Jest enough testing to get started - part 1

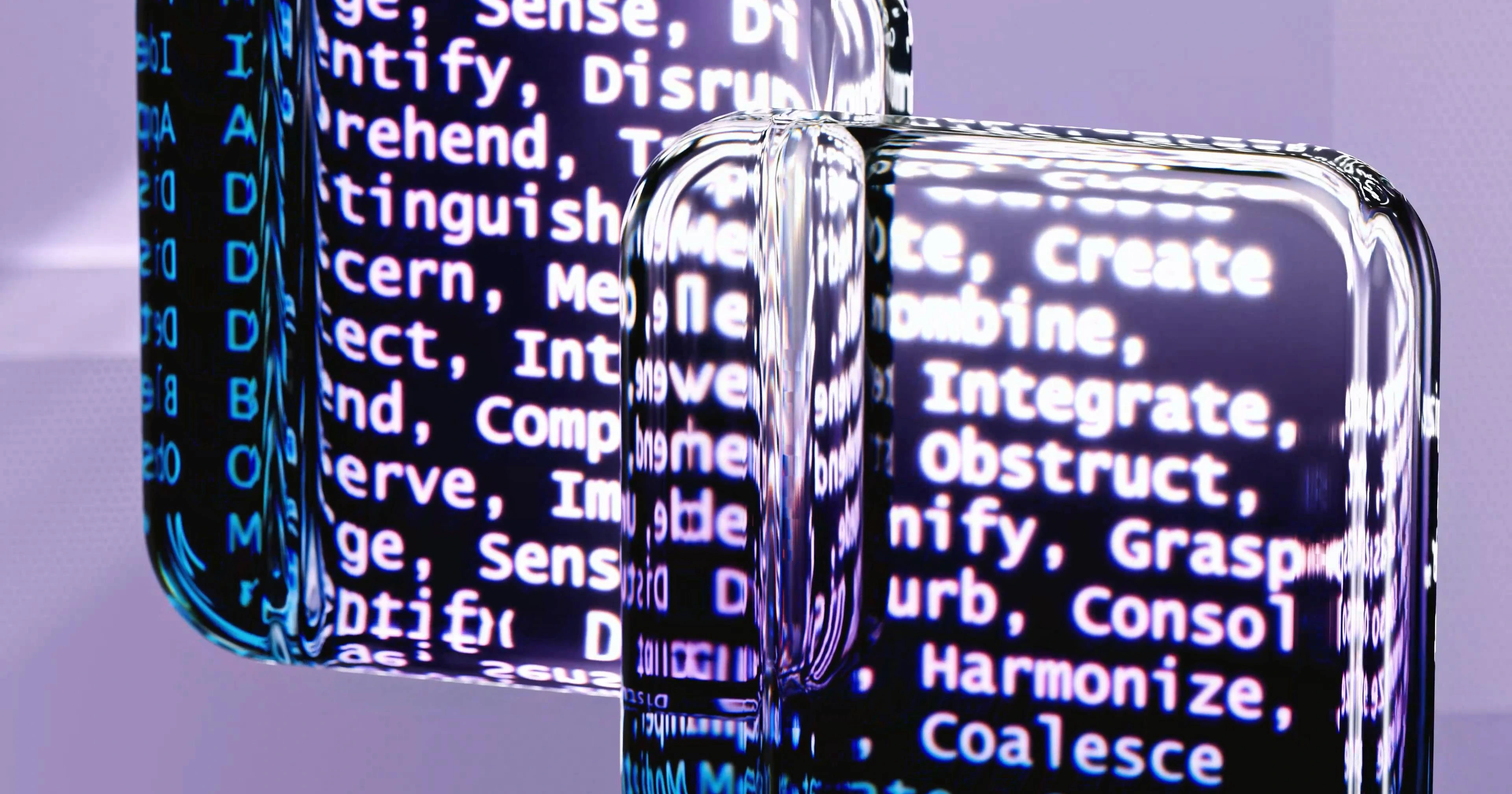
This article is a summary of namaste react's testing lesson so that whenever I need to refresh on this topic I can just run it by quickly. We will start everything from scratch so that we know what's going on behind the scenes. And yes, the title is a wordplay spun around the popular book - Just enough research.
Libraries used / needed
React Testing Library - A react wrapper around DOM testing library
Jest - Javascript Testing library which will work with RTL behind the scenes
If you are starting from scratch and let's we have parcel as our bundler which uses babel as a compiler, then we will require additional deps like
babel-jest @babel/core @babel/preset-env
jsdom which is testing environment used to simulate a bare-bones browser to parse your html. In the words from their readme: the goal of the project is to emulate enough of a subset of a web browser to be useful for testing and scraping real-world web applications.
@babel/preset-react
is used for our babel to convert jsx code to regular html for it to parse in the test cases@testing-library/jest-dom
makes your life easy by giving functions / jest-matchers like.toBeInTheDocument()
so that we don't have write spaghetti code to do something so simple.
I have listed down for two methods of installing them, one for a plain react.js app with parcel bundler and the other with clean slate Next.js
When using with parcel:
Installation of all deps:
npm i -D @testing-library/react jest babel-jest @babel/core @babel/preset-env jest-environment-jsdom @babel/preset-react @testing-library/jest-dom
Configure babel to use them:
//babel.config.js
module.exports = {
presets: [
['@babel/preset-env', {targets: {node: 'current'}}],
['@babel/preset-react', {runtime: "automatic"}],
],
};
Parcel and other bundlers will have a default configuration with babel which may cause a conflict with our new one, so we will have to setup a .parcelrc
(relavant stuff to your bundler) to disable default babel transpilation and handle the new requirements. Read more about this here https://nextjs.org/docs/pages/building-your-application/testing/jest
//.parcelrc
{
"extends": "@parcel/config-default",
"transformers": {
"*.{js,mjs,jsx,cjs,ts,tsx}": [
"@parcel/transformer-js",
"@parcel/transformer-react-refresh-wrap"
]
}
}
setting up the command for running jest in your package.json
:
{
"name": "testing-jest",
"version": "0.0.1",
"scripts": {
"test": "jest"
}
}
Now let's configure jest with
npx jest --init
It will give you options where we need to select
jsdom
for the test environment.babel
for coverageProvider
I have already included jest-environment-jsdom
in the initial command so no need to install it again. Jest version 28 and above does not include this library by default.
When using with turbopack (Next.js default template):
You can always refer the original docs for up to date process for installing and configuring jest here: https://nextjs.org/docs/pages/building-your-application/testing/jest but we shall go take the manual route cause we like the scenic route.
npm install -D jest jest-environment-jsdom @testing-library/react @testing-library/dom @testing-library/jest-dom ts-node
Now let's configure jest with
npm init jest@latest
Make sure you select jsdom
for the test environment and v8
for coverage provider. Either way, we are going to manually update the file cause we will be using nextJest
transformer which has all the necessary configuration options for Jest to work with Next.js:
import type { Config } from 'jest'
import nextJest from 'next/jest.js'
const createJestConfig = nextJest({
// Provide the path to your Next.js app to load next.config.js and .env files in your test environment
dir: './',
})
// Add any custom config to be passed to Jest
const config: Config = {
coverageProvider: 'v8',
testEnvironment: 'jsdom',
// Add more setup options before each test is run
// setupFilesAfterEnv: ['<rootDir>/jest.setup.ts'],
}
// createJestConfig is exported this way to ensure that next/jest can load the Next.js config which is async
export default createJestConfig(config)
(if you get stuck somewhere, go back to the docs for this process particularly as it can be updated based on newer versions in Next js)
if you run npm run test
and see no errors then congrats! we have successfully installed it all our deps.
Writing Tests
All our tests related files go under __tests__
folder under any sub-directories of your project and name your file as fileName.test.js
/ .spec.js
And now, how do we write a simple test case?
test("objective of the test", () => {
// actual implementation of the test
})
Just in case we are aware, running the above alone would still work and show as the test case as passed. So let's ask the test case to expect something
import {sum} from "../../lib/utils"
test("test sum function", () => {
const res = sum(3,3)
//Assertion
expect(res).toBe(6)
})
expect
is usually used to assert that something needs to be true, in this case, the sum of 3+3 has to be 6 so that we can verify our sum function is working as required.
How to test a react component then?
import { render, screen } from "@testing-library/react"
import '@testing-library/jest-dom'
import Page from '../page'
test("Check if heading is rendered", () => {
// render this component on the jsdom
render(<Page />)
// querying
const heading = screen.getByRole("heading")
// Assertion
expect(heading).toBeInTheDocument();
})
here's a flow of events of what's happening
the render() spins up the react component into a jsdom environment
then we get the first tag by the role of "heading" so it could be any from the h-tags family and put it into a
heading
const.and then we check if that exists.
How about for 2 heading tags? then we use .getAllByRole
which can be used to verify the exact number of buttons that needs to be used.
In the Next.js docs, they have given a slightly elaborate example
import '@testing-library/jest-dom'
import { render, screen } from '@testing-library/react'
import Page from '../app/page'
describe('Page', () => {
it('renders a heading', () => {
render(<Page />)
const heading = screen.getByRole('heading', { level: 1 })
expect(heading).toBeInTheDocument()
})
})
Here, describe
is just a way to group multiple test cases under a single umbrella. And it
is just an alias of test
. We can also see {level:1}
being used which asks specifically for h1 tag to be fetched on the screen.
import { render, screen } from "@testing-library/react"
import '@testing-library/jest-dom'
import Page from '../page'
describe('Home Page tests', () => {
it('renders a heading', () => {
render(<Page />)
const heading = screen.getByRole('heading', { level: 1 })
expect(heading).toBeInTheDocument()
})
it('should have 2 buttons', () => {
render(<Page />)
const buttons = screen.getAllByRole('button')
expect(buttons.length).toBe(2)
})
})
All of this, comes under the concept called Unit Testing, We test all these components in isolation and they are passed based on the parameters we have asserted. What happens when there is a redux store that needs to be used? Stay tuned for the next part cause it's already too long for a single blog, or go watch the course where you get better explanation for it. Ideally you should go read the docs but who does that in the age of AI amirite?
Part 2 will be linked here when I'm done completing it.
Credits:
Cover photo by Google DeepMind: https://www.pexels.com/photo/an-artist-s-illustration-of-artificial-intelligence-ai-this-illustration-depicts-language-models-which-generate-text-it-was-created-by-wes-cockx-as-part-of-the-visualising-ai-project-l-18069696/
Learning jest from Namaste React.
Drawing illustration made using excalidraw.
originally published on peerlist.
Subscribe to my newsletter
Read articles from Shreyas directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
