Frontend Development Best Practices: Lessons from My Experience

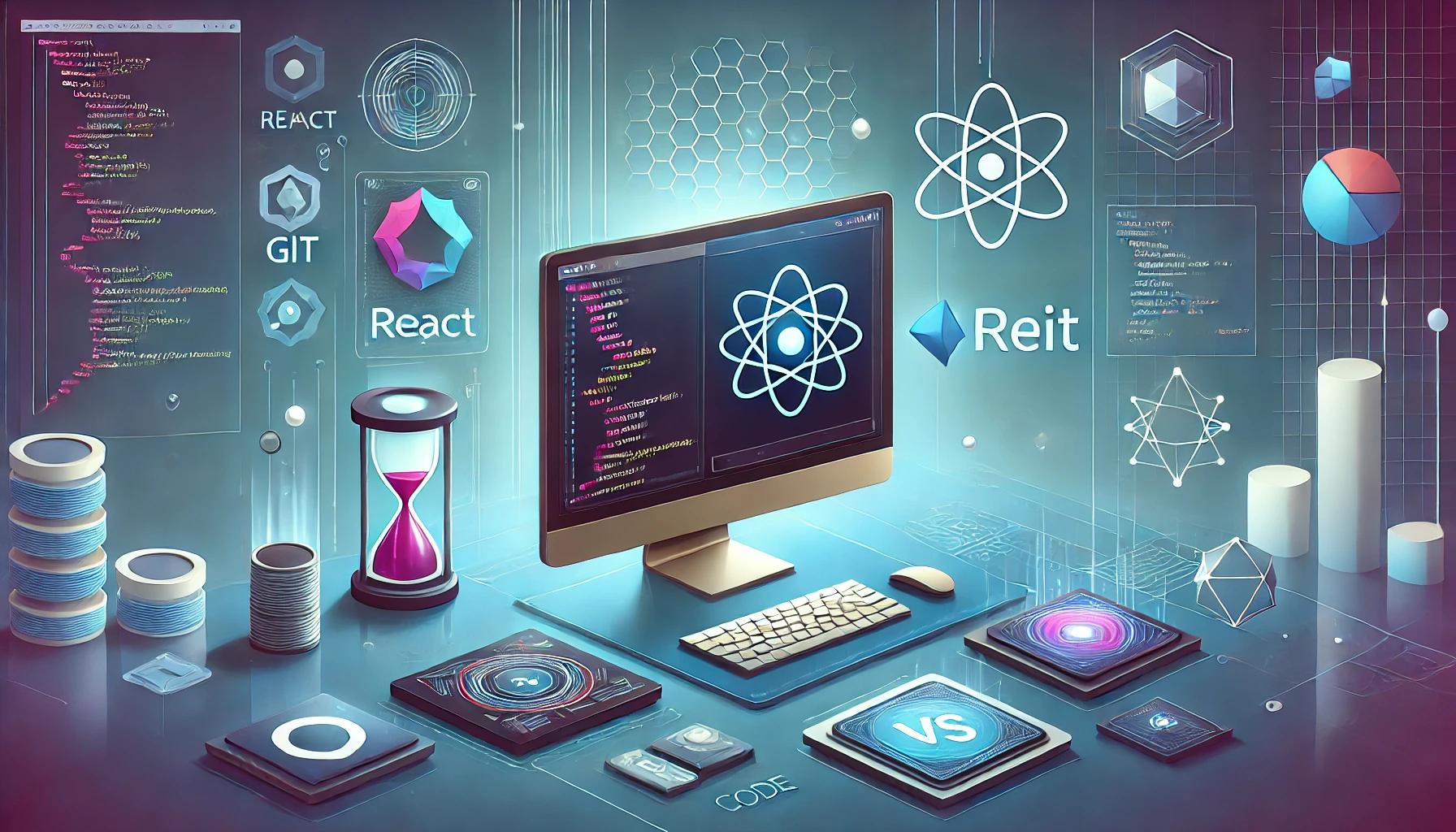
As a frontend developer, I have had the opportunity to guide and mentor junior developers in my company. Through this experience, I’ve compiled a structured set of best practices that help maintain consistency, code quality, and efficiency in frontend development. This blog aims to share these insights with a broader audience, helping developers at all levels improve their workflow.
1. Git Standards: Keeping Code Organized
Follow clear branching conventions like
feature/
,bugfix/
, andhotfix/
.Commit messages should be descriptive and formatted properly (e.g.,
feat: added login feature
).Always pull the latest changes before pushing your own updates.
Use pull requests (PRs) for reviews before merging.
Learn to handle merge conflicts efficiently.
2. Structuring a React Project for Scalability
A well-structured React project improves maintainability:
Organize code into
components/
,pages/
,hooks/
,utils/
, etc.Primitive Components: Simple reusable UI elements like
Button
,Input
,Typography
.Feature Components: Specific UI components such as
LoginForm
,UserProfile
.Layout Components: Elements like
Header
,Sidebar
,Grid
.Container Components: Manage business logic and data fetching.
3. Is Environment Configuration Needed for Frontend?
Yes, using .env
files helps manage API keys and environment-based configurations securely. For example:
REACT_APP_API_URL=https://api.example.com
Ensure sensitive data is never exposed on the frontend.
4. Selecting the Right Frontend Libraries
Use popular and well-maintained libraries with at least 100K+ weekly downloads.
Check for deprecated packages before using.
Read documentation and GitHub issue lists for lesser-known packages.
5. Code Quality with SonarQube
Install SonarLint in VS Code for real-time analysis.
Follow SonarQube rules to avoid code smells and maintain best practices.
6. Automating Code Formatting with ESLint and Prettier
Use Git hooks to format and lint code before committing.
Example using
husky
:
npx husky-init && npm install
7. Daily Code Pushes: Avoid Holding Code
Push code daily to avoid conflicts and lost progress.
If an issue isn't complete, push to a separate feature branch.
8. Knowing When to Ask for Help
Try solving an issue for 20 minutes to 2 hours before seeking help.
If stuck, check docs, Stack Overflow, and logs, then ask seniors.
9. Code Review and Peer Collaboration
Always submit pull requests (PRs) for review.
At least one peer review is necessary before merging code.
Code reviews help improve code quality and knowledge sharing.
10. Writing Test Cases & Following TDD
Write unit tests for components and functions.
Example with Jest & React Testing Library:
test("renders button", () => {
render(<Button text="Click me" />);
expect(screen.getByText("Click me")).toBeInTheDocument();
});
- Follow Test-Driven Development (TDD).
11. Learn React Before Writing HTML
Understand JSX, components, state, and props before writing raw HTML.
Avoid hardcoded HTML without React logic.
12. Structuring & Reusing Components Effectively
Avoid overcomplicating reusable components.
Determine if reusability increases technical debt.
Reuse only when necessary.
13. Importance of a Design System
Follow Figma designs precisely before coding.
Use predefined styles, colors, and components to maintain UI consistency.
14. Understanding Project Dependencies
Check package versions, deprecations, and compatibility.
Learn how Webpack, Vite, or Next.js bundling works.
15. Keeping Sensitive Data in the Backend
Anything on the frontend can be seen by users.
Store sensitive data only on the backend.
16. Working with Storybook
Storybook helps develop UI components in isolation.
Use it to test reusable components efficiently.
17. Essential VS Code Extensions
Code highlighting, IntelliSense, Emmet abbreviations.
Learn how AI tools can assist in development.
18. Agile Workflow & Estimations
Learn ticketing tools like Jira, Redmine.
Give realistic estimates based on effort and complexity.
19. Proper Documentation
Use comments, README files, and inline documentation.
Document major changes and complex logic.
20. Using Modern React Tools
Prefer Vite over Create React App for better performance.
Use Next.js for SSR, but remember Helmet for SEO.
21. Mock APIs When Backend is Not Ready
Use tools like Mock API, MockTurtle, MockAPI.io.
Avoid blocking frontend development due to missing backend endpoints.
22. Learning TypeScript
TypeScript reduces bugs and enhances scalability.
Adopt TypeScript gradually in new projects.
Example:
type User = {
name: string;
age: number;
};
Conclusion
These best practices have helped shape the frontend development process in my company. By following these guidelines, developers can improve code quality, team collaboration, and overall efficiency. Whether you're a junior developer or an experienced one, these principles will help you write cleaner, maintainable, and scalable frontend code.
Support My Work
If you found this guide on DevOps helpful and would like to support my work, consider buying me a coffee! Your support helps me continue creating beginner-friendly content and keeping it up-to-date with the latest in tech. Click the link below to show your appreciation:
I appreciate your support and happy learning! ☕
Subscribe to my newsletter
Read articles from akash javali directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

akash javali
akash javali
A passionate 'Web Developer' with a Master's degree in Electronics and Communication Engineering who chose passion as a career. I like to keep it simple. My goals are to focus on typography, and content and convey the message that you want to send. Well-organized person, problem solver, & currently a 'Senior Software Engineer' at an IT firm for the past few years. I enjoy traveling, watching TV series & movies, hitting the gym, or online gaming.