The Role of wait() and notify()

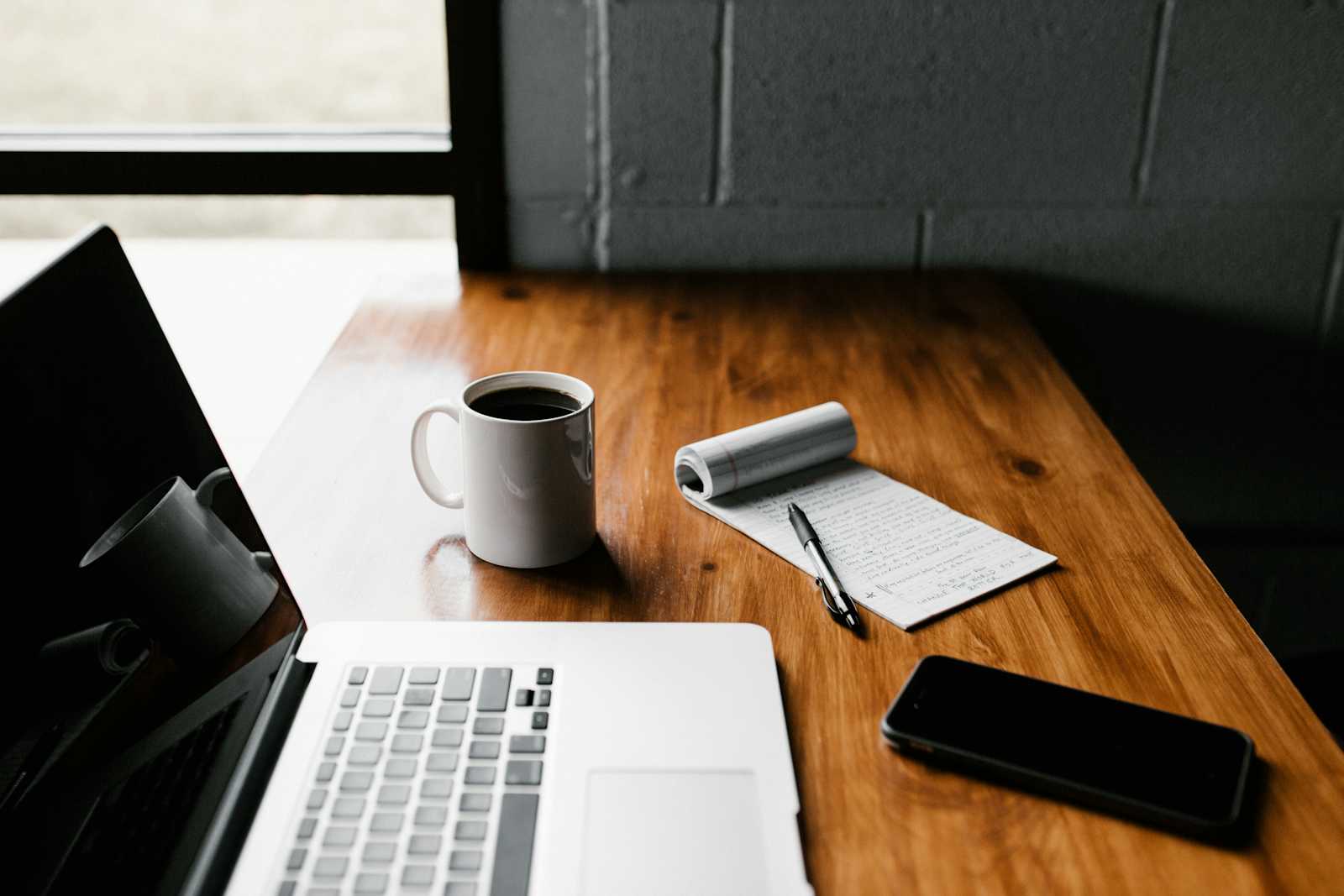
Introduction:
In our Thread LifeCycle blog, we mentioned the wait()
and notify()
methods while discussing the WAITING state of a thread. In today’s blog, we are going to understand these three important methods in detail.
Belongs to:
The wait()
& notify()
methods are defined within the Object class.
Note: The Object class is the parent class of all classes in Java.
Usage:
Now that we know where these three methods belong, let's discuss where and in which situations we can use these methods. All three methods are primarily used for inter-thread communication in a multithreaded environment.
Yes, in certain use cases, threads need to communicate with each other. When one or multiple threads depend on a certain condition, they need to wait until that condition is met before proceeding further.
Let's understand each method in detail with examples!
Remember: We can use these methods only within a synchronized code block.
1. wait()
We use this method when we want one thread to wait for another thread to call notify()
or notifyAll()
on the same object. This is useful in scenarios where one thread depends on the completion of certain actions performed by another thread.
Example:
class SharedResource {
synchronized void waitMethod() {
try {
System.out.println(Thread.currentThread().getName() + " is waiting...");
wait(); // Thread goes into waiting state
System.out.println(Thread.currentThread().getName() + " resumed after being notified.");
} catch (InterruptedException e) {
e.printStackTrace();
}
}
synchronized void notifyMethod() {
System.out.println("Notifying one waiting thread...");
notify(); // Notify a single thread
}
}
2. notify()
After completing the actions on which other threads are dependent, the current thread needs to notify those threads so they can resume execution and come out of the waiting state.
Example:
class NotifyExample {
public static void main(String[] args) {
SharedResource resource = new SharedResource();
Thread t1 = new Thread(resource::waitMethod, "Thread-1");
Thread t2 = new Thread(resource::notifyMethod, "Notifier");
t1.start();
try {
Thread.sleep(1000); // Ensure t1 starts waiting
} catch (InterruptedException e) {
e.printStackTrace();
}
t2.start(); // Notify t1
}
}
If you run the above code snippet, you will see that Thread-1
pauses its execution until the Notifier
thread calls notify()
.
I hope you now have a basic understanding of these methods. In another blog, we will discuss the precautions we must take before using these methods.
Until then, Happy Learning! 🎉
Subscribe to my newsletter
Read articles from Jayita Saha directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
