Deploying a Web Application on Kubernetes: From Container to Cluster.

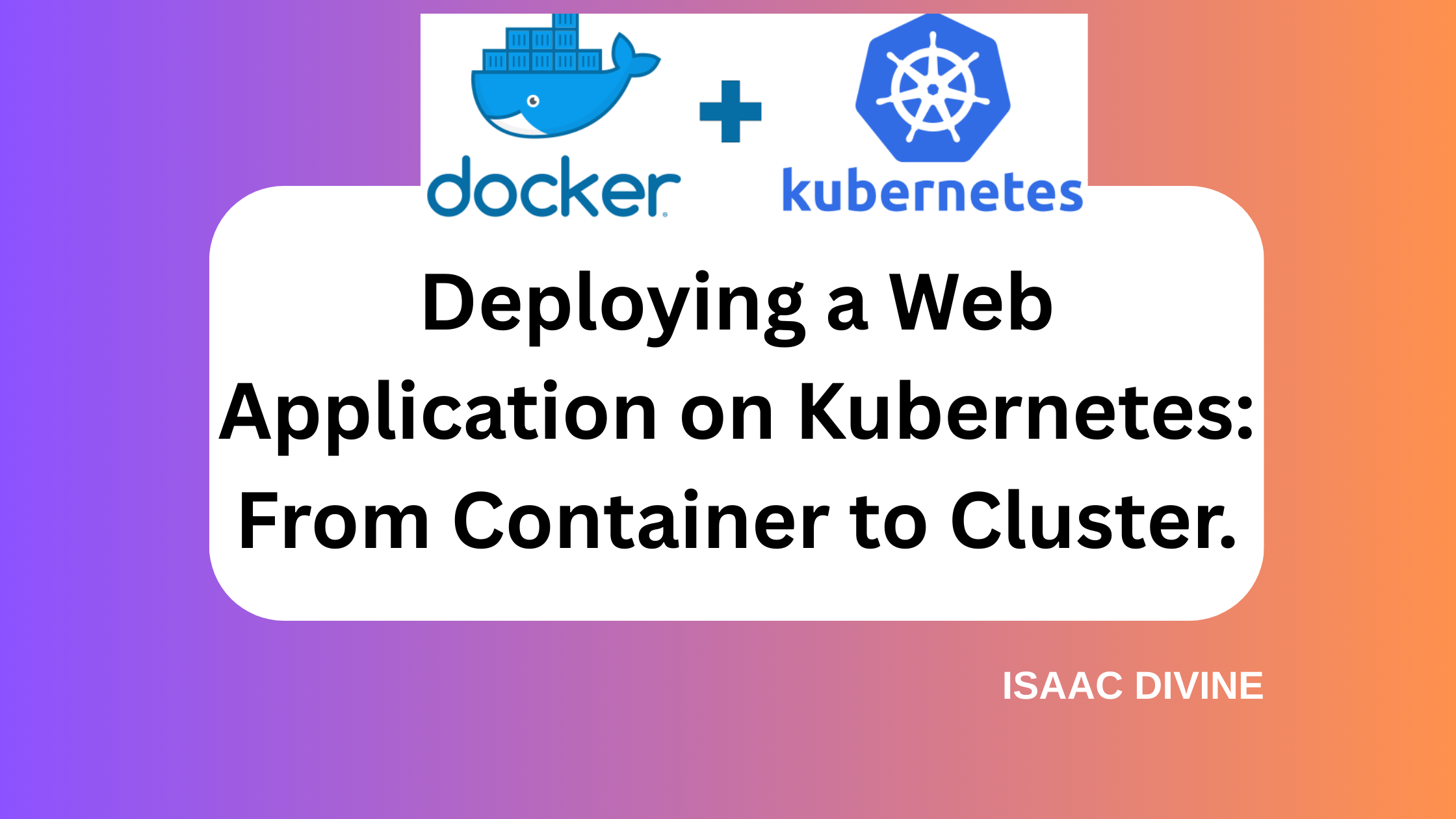
Kubernetes has become the go-to platform for deploying, managing, and scaling containerized applications. In this guide, we'll walk through the process of deploying a Python Flask web application on Kubernetes, covering everything from containerizing the app to running it inside a cluster. Let’s dive in!
STEP 1: Creating a Python Web Application
First, we need a simple web application to deploy. Below is a Flask app that returns a basic webpage.
No 1: Create app.py
from flask import Flask
app = Flask(__name__)
@app.route('/')
def home():
return "<h1>Welcome to My Flask App on Kubernetes by me Isaac Divine🚀</h1>"
if __name__ == '__main__':
app.run(host='0.0.0.0', port=5000)
No 2: Create requirements.txt
flask
STEP 2: Containerizing the Web Application
Before deploying to Kubernetes, we need to package our web application into a Docker container.
No 1: Create a Dockerfile
A Dockerfile defines how our container will be built.
# Use an official Python image as base
FROM python:3.9
# Set the working directory
WORKDIR /app
# Copy application files
COPY . .
# Install dependencies
RUN pip install -r requirements.txt
# Expose the port the app runs on
EXPOSE 5000
# Run the application
CMD ["python", "app.py"]
No 2: Build and push the Docker Image
Once the Dockerfile is ready, we build and push the image to a container registry.
# Build the Docker image
docker build -t myapp:latest .
# Tag the image (replace 'username' with your Docker Hub ID)
docker tag myapp:latest isaacdivine37/myapp:latest
# Push the image to Docker Hub
docker push isaacdivine37/myapp:latest
STEP 3: Setting Up Kubernetes ⛵
Now that we have our containerized application, it’s time to deploy it to Kubernetes.
No 1: Create Kubernetes YAML Files
We need two essential components:
Deployment.yaml (to define how our app runs in Kubernetes)
Service.yaml (to expose the app to the outside world)
Deployment.yaml (Manages the application pods)
apiVersion: apps/v1
kind: Deployment
metadata:
name: myapp-deployment
spec:
replicas: 2 # Run two instances of the app
selector:
matchLabels:
app: myapp
template:
metadata:
labels:
app: myapp
spec:
containers:
- name: myapp
image: username/myapp:latest #change the username to your dockerhub/ registry username
ports:
- containerPort: 5000
Service.yaml (Exposes the app externally)
apiVersion: v1
kind: Service
metadata:
name: myapp-service
spec:
selector:
app: myapp
ports:
- protocol: TCP
port: 80
targetPort: 5000
type: LoadBalancer # Change to ClusterIP for internal access
STEP 4: Deploying to Kubernetes
With our YAML files ready, it's time to deploy!
No 1: Apply the Kubernetes Configurations
first deploy the application yaml file
kubectl apply -f deployment.yaml # Deploy the application
Expose the application yaml file
kubectl apply -f service.yaml # Expose the application
No 2: Verify the Deployment
Check if the pods are running:
kubectl get pods
No 3: Get the External IP
kubectl get service myapp-service
Now, visit http://localhost:80 in your browser, and your web app should be live!
STEP 5: Scaling & Managing the Application
Need more instances? Scale up dynamically:
kubectl scale deployment myapp-deployment --replicas=5
Check again:
kubectl get pods
To monitor logs:
kubectl logs -f myapp-deployment-789fc9ccf8-585nz
STEP 6: Cleanup
When you're done testing, clean up:
kubectl delete -f service.yaml
kubectl delete -f deployment.yaml
Or delete everything at once:
kubectl delete all --all
Subscribe to my newsletter
Read articles from ISAAC DIVINE directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

ISAAC DIVINE
ISAAC DIVINE
🚀 Cloud Enthusiast | Turning ideas into scalable solutions ☁️ | Passionate about shaping the future of tech with cloud computing 🌐 | Always exploring the next big thing in the digital sky ✨"