What you are not told about frameworks

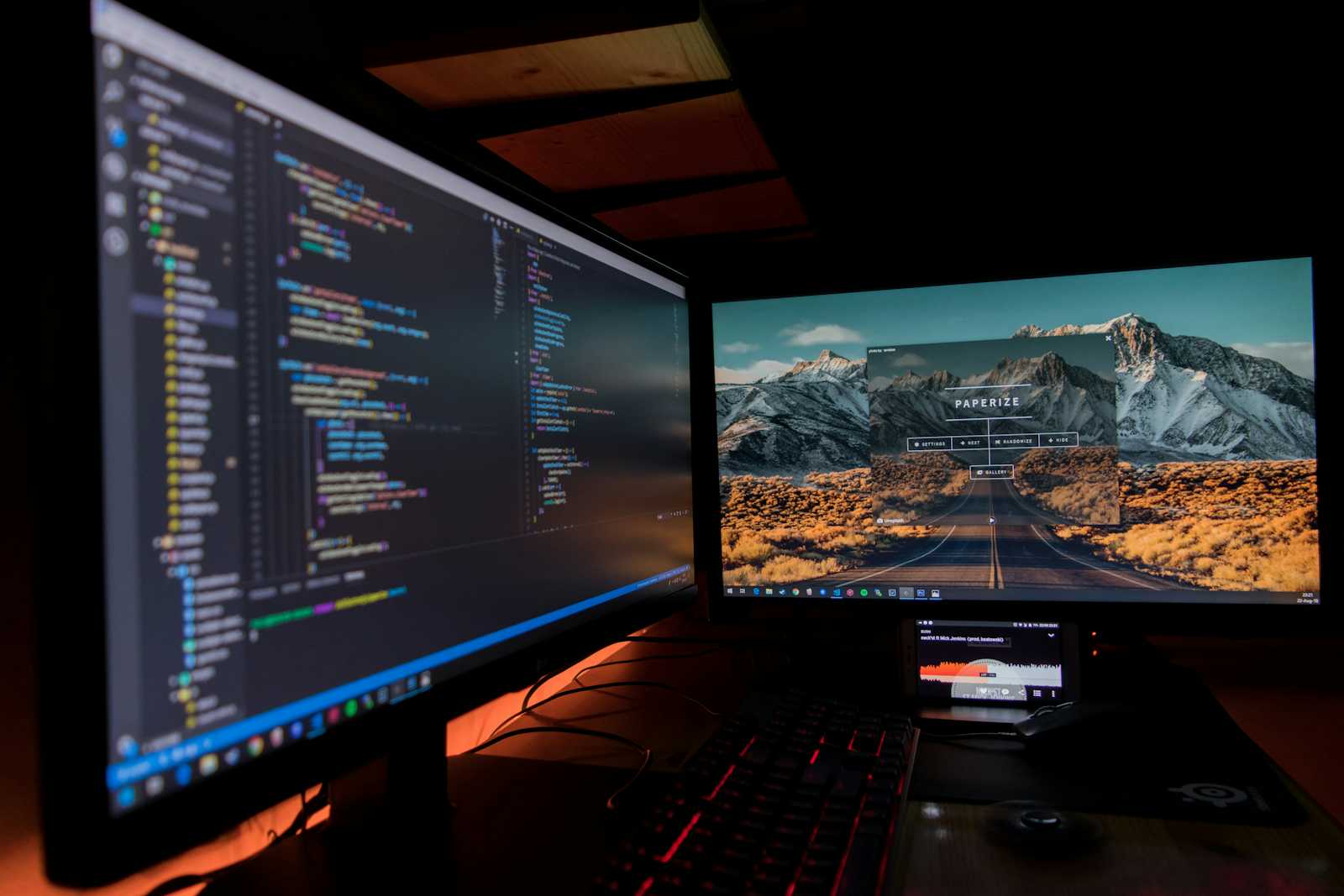
Frameworks are powerful tools that speed up development by providing reusable components and enforcing best practices. However, over-relying on them can introduce hidden risks, from performance issues to security vulnerabilities. In this article, we’ll explore these dangers with practical examples and discuss when a framework might be unnecessary.
1. Performance Overhead
Many frameworks come with built-in features that may not be needed for every project, leading to unnecessary processing.
Example: Raw PHP vs Laravel for a Simple Query
Laravel (Eloquent ORM)
// Fetch a user by email
$user = User::where('email', 'test@example.com')->first();
This query is simple, but Laravel loads the entire ORM stack, adding overhead.
Plain PHP (PDO)
$pdo = new PDO('mysql:host=localhost;dbname=mydb', 'user', 'password');
$stmt = $pdo->prepare("SELECT * FROM users WHERE email = ?");
$stmt->execute(['test@example.com']);
$user = $stmt->fetch(PDO::FETCH_ASSOC);
This raw query is faster and avoids unnecessary framework overhead.
2. Limited Customization & Flexibility
Frameworks impose design patterns that may not fit every project’s requirements.
Example: Custom Authentication vs Laravel Authentication
Laravel Authentication
Auth::attempt(['email' => $email, 'password' => $password]);
Laravel’s authentication system is convenient, but what if you need a non-standard hashing method?
Custom Authentication in PHP
$hash = hash('sha256', $password);
$query = $pdo->prepare("SELECT * FROM users WHERE email = ? AND password_hash = ?");
$query->execute([$email, $hash]);
$user = $query->fetch(PDO::FETCH_ASSOC);
This provides full control over password hashing without framework constraints.
3. Security Risks
Using frameworks without understanding their security mechanisms can be dangerous.
Example: Laravel CSRF Token Dependence
Laravel automatically provides CSRF protection, but a developer unaware of this might disable it incorrectly.
Route::post('/submit-form', [FormController::class, 'submit'])->withoutMiddleware(['VerifyCsrfToken']);
Disabling CSRF without proper measures can expose the application to attacks.
On the other hand, a manually implemented CSRF protection ensures understanding and control:
session_start();
$_SESSION['csrf_token'] = bin2hex(random_bytes(32));
This avoids blind reliance on the framework.
4. Bloated Codebase
Frameworks include unnecessary libraries, increasing application size.
Example: A Simple Static Page
React (Overkill for Static Content)
import React from 'react';
function App() {
return <h1>Hello, World!</h1>;
}
export default App;
This requires loading React’s entire runtime just to display text.
Pure HTML & CSS (Lighter & Faster)
<!DOCTYPE html>
<html>
<head><title>Hello</title></head>
<body>
<h1>Hello, World!</h1>
</body>
</html>
This loads instantly without any dependencies.
5. Harder Debugging
Frameworks abstract many processes, making debugging difficult.
Example: Laravel Query Debugging Issue
$users = User::all();
If an error occurs, Laravel may throw a generic exception. Debugging the actual SQL query requires enabling query logging.
DB::enableQueryLog();
$users = User::all();
$log = DB::getQueryLog();
print_r($log);
With plain SQL, debugging is straightforward.
echo "SELECT * FROM users";
6. Version Conflicts & Compatibility Issues
Frameworks evolve, and updates can break existing code.
Example: Laravel 5 vs Laravel 6 Authentication Changes
// Laravel 5
Auth::routes();
In Laravel 6, developers had to manually add authentication routes, causing issues for older applications.
When to Avoid Over-Reliance on Frameworks
Small Projects: A simple contact form does not need a full-fledged framework.
Performance-Critical Applications: High-speed APIs may perform better with raw PHP or Go.
Long-Term Projects: Custom-built solutions provide more control over future upgrades.
Conclusion
Frameworks are great for rapid development, but over-relying on them can lead to performance, security, and maintainability issues. Understanding the underlying technologies helps developers make informed decisions about when to use frameworks and when to go with custom solutions.
Would you rather have control over your application, or let the framework dictate how you build it? The choice is yours.
Subscribe to my newsletter
Read articles from Douglas Sabwa Indumwa directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Douglas Sabwa Indumwa
Douglas Sabwa Indumwa
I am a full-stack software developer driven by the goal of creating scalable solutions to automate business processes. Throughout my career, I have successfully developed web applications that serve thousands of users, both for-profit and non-profit. I am currently focusing on expanding my skills in DevSecOps.