๐ฆ Rust Series 1: From 0 to 1

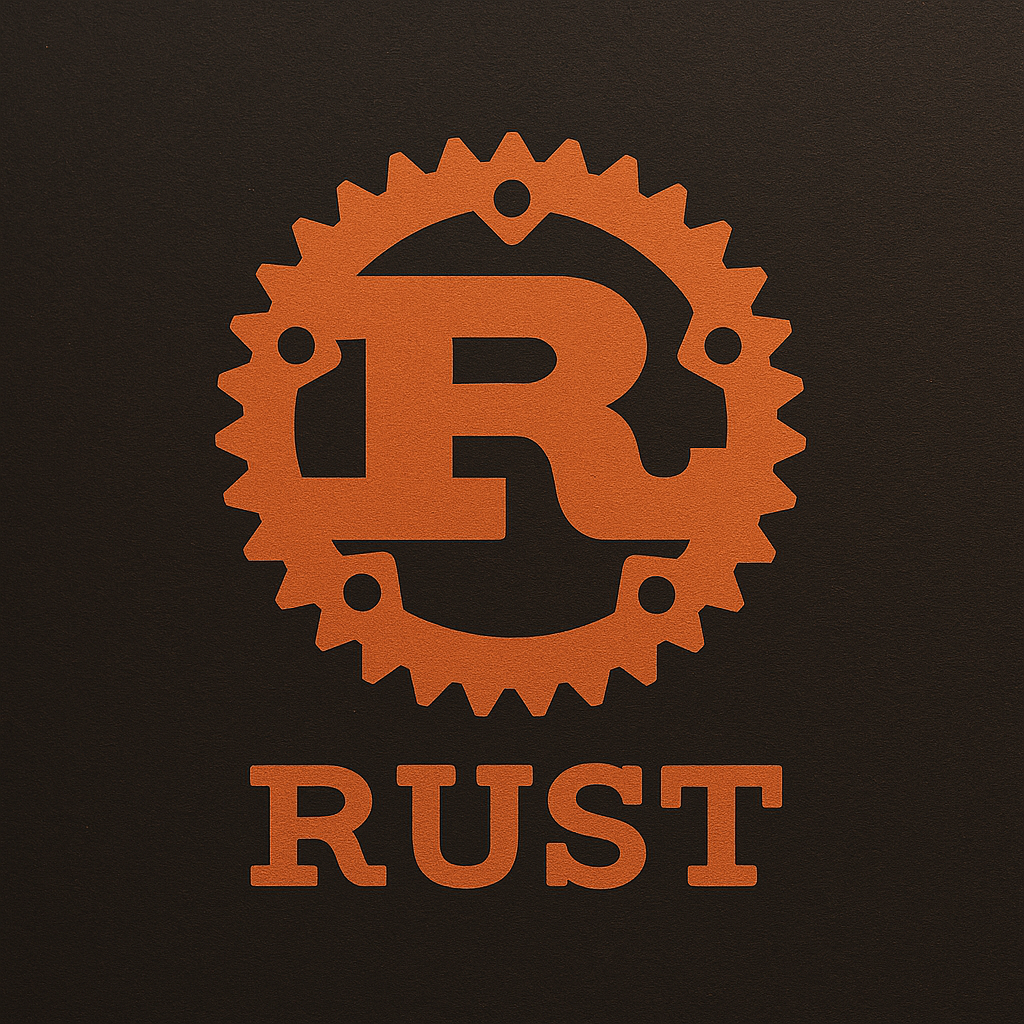
Welcome to Rust! In this series, we'll cover the basics of Rust programming, starting from installation and setup to writing and running your first program. We'll also cover key data types, variables, and basic operations with examples and edge cases.
โ 1. Installing Rust
๐ ๏ธ Install Rust using rustup
rustup
is the recommended way to install Rust as it manages updates and toolchains easily.
โก๏ธ Steps to install:
Open your terminal.
Run the following command:
curl --proto '=https' --tlsv1.2 -sSf https://sh.rustup.rs | sh
Follow the on-screen instructions.
It will install:
rustc
โ The Rust compilercargo
โ The Rust package managerrustup
โ The version manager
โ Verify Installation
After installation, restart your terminal and run:
rustc --version
cargo --version
Example output:
rustc 1.76.0 (f9e8851be 2025-03-01)
cargo 1.76.0 (f9e8851be 2025-03-01)
๐ 2. Setting Up Your First Rust Project
โก๏ธ Create a new Rust project
Use cargo
to create a new project:
cargo new hello-rust
This creates a directory hello-rust
with the following structure:
hello-rust
โโโ Cargo.toml // Project configuration file
โโโ src
โโโ main.rs // Main code file
๐ 3. Writing Your First Program
Open src/
main.rs
and write the following:
fn main() {
println!("Hello, Rust!");
}
โก๏ธ Run the program
Navigate to the project directory and run:
cargo run
โ Output:
Hello, Rust!
๐ข 4. Numbers in Rust
Rust has strict type-checking for numeric types:
โก๏ธ Signed Integers (i8
, i16
, i32
, i64
, i128
)
Signed integers can store negative values.
i32
is the default type for integers.
Example:
let x: i32 = -10;
let y: i32 = 20;
println!("Signed Integers: x = {}, y = {}", x, y);
โก๏ธ Unsigned Integers (u8
, u16
, u32
, u64
, u128
)
- Unsigned integers store only positive values.
Example:
let x: u32 = 10;
let y: u32 = 20;
println!("Unsigned Integers: x = {}, y = {}", x, y);
โก๏ธ Floating-Point Numbers (f32
, f64
)
f64
is the default type for floats.
Example:
let x: f32 = 10.5;
let y: f32 = 20.5;
println!("Floats: x = {}, y = {}", x, y);
โ Edge Cases
- Integer overflow example:
let x: u8 = 255;
let y = x + 1; // Causes overflow
println!("{}", y);
โก๏ธ Output: thread 'main' panicked at overflow
- Floating-point precision loss:
let x: f32 = 0.1 + 0.2;
println!("{}", x); // Output: 0.30000004
๐ฃ 5. Booleans in Rust
Rust uses true
and false
for booleans.
Example:
let is_true: bool = true;
let is_false: bool = false;
if is_true && !is_false {
println!("Both conditions are satisfied.");
} else {
println!("Conditions are not satisfied.");
}
โ Edge Cases:
- Boolean used in
if
expression:
let condition = 1 > 2;
if condition {
println!("True");
} else {
println!("False");
}
๐ท๏ธ 6. Variables in Rust
Variables are immutable by default.
Use
mut
to make them mutable.
Example:
let x = 10; // immutable
let mut y = 20; // mutable
y = 30; // OK since it's mutable
println!("x = {}, y = {}", x, y);
โ Edge Cases:
- Trying to modify an immutable variable:
let x = 10;
x = 20; // Compile-time error
- Shadowing example:
let x = 10;
let x = x + 20;
println!("{}", x); // Output: 30
๐ก 7. Strings in Rust
Rust has two types of strings:
String
โ Heap-allocated and growable&str
โ String slice (fixed size)
โก๏ธ Example of String
:
let greeting: String = String::from("Hello, Rust!");
println!("{}", greeting);
โก๏ธ Example of &str
:
let x: &str = "Hello";
let y: &str = "Rust";
println!("{} {}", x, y);
โ Edge Cases:
String
indexing is not allowed:
let s = String::from("hello");
println!("{}", s[0]); // Error: Rust strings are UTF-8
String
length:
let s = String::from("hello");
println!("{}", s.len()); // Output: 5
- Handling
Option<char>
when indexing:
let s = String::from("rust");
match s.chars().nth(2) {
Some(c) => println!("Third character: {}", c),
None => println!("No character at index"),
}
๐ช 8. Match Statement
Example:
let x = 5;
match x {
1 => println!("One"),
2 => println!("Two"),
3..=5 => println!("Three to Five"),
_ => println!("Other"),
}
โ Edge Cases:
- Missing cases:
let x = 7;
match x {
1 | 2 => println!("One or Two"),
_ => println!("Other"),
}
๐ 9. Functions in Rust
Example:
fn add(x: i32, y: i32) -> i32 {
x + y
}
fn main() {
let result = add(5, 10);
println!("Sum = {}", result);
}
โ Edge Cases:
- Mismatched return type:
fn add(x: i32, y: i32) -> i32 {
x + y;
} // Error due to missing return statement without `;`
๐ 10. Full Example
fn main() {
let x: i32 = 5;
let y: i32 = 10;
println!("Sum of {} and {} is {}", x, y, add(x, y));
}
fn add(a: i32, b: i32) -> i32 {
a + b
}
โก๏ธ Output:
Sum of 5 and 10 is 15
โก๏ธ Clone the repo:
git clone https://github.com/ichiragkumar/rust-basics.git
โก๏ธ Navigate to the project:
cd rust-basics
โก๏ธ Run the project:
cargo run
โก๏ธ Edit and Play Around:
Open src/main.rs
in your editor (like VSCode) and start modifying it:
โ
Add new functions
โ
Test edge cases
โ
Break things to see how Rust handles it
๐ฅ Start coding and share your progress! ๐
๐ Follow and Explore!
๐ Follow Chiragkumar on GitHub and X:
GitHub โ Star the repo and check out other cool projects! ๐
X (Twitter) โ Stay updated with Chiragkumar's coding journey! ๐งโ๐ป
Subscribe to my newsletter
Read articles from CHIRAG KUMAR directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

CHIRAG KUMAR
CHIRAG KUMAR
Full stack Developer