State Management in Jetpack Compose: Best Practices ๐

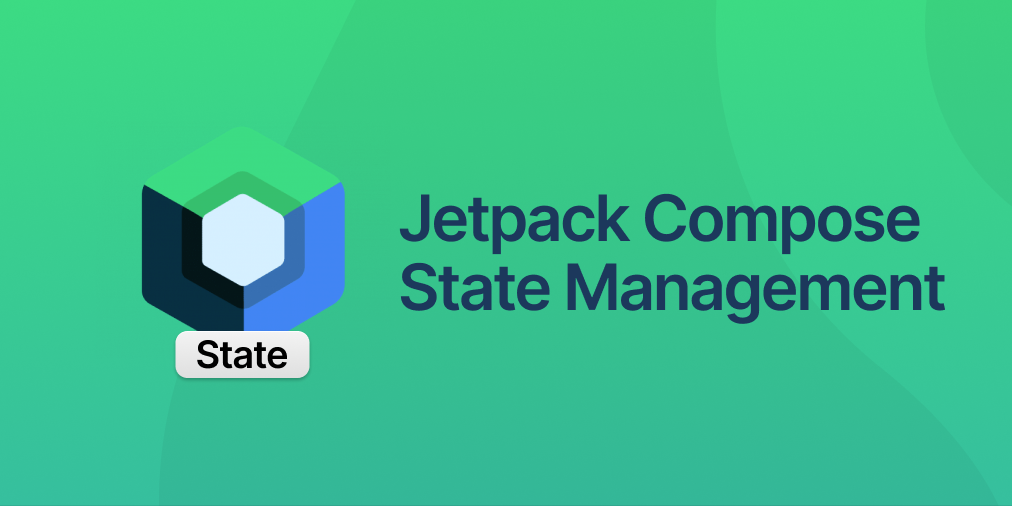
Managing UI state is a fundamental part of Android development, and with Jetpack Compose, it becomes easier and more efficient. However, misusing state can lead to unnecessary recompositions, performance issues, and complex debugging.
Understanding State in Jetpack Compose
State in Compose follows a declarative UI approach, meaning the UI is derived from state. This makes state management a crucial part of building stable and efficient applications.
Key Practices for Effective State Management
โ
Use remember
to retain state across recompositions
โ
Use rememberSaveable
to persist state across configuration changes
โ
Lift state to a ViewModel
for better separation of UI and business logic
Example: Counter App with ViewModel
class CounterViewModel : ViewModel() {
private val _count = MutableStateFlow(0)
val count: StateFlow<Int> = _count
fun increment() {
_count.value += 1
}
}
@Composable
fun CounterScreen(viewModel: CounterViewModel = viewModel()) {
val count by viewModel.count.collectAsState()
Button(onClick = { viewModel.increment() }) {
Text("Count: $count")
}
}
๐ More Resources:
๐ Jetpack Compose State Docs
๐ State Hoisting in Jetpack Compose
๐ก How do you handle state in Compose? Share your thoughts below!
Subscribe to my newsletter
Read articles from Arjun Yadav directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
