Finding and Removing Empty Files in a Directory

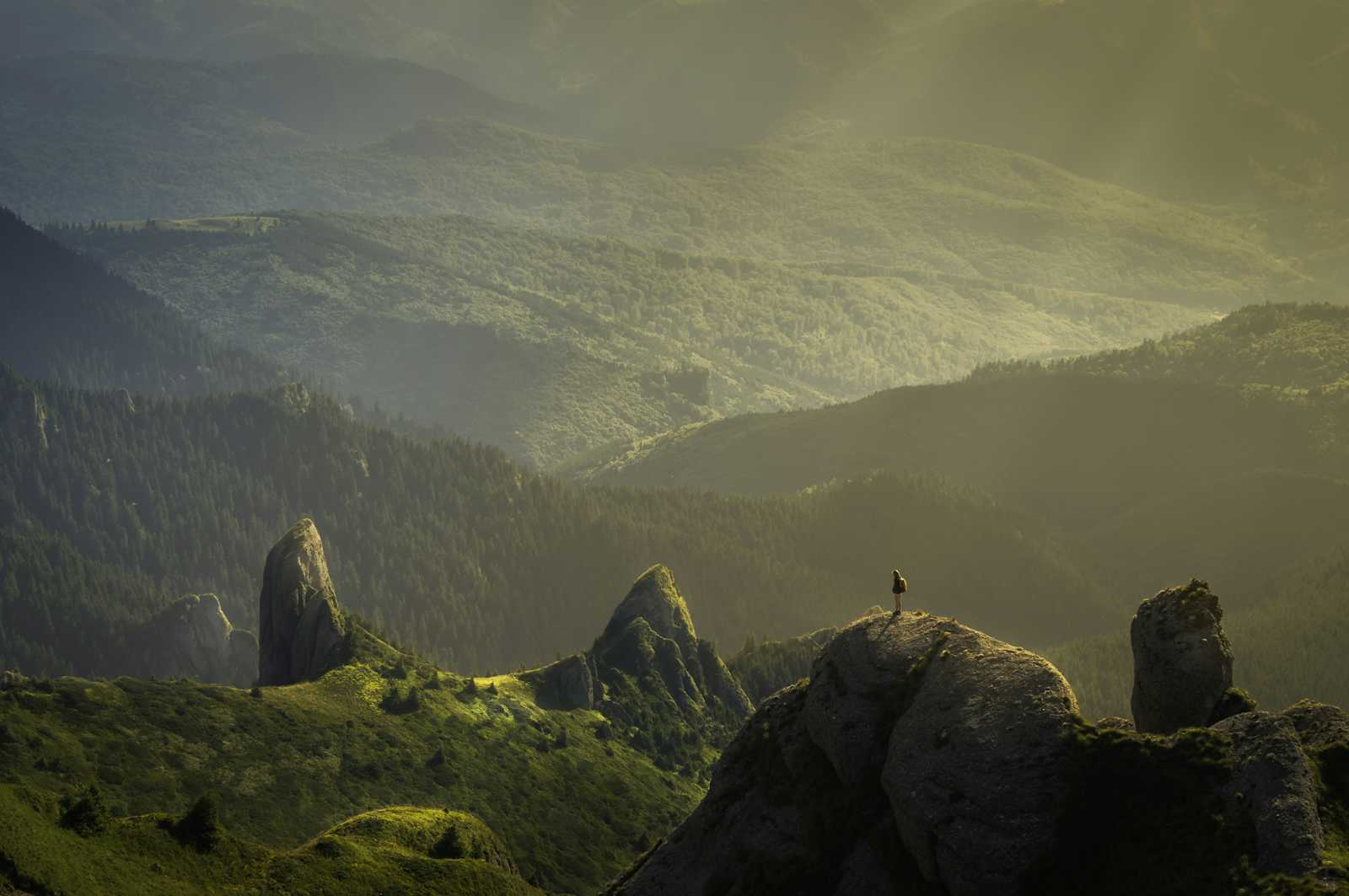
We all have created many files, forgotten about it and left it unattended. If you have run my Directory Structure Generator program uploaded earlier it has created many empty files which is not useful. This program solves this issue. This is a straightforward C program that locates and deletes empty files from a given directory. If a directory is not given, it will default to the current directory. This program is highly functional with several function calls, which is what makes it modular and readable.
How It Works
The program first checks whether the user has given a directory path or not. If not, it makes the path to "." (present working directory). Then it creates a Linux shell command using snprintf(). The command uses find to list empty files and rm to remove them. The program runs the command using system(), first listing the files before they are removed and listing them again after they are removed to indicate changes.
Why This Is Useful
Vacant files occupy unwanted space and fill directories. It is easier to clean up using this program, which automates the process. This is a minimal yet helpful program, and the fact that it involves several function calls makes it organized and effective.
#include <stdio.h>
#include <stdlib.h>
#include <sys/types.h>
#include <sys/stat.h>
#include <dirent.h>
#include <unistd.h>
#include <pwd.h>
#include <grp.h>
#include <time.h>
int main(int argc, char *argv[]) {
const char *dirpath = "."; // Default to current directory
// Check if a directory path is provided as an argument
if (argc > 1) {
dirpath = argv[1];
}
// Construct the Linux command to find and remove empty files
char command[1024];
char com[1024];
//places the command "find '%s' -type f -empty -exec rm -v {} +" in the char command.
// find '%s' of type file which is empty and remove -v=verbose {} + tells the code to
//go to the next file and complete the operation
snprintf(command, sizeof(command), "find '%s' -type f -empty -exec rm -v {} +", dirpath);
snprintf(com,sizeof(com),"ls '%s'",dirpath);
system(com);
// Execute the command
int ret = system(command);
system(com);
// Check if the command executed successfully
if (ret == -1) {
perror("Error executing command");
return EXIT_FAILURE;
}
return 0;
}
I know, by running my previous program Directory Structure Generator I am essentially creating a problem and this program solves it. But it does not hurt to try something new. Hope you learnt something new.
Happy Coding…
Subscribe to my newsletter
Read articles from Gagan G Saralaya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
