React Hook Form Best Practices: A Comprehensive Guide
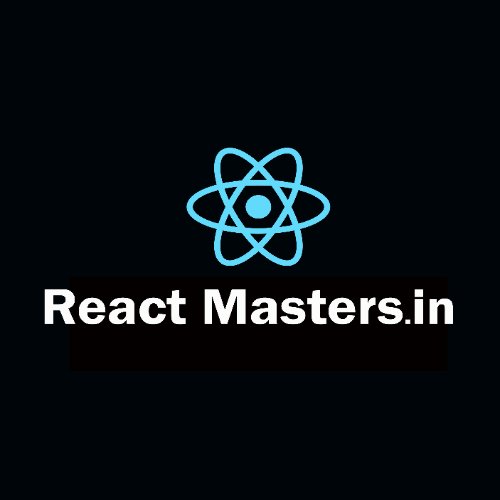
Introduction
Brief introduction to forms in React and the importance of efficient form handling.
Overview of React Hook Form and why it is popular among developers.
Target audience: Students and working professionals looking to optimize form handling in React applications.
1. What is React Hook Form?
Explanation of React Hook Form and its role in form management.
Comparison with traditional form handling approaches in React.
Benefits of using React Hook Form over controlled components and useState for form management.
2. Why Use React Hook Form?
Performance Optimization: Minimizing re-renders for better efficiency.
Ease of Validation: Built-in support for validation without extra re-renders.
Better User Experience: Faster form submissions and better error handling.
Reduced Boilerplate Code: Simplifies form handling compared to useState or Redux Form.
3. Setting Up React Hook Form in Your Project
Installation:
npm install react-hook-form
Basic example of setting up a form using
useForm()
.Integration with common UI libraries like Material-UI, Tailwind CSS, and Ant Design.
4. Understanding useForm Hook in React Hook Form
Explanation of
useForm()
hook and its key properties:register
handleSubmit
watch
reset
formState
5. What is Hook Form Controller?
Purpose of
Controller
in React Hook Form.When and why to use
Controller
instead ofregister
.Example usage with custom components like React-Select, DatePickers, and Input Masks.
6. Handling Validation in React Hook Form
Using built-in validation rules (
required
,minLength
,maxLength
,pattern
, etc.).Custom validation functions for complex validation needs.
Error handling using
formState.errors
and displaying error messages.Integrating third-party validation libraries like Yup or Zod.
7. Best Practices for React Hook Form
Keep Forms Minimal and Optimized: Avoid unnecessary re-renders.
Use Default Values Wisely: Prevent uncontrolled component errors.
Leverage Context API for Large Forms: Manage state efficiently.
Optimize Form Submission: Asynchronous form submission handling.
Ensure Proper Error Handling: User-friendly error messages.
Integrate Third-Party UI Libraries Effectively: Smooth component interaction.
8. Managing Dynamic Forms in React Hook Form
Handling dynamically generated form fields.
Using
useFieldArray
for dynamic input fields (e.g., adding and removing items).Optimizing performance with
key
attributes and controlled inputs.
9. Integrating React Hook Form with APIs and Fetch Requests
Submitting form data to a backend API.
Handling form submission with
fetch()
or Axios.Resetting form state after successful submission.
10. React Hook Form vs. Formik: Key Differences
Performance comparison between React Hook Form and Formik.
Key advantages of React Hook Form in terms of re-renders and validation.
When to choose Formik over React Hook Form.
11. Debugging and Troubleshooting Common Issues in React Hook Form
Common issues like uncontrolled components, form resets, and validation problems.
Debugging techniques using
react-hook-form-devtools
.Best practices to avoid performance bottlenecks.
12. Conclusion
Summary of key takeaways.
Why React Hook Form is a powerful tool for form handling in React applications.
Encouragement to explore more advanced features and experiment with React Hook Form in real-world applications.
Introduction
Forms are fundamental to any web application, allowing users to input data for various purposes like authentication, data collection, and communication. However, handling forms efficiently in React applications can be challenging due to state management and performance concerns.
React Hook Form is a lightweight, performant, and easy-to-use library for managing form state in React functional components. This guide explores the best practices of using React Hook Form, ensuring students and working professionals can build optimized and scalable forms with minimal re-renders.
By the end of this article, you will understand:
✅ Why React Hook Form is the preferred choice over other form-handling approaches.
✅ How to set up and optimize React Hook Form in real-world applications.
✅ The best practices to improve form performance and user experience.
1. What is React Hook Form?
React Hook Form is a library that simplifies form handling in React applications by reducing the amount of boilerplate code required. Unlike traditional form handling that relies on useState for each input field, React Hook Form uses uncontrolled components to optimize re-renders and improve performance.
Key Features of React Hook Form
✔️ Minimal re-renders for better efficiency.
✔️ Simple and intuitive API for handling form state.
✔️ Built-in validation support with custom error messages.
✔️ Integration with UI libraries like Material UI and Ant Design.
✔️ Supports dynamic and conditional form fields.
2. Why Use React Hook Form?
React Hook Form provides several advantages over traditional form-handling techniques like controlled components or Formik.
✅ Performance Optimization
React Hook Form leverages uncontrolled components, meaning form inputs are not re-rendered unnecessarily. This improves performance, especially in large forms.
✅ Simplified Form Handling
With useForm()
Developers can easily manage form state, handle validation, and submit data without manually tracking every input field.
✅ Flexible Validation System
React Hook Form supports both built-in and third-party validation methods like Yup or Zod, making it easy to enforce form validation rules.
✅ Less Boilerplate Code
Compared to Formik or controlled components, React Hook Form requires fewer lines of code, making form management simpler and more readable.
3. Setting Up React Hook Form in Your Project
To get started with React Hook Form, install it using npm or yarn:
bashCopyEditnpm install react-hook-form
# or
yarn add react-hook-form
Basic Form Setup
Import useForm()
and initialize the form:
jsxCopyEditimport { useForm } from "react-hook-form";
function MyForm() {
const { register, handleSubmit } = useForm();
const onSubmit = (data) => {
console.log(data);
};
return (
<form onSubmit={handleSubmit(onSubmit)}>
<input {...register("name")} placeholder="Enter your name" />
<button type="submit">Submit</button>
</form>
);
}
4. Understanding useForm Hook in React Hook Form
The useForm()
hook provides several important functions for managing forms:
Key Properties of useForm()
✔️ register – Registers input fields in the form.
✔️ handleSubmit – Handles form submission.
✔️ watch – Monitors field changes in real time.
✔️ reset – Resets the form fields.
✔️ formState – Manages validation errors and form submission state.
5. What is Hook Form Controller?
The Controller
component is used in React Hook Form when dealing with third-party UI components like Material UI, React-Select, or DatePicker.
Why Use Controller?
Some external components do not expose a ref
, making it impossible to use register
directly. Controller
acts as a bridge, allowing these components to work seamlessly with React Hook Form.
Example: Using Controller with React-Select
jsxCopyEditimport { Controller, useForm } from "react-hook-form";
import Select from "react-select";
function MyForm() {
const { control, handleSubmit } = useForm();
const onSubmit = (data) => console.log(data);
return (
<form onSubmit={handleSubmit(onSubmit)}>
<Controller
name="category"
control={control}
render={({ field }) => <Select {...field} options={[{ label: "Option 1", value: "1" }]} />}
/>
<button type="submit">Submit</button>
</form>
);
}
6. Handling Validation in React Hook Form
Validation is crucial in form handling to ensure users enter correct data. React Hook Form provides built-in validation methods and supports third-party libraries like Yup.
Built-in Validation Rules
✔️ required
– Field must not be empty.
✔️ minLength
/ maxLength
– Limits character count.
✔️ pattern
– Enforces a specific regex pattern.
Example: Applying Validation
jsxCopyEdit<input
{...register("email", {
required: "Email is required",
pattern: { value: /^\S+@\S+$/i, message: "Invalid email format" }
})}
/>
Third-Party Validation with Yup
jsxCopyEditimport { yupResolver } from "@hookform/resolvers/yup";
import * as yup from "yup";
const schema = yup.object().shape({
email: yup.string().email().required(),
});
const { register, handleSubmit } = useForm({ resolver: yupResolver(schema) });
7. Best Practices for React Hook Form
✅ Minimize Re-renders: Use uncontrolled components for optimal performance.
✅ Use Default Values: Prevent undefined errors by setting initial values.
✅ Leverage Context API for Large Forms: Manage form state efficiently.
✅ Ensure User-Friendly Validation Messages: Guide users with clear feedback.
✅ Optimize Form Submission: Use async handlers for API calls.
8. Managing Dynamic Forms in React Hook Form
Dynamic forms require adding and removing fields based on user actions.
✔️ Use useFieldArray()
to manage dynamic fields.
✔️ Optimize performance with key
attributes.
Example: Adding and Removing Fields Dynamically
jsxCopyEditimport { useForm, useFieldArray } from "react-hook-form";
function DynamicForm() {
const { register, control, handleSubmit } = useForm();
const { fields, append, remove } = useFieldArray({ control, name: "items" });
return (
<form>
{fields.map((field, index) => (
<div key={field.id}>
<input {...register(`items.${index}.name`)} />
<button type="button" onClick={() => remove(index)}>Remove</button>
</div>
))}
<button type="button" onClick={() => append({ name: "" })}>Add</button>
</form>
);
}
9. React Hook Form vs. Formik
Feature | React Hook Form | Formik |
Performance | ✅ High | ❌ Lower due to controlled components |
Validation | ✅ Built-in + Yup | ✅ Yup |
Ease of Use | ✅ Simple API | ❌ More boilerplate |
10. Conclusion
React Hook Form is a game-changer for managing forms in React applications. It offers better performance, simpler validation handling, and an optimized user experience compared to traditional methods.
By following best practices such as using useForm()
, leveraging Controller
for third-party UI components, and optimizing form validation, developers can create highly efficient and user-friendly forms.
Subscribe to my newsletter
Read articles from React Masters directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
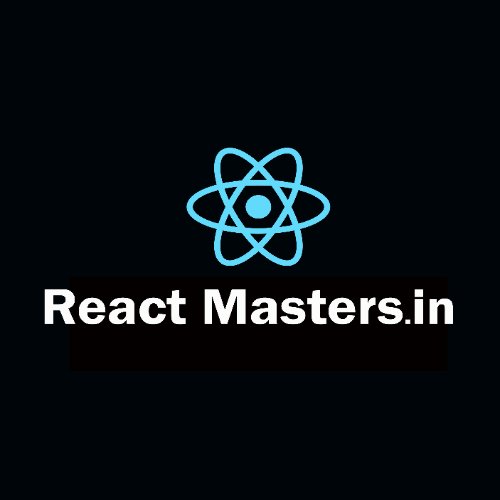
React Masters
React Masters
React Masters is one of the leading React JS training providers in Hyderabad. We strive to change the conventional training modes by bringing in a modern and forward program where our students get advanced training. We aim to empower the students with the knowledge of React JS and help them build their career in React JS development. React Masters is a professional React training institute that teaches the fundamentals of React in a way that can be applied to any web project. Industry experts with vast and varied knowledge in the industry give our React Js training. We have experienced React developers who will guide you through each aspect of React JS. You will be able to build real-time applications using React JS.