Unit – I Basic of Python data structure - Dictionary, Tuple and Set
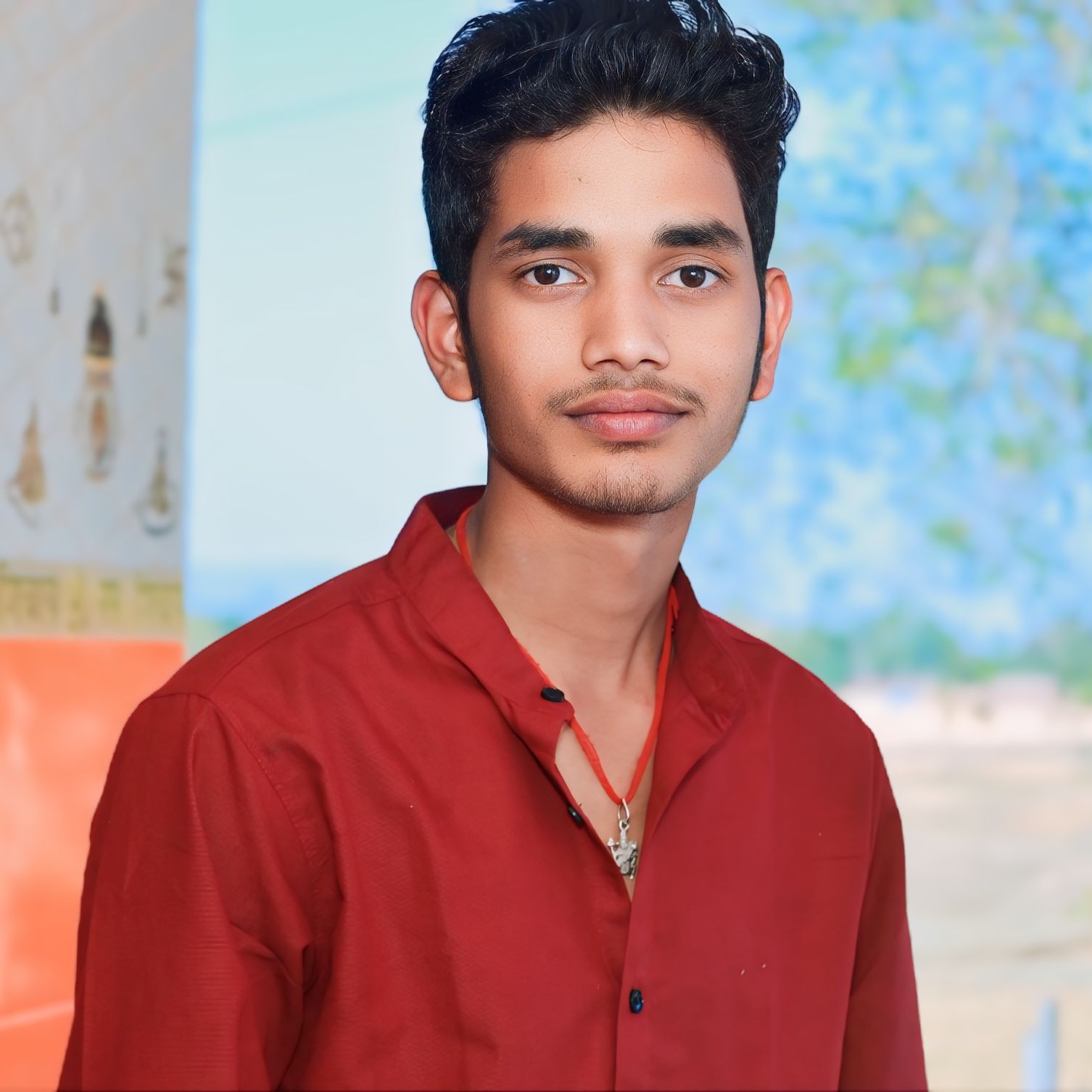
https://www.python.org/
for more content you can refer python documentation..
Use of String and list operations in Python programs.
In Python, strings and lists are two of the most commonly used data types. They are both versatile and can be manipulated in various ways. Below is an introduction to both, along with examples of their operations.
Strings
A string is a sequence of characters enclosed in quotes (single, double, or triple quotes). Strings are immutable, meaning that once they are created, their contents cannot be changed.
In other words
A string is a sequence of characters enclosed in quotes (' ' or " "). Strings are immutable, meaning they cannot be changed once created.
Common String Operation
1. Creating a String:
my_string = "Hello, World!"
2. Accessing Characters: You can access individual characters in a string using indexing.
first_char = my_string[0] # 'H'
last_char = my_string[-1] # '!'
3. Slicing: You can extract a substring using slicing.
substring = my_string[0:5] # 'Hello'
4. String Length: You can find the length of a string using the len() function.
length = len(my_string) # 13
5. String Methods: Strings come with various built-in methods.
upper_string = my_string.upper() # 'HELLO, WORLD!'
lower_string = my_string.lower() # 'hello, world!'
replaced_string = my_string.replace("World", "Python") # 'Hello, Python!'
6. Concatenation: You can concatenate strings using the + operator.
new_string = my_string + " How are you?" # 'Hello, World! How are you?'
Example of String Operations
# Example: String Manipulation
greeting = "Hello, World!"
print(greeting) # Output: Hello, World!
# Accessing characters
print(greeting[7]) # Output: W
# Slicing
print(greeting[0:5]) # Output: Hello
# String length
print(len(greeting)) # Output: 13
# String methods
print(greeting.upper()) # Output: HELLO, WORLD!
print(greeting.replace("World", "Python")) # Output: Hello, Python!
LIST
A list in Python is a built-in data structure used to store multiple items in a single variable. It is ordered, mutable (modifiable), and allows duplicate values. Lists can store different types of data, such as integers, strings, and even other lists.
Key Features of Lists:
Ordered – Items have a defined sequence.
Mutable – You can modify elements after creation.
Allows Duplicates – Can contain multiple occurrences of the same value.
Can Store Different Data Types – Integers, strings, floats, and even other lists.
Common List Operations
- Creating a List:
my_list = [10, 20, 30, "apple", 3.14]
print(my_list) # Output: [10, 20, 30, 'apple', 3.14]
2. Accessing List Elements:
Elements in a list can be accessed using indexing (starts from 0).
print(my_list[0]) # Output: 10
print(my_list[-1]) # Output: 3.14 (Last element)
3. Modifying List Elements:
Lists are mutable, meaning elements can be changed.
my_list[1] = 50
print(my_list) # Output: [10, 50, 30, 'apple', 3.14]
4. Adding Elements to a List :
(a) Using append() (Adds to the end)
my_list.append("banana")
print(my_list) # Output: [10, 50, 30, 'apple', 3.14, 'banana']
(b) Using insert() (Adds at a specific index)
my_list.insert(2, "grape")
print(my_list) # Output: [10, 50, 'grape', 30, 'apple', 3.14, 'banana']
(c) Using extend() (Merging another list)
new_list = [100, 200]
my_list.extend(new_list)
print(my_list) # Output: [10, 50, 'grape', 30, 'apple', 3.14, 'banana', 100, 200]
5. Removing Elements from a List
(a) Using remove() (Removes first occurrence of a value)
my_list.remove("apple")
print(my_list) # Output: [10, 50, 'grape', 30, 3.14, 'banana', 100, 200]
(b) Using pop() (Removes by index, returns the removed item)
removed_item = my_list.pop(2)
print(removed_item) # Output: grape
print(my_list) # Updated list
(c) Using clear() (Removes all elements)
my_list.clear()
print(my_list) # Output: []
6. List Slicing
Extracts a portion of the list.
numbers = [1, 2, 3, 4, 5, 6, 7]
print(numbers[1:5]) # Output: [2, 3, 4, 5]
print(numbers[:4]) # Output: [1, 2, 3, 4]
print(numbers[3:]) # Output: [4, 5, 6, 7]
7. Looping Through a List
(a) Using for loop
fruits = ["apple", "banana", "cherry"] for fruit in fruits:
print(fruit)
(b) Using while loop
i = 0
while i < len(fruits):
print(fruits[i])
Set
● Sets in Python
● Create a Set, Accessing Python Sets, Delete from sets, Update sets
● Python Set Operations
Sets in Python
A set is an unordered collection of unique elements in Python. Sets are mutable, meaning you can add or remove elements after the set has been created. They are useful for membership testing, removing duplicates from a sequence, and performing mathematical operations like unions and intersections.
Creating a Set
You can create a set using curly braces {} or the set() constructor.
Example:
- Creating a set using curly braces
my_set = {1, 2, 3, 4, 5}
print(my_set) # Output: {1, 2, 3, 4, 5}
- Creating a set using the set() constructor
another_set = set([1, 2, 3, 4, 5])
print(another_set) # Output: {1, 2, 3, 4, 5}
Accessing Python Sets
Sets are unordered, so you cannot access elements by index like you would with lists or tuples. However, you can iterate through the elements of a set.
Example:
my_set = {1, 2, 3, 4, 5}
# Iterating through a set
for element in my_set:
print(element)
Deleting from Sets
You can remove elements from a set using the remove(), discard(), or pop() methods.
remove(element): Removes the specified element from the set. Raises a KeyError if the element is not found.
discard(element): Removes the specified element from the set if it exists. Does not raise an error if the element is not found.
pop(): Removes and returns an arbitrary element from the set. Raises a KeyError if the set is empty.
Example:
my_set = {1, 2, 3, 4, 5}
# Using remove()
my_set.remove(3)
print(my_set) # Output: {1, 2, 4, 5}
# Using discard()
my_set.discard(2)
print(my_set) # Output: {1, 4, 5}
# Using pop()
popped_element = my_set.pop()
print(popped_element) # Output: 1 (or another element, since sets are unordered)
print(my_set) # Output: {4, 5}
Updating Sets
You can add elements to a set using the add() method or update a set with multiple elements using the update() method.
add(element): Adds a single element to the set.
update(iterable): Adds multiple elements from an iterable (like a list or another set) to the set.
Example :
my_set = {1, 2, 3}
# Using add()
my_set.add(4)
print(my_set) # Output: {1, 2, 3, 4}
# Using update()
my_set.update([5, 6])
print(my_set) # Output: {1, 2, 3, 4, 5, 6}
Python Set Operations
Sets support various mathematical operations, such as union, intersection, difference, and symmetric difference.
Union (|): Combines two sets, returning a new set with all unique elements from both sets.
Example :
set_a = {1, 2, 3}
set_b = {3, 4, 5}
union_set = set_a | set_b
print(union_set) # Output: {1, 2, 3, 4, 5}
- Intersection (&): Returns a new set with elements that are common to both sets.
Example:
intersection_set = set_a & set_b
print(intersection_set) # Output: {3}
- Difference (-): Returns a new set with elements in the first set that are not in the second set.
Example :
difference_set = set_a - set_b
print(difference_set) # Output: {1, 2}
- Symmetric Difference (^): Returns a new set with elements in either set, but not in both.
Example :
symmetric_difference_set = set_a ^ set_b
print(symmetric_difference_set) # Output: {1, 2, 4, 5}
- Subset and Superset:
issubset(): Checks if one set is a subset of another.
issuperset(): Checks if one set
Tuples
A tuple is a built-in data structure in Python that is used to store an ordered collection of items. Tuples are similar to lists, but they have some key differences, primarily that they are immutable, meaning that once a tuple is created, its contents cannot be changed.
A tuple in Python is an immutable and ordered collection of elements. Here are its key features:
1. Immutable
Once created, tuples cannot be changed (no adding, removing, or modifying elements).
This makes them hashable and suitable for use as dictionary keys.
2. Ordered
- Elements are stored in a fixed order, meaning you can access them using indexing (tuple[index]).
3. Allows Duplicate Values
- Unlike sets, tuples can contain duplicate elements ((1, 2, 2, 3)).
4. Can Store Multiple Data Types
- A tuple can hold different types of data (e.g., ("hello", 42, 3.14, True)).
5. Supports Indexing & Slicing
Access elements via indexing (my_tuple[0]).
Creating Tuples
Tuples can be created by placing a comma-separated sequence of items inside parentheses (). Here are a few examples:
# An empty tuple
empty_tuple = ()
# A tuple with one item (note the comma)
single_item_tuple = (1,)
# A tuple with multiple items
multi_item_tuple = (1, 2, 3, "hello", 4.5)
You can also create a tuple without parentheses by just using commas:
another_tuple = 1, 2, 3
Accessing Tuples
You can access the elements of a tuple using indexing, which starts at 0.
**Iterate Over Tuples :-**You can iterate over the elements of a tuple using a for loop:
for item in multi_item_tuple:
print(item)
Slicing Tuples:- Slicing allows you to access a subset of a tuple. You can use the colon : operator to specify the start and end indices:
# Slicing from index 1 to 3 (exclusive)
sliced_tuple = multi_item_tuple[1:3] # Output: (2, 3)
Tuples are Immutable
One of the defining characteristics of tuples is that they are immutable. This means that once a tuple is created, you cannot modify its contents (i.e., you cannot add, remove, or change elements).
For example:
# This will raise a TypeError
multi_item_tuple[0] = 10 # TypeError: 'tuple' object does not support item assignment
Subscribe to my newsletter
Read articles from Sagar Sangam directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
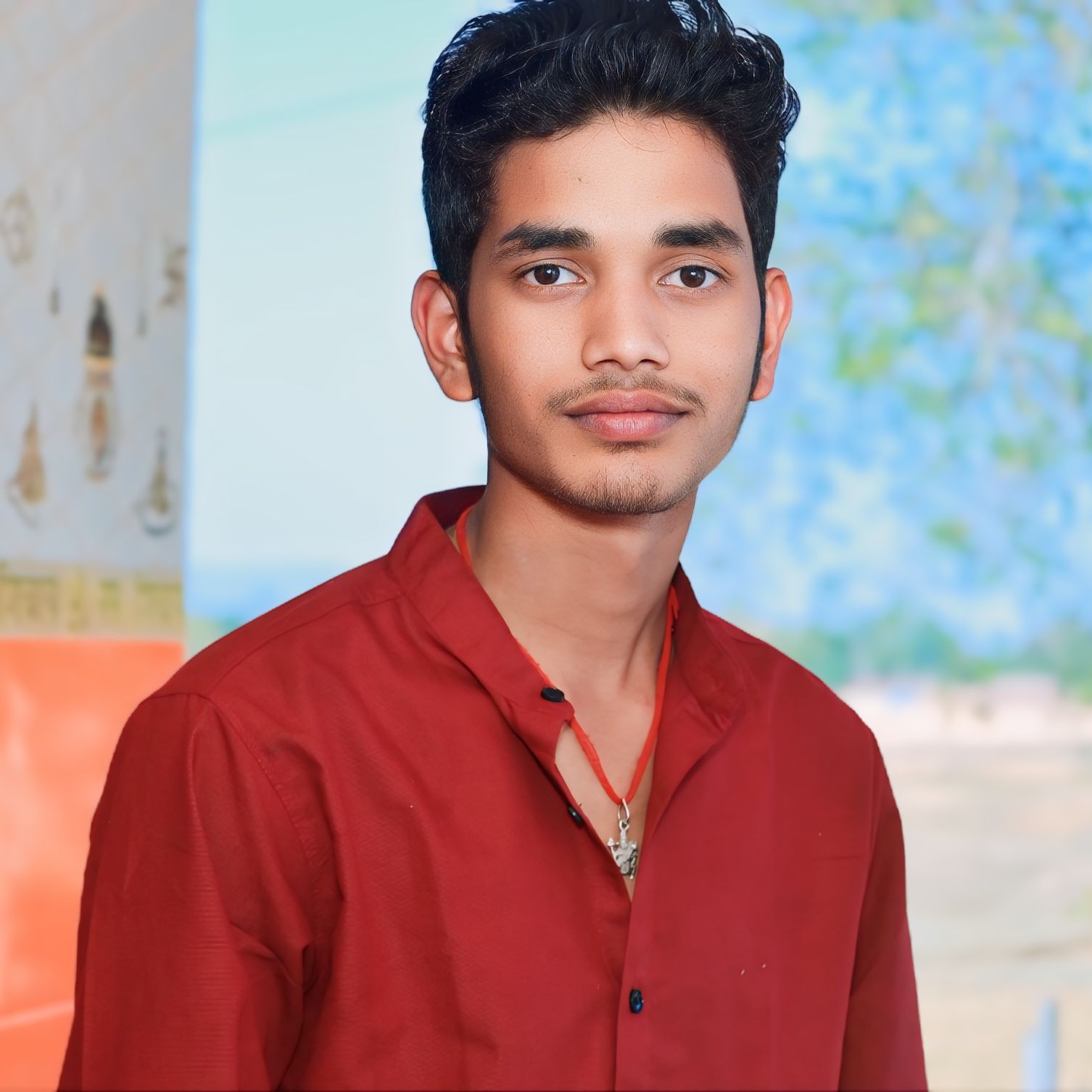