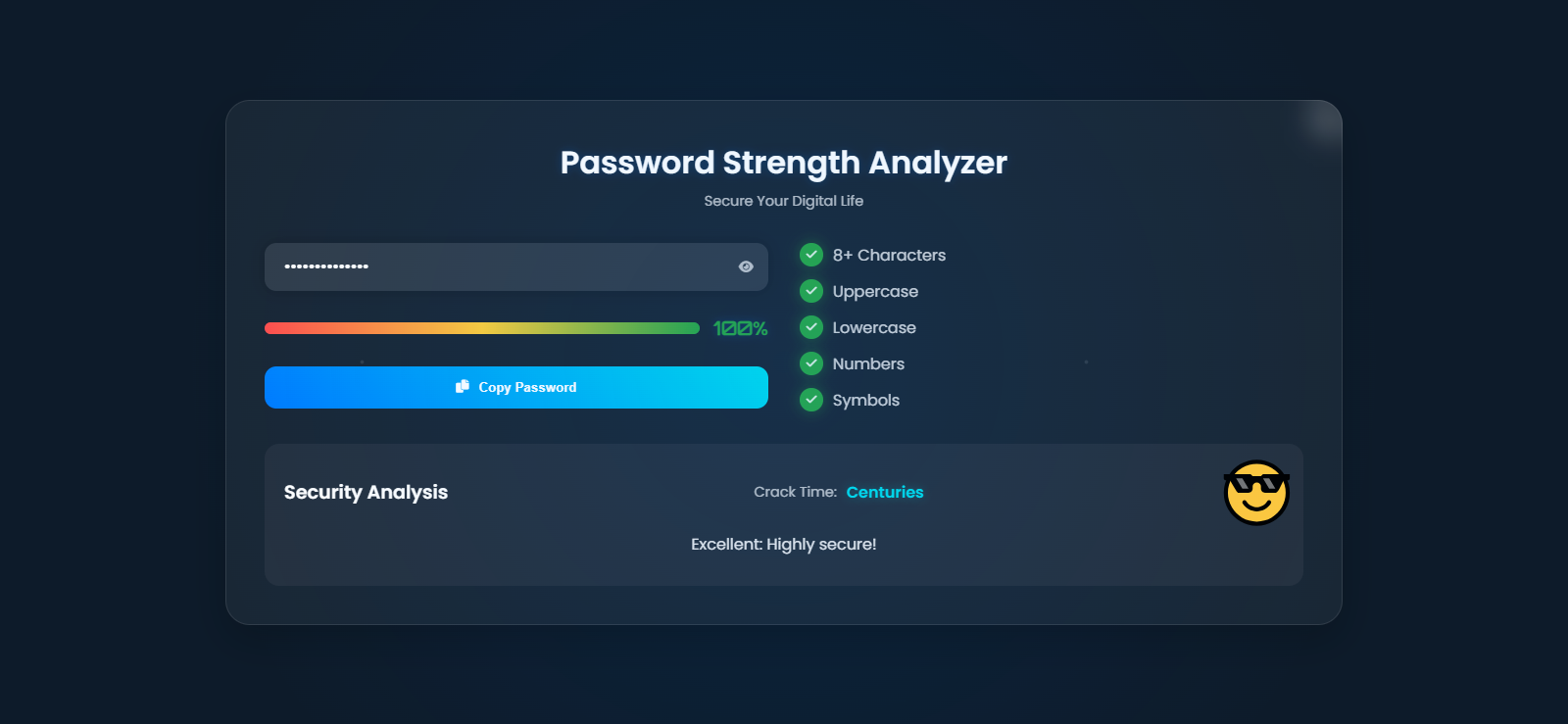
We all know the frustration of creating a new password. Too short? Not enough special characters? No uppercase letters? The requirements seem endless! But there's a good reason for this - weak passwords are like leaving your front door unlocked in a digital world filled with potential intruders.
Today, I'm excited to share a project I've been working on: a Password Strength Analyzer that provides real-time visual feedback. This tool not only checks how strong your password is but also gives you tips to improve it and even tells you if your password has been compromised in data breaches.
The Live Demo
Before we get into the code, feel free to check out the live demo here: Password Strength Checker
What Makes This Project Special
This isn't just another password checker. Here's what makes our analyzer stand out:
๐ Real-time strength analysis with a colorful progress bar
๐ Detailed criteria breakdown showing exactly what your password needs
โ ๏ธ Data breach checking using the "Have I Been Pwned" API
โฑ๏ธ Crack time estimation showing how quickly a hacker might crack your password
๐ Dark/light mode for comfortable viewing any time of day
๐ซ Modern UI with animations that make security checking actually enjoyable
Let's dive into how I built this tool and how it works!
Building Our Password Analyzer
The HTML Structure
Our application has a clean, intuitive layout divided into three main panels:
Left Panel: Contains the password input field and strength meter
Right Panel: Shows the criteria breakdown (length, uppercase, lowercase, etc.)
Feedback Panel: Provides security analysis and estimated crack time
The HTML structure creates a card-based layout with a glowing header and animated background effects.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Password Strength Analyzer</title>
<link rel="stylesheet" href="styles.css">
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/6.0.0/css/all.min.css">
<link href="https://fonts.googleapis.com/css2?family=Poppins:wght@400;600&family=Orbitron&display=swap" rel="stylesheet">
</head>
<body>
<div class="container">
<div class="theme-toggle">
<i class="fas fa-moon"></i>
</div>
<div class="card">
<div class="header-glow">
<h1>Password Strength Analyzer</h1>
<div class="subtitle">Secure Your Digital Life</div>
</div>
<div class="main-content">
<div class="left-panel">
<div class="input-container">
<input type="password" id="password" placeholder="Enter your password" aria-label="Password input">
<button class="toggle-visibility" aria-label="Toggle password visibility">
<i class="fas fa-eye"></i>
</button>
</div>
<div class="strength-meter">
<div class="progress-container">
<div class="progress-bar" id="strength-bar">
<div class="glow-effect"></div>
</div>
<div class="strength-particles"></div>
</div>
<span class="strength-score" id="strength-score">
<span class="score-number">0</span>%
</span>
</div>
<button class="copy-btn" id="copy-btn">
<i class="fas fa-copy"></i>
<span>Copy Password</span>
</button>
</div>
<div class="right-panel">
<div class="complexity-breakdown">
<div class="criteria" data-criteria="length">
<span class="check"><i class="fas fa-check"></i></span>
<span class="criteria-text">8+ Characters</span>
<span class="tooltip">Minimum length for good security</span>
</div>
<div class="criteria" data-criteria="uppercase">
<span class="check"><i class="fas fa-check"></i></span>
<span class="criteria-text">Uppercase</span>
<span class="tooltip">A-Z characters</span>
</div>
<div class="criteria" data-criteria="lowercase">
<span class="check"><i class="fas fa-check"></i></span>
<span class="criteria-text">Lowercase</span>
<span class="tooltip">a-z characters</span>
</div>
<div class="criteria" data-criteria="numbers">
<span class="check"><i class="fas fa-check"></i></span>
<span class="criteria-text">Numbers</span>
<span class="tooltip">0-9 digits</span>
</div>
<div class="criteria" data-criteria="symbols">
<span class="check"><i class="fas fa-check"></i></span>
<span class="criteria-text">Symbols</span>
<span class="tooltip">!@#$%^&* etc.</span>
</div>
</div>
</div>
<div class="feedback-panel" id="feedback">
<div class="feedback-header">
<h3>Security Analysis</h3>
<div class="crack-time-container">
<span class="crack-time-label">Crack Time:</span>
<p id="crack-time"><span>Instant</span></p>
</div>
<span id="emoji-feedback">๐</span>
</div>
<p id="feedback-text">Start typing to analyze</p>
</div>
</div>
</div>
<div class="background-effects">
<div class="particle-layer"></div>
<div class="gradient-overlay"></div>
</div>
</div>
<script src="script.js"></script>
</body>
</html>
Styling with CSS
The styling creates a modern, professional look with a dark theme by default. I've added several interactive elements:
Animated progress bar that changes color based on password strength
Glowing effects on interactive elements
Tooltips that appear when hovering over criteria
Responsive design that works on mobile and desktop
Particle background for visual interest
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
body {
font-family: 'Poppins', sans-serif;
background: #0d1b2a;
min-height: 100vh;
display: flex;
justify-content: center;
align-items: center;
overflow-y: auto;
transition: all 0.5s ease;
}
body.dark {
background: #1a1a2e;
}
.container {
position: relative;
padding: 30px;
z-index: 1;
width: 100%;
max-width: 1200px;
}
.card {
background: rgba(255, 255, 255, 0.05);
border-radius: 25px;
padding: 2.5rem;
width: 100%;
box-shadow: 0 15px 40px rgba(0, 0, 0, 0.2), inset 0 0 0 1px rgba(255, 255, 255, 0.1);
backdrop-filter: blur(15px);
position: relative;
overflow: hidden;
display: flex;
flex-direction: column;
min-height: 0;
}
.header-glow {
text-align: center;
margin-bottom: 2rem;
position: relative;
}
h1 {
color: #fff;
font-size: 2rem;
font-weight: 600;
text-shadow: 0 0 10px rgba(0, 123, 255, 0.3);
}
.subtitle {
color: rgba(255, 255, 255, 0.7);
font-size: 0.9rem;
margin-top: 5px;
}
.main-content {
display: flex;
gap: 2rem;
flex-wrap: wrap;
}
.left-panel, .right-panel {
flex: 1;
min-width: 300px;
}
.input-container {
position: relative;
margin-bottom: 1.5rem;
}
#password {
width: 100%;
padding: 14px 50px 14px 20px;
border: none;
border-radius: 12px;
background: rgba(255, 255, 255, 0.1);
color: #fff;
font-size: 1.1rem;
transition: all 0.3s ease;
box-shadow: 0 0 15px rgba(0, 0, 0, 0.2);
}
#password:focus {
outline: none;
background: rgba(255, 255, 255, 0.15);
box-shadow: 0 0 20px rgba(0, 123, 255, 0.3);
}
.toggle-visibility {
position: absolute;
right: 15px;
top: 50%;
transform: translateY(-50%);
background: none;
border: none;
cursor: pointer;
color: rgba(255, 255, 255, 0.7);
transition: color 0.3s ease;
}
.toggle-visibility:hover {
color: #fff;
}
.strength-meter {
display: flex;
align-items: center;
gap: 15px;
margin-bottom: 1.5rem;
}
.progress-container {
flex: 1;
position: relative;
}
.progress-bar {
height: 12px;
background: rgba(255, 255, 255, 0.1);
border-radius: 6px;
overflow: hidden;
position: relative;
}
.progress-bar::after {
content: '';
position: absolute;
height: 100%;
width: var(--strength, 0%);
background: linear-gradient(90deg, #ff4d4d, #ffcd39, #28a745);
transition: width 0.6s ease;
}
.glow-effect {
position: absolute;
top: -50%;
left: 0;
width: 100%;
height: 200%;
background: linear-gradient(transparent, rgba(255, 255, 255, 0.2), transparent);
transform: rotate(30deg);
animation: glow 3s infinite;
}
.strength-score {
font-size: 1.2rem;
font-weight: 700;
color: #fff;
text-shadow: 0 0 10px rgba(0, 123, 255, 0.3);
}
.score-number {
font-family: 'Orbitron', sans-serif;
}
.complexity-breakdown {
display: flex;
flex-direction: column;
gap: 12px;
}
.criteria {
display: flex;
align-items: center;
gap: 10px;
position: relative;
color: rgba(255, 255, 255, 0.8);
transition: all 0.3s ease;
}
.criteria:hover {
transform: translateX(5px);
}
.check {
width: 24px;
height: 24px;
border-radius: 50%;
background: rgba(255, 255, 255, 0.1);
display: flex;
align-items: center;
justify-content: center;
font-size: 12px;
color: transparent;
transition: all 0.4s ease;
position: relative;
}
.check.active {
background: #28a745;
color: #fff;
box-shadow: 0 0 15px rgba(40, 167, 69, 0.5);
}
.check.active::after {
content: '';
position: absolute;
width: 100%;
height: 100%;
border-radius: 50%;
background: rgba(40, 167, 69, 0.3);
animation: pulse 2s infinite;
}
.tooltip {
position: absolute;
background: rgba(0, 0, 0, 0.9);
color: #fff;
padding: 8px 12px;
border-radius: 8px;
font-size: 0.9rem;
top: -40px;
left: 50%;
transform: translateX(-50%);
opacity: 0;
pointer-events: none;
transition: all 0.3s ease;
}
.criteria:hover .tooltip {
opacity: 1;
top: -50px;
}
.feedback-panel {
background: rgba(255, 255, 255, 0.05);
padding: 20px;
border-radius: 15px;
position: relative;
overflow: hidden;
flex-grow: 1;
width: 100%;
}
.feedback-header {
display: flex;
justify-content: space-between;
align-items: center;
margin-bottom: 10px;
}
h3 {
color: #fff;
font-size: 1.2rem;
}
#emoji-feedback {
font-size: 2.5rem;
transition: transform 0.3s ease;
}
#feedback-text {
color: rgba(255, 255, 255, 0.9);
margin-bottom: 10px;
text-align: center;
}
.crack-time-container {
display: flex;
align-items: center;
gap: 10px;
}
.crack-time-label {
color: rgba(255, 255, 255, 0.7);
font-size: 0.9rem;
}
#crack-time span {
font-weight: 600;
color: #00ddeb;
text-shadow: 0 0 10px rgba(0, 221, 235, 0.3);
}
.copy-btn {
width: 100%;
padding: 14px;
background: linear-gradient(45deg, #007bff, #00ddeb);
color: #fff;
border: none;
border-radius: 12px;
cursor: pointer;
font-weight: 600;
display: flex;
align-items: center;
justify-content: center;
gap: 10px;
transition: all 0.3s ease;
position: relative;
overflow: hidden;
}
.copy-btn:hover {
transform: translateY(-2px);
box-shadow: 0 5px 20px rgba(0, 123, 255, 0.4);
}
.copy-btn::after {
content: '';
position: absolute;
width: 200%;
height: 200%;
background: rgba(255, 255, 255, 0.1);
transform: rotate(30deg);
top: -50%;
left: -50%;
animation: shine 4s infinite;
}
.theme-toggle {
position: absolute;
top: 30px;
right: 30px;
cursor: pointer;
font-size: 1.8rem;
color: rgba(255, 255, 255, 0.7);
transition: all 0.3s ease;
}
.theme-toggle:hover {
color: #fff;
transform: rotate(180deg);
}
.background-effects {
position: fixed;
top: 0;
left: 0;
width: 100%;
height: 100%;
pointer-events: none;
}
.particle-layer {
position: absolute;
width: 100%;
height: 100%;
background: url('data:image/svg+xml,%3Csvg xmlns="http://www.w3.org/2000/svg" viewBox="0 0 800 800"%3E%3Ccircle fill="rgba(255,255,255,0.1)" cx="400" cy="400" r="2"/%3E%3C/svg%3E') repeat;
animation: float 20s infinite linear;
}
.gradient-overlay {
position: absolute;
width: 100%;
height: 100%;
background: radial-gradient(circle at center, rgba(0, 123, 255, 0.1) 0%, transparent 70%);
animation: pulse 10s infinite;
}
@keyframes glow {
0%, 100% { transform: translateX(-100%) rotate(30deg); }
50% { transform: translateX(100%) rotate(30deg); }
}
@keyframes pulse {
0%, 100% { opacity: 0.5; }
50% { opacity: 1; }
}
@keyframes shine {
0% { transform: translateX(-100%) rotate(30deg); }
100% { transform: translateX(100%) rotate(30deg); }
}
@keyframes float {
0% { background-position: 0 0; }
100% { background-position: 100px 100px; }
}
@media (max-width: 768px) {
.main-content {
flex-direction: column;
}
.left-panel, .right-panel {
width: 50%%;
}
.card {
padding: 1.5rem;
}
}
@media (max-height: 600px) {
.card {
max-height: 100vh;
overflow-y: auto;
}
}
The JavaScript Logic
This is where the magic happens! Our JavaScript handles:
Password strength calculation based on multiple criteria
API integration with "Have I Been Pwned" to check for compromised passwords
Real-time UI updates as the user types
Copy functionality for the password field
Theme toggling between light and dark modes
Let's break down some of the key functions:
document.addEventListener('DOMContentLoaded', () => {
const passwordInput = document.getElementById('password');
const strengthBar = document.getElementById('strength-bar');
const strengthScore = document.getElementById('strength-score');
const scoreNumber = strengthScore.querySelector('.score-number');
const feedbackText = document.getElementById('feedback-text');
const emojiFeedback = document.getElementById('emoji-feedback');
const crackTime = document.getElementById('crack-time').querySelector('span');
const toggleVisibility = document.querySelector('.toggle-visibility');
const copyBtn = document.getElementById('copy-btn');
const themeToggle = document.querySelector('.theme-toggle');
const checks = document.querySelectorAll('.check');
const commonPasswords = ['password123', '123456', 'qwerty', 'admin'];
// Function to calculate SHA-1 hash (using subtle crypto)
async function sha1(str) {
const buffer = new TextEncoder().encode(str);
const hashBuffer = await crypto.subtle.digest('SHA-1', buffer);
const hashArray = Array.from(new Uint8Array(hashBuffer));
return hashArray.map(b => b.toString(16).padStart(2, '0')).join('');
}
// Check if password has been pwned using HIBP API
async function checkPwned(password) {
if (!password) return { pwned: false, count: 0 };
try {
const hash = await sha1(password);
const prefix = hash.slice(0, 5);
const suffix = hash.slice(5).toUpperCase();
const response = await fetch(`https://api.pwnedpasswords.com/range/${prefix}`, {
headers: { 'User-Agent': 'PasswordStrengthChecker' }
});
const text = await response.text();
const lines = text.split('\n');
for (const line of lines) {
const [hashSuffix, count] = line.split(':');
if (hashSuffix === suffix) {
return { pwned: true, count: parseInt(count, 10) };
}
}
return { pwned: false, count: 0 };
} catch (error) {
console.error('Error checking HIBP:', error);
return { pwned: false, count: 0, error: true };
}
}
function calculateStrength(password) {
let score = 0;
const criteria = {
length: password.length >= 8,
uppercase: /[A-Z]/.test(password),
lowercase: /[a-z]/.test(password),
numbers: /[0-9]/.test(password),
symbols: /[^A-Za-z0-9]/.test(password)
};
if (criteria.length) score += 20;
if (criteria.uppercase) score += 20;
if (criteria.lowercase) score += 20;
if (criteria.numbers) score += 20;
if (criteria.symbols) score += 20;
score += Math.min(20, password.length - 8) * 2;
if (commonPasswords.includes(password.toLowerCase())) score = Math.min(score, 20);
return Math.min(100, score);
}
function estimateCrackTime(score) {
if (score < 20) return 'Instant';
if (score < 40) return 'Seconds';
if (score < 60) return 'Minutes';
if (score < 80) return 'Hours';
if (score < 95) return 'Days';
return 'Centuries';
}
async function getFeedback(score, password) {
if (!password) return 'Start typing to analyze';
const pwnedResult = await checkPwned(password);
if (pwnedResult.error) return 'Error checking breach status';
if (pwnedResult.pwned) {
return `WARNING: This password was found in ${pwnedResult.count} breaches!`;
}
if (commonPasswords.includes(password.toLowerCase())) return 'Warning: Common password detected!';
if (score < 40) return 'Weak: Add more complexity';
if (score < 60) return 'Fair: Include diverse characters';
if (score < 80) return 'Good: Almost there!';
return 'Excellent: Highly secure!';
}
function getEmoji(score, pwned) {
if (pwned) return 'โ ๏ธ';
if (score < 20) return '๐';
if (score < 40) return '๐';
if (score < 60) return '๐';
if (score < 80) return '๐';
return '๐';
}
async function updateUI() {
const password = passwordInput.value;
const score = calculateStrength(password);
const pwnedResult = await checkPwned(password);
// Update strength bar and score
strengthBar.style.setProperty('--strength', `${score}%`);
scoreNumber.textContent = score;
strengthScore.style.color = pwnedResult.pwned ? '#ff4d4d' : score < 40 ? '#ff4d4d' : score < 80 ? '#ffcd39' : '#28a745';
// Update criteria checks
checks.forEach(check => {
const criterion = check.parentElement.dataset.criteria;
const isMet = {
length: password.length >= 8,
uppercase: /[A-Z]/.test(password),
lowercase: /[a-z]/.test(password),
numbers: /[0-9]/.test(password),
symbols: /[^A-Za-z0-9]/.test(password)
}[criterion];
check.classList.toggle('active', isMet);
});
// Update feedback
feedbackText.textContent = await getFeedback(score, password);
emojiFeedback.textContent = getEmoji(score, pwnedResult.pwned);
emojiFeedback.style.transform = `scale(${1 + score/200})`;
crackTime.textContent = estimateCrackTime(score);
// Flash warning if pwned
if (pwnedResult.pwned) {
feedbackText.style.color = '#ff4d4d';
feedbackText.style.fontWeight = 'bold';
} else {
feedbackText.style.color = 'rgba(255, 255, 255, 0.9)';
feedbackText.style.fontWeight = 'normal';
}
}
// Debounce function to limit API calls
function debounce(func, wait) {
let timeout;
return function executedFunction(...args) {
const later = () => {
clearTimeout(timeout);
func(...args);
};
clearTimeout(timeout);
timeout = setTimeout(later, wait);
};
}
// Event Listeners
const debouncedUpdateUI = debounce(updateUI, 500); // Debounce to 500ms
passwordInput.addEventListener('input', debouncedUpdateUI);
toggleVisibility.addEventListener('click', () => {
const type = passwordInput.type === 'password' ? 'text' : 'password';
passwordInput.type = type;
toggleVisibility.querySelector('i').classList.toggle('fa-eye');
toggleVisibility.querySelector('i').classList.toggle('fa-eye-slash');
});
copyBtn.addEventListener('click', () => {
navigator.clipboard.writeText(passwordInput.value);
copyBtn.querySelector('span').textContent = 'Copied!';
setTimeout(() => copyBtn.querySelector('span').textContent = 'Copy Password', 2000);
});
themeToggle.addEventListener('click', () => {
document.body.classList.toggle('dark');
themeToggle.querySelector('i').classList.toggle('fa-moon');
themeToggle.querySelector('i').classList.toggle('fa-sun');
});
// Initial UI update
updateUI();
});
// Custom property for strength bar animation
document.styleSheets[0].insertRule(`
.progress-bar::after {
width: var(--strength, 0%);
}
`, 0);
How the Password Strength Algorithm Works
Our algorithm considers several factors when determining password strength:
1. Basic Criteria Checking
Each of these criteria contributes 20 points to the overall score:
โ Length (8+ characters)
โ Uppercase letters
โ Lowercase letters
โ Numbers
โ Special symbols
2. Length Bonus
Beyond the minimum 8 characters, each additional character adds 2 points (up to a max of 20 bonus points). This encourages users to create longer passwords, which are inherently more secure.
3. Common Password Penalty
If the password matches a known common password (like "password123"), the score is capped at 20 regardless of other criteria. This discourages users from using passwords that are technically complex but widely known.
4. Data Breach Checking
The application uses the "Have I Been Pwned" API to check if a password has appeared in known data breaches. This is done securely by only sending the first 5 characters of the password's SHA-1 hash.
Here's how the API check works:
We generate a SHA-1 hash of the password
We send only the first 5 characters of the hash to the API
The API returns all matching hashes starting with those 5 characters
We check if our full hash is in the returned list, all within the user's browser
This "k-anonymity" approach ensures the full password is never sent over the network.
Security Considerations
When building a password tool, security is paramount. Here are some measures I've implemented:
All password analysis happens locally in the browser - passwords are never sent to my server
The HIBP API integration uses k-anonymity to check breached passwords without revealing them
The copy feature helps users securely transfer complex passwords
Tooltips provide educational content about password security
The UI/UX Design Process
I wanted to create an interface that makes security both informative and engaging. Some design decisions include:
Color psychology: Red for weak, yellow for medium, green for strong
Emoji feedback: Visual cues that anyone can understand at a glance
Animated elements: Drawing attention to important security information
Clean spacing: Ensuring readability and reducing cognitive load
Dark mode default: Easier on the eyes and creates a "security console" feel
Enhancing the User Experience
Small details make a big difference in user experience:
The strength bar animates smoothly rather than jumping between values
Criteria checks have a subtle pulse animation when activated
The copy button has a confirmation message that appears briefly
Feedback text changes color for warnings about compromised passwords
The theme toggle rotates when clicked
Future Enhancements
This project has room to grow! Here are some features I'm considering adding:
๐ Password generation functionality
๐ More detailed password analytics
๐ง Machine learning-based strength analysis
๐ Multi-language support
๐ฑ Native mobile applications
The Technical Challenges
Building this tool wasn't without challenges. Some interesting problems I solved:
Challenge 1: Debouncing API Calls
To prevent overwhelming the HIBP API with requests on every keystroke, I implemented a debounce function:
function debounce(func, wait) {
let timeout;
return function executedFunction(...args) {
const later = () => {
clearTimeout(timeout);
func(...args);
};
clearTimeout(timeout);
timeout = setTimeout(later, wait);
};
}
const debouncedUpdateUI = debounce(updateUI, 500);
This ensures the API is only called after the user stops typing for 500ms.
Challenge 2: Secure Password Checking
Checking if a password has been breached without sending the password is tricky. The solution uses the k-anonymity model:
async function checkPwned(password) {
if (!password) return { pwned: false, count: 0 };
try {
const hash = await sha1(password);
const prefix = hash.slice(0, 5);
const suffix = hash.slice(5).toUpperCase();
const response = await fetch(`https://api.pwnedpasswords.com/range/${prefix}`);
const text = await response.text();
const lines = text.split('\n');
for (const line of lines) {
const [hashSuffix, count] = line.split(':');
if (hashSuffix === suffix) {
return { pwned: true, count: parseInt(count, 10) };
}
}
return { pwned: false, count: 0 };
} catch (error) {
console.error('Error checking HIBP:', error);
return { pwned: false, count: 0, error: true };
}
}
Challenge 3: Creating Smooth Visual Transitions
Getting the progress bar to transition smoothly required a bit of CSS magic:
.progress-bar::after {
content: '';
position: absolute;
height: 100%;
width: var(--strength, 0%);
background: linear-gradient(90deg, #ff4d4d, #ffcd39, #28a745);
transition: width 0.6s ease;
}
Combined with JavaScript custom property updates:
strengthBar.style.setProperty('--strength', `${score}%`);
Conclusion
Password security doesn't have to be boring or confusing. With the right visual feedback and user experience, we can make security accessible and even enjoyable for everyone.
This Password Strength Analyzer demonstrates how thoughtful UI/UX design combined with solid security practices can create a tool that's both useful and engaging. Feel free to use it the next time you need to create a secure password!
What password strength tools do you use? Would you add any other features to this analyzer? Let me know in the comments below!
Resources and Further Reading
Subscribe to my newsletter
Read articles from Learn Computer Academy directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
