A Deep Dive into Mandelbrot Fractal Rendering Through Reactive Programming
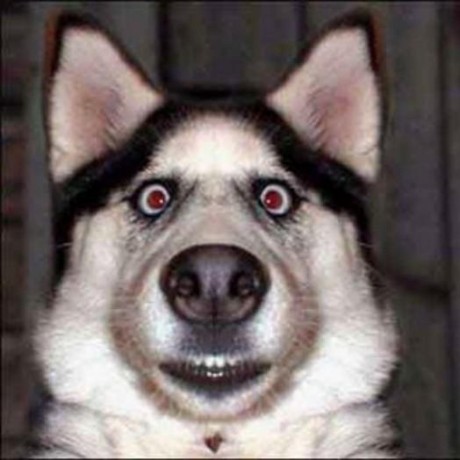
Introduction
In the realm of modern web development, performance and responsiveness are paramount. Today, we'll explore a way to showcase the power of reactive programming, WebSockets, and real-time data visualization through a Mandelbrot fractal generator. A Mandelbrot is a popular fractal, which is a complex mathematical object named after mathematician Benoît Mandelbrot that is generated by iterating a simple quadratic equation on the complex plane. It uses math to draw a pretty picture. Here is the rabbit hole, and result looks something like this:
Architectural Overview
Our application leverages the following tech stack:
Spring Boot: Provides the application framework
Project Reactor: Enables reactive programming
WebSockets: Facilitates real-time, bidirectional communication
HTML5 Canvas: Renders the fractal dynamically
Getting Started
Here is a link to the code base that we will be using. We will be opening a bi-directional channel between the data consumer (the browser) and the data producer (the Mandelbrot calculator) from the client side. Opening the channel will trigger a handler to start the calculation, which will then send individual computed pixels downstream back to the client. The client, upon receiving them, will draw them in an HTML canvas
.
Our Data Source: The Mandelbrot Calculation
The Mandelbrot set is generated by iteratively applying the function z = z² + c to complex numbers, and coloring pixels based on how quickly they diverge to infinity. Wikihow explains this very nicely. Below is a Java implementation. We’ll be performing this calculation for every pixel in an 800×800 grid, so in total 640,000 times.
static String computeMandelbrot(int x, int y) {
// Convert pixel (x, y) to complex number (real and imaginary parts)
double real = X_MIN + (x * (X_MAX - X_MIN) / WIDTH);
double imaginary = Y_MIN + (y * (Y_MAX - Y_MIN) / HEIGHT);
double zr = 0.0; // Z(real) part
double zi = 0.0; // Z(imaginary) part
int iterations = 0;
// Iterate Mandelbrot formula: Z(n+1) = Z(n)^2 + C
while (zr * zr + zi * zi <= 4.0 && iterations < MAX_ITER) {
double temp = zr * zr - zi * zi + real;
zi = 2.0 * zr * zi + imaginary;
zr = temp;
iterations++;
}
// Convert the result to string format for WebSocket transmission
// E.g., "x,y,iterations" for later rendering
return x + "," + y + "," + iterations;
}
Reactive Streams: The Core of Our Application
At the heart of our application lies Project Reactor's reactive programming model, which transforms how we think about data flow and computation.
Reactive Streams became a part of the Java standard library since Java 9 in 2017, and Spring Framework 5 came out shortly after with WebFlux, a reactive, non-blocking web framework. Spring at the time declared their intention to deprecate the popular RestTemplate
, and have been encouraging developers to adopt the reactive paradigm in their work, using WebFlux’s WebClient
. If you are a Spring Boot developer and haven’t yet used Reactive Streams, you will soon.
Let's dip our toes into the reactive concepts that make this application work. We’ll explore what non-blocking means, how Reactive Streams handles concurrency, and take a look into some of its multi-threading capabilities.
Reactive Primer
There are plenty of resources online that will go into the details of how Reactive Streams works, for the purpose of this exercise, I will try to keep it concise. Reactive Streams emits data reactively through a Publisher
, which means it only produces data when it is requested by a Subscriber
. In this exercise, we will be using Flux
, which is an instance of a Publisher. It produces a “stream”, which is a sequence of data elements made available over time.
This is different from the java.util.Stream
feature, which shares similarities in its application but processes finite, synchronous sequences of data and the result is delivered as a one-time event. Even if the streams are set to be multi-threaded, the thread implementing the stream sleeps until it is finished.
Flux<String>: Our Data Stream Powerhouse
The generateData()
method in our Mandelbrot
class is where the reactive magic happens. The computeMandelbrot
method contains a computationally expensive process, determining a single Mandelbrot set point. The resulting sequence of points, defined as a set of x and y coordinates and a number representing iterations for that point, will be returned in a String wrapped by the Flux
. No calculations will happen until a subscriber subscribes to it.
Think of this Flux
like a dynamic conveyor belt of data, where each pixel's Mandelbrot set iterations are computed independently and, as we’ll discuss further, often concurrently.
public static Flux<String> generateData() {
return Flux.range(0, HEIGHT)
.flatMap(y -> Flux.range(0, WIDTH)
.map(x -> computeMandelbrot(x, y))
);
}
Let’s examine at what is happening here. HEIGHT
and WIDTH
are both set to 800. Flux.range
will produce a sequence of integers commensurate to the range.
Transformation Functions
As you can see in the code, one sequence (the x coordinate) is defined in a function that is chained to the other sequence (the y coordinate). This results in the x coordinates wrapped inside the series of y coordinates, eg. [y1[x1, x2, x3, ...], y2[x1, x2, x3], ... ]
.
A couple of transformation functions take these results, and transform them into usable data:
FlatMap
flattens that to[[y1, x1], [y1, x2], ..., [y2, x1], [y2, x2], ...]
.Map
takes each coordinate set produced byFlatMap
and passes them tocomputeMandelBrot
, which returns them in a String representation of a list that includes both coordinates and the number of iterations (from thecomputeMandelbrot
code snippet above:
return x + "," + y + "," + iterations;
Because the range
was produced by the Flux
object, any transformations applied to the sequence of integers remains wrapped by the Flux
. This includes the returning String, the final step of the transformations created by the chain of functions. This String gets returned to the WebSocketHandler
class’s handle
method.
WebFlux WebSocket Streaming
A Quick WebSocket Primer
WebSocket is a bi-directional communication protocol that works over standard HTTP channels, and uses HTTP to establish a connection through an Upgrade header, that is requested by the client and used by the server to change protocols from HTTP to WebSocket. Instead of using a open connection → send request → receive response → response -> close connection
paradigm like HTTP, WebSocket keeps the connection open and both sides can send and receive messages at any time. It provides live updates for all kinds of scenarios: observability, financial trading, live tracking, chat applications, gaming, etc.
How We Use WebSockets In This Example
The WebSocket handler transforms the returned String into a format that the WebSocketSession
can understand. From examining the Spring code, you can see that session.send(…)
accepts a Publisher<WebSocketMessage> messages
parameter. Flux
is a child class of Publisher
, and session::textMessage
takes a String as a parameter and transforms it into a WebSocketMessage
object.
The .then()
function chained to the .send()
closes the connection after the send()
has completed. Until then, the WebSocket channel remains open and a receiver method can also be implemented to receive messages from the client, but that is a story for another day.
public Mono<Void> handle(WebSocketSession session) {
return session.send(
Mandelbrot.generateData()
.map(session::textMessage)
)
.then();
}
The WebSocket channel is created by the client, in this scenario the web browser, using the WebSocket library:
const socket = new WebSocket("ws://localhost:8080/ws/mandelbrot");
Side-note
On the server side, a HandlerMapping
instance is created, which listens to the /ws/mandelbrot
path on HTTP and maps the request to the handler.
@Configuration
public class WebSocketConfig {
@Bean
public HandlerMapping webSocketHandlerMapping(MandelbrotWebSocketHandler mandelbrotWebSocketHandler) {
// Map the WebSocket endpoint "/ws/mandelbrot" to the handler
return new SimpleUrlHandlerMapping(Map.of("/ws/mandelbrot", mandelbrotWebSocketHandler), 10);
}
}
This is everything you need to connect to a Reactive Stream through WebSockets.
Final Details About This Implementation
I mentioned above that a Publisher
only produce data when it is requested by a Subscriber
. While Flux<String> is a Publisher, you may notice that nothing is subscribed to it in the code. That’s because in this context, the subscription happens when a WebSocket client opens a connection. If you look at the Spring code that handles the open connection, you’ll see the .subscribe
. The Spring Framework WebFlux’s implementation of WebSockets handles it.
Mono<Void>
in the handler represents a promise of a completed asynchronous action. It:
Signals the completion of the WebSocket data transmission;
Enables non-blocking, reactive communication to handle the entire data stream without blocking system resources.
What is Mono Anyway?
Mono
is another Publisher. It differs from Flux
in that while Flux
handles a sequence of data elements, from 0 to N limited by your system’s resource, Mono
accepts a stream of 0 to 1 data elements. A simplified way of looking at it is like the reactive alternative to java.util.Optional
.
Non-Blocking Calls: Breaking Free from Synchronous Constraints
What is Non-Blocking Calls?
Non-blocking calls is a programming paradigm that allows a program to continue executing other tasks while waiting for expensive operations such as intense computations or input/output operations to complete, rather than stopping and waiting for each operation to finish.
How Non-Blocking Actually Works
Uses event loops and callback mechanisms
Leverages asynchronous programming models
Implements promises/futures for result handling
May harness thread pooling for efficient execution
Traditional Blocking Model: The Traffic Jam Analogy
Imagine a traditional blocking I/O operation or computationally expensive process like a single-lane road:
[Program Start]
↓
[I/O Operation]
↓
WAIT
↓
[Next Task]
When an I/O operation or expensive process starts (like reading a file or network request), everything else STOPS
The entire application thread is essentially frozen
Other potential tasks are queued and cannot proceed
Resources are inefficiently utilized
Non-Blocking Model: The Multi-Lane Highway
[Program Start]
↓
[I/O Operation Initiated]
↓
[Continue Other Tasks]
↓
[I/O Callback/Completion Handler]
Key Characteristics:
Initiates I/O operation or expensive process
IMMEDIATELY continues with other tasks
Receives notification when operation or process is complete
Maximizes CPU and system resource utilization
Practical Example in Our Mandelbrot Application
Let's examine how non-blocking works in our fractal viewer:
public static Flux<String> generateData() {
return Flux.range(0, HEIGHT)
.flatMap(y -> Flux.range(0, WIDTH)
.map(x -> computeMandelbrot(x, y))
// Non-blocking: each computation can happen concurrently
);
}
Non-Blocking Benefits Here:
Concurrent Computation: Each pixel can be calculated independently
No Waiting: If one pixel computation is slow, others continue
Efficient Resource Use: Can utilize multiple CPU cores
Responsive Application: The rest of the application remains interactive during rendering
Reactive Programming Connection
In reactive programming (like our Mandelbrot viewer):
Non-blocking is a fundamental principle
Streams can be processed concurrently
Backpressure mechanisms prevent system overload
Enables responsive, efficient applications
Potential Drawbacks
Non-blocking processes aren't a silver bullet:
More complex programming model
Requires careful error handling
Can introduce overhead for simple, quick operations
Steeper learning curve
Summing Up Non-Blocking Calls
Non-blocking calls represent a paradigm shift in how we think about computational resources. It's not just a technical implementation, but a philosophy of efficient, responsive computing.
By understanding and implementing non-blocking techniques, developers can create more scalable, responsive, and efficient applications.
Reactive Streams: Concurrency in Action
Granular thread controls can be added to our Mandelbrot renderer that demonstrate these concepts:
public static Flux<String> generateData() {
return Flux.range(0, HEIGHT)
.flatMap(y -> Flux.range(0, WIDTH)
.map(x -> computeMandelbrot(x, y))
// Parallel execution magic happens here
.subscribeOn(Schedulers.parallel())
);
}
Parallel Threading: Increase Performance
The Bottleneck in Single-Threaded Processing
In a single-threaded WebSocket implementation, data generation and transmission would occur sequentially:
Compute a Mandelbrot point
Prepare the WebSocket message
Send the message
Repeat for each point
This approach leads to:
Increased latency
Reduced throughput
Inefficient CPU utilization
Parallel Threading Transformation
By using subscribeOn(Schedulers.parallel())
, we introduce a game-changing approach:
public static Flux<String> generateData() {
return Flux.range(0, HEIGHT)
.flatMap(y -> Flux.range(0, WIDTH)
.map(x -> computeMandelbrot(x, y))
.subscribeOn(Schedulers.parallel())
);
}
Parallel Thread Utilization
In this scenario, we are:
Creating a thread pool optimized for parallel processing;
Allowing simultaneous computation of multiple Mandelbrot points;
Telling it to apply the thread pool to all the processes in the stream.
Scheduler Types Explained
Schedulers provide thread pools for worker threads. While there are several different types of Schedulers, the most commonly used ones are Schedulers.parallel()
and Schedulers.boundedElastic()
.
Schedulers.parallel()
Optimized for non-blocking computational work;
By default, creates a pool of threads equal to the number of CPU cores;
Ideal for CPU-intensive tasks like fractal computation.
Schedulers.boundedElastic()
Creates threads on-demand
Prevents thread exhaustion
Useful for operations that might block, e.g. I/O-bound tasks
How Schedulers Are Invoked
The code uses Flux.subscribeOn
. This applies the parallel Scheduler to operations defined in the entire chain of functions, starting from directly after the Flux
is defined.
Another way of applying a thread pool to the reactive operations is by using Flux.publishOn
. This will apply the thread pool to all the operations downstream, e.g.:
public static Flux<String> generateData() {
return Flux.range(0, HEIGHT)
.map(x -> notUsingThreadPool(x))
.publishOn(Schedulers.parallel())
.map(x -> usingThreadPool(x))
}
Concrete Performance Benefits
Consider our 800x800 pixel Mandelbrot visualization:
Total points to compute: 640,000;
Without parallelism: Sequential processing;
- With
subscribeOn(Schedulers.parallel())
: Parallel processing reduces the total compute time by the number of threads. you can see in the visual examples that the screen is painted in much broader strokes in the multithreaded example below.
A note about these .GIF examples
Performance Metrics: Tracking the Reactive
The client-side JavaScript implements performance tracking:
Key Metrics Monitored
Frames Per Second (FPS): Rendering performance
Data Throughput: WebSocket events per second
Processing Time: Average time per data point
Error Tracking: Connection reliability
Performance Calculation Mechanism
function showMetrics() {
const elapsedTime = (performance.now() - startTime) / 1000;
const fps = (frameCounter / elapsedTime).toFixed(2);
const dataRate = (dataCounter / elapsedTime).toFixed(2);
const avgProcessingTime = dataCounter > 0
? (processingTime / dataCounter).toFixed(2)
: 0;
updateMetricsDisplay(fps, dataRate, avgProcessingTime, errorCount);
}
Challenges and Learnings
Reactive Programming Insights
Thinking in streams, not traditional sequential logic
Embracing asynchronous, non-blocking paradigms
Managing complex data transformations elegantly
Future Enhancements
Implement interactive zooming
Add custom color scheme generation
Explore more complex fractal algorithms
Implement dynamic rendering parameters
Conclusion
This Mandelbrot fractal viewer is more than a visualization tool—it's a demonstration to the power of reactive programming. By combining Spring Boot, WebSockets, and Project Reactor, we've created a high-performance, real-time data streaming application. These same concepts can be used in much more business-critical applications.
Key Takeaways
Reactive streams enable powerful, concurrent computations
WebSockets provide real-time, efficient communication
Resources and Further Learning
Happy reactive coding! 🚀📊🔬
Subscribe to my newsletter
Read articles from Steve Meckman directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
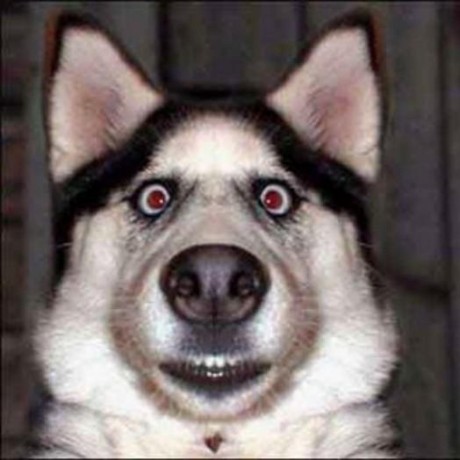