How to Set Up a MERN Stack Project from Scratch

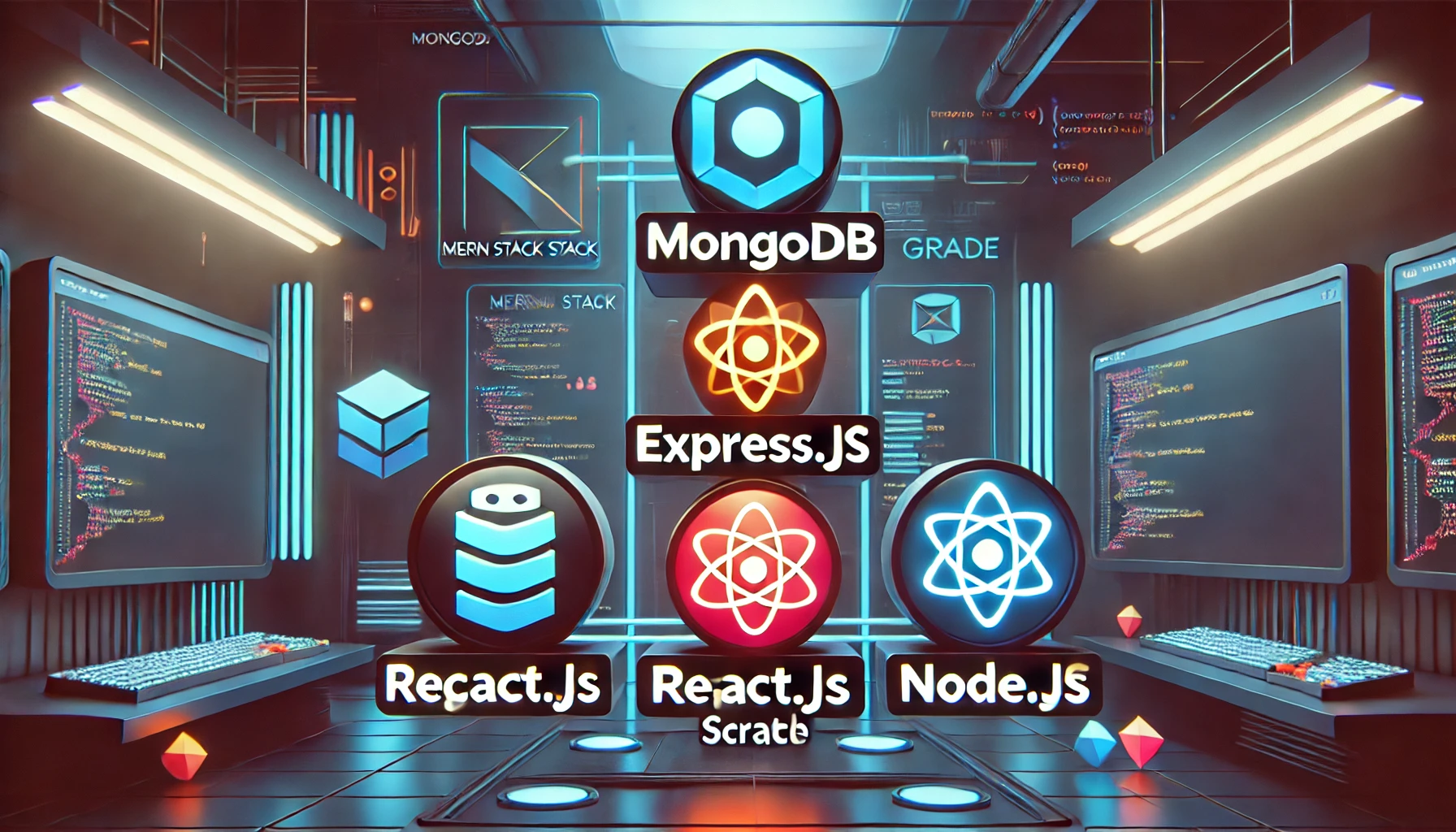
Introduction
The MERN stack (MongoDB, Express.js, React.js, and Node.js) is a popular technology stack for building full-stack web applications. It provides a seamless development experience using JavaScript for both frontend and backend.
In this guide, we’ll cover:
✅ Setting up the backend (Node.js + Express + MongoDB)
✅ Setting up the frontend (React.js)
✅ Connecting both for a fully functional MERN application
1️⃣ Setting Up the Backend (Node.js + Express + MongoDB)
Step 1: Initialize a Node.js Project
First, create a new project folder and initialize a Node.js application:
mkdir mern-project && cd mern-project
npm init -y
This will generate a package.json
file.
Step 2: Install Required Dependencies
Install the backend dependencies:
npm install express mongoose dotenv cors
For development, install Nodemon to restart the server automatically when files change:
npm install --save-dev nodemon
Update package.json
to include a script for running the server:
"scripts": {
"start": "node index.js",
"dev": "nodemon index.js"
}
Step 3: Set Up Express Server
Create a new file index.js
and set up a basic Express server:
const express = require("express");
const mongoose = require("mongoose");
const cors = require("cors");
require("dotenv").config();
const app = express();
app.use(express.json());
app.use(cors());
app.get("/", (req, res) => {
res.send("MERN Backend is running!");
});
const PORT = process.env.PORT || 5000;
app.listen(PORT, () => console.log(`Server running on port ${PORT}`));
Step 4: Connect to MongoDB
Create a .env
file to store environment variables:
MONGO_URI=your_mongodb_connection_string
Update index.js
to connect to MongoDB:
mongoose.connect(process.env.MONGO_URI, {
useNewUrlParser: true,
useUnifiedTopology: true,
})
.then(() => console.log("MongoDB Connected"))
.catch(err => console.error(err));
Run the backend:
npm run dev
Your backend is now live on http://localhost:5000
🎉
2️⃣ Setting Up the Frontend (React.js)
Step 1: Create a React App
In the same project folder, create a frontend React app:
npx create-react-app client
Go into the client
folder and start the app:
cd client
npm start
This will start the React app at http://localhost:3000
.
Step 2: Install Dependencies
Install Axios (for API calls) and React Router (for navigation):
npm install axios react-router-dom
3️⃣ Connecting Frontend & Backend
Step 1: Set Up API Calls in React
Inside the client/src
folder, create a file api.js
to connect the frontend with the backend:
import axios from "axios";
const API_URL = "http://localhost:5000";
export const fetchMessage = async () => {
const response = await axios.get(`${API_URL}/`);
return response.data;
};
Step 2: Update App.js
to Fetch Backend Data
Modify App.js
to display data from the backend:
import React, { useEffect, useState } from "react";
import { fetchMessage } from "./api";
function App() {
const [message, setMessage] = useState("");
useEffect(() => {
fetchMessage().then(data => setMessage(data));
}, []);
return <h1>{message}</h1>;
}
export default App;
Now, when you run the React app, it will display the response from the backend 🎉
4️⃣ Deploying the MERN Stack Application
Deploying the Backend
You can deploy the backend using Render, Railway, or Heroku.
Steps for Render:
Go to Render and create an account.
Create a new Web Service.
Connect your GitHub repository and deploy.
Deploying the Frontend
For frontend deployment, you can use Vercel or Netlify.
Steps for Vercel:
Install Vercel CLI:
npm install -g vercel
Deploy your frontend:
vercel
Once deployed, your MERN stack project is live 🚀
Conclusion
You now have a fully functional MERN stack project running locally! You can start adding authentication, database models, and more features.
🚀 Next Steps:
✅ Add authentication with JWT
✅ Deploy the backend to Render
✅ Deploy the frontend to Vercel
💬 What do you want to learn next? Comment below!
Subscribe to my newsletter
Read articles from Purnendu Ghoshal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
