Understanding Time Complexity and the Impact of console.log on Node.js Server Performance

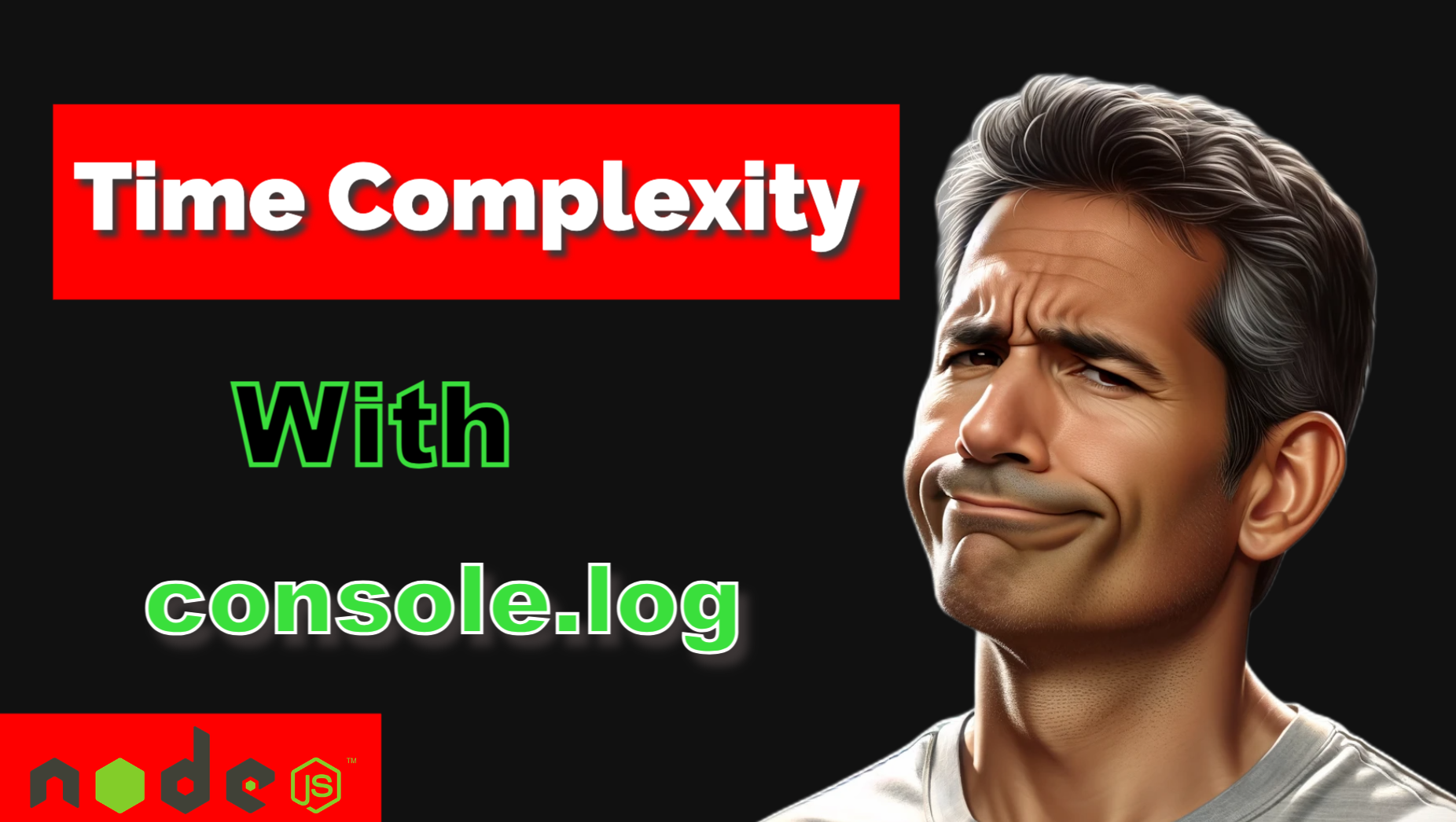
When building applications in Node.js, developers often focus on functionality and correctness, but performance optimization is equally critical—especially for server-side applications where efficiency directly impacts user experience and resource usage. One often-overlooked factor that can subtly degrade performance is the use of console.log
. While it’s a handy debugging tool, excessive or unnecessary use of console.log
in a Node.js server can increase runtime and introduce inefficiencies. In this article, we’ll explore time complexity, how console.log
affects Node.js performance, and why you should avoid unnecessary logging in production environments.
What is Time Complexity?
Time complexity is a concept from computer science that describes the amount of time an algorithm takes to complete as a function of the size of its input. It’s typically expressed using Big O notation (e.g., O(n), O(n²), O(1)), which provides an upper bound on the growth rate of the algorithm’s execution time.
O(1): Constant time – the execution time doesn’t change regardless of input size.
O(n): Linear time – the execution time grows proportionally with the input size.
O(n²): Quadratic time – the execution time grows exponentially with the input size.
In the context of a Node.js server, time complexity helps us understand how certain operations—like loops, database queries, or I/O tasks—affect the overall runtime of a request. Even small, seemingly innocuous operations can add up, especially under high load.
How console.log Works in Node.js
In Node.js, console.log
is a synchronous operation that writes output to the standard output stream (stdout
), typically the terminal or a log file. While it’s invaluable for debugging during development, its synchronous nature means it blocks the event loop—the heart of Node.js’s single-threaded, non-blocking architecture—until the operation completes.
Here’s a breakdown of what happens when you call console.log
:
Serialization: The argument passed to
console.log
(e.g., a string, object, or array) is converted to a string. For complex objects, this involves JSON serialization, which can be computationally expensive.I/O Operation: The serialized output is written to
stdout
. This involves interaction with the operating system’s I/O subsystem, which is inherently slower than in-memory operations.Blocking: Since
console.log
is synchronous, the Node.js event loop pauses until the write operation finishes, delaying the execution of other tasks (e.g., handling incoming requests).
While a single console.log
might seem trivial, its impact compounds in scenarios with high frequency (e.g., inside loops) or high concurrency (e.g., a busy server handling thousands of requests per second).
The Performance Impact on a Node.js Server
Let’s consider a practical example to illustrate how console.log
affects server runtime.
Imagine a simple Express.js server that processes a list of items:
const express = require('express');
const app = express();
app.get('/process', (req, res) => {
const items = Array.from({ length: 1000 }, (_, i) => i); // 1000 items
for (let item of items) {
console.log(`Processing item: ${item}`);
}
res.send('Done');
});
app.listen(3000, () => console.log('Server running on port 3000'));
In this code:
The
/process
endpoint iterates over 1,000 items.For each item,
console.log
is called, serializing the string and writing it tostdout
.
On a typical machine, writing to stdout
might take a few microseconds per call. For 1,000 iterations, this could add several milliseconds to the request’s runtime. While milliseconds might not sound like much, consider the following scenarios:
High Load: If the server handles 1,000 requests per second, those extra milliseconds accumulate, reducing throughput and increasing latency.
Larger Data: If
console.log
is used to output large objects (e.g., a JSON response), serialization time increases, amplifying the delay.Production Logging: In production,
stdout
might be redirected to a file or a logging service, introducing additional I/O overhead.
Now, compare this to the same code without console.log
:
app.get('/process', (req, res) => {
const items = Array.from({ length: 1000 }, (_, i) => i);
for (let item of items) {
// No console.log
}
res.send('Done');
});
Without console.log
, the loop executes purely in memory, completing in microseconds rather than milliseconds. This difference becomes critical in performance-sensitive applications.
Time Complexity of console.log
While console.log
itself is an O(1) operation (constant time per call), its actual runtime depends on:
Input Size: Logging a large object requires more time for serialization than a simple string.
Frequency: Using
console.log
inside an O(n) loop results in O(n) total logging time.Environment: Writing to a terminal is faster than writing to a file or network stream, but both are slower than skipping the operation entirely.
In essence, unnecessary console.log
statements introduce a hidden cost that scales with usage, turning an otherwise efficient algorithm into a slower one.
Why Avoid Unnecessary console.log in Production?
Performance Overhead: As demonstrated,
console.log
increases runtime by blocking the event loop and performing I/O. In a high-traffic server, this can lead to bottlenecks.Resource Usage: Writing to
stdout
consumes CPU and I/O resources, which could be better allocated to handling requests or performing business logic.Scalability Issues: Under heavy load, excessive logging can overwhelm the system, filling disk space (if redirected to files) or slowing down external logging services.
Security Risks: Logging sensitive data (e.g., user inputs or API keys) to
stdout
can accidentally expose it, especially if logs aren’t properly managed.Cluttered Output: Unnecessary logs make it harder to spot critical information during debugging or monitoring.
Best Practices for Logging in Node.js
Instead of relying on console.log
, adopt these strategies:
Use a Logging Library: Libraries like
winston
orpino
offer asynchronous logging, configurable levels (e.g., info, debug, error), and better performance. For example:const winston = require('winston'); const logger = winston.createLogger({ level: 'info', transports: [new winston.transports.File({ filename: 'app.log' })], }); logger.info('Processing item');
Conditional Logging: Enable logging only during development or when explicitly needed:
const isDev = process.env.NODE_ENV === 'development'; if (isDev) console.log('Debug info');
Remove Debugging Logs: Strip
console.log
statements from production code using tools likebabel-plugin-transform-remove-console
.Monitor Performance: Profile your server with tools like
clinic.js
or Node.js’s built-in--inspect
flag to identify logging-related bottlenecks.
Conclusion
Time complexity is a fundamental lens through which we evaluate code efficiency, and even small operations like console.log
can tip the scales in a Node.js server. While it’s a great tool for development, unnecessary use in production introduces performance overhead, blocks the event loop, and hampers scalability. By understanding its impact and adopting better logging practices, you can ensure your Node.js server runs faster, uses resources efficiently, and scales seamlessly under load. So, the next time you’re tempted to sprinkle console.log
throughout your code, think twice—your server’s performance might thank you!
Subscribe to my newsletter
Read articles from Mohit Uchit directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Mohit Uchit
Mohit Uchit
I'm a passionate Backend Engineer with 3 years of experience, specializing in building scalable and efficient systems. I work extensively with Node.js, Express.js, and REST APIs to create robust server-side applications. My database expertise includes MySQL, MongoDB, and Sequelize, while I leverage Redis for caching and optimization. I’m a strong advocate for microservices architecture, designing solutions that are modular and resilient. Through this blog, I share insights, tutorials, and tips from my journey in backend development. Let’s connect and build something amazing together!