Setting Up Headless Ghost CMS for Seamless Vercel Integration: A Detailed Tutorial

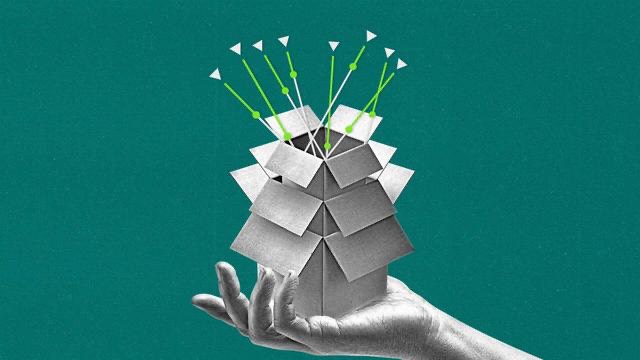
Transitioning to a Headless Ghost CMS architecture offers significant advantages in flexibility, performance, and scalability, especially when paired with modern frontend frameworks hosted on platforms like Vercel. This setup allows Ghost to function purely as a content backend, delivering data via its robust Content API, while Vercel handles the build, deployment, and scaling of your frontend application (e.g., built with Next.js, Nuxt.js, SvelteKit, or Astro).
This guide provides a comprehensive walkthrough of configuring your Ghost instance for headless operation and preparing your Vercel environment to connect to it securely and efficiently.
Prerequisites
Before proceeding, ensure you have the following:
A Running Ghost Instance: This can be self-hosted, running locally via Docker, or using Ghost(Pro). Crucially, this instance must be accessible over the network from where Vercel's build processes run (i.e., the public internet, unless using Vercel specific network configurations).
Admin Access to Your Ghost Instance: You need administrator privileges to create API integrations.
A Frontend Project: Your website's codebase (e.g., a Next.js application) ready to consume the Ghost Content API.
A Vercel Account: Sign up for a free or paid Vercel account.
Git Repository: Your frontend project should be hosted on a Git provider supported by Vercel (GitHub, GitLab, Bitbucket).
Part 1: Configuring Your Ghost CMS Instance for Headless Operation
The first phase involves setting up Ghost to expose its content via the API.
Step 1: Access Your Ghost Admin Panel
Log in to the administration interface of your Ghost installation. This is typically found at http://your-ghost-domain.com/ghost/
.
Step 2: Navigate to Integrations
In the Ghost admin sidebar menu, click on "Settings" and then select "Integrations".
Step 3: Create a New Custom Integration
Scroll down to the "Custom Integrations" section.
Click the "Add custom integration" button.
Give your integration a descriptive name. We recommend naming it clearly, such as "Vercel Frontend" or "Next.js Site".
Click "Create".
Step 4: Obtain Your Content API Key and API URL
Once the integration is created, you will be presented with crucial credentials:
Content API Key: This key is used by your frontend application to authenticate requests to the Ghost Content API. Treat this key like a password; keep it secure and do not expose it directly in your frontend codebase.
Admin API Key: This key grants write access. You do not need this for a standard headless setup fetching content. Ensure you are using the Content API Key.
API URL: This is the base URL for your Ghost instance's API endpoints. It is typically your main Ghost site URL (e.g.,
https://your-ghost-domain.com
).
Action: Carefully copy the Content API Key and note down your API URL. You will need these later for your frontend application and Vercel configuration.
Step 5: Ensure Ghost Instance Accessibility (Crucial for Vercel)
For Vercel to build your frontend application (especially during static generation like getStaticProps
in Next.js), its build servers need to be able to reach your Ghost API URL over the internet.
If using Ghost(Pro): Your API URL is inherently public.
If self-hosting:
Ensure your Ghost instance is running on a server with a publicly accessible domain name or IP address.
Check your firewall rules (e.g.,
ufw
, security groups on AWS/GCP/Azure) to ensure that incoming connections on the port Ghost is running on (usually port 2368 for HTTP or 443 for HTTPS if behind a reverse proxy like Nginx) are allowed, at least from Vercel's IP ranges if necessary, though allowing general public access is more common for APIs.Ensure your web server (like Nginx) is correctly configured to proxy requests to the Ghost process if you are using one.
Verification: Try accessing your API URL
directly in a browser or using a tool like curl
from a machine outside your local network. You should typically get a response related to Ghost. For example, curl
https://your-ghost-domain.com/ghost/api/content/settings/?key=YOUR_CONTENT_API_KEY
should return your site settings in JSON format.
Part 2: Configuring Your Frontend Application
Now, configure your frontend project (we will use Next.js examples, but the principles apply broadly) to connect to the Ghost API using the credentials obtained.
Step 6: Install the Ghost Content API Client
We recommend using the official JavaScript library provided by Ghost for easier interaction with the API.
npm install @tryghost/content-api
# or
yarn add @tryghost/content-api
# or
pnpm add @tryghost/content-api
Step 7: Set Up API Client Initialization Using Environment Variables
It is critical to use environment variables to store your Ghost API credentials, preventing them from being hardcoded into your source code.
Create
.env.local
file: In the root of your frontend project, create a file named.env.local
(this file should be listed in your.gitignore
file to prevent committing sensitive keys).Add Variables: Add your Ghost API URL and Content API Key to this file:
# .env.local GHOST_API_URL=https://your-ghost-domain.com GHOST_CONTENT_API_KEY=your_copied_content_api_key NEXT_PUBLIC_GHOST_API_URL=https://your-ghost-domain.com # Optional: If needed client-side
Note on
NEXT_PUBLIC_
prefix: In Next.js, environment variables prefixed withNEXT_PUBLIC_
are exposed to the browser. Only add this if you absolutely need to make API calls directly from the client-side (less common for fetching primary content). Your Content API Key should almost never be exposed client-side.*Initialize the API Client: Create a utility file (e.g.,
lib/ghost.js
orservices/ghost.js
) to initialize and export the API client instance:// lib/ghost.js import GhostContentAPI from '@tryghost/content-api'; const api = new GhostContentAPI({ url: process.env.GHOST_API_URL, key: process.env.GHOST_CONTENT_API_KEY, version: "v5.0" // Specify your desired Ghost API version }); export default api;
Step 8: Implement Data Fetching in Your Application
Use the initialized api
object within your framework's data fetching methods to retrieve content.
Example (Next.js
getStaticProps
):// pages/index.js (or similar) import api from '../lib/ghost'; export async function getStaticProps() { try { const posts = await api.posts.browse({ limit: 10, include: 'tags,authors' // Include related data }); const settings = await api.settings.browse(); return { props: { posts, settings }, revalidate: 60 // Optional: Revalidate static pages every 60 seconds (ISR) }; } catch (error) { console.error("Error fetching Ghost data:", error); // Handle error appropriately, maybe return an error prop or notFound return { props: { posts: [], settings: null, error: true } }; } } // Your page component using the fetched props... export default function HomePage({ posts, settings }) { // Render posts and use settings... return ( <div> {/* ... your page content ... */} </div> ); }
Part 3: Deploying Your Frontend to Vercel
With Ghost configured and your frontend application ready to fetch data, you can now deploy to Vercel.
Step 9: Prepare Your Project for Vercel
Ensure your project code, including the API client setup (but excluding .env.local
), is committed and pushed to your Git repository (GitHub, GitLab, Bitbucket).
Step 10: Import Your Project into Vercel
Log in to your Vercel dashboard.
Click "Add New..." -> "Project".
Select the Git repository containing your frontend application. Vercel will usually automatically detect the framework (e.g., Next.js).
Step 11: Configure Environment Variables in Vercel
This is where you provide Vercel with the necessary credentials to connect to your Ghost instance during the build process and potentially at runtime.
In the "Configure Project" screen in Vercel (or later via Project Settings -> Environment Variables), locate the "Environment Variables" section.
Add the following variables:
Name:
GHOST_API_URL
Value:https://your-ghost-domain.com
(Use the publicly accessible URL of your Ghost instance)Name:
GHOST_CONTENT_API_KEY
Value:your_copied_content_api_key
(Paste the key you obtained from Ghost)(Optional) If you used
NEXT_PUBLIC_GHOST_API_URL
, add that variable here as well.
Ensure these variables are available in the Production, Preview, and Development environments as needed by your setup. For build-time variables like these, Production is usually sufficient, but including them in Preview is good practice.
Crucially, ensure the
GHOST_API_URL
is the one Vercel's build servers can reach. If your Ghost instance is only available locally (http://localhost:2368
), Vercel cannot connect to it during the build.
Step 12: Configure Build Settings (If Necessary)
Vercel is excellent at detecting settings for popular frameworks like Next.js. Review the "Build & Development Settings" section:
Framework Preset: Should be detected (e.g., "Next.js").
Build Command: Usually
next build
or similar.Output Directory: Typically
.next
for Next.js.Install Command:
npm install
,yarn install
, orpnpm install
.
Adjust these only if Vercel's defaults are incorrect for your project structure.
Step 13: Deploy
Click the "Deploy" button. Vercel will:
Clone your repository.
Install dependencies.
Inject the environment variables you configured.
Run the build command (e.g.,
next build
). During this step, if using SSG (getStaticProps
), Vercel will execute your data fetching functions, making calls to yourGHOST_API_URL
using theGHOST_CONTENT_API_KEY
.Deploy the built output to Vercel's global edge network.
Part 4: Verification and Essential Post-Deployment Steps
Step 14: Verify the Connection
Once deployed, access your Vercel application URL (e.g., your-project.vercel.app
or your custom domain). Verify that content from your Ghost instance is being displayed correctly. Check the Vercel deployment logs for any build errors, particularly any related to fetching data from the Ghost API (e.g., connection timeouts, authentication errors).
Step 15: Configure CORS (Cross-Origin Resource Sharing) - IMPORTANT
If your frontend application needs to make requests to the Ghost API directly from the user's browser (client-side fetching), you must configure CORS settings in your Ghost instance. If all data fetching happens server-side (like in getStaticProps
/getServerSideProps
), this might not be strictly necessary, but it is good practice to configure it.
Locate
config.<environment>.json
: Access the configuration file for your Ghost environment (e.g.,config.production.json
) on the server where Ghost is hosted.Add
cors
block: Within theurl
setting block, add acors
block specifying the allowed origins.// config.production.json (example snippet) { "url": "https://your-ghost-domain.com", "server": { // ... other server settings ... }, "database": { // ... database settings ... }, "mail": { // ... mail settings ... }, "logging": { // ... logging settings ... }, "paths": { // ... paths settings ... }, "privacy": { // ... privacy settings ... }, // Add or modify this block: "cors": { "origin": "https://your-vercel-app-domain.com, https://*.vercel.app" // Replace with your actual production domain and Vercel preview domains // Use "true" to allow all origins (less secure, not recommended for production) } }
Restart Ghost: After modifying the configuration file, you must restart the Ghost process for the changes to take effect (
ghost restart
if using Ghost-CLI).
Step 16: Consider Webhooks for Content Updates (ISR/On-Demand Revalidation)
If you are using Static Site Generation (SSG) with Next.js (getStaticProps
) and Incremental Static Regeneration (ISR) (revalidate
option), or On-Demand Revalidation, you can significantly improve content freshness by setting up Ghost webhooks.
In Ghost Admin: Go to Settings -> Integrations -> Add webhook.
Event: Choose events like
post.published
,page.published
,post.updated
,page.updated
, etc.Target URL: Provide a Vercel Deploy Hook URL or a custom API route in your Next.js application designed to trigger revalidation for specific paths (
res.revalidate('/path-to-update')
). This instantly updates relevant static pages on Vercel without waiting for therevalidate
timer.
Step 17: Security Considerations Review
API Key Security: Never commit your
GHOST_CONTENT_API_KEY
to your Git repository. Rely solely on environment variables provided securely through the Vercel dashboard.Network Access: Restrict access to your Ghost instance's server/port if possible, although the API endpoint itself needs to be reachable by Vercel.
CORS: Configure CORS restrictively to only allow your known frontend domains.
You have now successfully configured Headless Ghost CMS to work seamlessly with your frontend application deployed on Vercel. This setup leverages the strengths of both platforms, providing a powerful content management experience and a highly performant, scalable frontend delivery mechanism.
Ready to build or migrate to a powerful Headless CMS solution? Tenten.co offers expert services in developing bespoke digital platforms using modern technologies like Headless Ghost, Next.js, and Vercel. We help businesses create fast, scalable, and engaging web experiences.
Book a free meeting with Tenten.co to discuss how we can elevate your digital strategy.
Subscribe to my newsletter
Read articles from Erik Chen directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
